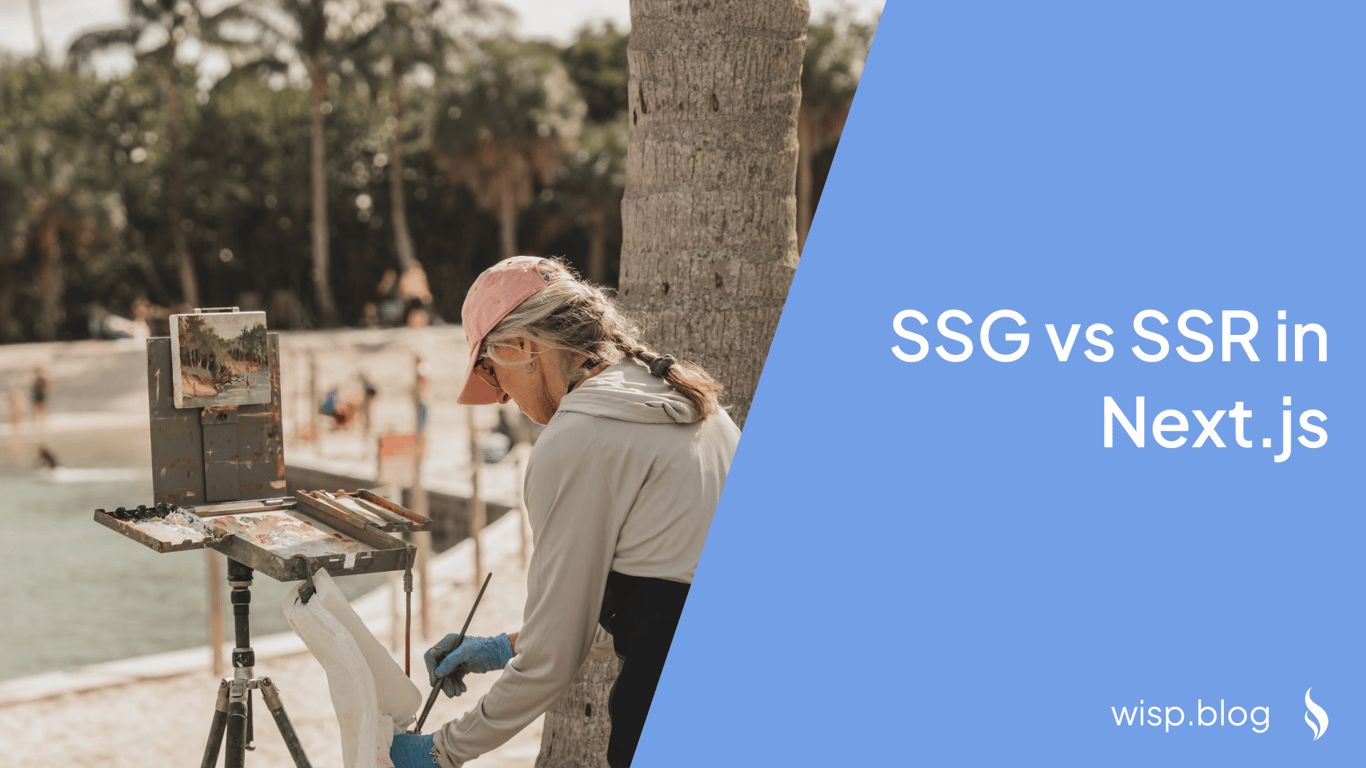
You've started building your Next.js application, and now you're faced with a crucial decision: Should you use Static Site Generation (SSG) or Server-Side Rendering (SSR)? If you're like many developers, you might find yourself puzzled by this choice, especially when dealing with dynamic content like e-commerce products or frequently updated data.
"If you skip the docs, you might run into a few 'gotchas'," as one developer noted on Reddit. This confusion is particularly evident when developers ask questions like, "Should I use SSR or SSG for product pages if the data changes frequently?"
Let's clear up this confusion and help you make the right choice for your application.
Understanding the Basics
Static Site Generation (SSG)
SSG pre-renders your pages at build time, generating HTML files that can be cached and served quickly to users. Think of it as preparing all your meals for the week on Sunday – everything is ready to go when needed.
// Example of SSG implementation
export async function getStaticProps() {
const data = await fetchProductData();
return {
props: { data },
revalidate: 60 // Optional: Enable ISR with 60-second intervals
}
}
Server-Side Rendering (SSR)
SSR generates pages on-demand for each request, ensuring content is always fresh but potentially introducing latency. It's like cooking meals to order – fresher but takes more time.
