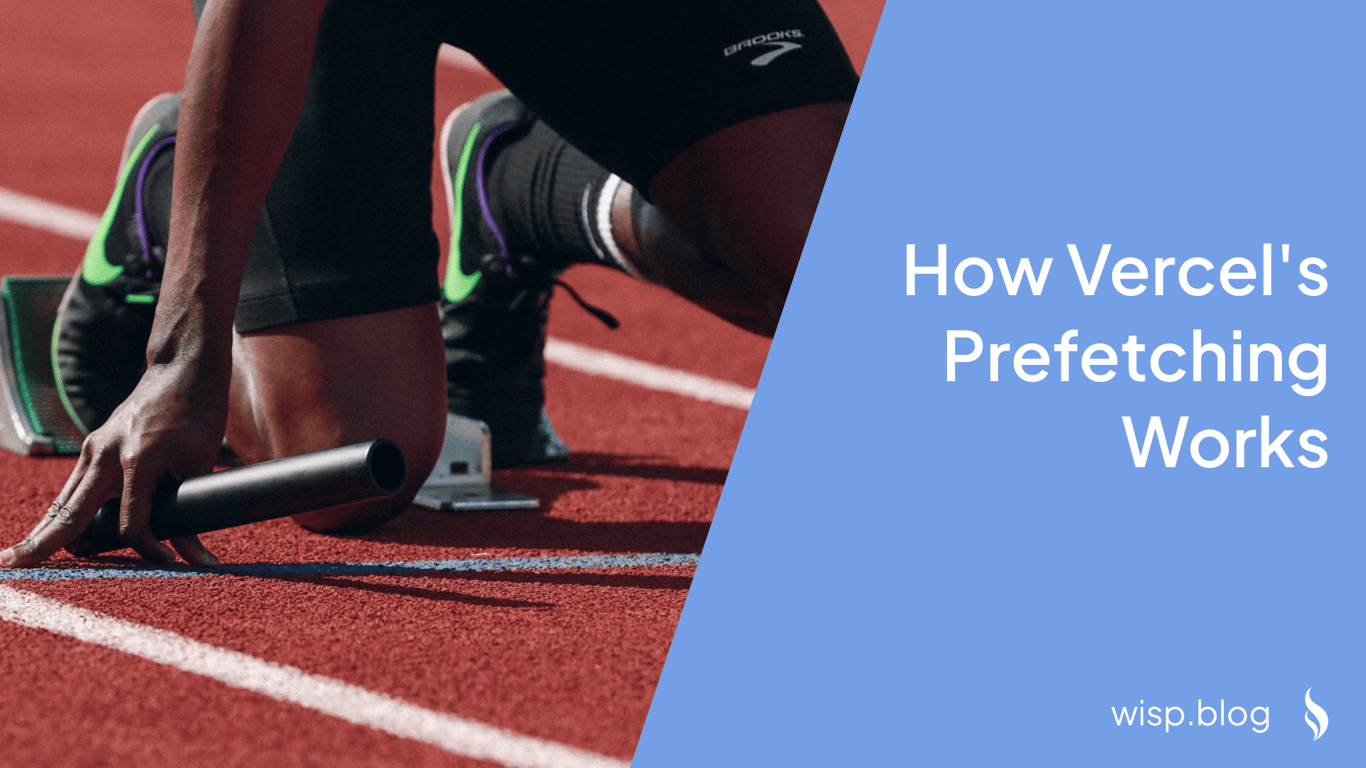
You've just deployed your Next.js application on Vercel, and while checking your logs, you notice something peculiar - there are numerous GET requests for pages you haven't even visited yet. If you're scratching your head wondering about these mysterious requests, you're not alone. Welcome to the world of Vercel's prefetching.
Understanding Prefetching in Vercel
Prefetching is a performance optimization technique where your application proactively loads resources before they're explicitly needed. Think of it as your app reading your mind - when you hover over a link, it starts loading the next page before you even click it.
But here's where things get interesting: Vercel's implementation goes beyond simple resource loading. When you hover over a link in a Next.js application, Vercel doesn't just fetch the HTML - it retrieves the entire page payload, including data dependencies and JavaScript bundles. This aggressive approach aims to create near-instantaneous page transitions.
The Mechanics Behind the Magic
Vercel employs several sophisticated techniques to make prefetching work:
Viewport Detection: Next.js automatically detects which links are visible in the user's viewport
Smart Timing: Prefetching typically triggers on link hover or when links become visible
Resource Prioritization: Critical resources are loaded first to ensure optimal performance
For example, when you're scrolling through a blog listing page, Next.js might start prefetching the content of visible article links before you even decide to click them.
