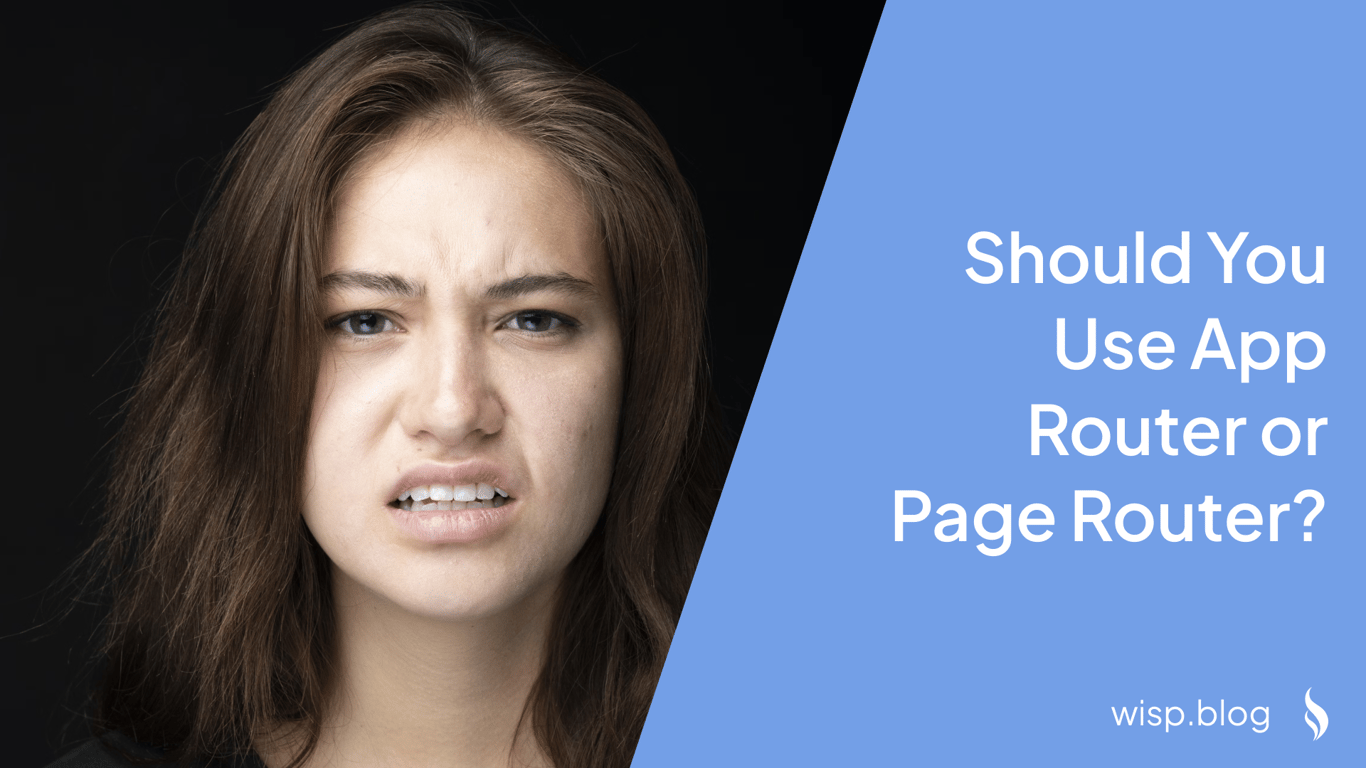
As the demand for dynamic and scalable web applications grows, Next.js has emerged as a popular framework for React developers. With the release of Next.js 14, developers face a critical decision: should they use the new App Router or stick with the traditional Page Router? This article explores the differences between the two, helping you make an informed choice for your next project.
The Evolution of Routing in Next.js
Next.js initially introduced the Page Router as its primary routing mechanism, leveraging a file-system-based approach. This method allowed developers to create routes simply by adding files to the /pages
directory. As the framework evolved, the introduction of the App Router in later versions marked a significant shift, integrating server-centric routing and React Server Components.
Historical Context: The Introduction of the Page Router
The Page Router was the foundation of Next.js, simplifying routing by mapping URLs directly to files. This approach was intuitive and easy to grasp, especially for developers transitioning from traditional web development frameworks.
Transition to the App Router
With the advent of React Server Components and the need for more complex and performant routing mechanisms, Next.js introduced the App Router. This new router is designed to handle more sophisticated routing scenarios, offering increased flexibility and performance enhancements.