// Example of SSR implementation
export async function getServerSideProps() {
const data = await fetchProductData();
return {
props: { data }
}
}
Incremental Static Regeneration (ISR)
ISR offers a hybrid approach, allowing you to update static pages after they're built. As one developer suggests, "If your product data changes frequently and you receive hundreds of hits per minute, maybe go for a short timeout like 30 seconds. Or if data isn't changing so frequently, you can get by with a 10-minute revalidation timeout."
Making the Right Choice
When to Choose SSG
Static Content
Blog posts
Documentation pages
Marketing landing pages
Portfolio websites
Predictable Content Updates
Product catalogs with scheduled updates
Event listings with fixed schedules
Company news and announcements
Performance PrioritySSG shines when performance is crucial. As noted in community discussions, static generation provides consistently fast loading times and reduced server load, making it ideal for high-traffic pages with relatively stable content.
When to Choose SSR
Highly Dynamic Content
User dashboards
Real-time inventory systems
Personalized content
Social media feeds
Authentication-Dependent Pages
User profiles
Admin panels
Protected content areas
Real-Time Data RequirementsWhen your application needs to display up-to-the-second information, SSR ensures users always see the latest data.
The Role of ISR
ISR bridges the gap between SSG and SSR, offering a compelling middle ground. It's particularly useful when:
You need the performance benefits of SSG but can't compromise on content freshness
Your data updates follow predictable patterns
You want to optimize server costs while maintaining reasonable content freshness
// Example of ISR implementation
export async function getStaticProps() {
const products = await fetchProducts();
return {
props: {
products,
},
revalidate: 60 * 5, // Revalidate every 5 minutes
}
}
Performance and SEO Considerations
Performance Impact
SSG Performance
Fastest possible page loads
Reduced server load
Better caching capabilities
Lower hosting costs
SSR Performance
Variable response times
Higher server requirements
Potential for bottlenecks under heavy load
More complex scaling needs
As one developer points out, "The complexity of building an SPA/SSG app is way lower than SSR app," which can impact both development time and maintenance costs.
SEO Implications
Both SSG and SSR provide excellent SEO capabilities, but they differ in implementation:
SSG for SEO
Perfect for content that doesn't change frequently
Guaranteed indexing of content
Faster page loads (a ranking factor)
More predictable crawling behavior
SSR for SEO
Ideal for time-sensitive content
Ensures search engines always see current content
Better for personalized content
Suitable for dynamic meta tags
Common Pitfalls and Solutions
SSG Challenges
Build Time Issues
// Solution: Use dynamic imports to reduce initial build time const DynamicComponent = dynamic(() => import('../components/Heavy'))
Content Freshness
// Solution: Implement ISR with appropriate revalidation export async function getStaticProps() { return { props: { data }, revalidate: 60 * 15 // Revalidate every 15 minutes } }
SSR Challenges
Server Load
// Solution: Implement caching where appropriate export async function getServerSideProps({ req, res }) { res.setHeader( 'Cache-Control', 'public, s-maxage=10, stale-while-revalidate=59' ) return { props: { data } } }
Performance Bottlenecks
Implement proper caching strategies
Use edge functions when available
Consider hybrid approaches
Best Practices and Recommendations
Optimizing Your Choice
Analyze Your Content Pattern
How often does your content change?
What's your traffic pattern?
What's your target audience's location?
Consider Resource ConstraintsAs highlighted in user discussions, "there's still no built-in cost control, so you really have to stay on top of your usage." Consider:
Server costs
Development complexity
Maintenance requirements
Implementation Strategy
// Hybrid approach example // SSG for static pages export async function getStaticProps() { const staticData = await fetchStaticContent() return { props: { staticData } } } // SSR for dynamic features export async function getServerSideProps() { const dynamicData = await fetchDynamicContent() return { props: { dynamicData } } }
Making the Final Decision
Consider these factors when making your choice:
Content Update Frequency
Static/Infrequent Updates → SSG
Regular/Scheduled Updates → ISR
Real-time Updates → SSR
Traffic Patterns
High Traffic, Static Content → SSG
Variable Traffic, Dynamic Content → SSR
High Traffic, Semi-dynamic → ISR
Development Resources
Limited Resources → SSG
Robust Infrastructure → SSR
Balanced Needs → ISR
Conclusion
The choice between SSG and SSR isn't always black and white. As we've seen from community discussions, each approach has its merits and ideal use cases. The key is understanding your specific needs and constraints.
Remember:
SSG for static, performance-critical content
SSR for dynamic, real-time requirements
ISR when you need the best of both worlds
By carefully considering your application's needs and following the guidelines outlined above, you can make an informed decision that balances performance, development complexity, and user experience.
Start with the simplest solution that meets your needs, and don't be afraid to evolve your approach as your application grows. After all, Next.js's flexibility allows you to mix and match these rendering strategies within the same application.