The Evolution of Prefetching
Vercel's prefetching implementation has evolved significantly, now leveraging modern web APIs like the Speculation Rules API for more efficient preloading:
<script type="speculationrules">
{
"prerender": [{ "source": "list" }],
"prefetch": [{ "source": "list" }]
}
</script>
This modern approach allows for more granular control over prefetching behavior while maintaining compatibility with older browsers through fallback mechanisms.
The Benefits of Vercel's Prefetching
1. Lightning-Fast Navigation
The most immediate benefit of prefetching is dramatically improved perceived performance. When a user clicks a prefetched link, the transition feels instantaneous because the content is already available in the browser's cache.
2. Improved User Experience
Users experience smoother navigation throughout your application. This is particularly noticeable in content-heavy applications where traditional page loads might cause jarring transitions.
3. Enhanced SEO Performance
Search engines increasingly consider page speed and user experience metrics in their rankings. The faster navigation enabled by prefetching can positively impact your Core Web Vitals scores.
4. Reduced Server Load
While it might seem counterintuitive, strategic prefetching can actually reduce server load by:
Distributing requests over time rather than in bursts
Leveraging browser caching effectively
Minimizing the need for repeated requests
The Gotchas: When Prefetching Becomes Problematic
1. Cost Implications
According to recent discussions in the Next.js community, one of the most significant concerns is the potential cost impact of aggressive prefetching. Users have reported:
Unexpected spikes in billing during high-traffic periods
Excessive function invocations from prefetch requests
Higher bandwidth costs from preloading unused resources
2. Performance Paradox
While prefetching aims to improve performance, it can sometimes have the opposite effect:
Multiple JSON files downloading simultaneously can overwhelm the browser
Excessive prefetching can impact Google Lighthouse scores negatively
Resource contention when too many pages are prefetched at once
3. Debugging Challenges
As one developer noted in the community discussions, prefetching can make debugging more complicated:
Log files become cluttered with prefetch requests
Difficult to distinguish between actual user navigation and prefetch requests
Complex performance profiling due to background loading
Optimizing Prefetching: Best Practices
1. Selective Prefetching
Instead of allowing Next.js to prefetch everything, be strategic about what you prefetch:
// Disable prefetching for specific links
<Link href="/heavy-page" prefetch={false}>
Heavy Content
</Link>
// Enable prefetching only for critical paths
<Link href="/important-page" prefetch={true}>
Critical Path
</Link>
2. Implementing Smart Prefetch Controls
Create a custom prefetching strategy based on:
User behavior patterns
Device capabilities and network conditions
Resource size and importance
Likelihood of navigation
3. Monitoring and Optimization
Keep track of your prefetching performance:
Use Vercel's analytics to monitor prefetch patterns
Track costs associated with prefetching
Analyze user navigation paths to optimize prefetch strategies
4. Caching Strategy
Implement an effective caching strategy to maximize the benefits of prefetching:
// Example of cache control headers in Next.js API routes
export async function getServerSideProps({ res }) {
res.setHeader(
'Cache-Control',
'public, s-maxage=10, stale-while-revalidate=59'
)
return {
props: {
// Your data here
}
}
}
Advanced Prefetching Techniques
1. Dynamic Prefetching
Implement dynamic prefetching based on user interaction patterns:
const PrefetchManager = {
trackUserBehavior: (link) => {
// Track which links users commonly navigate to
},
shouldPrefetch: (link) => {
// Decision logic based on collected data
return userNavigationProbability > threshold
}
}
2. Resource Prioritization
Prioritize prefetching based on resource importance:
// Example of prioritized prefetching
const PriorityPrefetch = {
HIGH: ['/', '/products', '/cart'],
MEDIUM: ['/blog', '/about'],
LOW: ['/terms', '/privacy']
}
Future-Proofing Your Prefetching Strategy
Emerging Technologies
Keep an eye on emerging technologies that could impact prefetching:
Speculation Rules API: This new browser API provides more granular control over prefetching and prerendering.
Vercel's Fluid Compute: A pricing model that can help manage costs associated with prefetching by charging based on actual CPU usage.
Best Practices for Scale
As your application grows:
Monitor Resource Usage
Track bandwidth consumption
Monitor server-side function calls
Analyze cache hit rates
Optimize for Cost
Implement rate limiting for prefetch requests
Use intelligent caching strategies
Consider selective prefetching based on user segments
Balance Performance and Cost
Regularly review prefetching strategies
Adjust based on real-world usage data
Consider A/B testing different prefetch approaches
Conclusion
Vercel's prefetching is a powerful feature that can significantly enhance user experience when implemented thoughtfully. While it comes with potential challenges around costs and complexity, these can be effectively managed through careful monitoring and optimization.
The key is finding the right balance for your specific use case - between aggressive prefetching for performance and controlled prefetching for cost management. By following the best practices outlined above and staying informed about new developments in prefetching technologies, you can make the most of this feature while avoiding its potential pitfalls.
Remember: The goal isn't to prefetch everything, but to prefetch the right things at the right time for your users.