App Router vs Page Router
Fundamental Differences
App RouterThe App Router in Next.js 14 adopts a server-centric approach, leveraging React Server Components to deliver faster and more dynamic content. This mechanism allows developers to create nested routes within the /app
directory, automating complex routing logic and improving performance by engaging the server-side rendering capabilities of Next.js.
The Page Router remains a client-side routing solution, rooted in the traditional file-system-based methodology. Each file within the /pages
directory corresponds to a specific route, simplifying route management and ensuring a straightforward development process, particularly for simpler applications and static sites.
Performance and Flexibility
App RouterThe App Router offers enhanced performance and flexibility, making it suitable for larger and more complex projects. By offloading much of the rendering work to the server, it reduces client-side load and speeds up page transitions, especially for dynamic content-heavy applications. However, this flexibility comes with increased complexity, requiring a deeper understanding of server-side rendering and React Server Components.
Page RouterOn the other hand, the Page Router is geared towards simplicity. Its client-side nature makes it ideal for smaller projects or static sites where server-side rendering is not a priority. This simplicity often translates to quicker development times and easier maintenance, although it may not perform as efficiently as the App Router in handling large-scale dynamic content.
Use Cases
When to Use App RouterThe App Router is suitable for projects that require:
Advanced performance optimization
Complex and dynamic routing scenarios
Integration with server-side features and React Server Components
A high degree of flexibility in route management
The Page Router is ideal for projects that benefit from:
Simple and intuitive route management
Static sites or applications with straightforward routing needs
Quick setup and reduced complexity for beginners or small teams
Detailed Features of Each Router
App RouterSetting up the App Router involves creating nested directories within the /app
folder. Each folder represents a route segment, and special files like page.js
, layout.js
, and error.js
define the content and behavior of these routes.
For example:
/app
└── dashboard
├── page.js
├── settings
│ └── page.js
└── analytics
├── page.js
└── overview
└── page.js
In this structure, dashboard/settings/page.js
corresponds to the /dashboard/settings
route, demonstrating how nested folders create nested routes.
The Page Router relies on a simpler directory structure within the /pages
directory. Each file directly represents a route, making the setup process more straightforward. Dynamic routes are created using bracket notation in the file names.
For instance:
/pages
├── index.js
├── about.js
├── blog
│ ├── [id].js
│ └── index.js
└── contact.js
In this case, blog/[id].js
corresponds to dynamic routes like /blog/1
or /blog/welcome-to-my-blog
, providing a clear and intuitive way to manage routes.
Practical Examples
Setting Up a Simple Project with Page Router
To set up a simple project using the Page Router, follow these steps:
Install Next.js: First, create a new Next.js project.
npx create-next-app@latest my-simple-site
cd my-simple-site
Directory Structure: Navigate to the
/pages
directory to define your routes.
/pages
├── index.js
├── about.js
├── blog
│ ├── [id].js
│ └── index.js
└── contact.js
Create Pages: Add content to your pages.
index.js
:import Head from 'next/head'; export default function Home() { return ( <div> <Head> <title>Home Page</title> </Head> <h1>Welcome to My Simple Site</h1> </div> ); }
about.js
:import Head from 'next/head'; export default function About() { return ( <div> <Head> <title>About Us</title> </Head> <h1>About Us</h1> <p>This is the about page.</p> </div> ); }
blog/[id].js
:import { useRouter } from 'next/router'; import Head from 'next/head'; export default function BlogPost() { const router = useRouter(); const { id } = router.query; return ( <div> <Head> <title>Blog Post {id}</title> </Head> <h1>Blog Post {id}</h1> <p>This is blog post {id}.</p> </div> ); }
Run the Project: Start the development server.
npm run dev
Navigate to http://localhost:3000 to see your site in action.
Setting Up a Complex Project with App Router
For a more complex project using the App Router, follow these steps:
Install Next.js: Start by creating a new Next.js project.
npx create-next-app@latest my-complex-site
cd my-complex-site
Directory Structure: Navigate to the
/app
directory to define your routes.
/app
└── dashboard
├── page.js
├── settings
│ └── page.js
└── analytics
├── page.js
└── overview
└── page.js
Create Pages and Layouts: Add content to your pages and define layouts.
dashboard/page.js
:import Head from 'next/head'; export default function Dashboard() { return ( <div> <Head> <title>Dashboard</title> </Head> <h1>Dashboard</h1> <p>Welcome to the dashboard.</p> </div> ); }
dashboard/settings/page.js
:import Head from 'next/head'; export default function Settings() { return ( <div> <Head> <title>Settings</title> </Head> <h1>Settings</h1> <p>Manage your settings here.</p> </div> ); }
dashboard/analytics/overview/page.js
:import Head from 'next/head'; export default function Overview() { return ( <div> <Head> <title>Analytics Overview</title> </Head> <h1>Analytics Overview</h1> <p>Overview of your analytics data.</p> </div> ); }
Run the Project: Start the development server.
npm run dev
Visit http://localhost:3000/dashboard to explore your complex site.
