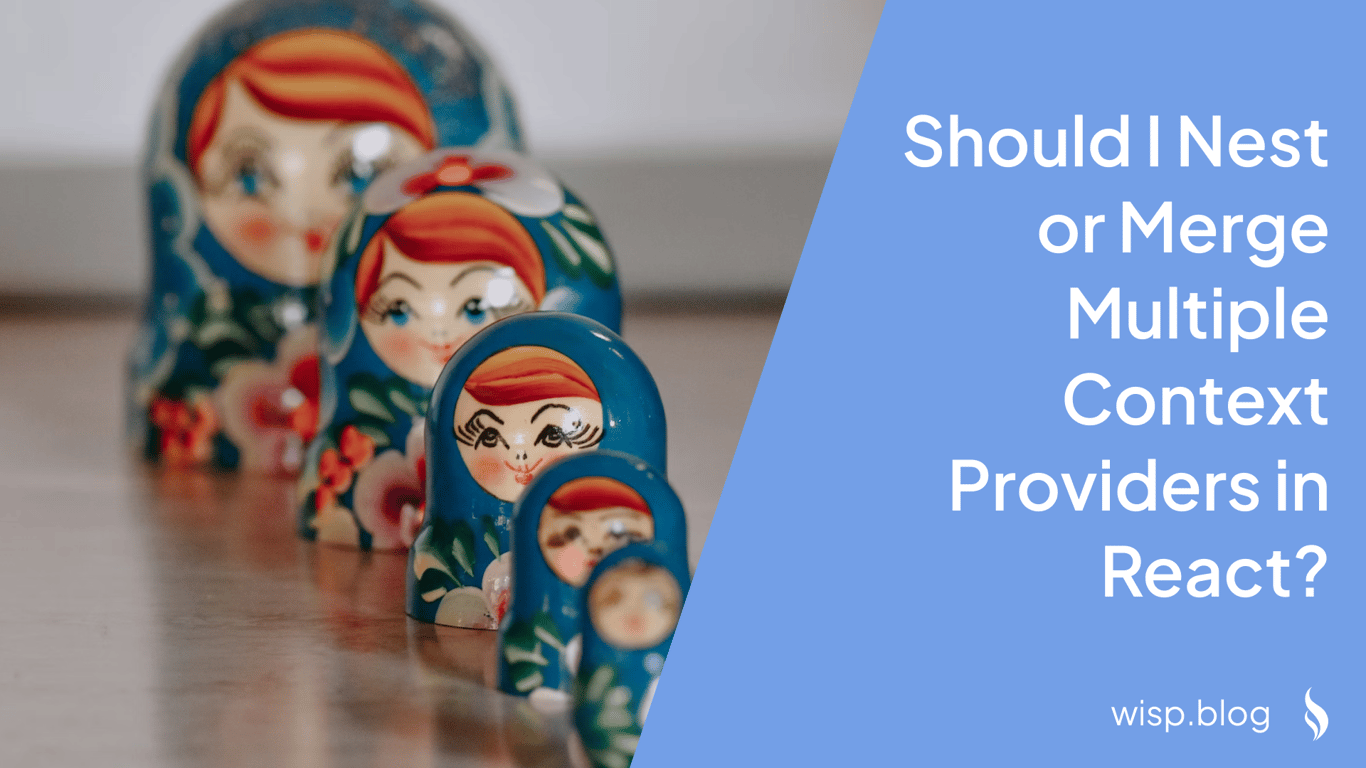
You've built a React application that's growing in complexity. As you add features like authentication, notifications, and theme management, you find yourself wrestling with multiple Context Providers. Your App.tsx
starts looking like a Russian nesting doll of providers:
<AuthProvider>
<ThemeProvider>
<NotificationProvider>
<SettingsProvider>
<App />
</SettingsProvider>
</NotificationProvider>
</ThemeProvider>
</AuthProvider>
You stare at this code, wondering if there's a better way. The nested structure feels messy, and you're concerned about its impact on readability and performance. You're not alone – this is a common pain point for React developers as their applications scale.
The Context Conundrum
React's Context API is a powerful tool for sharing state across components without prop drilling. It's perfect for managing global state like user authentication, theme preferences, or system-wide notifications. However, as applications grow, developers often find themselves managing multiple contexts with different purposes.
