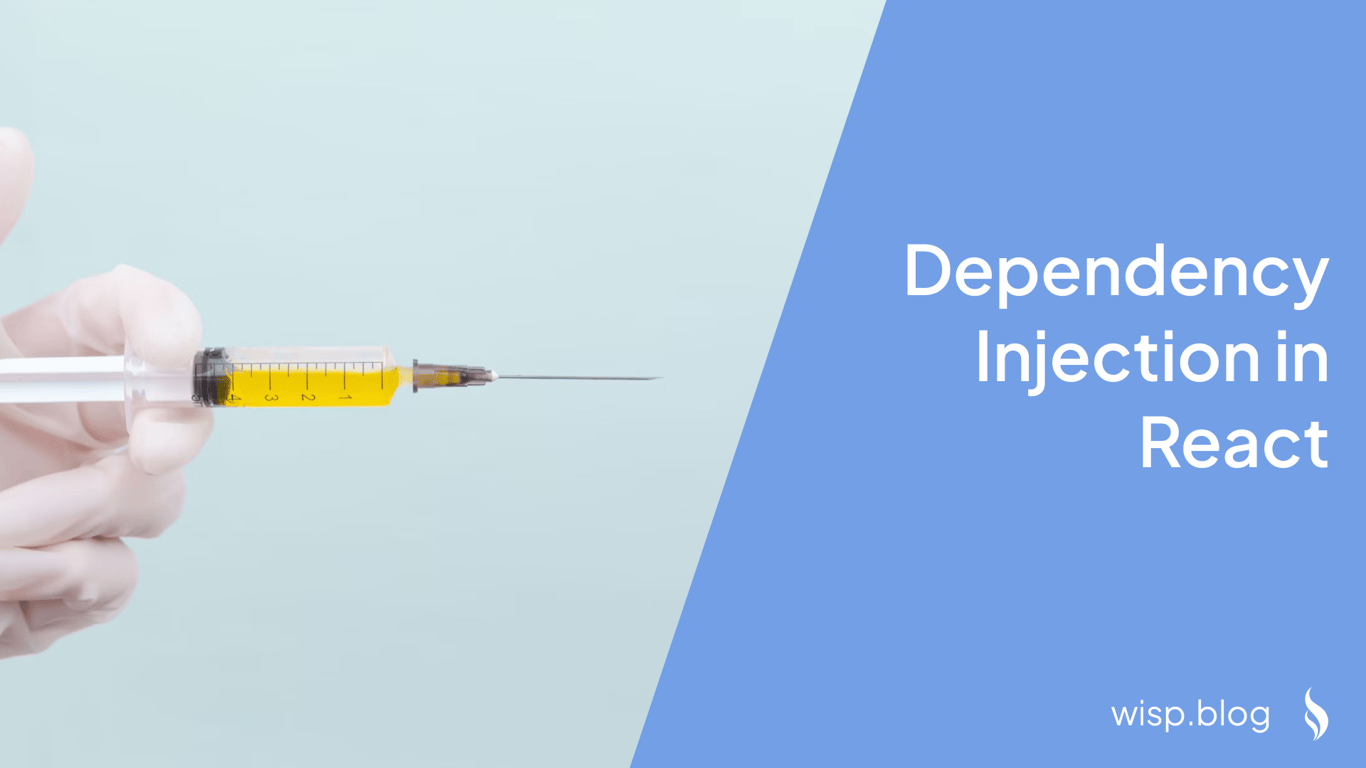
You've built a React component that fetches and displays user data. It works perfectly in your development environment. But when you try to write tests for it, you realize the API calls are hardcoded inside the component. Now you're stuck wondering how to mock these API calls without turning your codebase into an over-engineered mess.
If this sounds familiar, you're not alone. Many React developers struggle with managing dependencies in their components, often questioning whether sophisticated patterns like Dependency Injection (DI) are worth the complexity they introduce.
What is Dependency Injection and Why Should React Developers Care?
At its core, Dependency Injection is about making your components more flexible by providing them with their dependencies from the outside, rather than having them create or manage their own dependencies internally.
