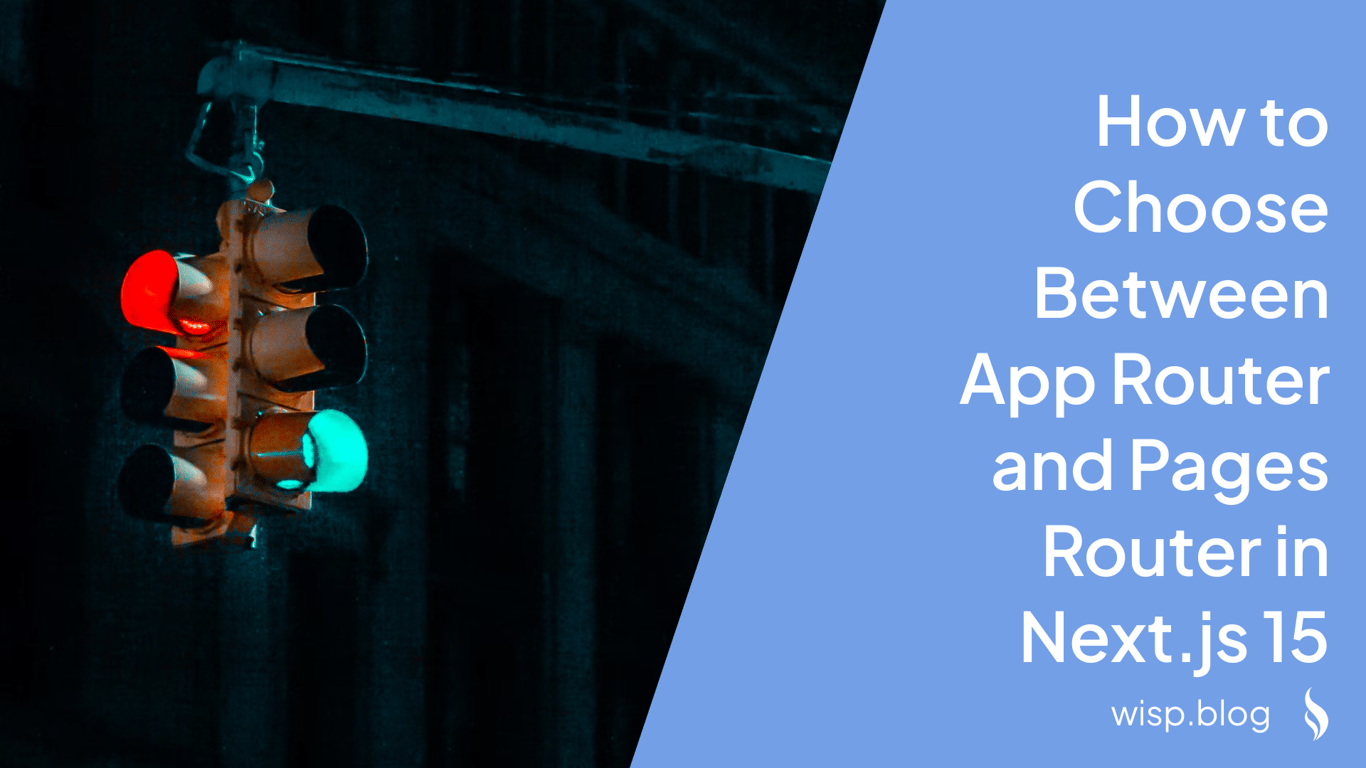
Are you wrestling with the decision between Next.js App Router and Pages Router? You're not alone. Many developers find themselves caught between the modern features of App Router and the battle-tested stability of Pages Router, especially when SEO is a crucial consideration.
In this comprehensive guide, we'll help you make an informed decision that aligns with your project needs while ensuring optimal search engine visibility.
Understanding the Core Differences
Next.js offers two distinct routing systems: the newer App Router and the traditional Pages Router. While both serve the same fundamental purpose of handling navigation in your application, they differ significantly in their approach and capabilities.
App Router: The Modern Approach
The App Router represents Next.js's vision for the future of web development. It leverages React's latest features and introduces several modern concepts:
Server Components by Default: Components are server-rendered unless explicitly marked with 'use client'
Nested Layouts: More flexible layout management with shared UI elements
Streaming with Suspense: Built-in support for streaming server-side rendering
Advanced Caching: More granular control over data caching strategies
Pages Router: The Proven Solution
The Pages Router has been the backbone of Next.js applications for years, offering:
File-system Based Routing: Simple and intuitive routing based on files in the
pages
directoryAutomatic Static Optimization: Built-in static page generation for improved performance
API Routes: Straightforward backend API creation within your Next.js application
Stable and Well-documented: Years of production testing and community knowledge
SEO Implications: A Critical Consideration
One of the most pressing concerns when choosing between routers is their impact on SEO. Let's address this head-on:
App Router SEO Considerations
The App Router introduces Server Components as the default, which is excellent for SEO as it ensures content is readily available to search engine crawlers. However, some developers have expressed concerns about the 'use client' directive:
"Concern that marking components with 'use client' leads to client-side rendering, negatively impacting SEO," as noted in recent community discussions.