Migration Strategies
Upgrading a project from the Page Router to the App Router requires careful planning and execution. Here are some steps and considerations to ensure a smooth transition:
Best Practices for Migration
Assessment: Evaluate the complexity of your current project. Understand the implications of migrating to the App Router, particularly if your project heavily relies on static site generation (SSG) or server-side rendering (SSR) features provided by the Page Router.
Backup: Before making any changes, ensure that you have a complete backup of your project. This step is crucial to avoid any data loss during the migration process.
Incremental Migration: Instead of migrating the entire project at once, consider an incremental approach. Start by migrating a few pages or sections to the App Router to test and understand the new routing mechanisms.
Update Directory Structure: Create the necessary directories and files within the
/app
folder, following the App Router conventions.Refactor Code: Update your route definitions and components to align with the App Router's structure and conventions. Pay attention to the use of server components and dynamic routing patterns.
Testing: Thoroughly test the migrated sections to ensure they function correctly. Check for any issues related to routing, data fetching, and performance.
Documentation: Document the migration process, including any changes made to the directory structure, route definitions, and component logic. This documentation will be valuable for future maintenance and updates.
Step-by-Step Guide for Migration
Create New Directories: Set up the
/app
directory and create subdirectories for each route segment.
/app
└── new-route
├── page.js
└── settings
└── page.js
Move Components: Transfer your existing components from the
/pages
directory to the appropriate locations within the/app
directory.Update Import Statements: Ensure that all import statements are updated to reflect the new file paths in the
/app
directory.Refactor Route Definitions: Update your route definitions to use the new App Router conventions. Replace any Page Router-specific code with equivalent App Router logic.
Test Routes: Verify that the new routes are functioning as expected. Check for any issues with navigation, data fetching, and rendering.
Gradual Rollout: Gradually migrate more pages and components, testing each step to ensure stability and performance.
Final Migration: Once all components and routes have been migrated, remove the old
/pages
directory and associated files.
Considerations for Migrating Existing Projects
Compatibility: Ensure that all third-party libraries and dependencies are compatible with the App Router.
Performance Optimization: Take advantage of the App Router's performance benefits by optimizing data fetching and rendering strategies.
Team Training: Educate your development team on the new routing mechanisms and best practices for using the App Router.
By following these best practices and steps, you can successfully migrate your project from the Page Router to the App Router, leveraging the latest features and performance enhancements offered by Next.js 14.
Pros and Cons
Understanding the strengths and weaknesses of each router can help you make a more informed decision when starting a new Next.js 14 project.
Pros of App Router
Enhanced Performance: The App Router leverages server-side rendering and React Server Components, which can significantly improve performance, especially for dynamic content.
Greater Flexibility: Provides more control over complex routing scenarios, making it ideal for larger applications with sophisticated routing needs.
Modern Features: Access to the latest features and optimizations in Next.js, including layouts, nested routes, and advanced data fetching techniques.
Cons of App Router
Steeper Learning Curve: The complexity of the App Router means that it requires a deeper understanding of server-side rendering and React Server Components, which may be challenging for beginners.
Increased Complexity: Managing routes and components in a more granular manner can introduce additional complexity, making the project harder to maintain.
Pros of Page Router
Simplicity and Ease of Use: The Page Router's straightforward, file-system-based approach is easy to understand and implement, making it ideal for small projects and beginners.
Quick Setup: Faster to set up and get running, which can be beneficial for prototypes, small-scale projects, or when time is a constraint.
Reduced Overhead: Less complex routing and component management reduces the overhead, making it easier to maintain over time.
Cons of Page Router
Limited Flexibility: May struggle with more complex routing scenarios and dynamic content, as it lacks the advanced features provided by the App Router.
Performance Constraints: Client-side routing can lead to slower performance for dynamic content-heavy applications compared to server-side rendering.
Conclusion
Choosing between the App Router and the Page Router in Next.js 14 depends on your project requirements, complexity, and your team's familiarity with Next.js and server-side rendering. For modern, dynamic, and performance-intensive applications, the App Router provides a robust and flexible solution. However, for simpler projects or when ease of use and quick setup are priorities, the Page Router remains a viable option.
By understanding the fundamental differences, performance considerations, and practical use cases, you can make an informed decision that aligns with your development goals.