Let's look at a common scenario:
// Without Dependency Injection
function UserProfile() {
// Component creates its own API service
const apiService = new UserApiService();
const [userData, setUserData] = useState(null);
useEffect(() => {
apiService.fetchUser(123).then(setUserData);
}, []);
return <div>{userData?.name}</div>;
}
This component works, but it's tightly coupled to UserApiService
. Want to test it with mock data? Good luck. Need to switch to a different API implementation? You'll need to modify the component code.
Here's the same component with Dependency Injection:
// With Dependency Injection
function UserProfile({ apiService }) {
const [userData, setUserData] = useState(null);
useEffect(() => {
apiService.fetchUser(123).then(setUserData);
}, [apiService]);
return <div>{userData?.name}</div>;
}
Now the component receives its dependencies through props, making it:
Easier to test (just pass in a mock service)
More flexible (can work with any service implementation)
More maintainable (dependencies are explicit)
The Reality Check: When Do You Actually Need DI?
Before we dive deeper, let's address the elephant in the room: many developers feel that formal Dependency Injection in React is overkill. As one developer noted on Reddit, "option 1 feels overkill for most React web apps, right?"
They have a point. React's component model and props system already provides a form of dependency injection out of the box. For many simple applications, passing props and using React's Context API might be all you need.
Practical Approaches to Dependency Injection in React
Let's explore three practical approaches to implementing DI in React, starting from the simplest to more sophisticated solutions.
1. Props-Based Injection
The simplest form of dependency injection in React is through props. This approach is perfect for smaller applications or when you're just starting to implement DI.
// Service definition
class AuthService {
login(credentials) {
return fetch('/api/login', {
method: 'POST',
body: JSON.stringify(credentials)
});
}
}
// Component using the service
function LoginForm({ authService }) {
const handleSubmit = async (credentials) => {
await authService.login(credentials);
};
return <form onSubmit={handleSubmit}>...</form>;
}
// Usage
const App = () => {
const authService = new AuthService();
return <LoginForm authService={authService} />;
};
2. React Context for Dependency Injection
When prop drilling becomes cumbersome, React's Context API provides a clean solution for dependency injection. This approach is particularly useful for services that need to be accessed by many components.
// Create a context for your services
const ServicesContext = React.createContext(null);
// Create a provider component
function ServicesProvider({ children }) {
const services = {
auth: new AuthService(),
api: new ApiService(),
analytics: new AnalyticsService()
};
return (
<ServicesContext.Provider value={services}>
{children}
</ServicesContext.Provider>
);
}
// Create a custom hook for accessing services
function useServices() {
const context = useContext(ServicesContext);
if (!context) {
throw new Error('useServices must be used within ServicesProvider');
}
return context;
}
// Use in components
function LoginForm() {
const { auth } = useServices();
const handleSubmit = async (credentials) => {
await auth.login(credentials);
};
return <form onSubmit={handleSubmit}>...</form>;
}
3. Custom Hooks for Business Logic Injection
A more React-idiomatic approach is to encapsulate dependencies within custom hooks. This approach addresses the concern raised by developers who feel traditional DI patterns are "applying a solution to a problem that doesn't really exist" in React.
// Create a hook that encapsulates the service dependency
function useAuth(authService) {
const login = useCallback(async (credentials) => {
try {
await authService.login(credentials);
// Additional logic here
} catch (error) {
// Error handling
}
}, [authService]);
return { login };
}
// Use in components
function LoginForm() {
const { login } = useAuth(new AuthService());
return <form onSubmit={login}>...</form>;
}
Best Practices and Common Pitfalls
Best Practices
Start Simple Don't rush to implement complex DI patterns if props are sufficient. As noted in community discussions, "Not a fan of moving every single tiny callback/helper out of my components". Only extract and inject dependencies when there's a clear benefit.
Maintain Clear Boundaries Keep your service interfaces clean and focused. A common mistake is creating services that do too much, making them harder to mock and test.
// Bad: Service doing too much
class UserService {
async fetchUser(id) { /* ... */ }
async updateProfile(data) { /* ... */ }
async handlePayments(payment) { /* ... */ }
async manageSubscriptions(sub) { /* ... */ }
}
// Good: Focused services
class UserProfileService {
async fetchUser(id) { /* ... */ }
async updateProfile(data) { /* ... */ }
}
class PaymentService {
async processPayment(payment) { /* ... */ }
}
Consider Testing Early Design your DI implementation with testing in mind. This often leads to better architectural decisions.
