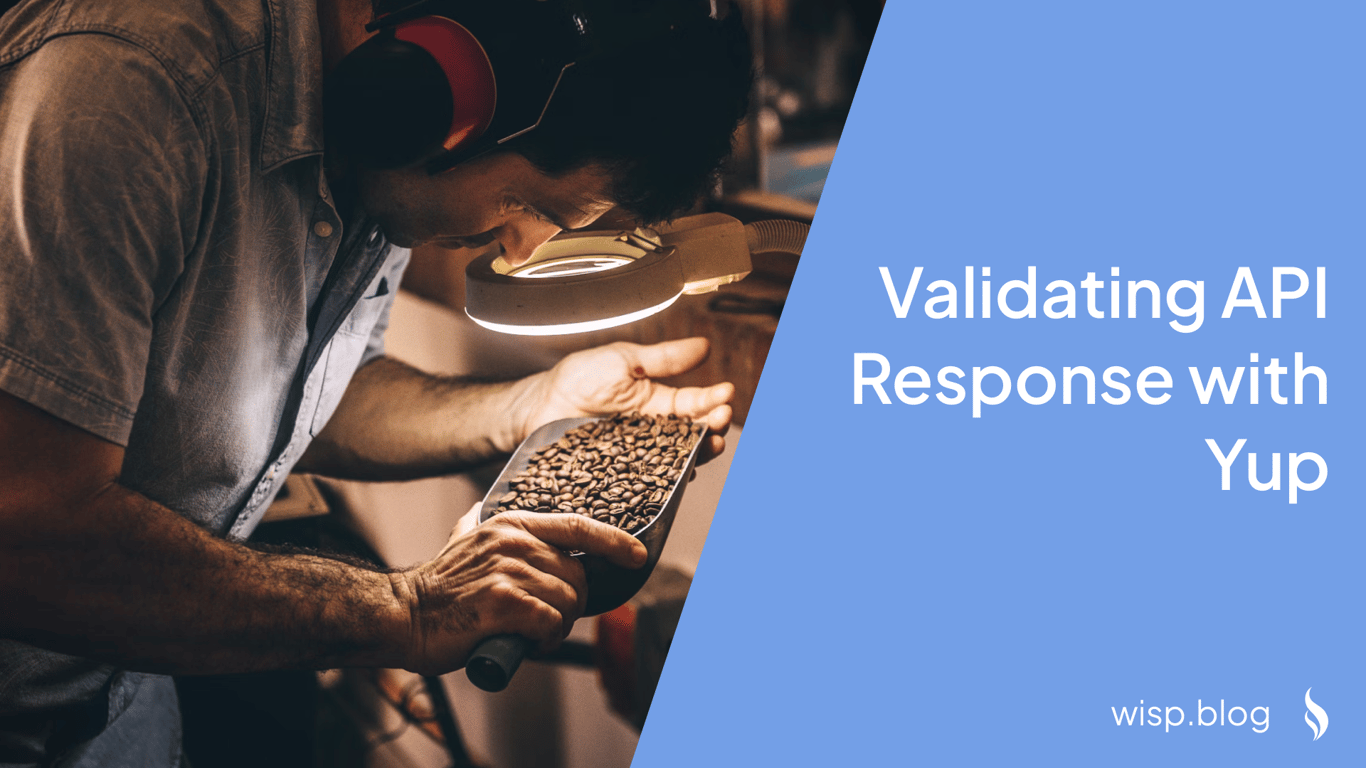
You've just integrated a third-party API into your application, and everything seems to work perfectly in development. But then, in production, your app starts crashing mysteriously. After hours of debugging, you discover the culprit: the API occasionally returns data in an unexpected format, and your application isn't prepared to handle these variations.
This is a common pain point many developers face. While we often focus on validating user inputs, we sometimes overlook the importance of validating API responses. In this comprehensive guide, we'll explore how to use Yup, a powerful JavaScript schema validation library, to ensure your application robustly handles API responses.
Why Validate API Responses?
Before diving into the implementation, let's understand why API response validation is crucial:
Protection Against Breaking Changes: APIs can change over time, and without proper validation, these changes can silently break your application.
Type Safety: While TypeScript provides compile-time type checking, runtime validation ensures the actual data matches your expectations.
Better Error Handling: Structured validation helps you catch and handle data inconsistencies gracefully instead of letting them cause runtime errors.
Documentation: Schema definitions serve as living documentation of your expected data structures.
Understanding Yup
Yup is a schema builder for runtime value parsing and validation. While it's commonly used for form validation, its capabilities extend far beyond that. Here's what makes Yup particularly suitable for API response validation:
Declarative Schema Definition: Define your expected data structure in a clear, readable format
Type Coercion: Automatically transform values to the correct type when possible
Rich Validation Rules: Extensive built-in validators and the ability to create custom ones
TypeScript Support: Excellent TypeScript integration with type inference
Nested Object Validation: Easily validate complex, nested data structures
Here's a simple example of a Yup schema:
import * as Yup from 'yup';
const userSchema = Yup.object().shape({
id: Yup.number().required('User ID is required'),
username: Yup.string().required('Username is required'),
email: Yup.string().email('Invalid email format').required('Email is required'),
age: Yup.number().positive('Age must be a positive number').integer('Age must be an integer')
});
Implementing API Response Validation
Let's look at how to implement API response validation in a real-world scenario. We'll create a robust validation system for a typical REST API response.