The good news? These concerns are largely unfounded. Even with client components, the App Router maintains strong SEO capabilities through:
Hybrid Rendering: Server-side rendering for initial page load, regardless of client components
Metadata API: Built-in tools for managing SEO metadata
Automatic Static Optimization: Static generation of pages where possible
Pages Router SEO Benefits
The Pages Router has proven its SEO effectiveness through:
Predictable Server-Side Rendering: Clear distinction between SSR and client-side rendering
Built-in Head Management: Easy metadata management with
next/head
Static Generation: Automatic static optimization for better crawling
Making the Right Choice: Key Decision Factors
Choose App Router When:
Building a New Project
You're starting fresh without legacy constraints
You want to leverage the latest React features
Your team is ready to learn new concepts
Need Advanced Features
Nested layouts are crucial for your UI
You require streaming server-side rendering
Complex data fetching patterns are necessary
Long-term Investment
You're building for the future
Performance optimization is a top priority
You want access to the latest Next.js features
Stick with Pages Router When:
Maintaining Existing Projects
You have a stable application that works well
Your team is familiar with the current setup
Time-to-market is crucial
Prioritizing Stability
You need proven, production-tested solutions
Your project has strict stability requirements
You want to avoid potential migration issues
Simple Application Needs
Your routing requirements are straightforward
You don't need nested layouts
Basic SSR and static generation suffice
Practical Implementation Tips
Optimizing SEO in App Router
To ensure optimal SEO performance with App Router, follow these best practices:
Implement Root Layout SEO
// app/layout.tsx
import { Metadata } from 'next'
export const metadata: Metadata = {
title: {
template: '%s | Your Site Name',
default: 'Your Site Name',
},
description: 'Your site description',
robots: {
index: true,
follow: true,
},
}
export default function RootLayout({
children,
}: {
children: React.ReactNode
}) {
return (
<html lang="en">
<body>{children}</body>
</html>
)
}
Strategic Use of Client Components
// Keep your page.tsx server-side
export default async function Page() {
return (
<main>
<h1>Server Component</h1>
<ClientInteractivity />
</main>
)
}
// Separate interactive elements into client components
'use client'
function ClientInteractivity() {
return <button onClick={() => console.log('clicked')}>Click me</button>
}
Optimizing SEO in Pages Router
For Pages Router implementations, consider:
Consistent Metadata Management
// pages/_app.tsx
import Head from 'next/head'
function MyApp({ Component, pageProps }) {
return (
<>
<Head>
<title>Your Site Name</title>
<meta name="description" content="Your site description" />
</Head>
<Component {...pageProps} />
</>
)
}
export default MyApp
Leverage getStaticProps for SEO
// pages/blog/[slug].tsx
export async function getStaticProps({ params }) {
const post = await getPost(params.slug)
return {
props: {
post,
// Add metadata for SEO
metadata: {
title: post.title,
description: post.excerpt,
},
},
}
}
Community Insights and Real-world Experience
The Next.js community has shared valuable insights about both routing systems. According to recent discussions, many developers express concerns about App Router's stability in production environments.
Common Challenges and Solutions
App Router Challenges
Stability Issues: Some developers report unexpected behavior in production
Learning Curve: New concepts like Server Components require additional learning
Migration Complexity: Moving from Pages to App Router can be challenging
Pages Router Challenges
Limited Layout Options: Less flexible than App Router's nested layouts
Feature Access: Missing out on newer Next.js features
Future-proofing: Potential technical debt as the framework evolves
Best Practices for Either Choice
Regardless of which router you choose, follow these guidelines:
Structure Your Application Thoughtfully
Keep components organized and well-documented
Maintain clear separation between server and client code
Use appropriate data fetching methods
Monitor Performance
Regularly check Core Web Vitals
Use built-in Next.js analytics
Monitor SEO metrics through Google Search Console
Plan for the Future
Document your routing decisions
Keep your dependencies updated
Stay informed about Next.js updates
Conclusion
The choice between App Router and Pages Router isn't just about technical capabilities—it's about aligning with your project's needs, team expertise, and SEO requirements. While App Router represents the future of Next.js with its modern features and improved performance capabilities, Pages Router remains a reliable choice for teams prioritizing stability and simplicity.
Remember:
Choose App Router for new projects wanting to leverage modern features
Stick with Pages Router for stable, straightforward applications
Prioritize SEO considerations regardless of your choice
By understanding these differences and following the implementation guidelines above, you can make an informed decision that serves your project's needs while maintaining strong SEO performance.
For more detailed information, consult the official Next.js documentation and stay engaged with the community discussions for real-world insights and updates.