// Easy to test
function UserProfile({ userService }) {
// ...
}
// In tests
const mockUserService = {
fetchUser: jest.fn()
};
render(<UserProfile userService={mockUserService} />);
Common Pitfalls
Over-engineering One of the most common criticisms of DI in React is over-engineering. As highlighted in community discussions, "this is applying a solution to a problem that doesn't really exist". Keep your implementation proportional to your needs.
Circular Dependencies When using Context for DI, be careful not to create circular dependencies between services:
// Problematic: Circular dependency
class UserService {
constructor(authService) {
this.authService = authService;
}
}
class AuthService {
constructor(userService) {
this.userService = userService;
}
}
// Better: Use interfaces and clear boundaries
class UserService {
constructor(authProvider) {
this.authProvider = authProvider;
}
}
Prop Drilling vs. Context Trade-off Don't immediately reach for Context when you see prop drilling. Sometimes, component composition is a better solution:
// Instead of creating a context for a deeply nested dependency
function ParentComponent({ service }) {
return (
<div>
<SomeComponent>
<AnotherComponent>
<DeepComponent service={service} />
</AnotherComponent>
</SomeComponent>
</div>
);
}
// Consider component composition
function ParentComponent({ service }) {
const deepComponent = <DeepComponent service={service} />;
return (
<div>
<SomeComponent>
<AnotherComponent>
{deepComponent}
</AnotherComponent>
</SomeComponent>
</div>
);
}
Real-World Implementation Strategies
Combining Different Approaches
In practice, you might want to use different DI approaches for different scenarios. Here's a real-world example combining Context and custom hooks:
// Create a services context
const ServicesContext = React.createContext(null);
// Create service instances
const createServices = () => ({
api: new ApiService(),
auth: new AuthService(),
analytics: new AnalyticsService()
});
// Provider component
function ServicesProvider({ children }) {
const [services] = useState(createServices);
return (
<ServicesContext.Provider value={services}>
{children}
</ServicesContext.Provider>
);
}
// Custom hook for specific business logic
function useUserManagement() {
const { api, analytics } = useContext(ServicesContext);
const fetchUser = useCallback(async (id) => {
const user = await api.fetchUser(id);
analytics.trackUserFetch(id);
return user;
}, [api, analytics]);
return { fetchUser };
}
// Component using the hook
function UserProfile({ userId }) {
const { fetchUser } = useUserManagement();
const [user, setUser] = useState(null);
useEffect(() => {
fetchUser(userId).then(setUser);
}, [userId, fetchUser]);
return <div>{user?.name}</div>;
}
Testing Considerations
When implementing DI, always consider how it affects testing. Here's an example of how different DI approaches affect test setup:
// Testing with prop injection
test('UserProfile with prop injection', () => {
const mockService = {
fetchUser: jest.fn().mockResolvedValue({ name: 'Test User' })
};
render(<UserProfile userService={mockService} />);
expect(mockService.fetchUser).toHaveBeenCalled();
});
// Testing with Context
test('UserProfile with context', () => {
const mockServices = {
api: {
fetchUser: jest.fn().mockResolvedValue({ name: 'Test User' })
},
analytics: {
trackUserFetch: jest.fn()
}
};
render(
<ServicesContext.Provider value={mockServices}>
<UserProfile />
</ServicesContext.Provider>
);
});
Conclusion
Dependency Injection in React doesn't have to be complicated. Start with simple props-based injection and gradually adopt more sophisticated patterns as your application grows. Remember that the goal is to make your code more maintainable and testable, not to implement patterns for their own sake.
As one developer wisely noted on Reddit, the best approach is to "use it whenever a component gets too big (and composition can't help) or when some logic must be reusable." This pragmatic approach helps you avoid over-engineering while still benefiting from the advantages of dependency injection.
Whether you choose props, Context, custom hooks, or a combination of these approaches, the key is to maintain a balance between flexibility and simplicity that works for your specific use case.