1. Define Base Response Schema
First, let's create a base schema for common API response structures:
const baseResponseSchema = Yup.object().shape({
status: Yup.string().oneOf(['success', 'error']).required(),
message: Yup.string(),
timestamp: Yup.date().default(() => new Date()),
data: Yup.mixed() // Will be refined in specific endpoints
});
2. Create Endpoint-Specific Schemas
For each endpoint, extend the base schema with specific data requirements:
const userResponseSchema = baseResponseSchema.shape({
data: Yup.object().shape({
id: Yup.number().required(),
username: Yup.string().required(),
email: Yup.string().email().required(),
profile: Yup.object().shape({
firstName: Yup.string(),
lastName: Yup.string(),
avatar: Yup.string().url()
})
})
});
3. Create a Validation Wrapper
To make validation reusable across your application, create a wrapper function:
async function validateApiResponse(schema, response) {
try {
const validatedData = await schema.validate(response, {
strict: true,
abortEarly: false // Collect all errors instead of stopping at first failure
});
return {
isValid: true,
data: validatedData,
errors: null
};
} catch (error) {
return {
isValid: false,
data: null,
errors: error.errors
};
}
}
4. Implement in API Calls
Now you can use this validation in your API calls:
async function fetchUserData(userId) {
try {
const response = await fetch(`/api/users/${userId}`);
const data = await response.json();
const validation = await validateApiResponse(userResponseSchema, data);
if (!validation.isValid) {
console.error('API Response Validation Failed:', validation.errors);
throw new Error('Invalid API Response');
}
return validation.data;
} catch (error) {
// Handle errors appropriately
throw error;
}
}
Best Practices and Advanced Techniques
1. Performance Optimization
While validation is important, it's crucial to consider its performance impact. Here are some optimization strategies:
// Cache schema instances
const cachedSchema = Yup.object().shape({
// ... schema definition
}).strict();
// Use selective validation for large objects
const partialSchema = Yup.object().shape({
criticalField: Yup.string().required(),
}).noUnknown(false); // Allow unknown fields
2. Custom Validators
Sometimes you need validation rules specific to your business logic:
const customSchema = Yup.object().shape({
orderStatus: Yup.string()
.test('valid-status-transition', 'Invalid status transition', function(value) {
const currentStatus = this.parent.currentStatus;
const validTransitions = {
'pending': ['processing', 'cancelled'],
'processing': ['completed', 'failed'],
// ... more status transitions
};
return validTransitions[currentStatus]?.includes(value);
})
});
3. Error Handling Strategies
Implement comprehensive error handling to make debugging easier:
const enhancedValidation = async (schema, data) => {
try {
return await schema.validate(data, {
abortEarly: false,
context: { environment: process.env.NODE_ENV }
});
} catch (err) {
if (err.name === 'ValidationError') {
// Transform Yup errors into a more useful format
const formattedErrors = err.inner.reduce((acc, error) => ({
...acc,
[error.path]: error.message
}), {});
throw new ApiValidationError({
message: 'Response validation failed',
errors: formattedErrors,
originalData: data
});
}
throw err;
}
};
4. TypeScript Integration
When using TypeScript, leverage Yup's type inference capabilities:
import * as Yup from 'yup';
const userSchema = Yup.object({
id: Yup.number().required(),
name: Yup.string().required(),
email: Yup.string().email().required()
});
// Infer the type from the schema
type User = Yup.InferType<typeof userSchema>;
// Now TypeScript knows the exact shape of your data
const processUser = (user: User) => {
// TypeScript provides full type safety here
console.log(user.name.toUpperCase());
};
Common Pitfalls and Solutions
1. Handling Nullable Fields
One common issue is dealing with fields that might be null:
const schema = Yup.object().shape({
optionalField: Yup.string().nullable(),
conditionalField: Yup.string().when('optionalField', {
is: null,
then: Yup.string().required(),
otherwise: Yup.string()
})
});
2. Dealing with Arrays
Validating arrays of objects requires special attention:
const itemSchema = Yup.object().shape({
id: Yup.number().required(),
name: Yup.string().required()
});
const arraySchema = Yup.object().shape({
items: Yup.array()
.of(itemSchema)
.min(1, 'At least one item is required')
.required()
});
3. Circular References
When dealing with self-referential data structures:
const commentSchema = Yup.object().shape({
id: Yup.number().required(),
content: Yup.string().required(),
replies: Yup.lazy(() =>
Yup.array().of(commentSchema)
)
});
Conclusion
Implementing robust API response validation with Yup is a crucial step in building reliable applications. While it might seem like extra work initially, the benefits of catching data inconsistencies early and having self-documenting schemas far outweigh the setup costs.
Remember these key takeaways:
Always validate API responses to protect against unexpected data structures
Use schema caching and selective validation for performance optimization
Implement comprehensive error handling
Leverage TypeScript integration for better type safety
Consider business logic in your validation rules
By following these practices, you'll build more robust applications that can handle API responses gracefully and maintain data integrity throughout your application.