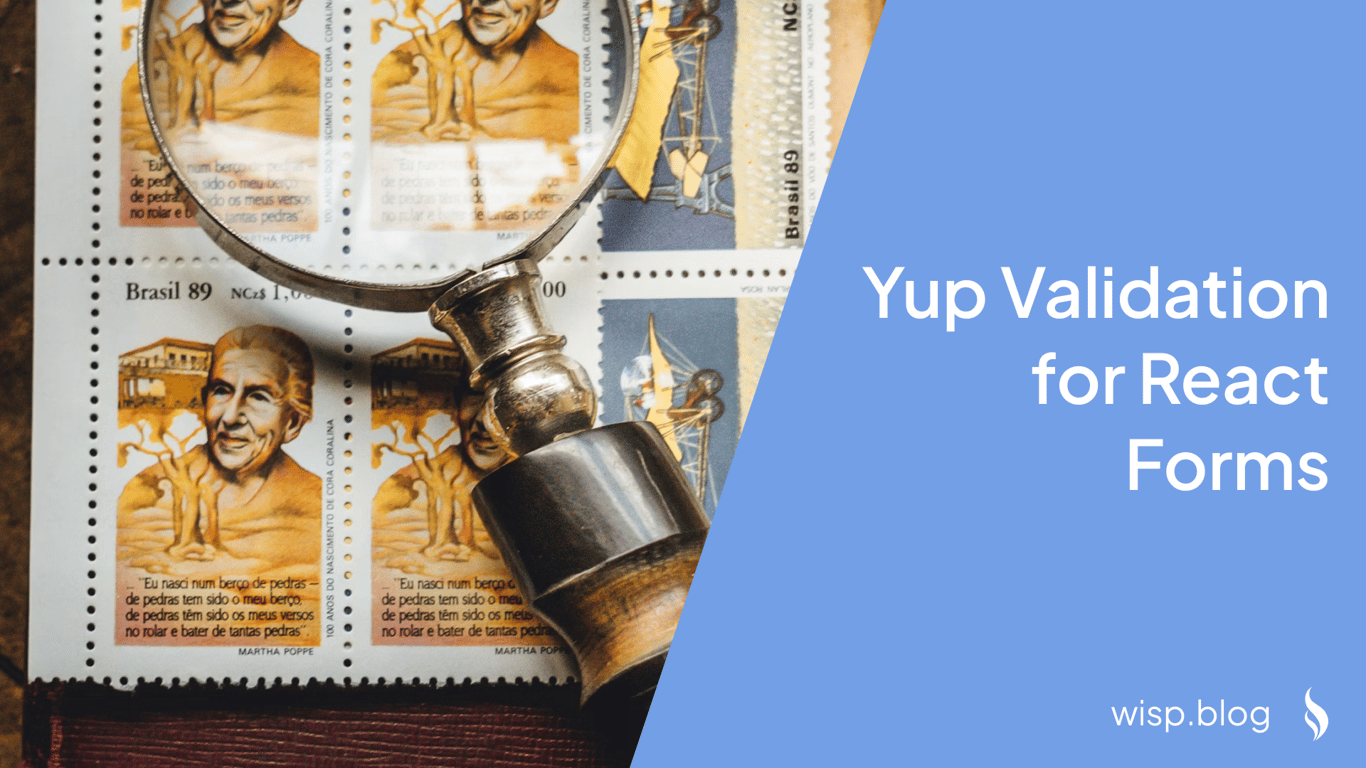
Are you wrestling with form validation in your React applications? You're not alone. Many developers find themselves struggling with validation libraries, trying to piece together the best approach while dealing with issues like invalid form submissions, messy validation code, and confusing documentation.
While there are several validation libraries available (with Zod gaining popularity recently), Yup remains a solid choice for its simplicity and extensive feature set, especially if you're working primarily with JavaScript.
Why Form Validation Matters
Before diving into Yup, let's understand why proper form validation is crucial:
Data Integrity: Invalid form submissions can corrupt your database or cause inconsistencies in your application state
User Experience: Immediate feedback helps users correct mistakes before submission
Server Efficiency: Validating on the client side reduces unnecessary server requests
Security: Proper validation helps prevent malicious data from reaching your backend
User Retention: Forms that are easy to complete and provide clear feedback keep users engaged
What is Yup?
Yup is a JavaScript schema builder for value parsing and validation. It provides an intuitive API that works seamlessly with popular form management libraries like Formik and React Hook Form.
Key Benefits of Using Yup
Declarative Schema Definition
Write validation rules in a clear, readable format
Chain multiple validation methods together
Create reusable validation schemas across your application
Built-in Type Casting
Automatically converts input values to the correct type
Handles common data types (strings, numbers, dates)
Supports complex object and array validation
Rich Validation Methods
Includes pre-built validators for common scenarios
Supports custom validation logic
Handles async validation for server-side checks
Getting Started with Yup
Let's start with a basic example of how to create and use a Yup validation schema:
import * as Yup from 'yup';
const userSchema = Yup.object().shape({
email: Yup.string()
.email('Invalid email address')
.required('Email is required'),
password: Yup.string()
.min(8, 'Password must be at least 8 characters')
.required('Password is required'),
age: Yup.number()
.positive('Age must be a positive number')
.integer('Age must be an integer')
.required('Age is required')
});
Integrating Yup with Form Libraries
Using Yup with Formik
Formik is one of the most popular form management libraries for React, and it has built-in support for Yup validation. Based on common developer experiences, here's how to integrate them effectively:
import { Formik, Form, Field } from 'formik';
import * as Yup from 'yup';
const SignupSchema = Yup.object().shape({
firstName: Yup.string()
.min(2, 'Too Short!')
