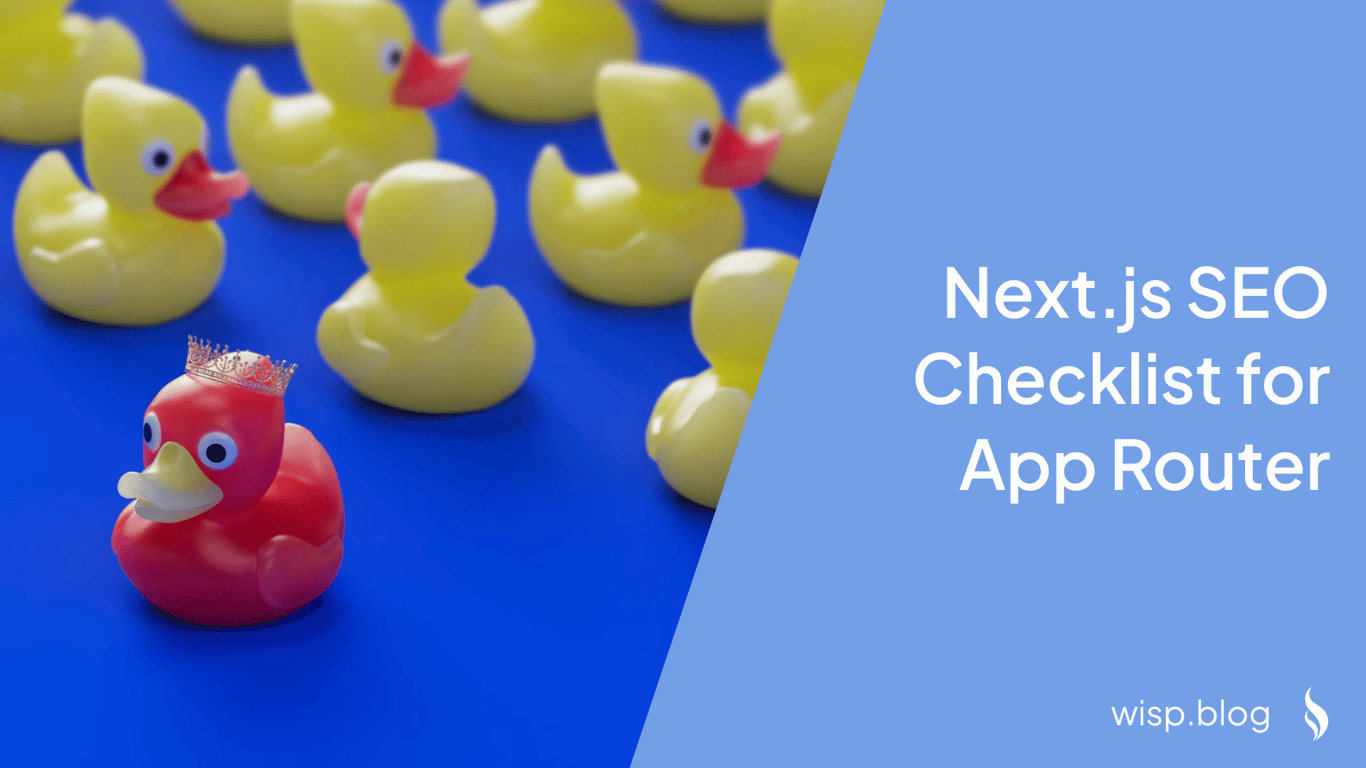
Are you struggling to optimize your Next.js application for search engines? You're not alone. Many Next.js developers face challenges with technical SEO, especially when dealing with server-side rendering and dynamic content. The good news is that Next.js provides powerful built-in features to enhance your site's SEO performance.
In this comprehensive guide, we'll walk through everything you need to know about optimizing your Next.js application for search engines. Whether you're worried about Google's ability to crawl your React-based site or looking to improve your rankings, this checklist has you covered.
Why SEO Matters for Next.js Applications
Before diving into the technical details, let's understand why SEO is crucial for your Next.js application. According to recent studies, 68% of online experiences begin with a search engine. If your site isn't optimized for search engines, you're potentially missing out on a significant amount of organic traffic.
Many developers worry that React-based applications have inherent SEO problems. As one developer expressed on Reddit:
"After speaking with another developer, he mentioned that React has problems with SEO, as Google cannot crawl it or something? This isn't good news for me as I really do want this site to grow on Google's rankings!"
However, Next.js solves these traditional React SEO challenges through its powerful features:
Server-Side Rendering (SSR): Generates HTML on the server, making your content immediately visible to search engines
Static Site Generation (SSG): Creates static HTML at build time for optimal performance
Automatic Image Optimization: Improves Core Web Vitals scores
Built-in Performance Features: Includes automatic code splitting and lazy loading
Essential Next.js SEO Features
1. Choose the Right Rendering Strategy
The foundation of good SEO in Next.js starts with selecting the appropriate rendering strategy for your content:
Static Site Generation (SSG): Best for blog posts, documentation, and marketing pages
Server-Side Rendering (SSR): Ideal for dynamic content that needs real-time updates
Incremental Static Regeneration (ISR): Perfect for content that changes occasionally
Client-Side Rendering (CSR): Use sparingly, only for private or user-specific content
2. Optimize Meta Tags and Headers
Meta tags are crucial for SEO. Next.js makes it easy to manage them using the built-in next/head
component:
import Head from 'next/head'
function YourPage() {
return (
<>
<Head>
<title>Your SEO-Optimized Title | Brand Name</title>
<meta name="description" content="A compelling meta description between 150-160 characters that accurately summarizes your page content and encourages clicks." />
{/* Open Graph tags for social sharing */}
<meta property="og:title" content="Your SEO-Optimized Title" />
<meta property="og:description" content="Your compelling description" />
<meta property="og:image" content="https://yourdomain.com/og-image.jpg" />
{/* Twitter Card tags */}
<meta name="twitter:card" content="summary_large_image" />
<meta name="twitter:title" content="Your SEO-Optimized Title" />
<meta name="twitter:description" content="Your compelling description" />
<meta name="twitter:image" content="https://yourdomain.com/twitter-image.jpg" />
</Head>
{/* Your page content */}
</>
)
}
3. Implement Structured Data
Structured data helps search engines understand your content better and can lead to rich snippets in search results. Use JSON-LD format:
<Head>
<script
type="application/ld+json"
dangerouslySetInnerHTML={{
__html: JSON.stringify({
"@context": "https://schema.org",
"@type": "Article",
"headline": "Your Article Title",
"image": [
"https://example.com/photos/1x1/photo.jpg",
"https://example.com/photos/4x3/photo.jpg",
"https://example.com/photos/16x9/photo.jpg"
],
"datePublished": "2024-01-01T08:00:00+08:00",
"dateModified": "2024-01-01T09:20:00+08:00",
"author": [{
"@type": "Person",
"name": "John Doe",
"url": "http://example.com/profile/johndoe"
}]
})
}}
/>
</Head>
4. Optimize Images and Media
Next.js provides the Image
component for automatic image optimization. This is crucial for Core Web Vitals and overall SEO performance:
import Image from 'next/image'
function OptimizedImage() {
return (
<Image
src="/path/to/your/image.jpg"
alt="Descriptive alt text for SEO"
width={800}
height={600}
priority={true} // For LCP images
loading="lazy" // For images below the fold
/>
)
}
5. Create a Dynamic Sitemap
A sitemap helps search engines discover and index your pages. Use the sitemap metadata file to automatically generate one.
Create sitemap.ts
in any app route. In the file, you will export a default function that returns an array of URLs. You can use this to programmatically generate the sitemap from different route segments of your site:
import type { MetadataRoute } from 'next'
export default function sitemap(): MetadataRoute.Sitemap {
return [
{
url: 'https://acme.com',
lastModified: new Date(),
changeFrequency: 'yearly',
priority: 1,
},
{
url: 'https://acme.com/about',
lastModified: new Date(),
changeFrequency: 'monthly',
priority: 0.8,
},
{
url: 'https://acme.com/blog',
lastModified: new Date(),
changeFrequency: 'weekly',
priority: 0.5,
},
]
}
6. Monitor and Optimize Performance
Next.js applications should be regularly monitored for SEO performance. Here's a checklist of tools and metrics to track:
Core Web Vitals
Largest Contentful Paint (LCP): < 2.5 seconds
First Input Delay (FID): < 100 milliseconds
Cumulative Layout Shift (CLS): < 0.1
Google Search Console
Monitor indexing status
Track search performance
Identify and fix technical issues
Bing Webmaster Tools
Similar monitoring for Bing search engine
Different audience insights
Lighthouse Scores
Aim for scores above 90 in all categories
Pay special attention to Performance and SEO scores
Advanced SEO Optimization Tips
1. Implement Dynamic OG Images
Create dynamic Open Graph images for better social sharing:
import { ImageResponse } from 'next/server'
export const runtime = 'edge'
export async function GET(request) {
return new ImageResponse(
(
<div
style={{
fontSize: 128,
background: 'white',
width: '100%',
height: '100%',
display: 'flex',
alignItems: 'center',
justifyContent: 'center',
}}
>
Dynamic OG Image
</div>
),
{
width: 1200,
height: 630,
},
)
}
2. Optimize for Core Web Vitals
Implement these best practices to improve your Core Web Vitals scores:
Use
next/font
for optimized font loading:
import { Inter } from 'next/font/google'
const inter = Inter({ subsets: ['latin'] })
export default function RootLayout({ children }) {
return (
<html lang="en" className={inter.className}>
<body>{children}</body>
</html>
)
}
Implement route prefetching for faster navigation:
import Link from 'next/link'
export default function Navigation() {
return (
<Link href="/about" prefetch={true}>
About Us
</Link>
)
}
Common SEO Mistakes to Avoid
Not Using Canonical URLs
Implement canonical URLs to prevent duplicate content issues
Use the
next/head
component to add canonical tags
Ignoring Mobile Optimization
Ensure your site is mobile-friendly
Test regularly using Google's Mobile-Friendly Test
Poor Internal Linking
Create a logical site structure
Use descriptive anchor texts
Conclusion
Implementing proper SEO for your Next.js application requires attention to detail and regular maintenance. By following this checklist, you'll be well on your way to improving your search engine rankings and increasing organic traffic.
Remember to:
Choose the appropriate rendering strategy for your content
Optimize meta tags and structured data
Implement proper image optimization
Monitor performance regularly
Stay updated with Next.js SEO best practices
For more detailed information, check out the official Next.js documentation on SEO and join the Next.js community discussions for the latest tips and tricks.