The challenge isn't just about having multiple contexts – it's about organizing them effectively while maintaining:
Code readability and maintainability
Optimal performance
Clear separation of concerns
Predictable state updates
Understanding the Trade-offs
Before diving into solutions, let's understand what happens when you use multiple Context Providers:
Nesting Context Providers
// Traditional nested approach
const App = () => (
<AuthProvider>
<ThemeProvider>
<NotificationProvider>
{/* Your app components */}
</NotificationProvider>
</ThemeProvider>
</AuthProvider>
);
Advantages:
Clear visual hierarchy
Explicit dependencies between contexts
Easier to add/remove individual providers
Disadvantages:
Can become visually cluttered
Harder to maintain as the number of providers grows
Potential for unnecessary re-renders if not optimized properly
Merging Context Providers
Alternatively, you can combine multiple providers into a single component:
const combineComponents = (...components) => {
return components.reduce(
(AccumulatedComponents, CurrentComponent) => {
return ({ children }) => (
<AccumulatedComponents>
<CurrentComponent>{children}</CurrentComponent>
</AccumulatedComponents>
);
},
({ children }) => <>{children}</>,
);
};
const AppProviders = combineComponents(
AuthProvider,
ThemeProvider,
NotificationProvider
);
const App = () => (
<AppProviders>
{/* Your app components */}
</AppProviders>
);
Advantages:
Cleaner component tree
Single import for all providers
Easier to maintain in larger applications
Disadvantages:
Less obvious provider relationships
Might make debugging more challenging
Could hide important context dependencies
Best Practices for Context Management
Based on real-world experiences and community feedback, here are proven strategies for managing multiple contexts effectively:
1. Keep Contexts Close to Their Usage
As discussed in the React community, not every context needs to wrap your entire application. For example:
// Bad: Wrapping entire app with MobileMenuProvider
const App = () => (
<MobileMenuProvider>
<EntireApplication />
</MobileMenuProvider>
);
// Good: Scoping MobileMenuProvider to Navigation
const Navigation = () => (
<MobileMenuProvider>
<Nav />
</MobileMenuProvider>
);
This approach reduces unnecessary re-renders and makes your code more maintainable by keeping "related things as close as possible."
2. Optimize for Performance
Context updates can trigger re-renders in all consuming components. Here's how to minimize performance impacts:
// Bad: Frequent context updates
const NotificationContext = React.createContext();
const NotificationProvider = ({ children }) => {
const [notifications, setNotifications] = useState([]);
// This could cause frequent re-renders
const addNotification = (notification) => {
setNotifications(prev => [...prev, notification]);
};
return (
<NotificationContext.Provider value={{ notifications, addNotification }}>
{children}
</NotificationContext.Provider>
);
};
// Good: Optimized updates with memoization
const NotificationProvider = ({ children }) => {
const [notifications, setNotifications] = useState([]);
const addNotification = useCallback((notification) => {
setNotifications(prev => [...prev, notification]);
}, []);
const value = useMemo(() => ({
notifications,
addNotification
}), [notifications, addNotification]);
return (
<NotificationContext.Provider value={value}>
{children}
</NotificationContext.Provider>
);
};
3. Consider a Hybrid Approach
You don't have to choose between completely nested or completely merged providers. Consider grouping related contexts while keeping others separate:
// Group related providers
const UserRelatedProviders = combineComponents(
AuthProvider,
UserPreferencesProvider
);
const UIRelatedProviders = combineComponents(
ThemeProvider,
NotificationProvider
);
// Use them together
const App = () => (
<UserRelatedProviders>
<UIRelatedProviders>
<AppContent />
</UIRelatedProviders>
</UserRelatedProviders>
);
This approach provides a balance between organization and maintainability.
Making the Decision
When deciding between nesting and merging context providers, consider these factors:
Application Size and Complexity
For smaller applications with few contexts, nesting is often simpler and more straightforward
Larger applications benefit from merged providers for better organization
Team Size and Experience
Nested providers are more explicit and easier for new team members to understand
Merged providers require better documentation but offer cleaner code structure
Performance Requirements
If you're experiencing performance issues, merged providers with proper memoization can help
Use React DevTools to profile your application and identify unnecessary re-renders
Maintenance Considerations
Consider how often you'll need to modify the provider structure
Think about debugging needs and how easily you can isolate issues
Conclusion
There's no one-size-fits-all answer to managing multiple context providers in React. The best approach depends on your specific needs:
Use nested providers when:
You have a small number of contexts
You need clear visibility of context relationships
You're working with a team new to React
Use merged providers when:
You have many contexts to manage
Code organization is a priority
You need to optimize performance
Remember that React's Context API is just one tool in your state management toolbox. For some cases, you might want to consider alternatives like Zustand or local state management, especially when dealing with frequent updates or performance-critical features.
The key is to start simple and refactor as needed based on your application's requirements and real-world usage patterns. Don't be afraid to experiment with different approaches – the best solution is the one that works best for your specific use case.