.max(50, 'Too Long!')
.required('Required'),
email: Yup.string()
.email('Invalid email')
.required('Required'),
});
function SignupForm() {
return (
<Formik
initialValues={{
firstName: '',
email: '',
}}
validationSchema={SignupSchema}
onSubmit={values => {
console.log(values);
}}
>
{({ errors, touched }) => (
<Form>
<Field name="firstName" />
{errors.firstName && touched.firstName ? (
<div>{errors.firstName}</div>
) : null}
<Field name="email" type="email" />
{errors.email && touched.email ? (
<div>{errors.email}</div>
) : null}
<button type="submit">Submit</button>
</Form>
)}
</Formik>
);
}
Using Yup with React Hook Form
For developers looking for better performance and less boilerplate, React Hook Form with Yup is an excellent choice:
import { useForm } from 'react-hook-form';
import { yupResolver } from '@hookform/resolvers/yup';
import * as Yup from 'yup';
const schema = Yup.object().shape({
username: Yup.string()
.required('Username is required')
.min(3, 'Username must be at least 3 characters'),
email: Yup.string()
.required('Email is required')
.email('Email is invalid'),
});
function RegistrationForm() {
const { register, handleSubmit, formState: { errors } } = useForm({
resolver: yupResolver(schema)
});
const onSubmit = data => console.log(data);
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input {...register('username')} />
{errors.username && <p>{errors.username.message}</p>}
<input {...register('email')} />
{errors.email && <p>{errors.email.message}</p>}
<button type="submit">Register</button>
</form>
);
}
Handling Common Pain Points
Managing Optional Fields
One of the most common issues developers face is handling optional fields. Here's the recommended approach:
const schema = Yup.object().shape({
optionalField: Yup.string()
.nullable()
.transform((curr, orig) => orig === '' ? null : curr)
.min(5, 'Must be at least 5 characters'),
});
This solution ensures that empty strings are treated as null values, preventing unnecessary validation errors that often frustrate users.
Custom Validation Rules
For specific business requirements, you can create custom validation rules:
const schema = Yup.object().shape({
password: Yup.string()
.required('Password is required')
.test('passwordStrength', 'Password is too weak', value => {
const hasUpperCase = /[A-Z]/.test(value);
const hasLowerCase = /[a-z]/.test(value);
const hasNumber = /[0-9]/.test(value);
return hasUpperCase && hasLowerCase && hasNumber;
}),
});
Conditional Validation
For forms with interdependent fields, use conditional validation:
const schema = Yup.object().shape({
isEmployed: Yup.boolean(),
companyName: Yup.string().when('isEmployed', {
is: true,
then: Yup.string().required('Company name is required when employed'),
otherwise: Yup.string()
})
});
Complex Data Structures
For nested objects and arrays, which are common in real-world applications:
const addressSchema = Yup.object().shape({
street: Yup.string().required('Street is required'),
city: Yup.string().required('City is required'),
zipCode: Yup.string().matches(/^\d{5}$/, 'Invalid zip code')
});
const userSchema = Yup.object().shape({
name: Yup.string().required('Name is required'),
addresses: Yup.array()
.of(addressSchema)
.min(1, 'At least one address is required')
});
Best Practices and Performance Tips
1. Centralize Your Validation Schemas
To maintain consistency and reduce duplication, create a central location for your validation schemas:
// schemas/validation.js
export const emailSchema = Yup.string()
.email('Invalid email address')
.required('Email is required');
export const passwordSchema = Yup.string()
.min(8, 'Password must be at least 8 characters')
.matches(/[A-Z]/, 'Password must contain at least one uppercase letter')
.matches(/[0-9]/, 'Password must contain at least one number')
.required('Password is required');
2. Optimize Performance
For large forms, consider these performance optimization techniques:
// Use validation debouncing
const debouncedValidation = debounce((value) => {
schema.validate(value).catch(() => {});
}, 500);
// Implement field-level validation
const schema = Yup.object().shape({
email: emailSchema,
password: passwordSchema,
}, [['email', 'password']]); // Only validate these fields together
3. Handle Async Validation
When performing server-side validation (like checking username availability):
const schema = Yup.object().shape({
username: Yup.string()
.required('Username is required')
.test('unique', 'Username already taken',
async (value) => {
if (!value) return true; // Skip API call if empty
const response = await checkUsernameAvailability(value);
return response.isAvailable;
}
)
});
Common Pitfalls and Solutions
Controlled Component Warnings
Many developers encounter the "A component is changing an uncontrolled input" warning. Here's how to fix it:
// Instead of undefined, use empty string or null
const initialValues = {
name: '', // Not undefined
email: null, // Or null
};
Accessing Form Values Outside Formik
To solve the common issue of accessing form values outside Formik context:
function FormWrapper() {
const formikRef = useRef();
const getFormValues = () => {
return formikRef.current?.values;
};
return (
<Formik
innerRef={formikRef}
// ... other props
>
{/* form content */}
</Formik>
);
}
Conclusion
Yup provides a robust solution for form validation in React applications. While alternatives like Zod exist and are gaining popularity, Yup remains a solid choice, especially for JavaScript projects.
Key takeaways:
Start with simple validation rules and add complexity as needed
Centralize your validation schemas for consistency
Use appropriate integration patterns with your chosen form library
Consider performance implications for large forms
Handle optional fields properly to avoid validation frustrations
By following these guidelines and leveraging Yup's features effectively, you can create robust form validation that enhances your application's user experience and data integrity.
For more information and examples, check out: