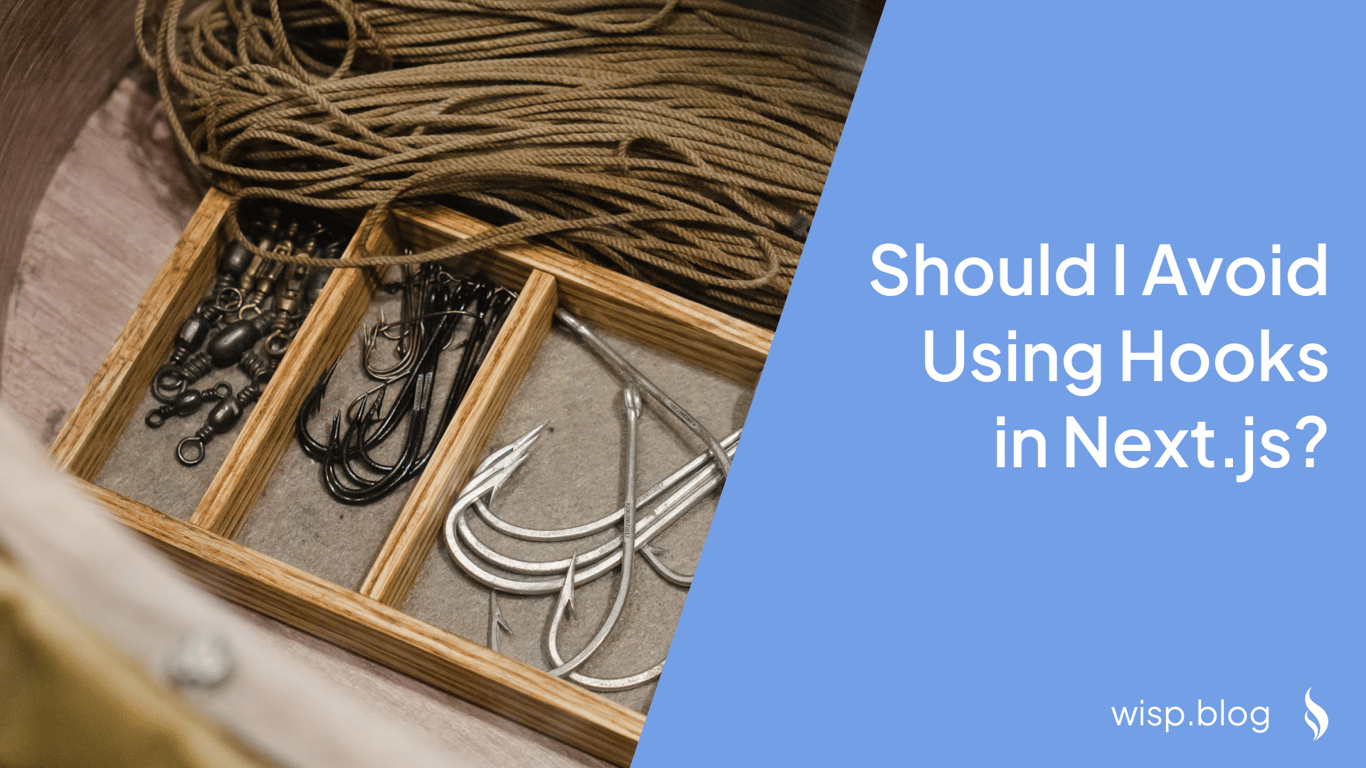
You've just migrated your React application to Next.js, and suddenly you're bombarded with warnings about hooks, server components, and mysterious 'use client' directives. Your team is raising concerns about server optimization and caching, leaving you wondering if you should abandon hooks altogether. Sound familiar?
The confusion is real, especially when you're trying to fetch data in server components and pass it to client components marked with 'use client'. You might have heard Next.js developers warning that "hooks are bad for server optimization" – but what does this really mean, and is it true?
Let's clear up this confusion once and for all.
Understanding the Next.js Component Model
Before diving into whether hooks are "good" or "bad," it's crucial to understand how Next.js handles components differently from traditional React applications. Next.js introduces two fundamental types of components:
Server Components: These are the default in Next.js 13+ and run exclusively on the server. They're perfect for:
Fetching data
Accessing backend resources directly
Keeping sensitive information server-side
Reducing client-side JavaScript
Client Components: These are components marked with the 'use client' directive and run on the browser. They're essential for:
Interactive features
State management
Browser APIs
Event listeners
Here's where the confusion often starts: Server Components cannot use hooks. This isn't because hooks are "bad," but because Server Components don't maintain state or handle interactivity – they're purely for rendering static content and handling server-side operations.
The Truth About Hooks in Next.js
Let's address the elephant in the room: Hooks are not bad in Next.js. In fact, they're essential for building interactive applications. The key is understanding where and how to use them.
Consider this common scenario:
// This won't work in a Server Component
function ServerComponent() {
const [count, setCount] = useState(0); // ❌ Error!
return <button onClick={() => setCount(count + 1)}>{count}</button>;
}
// This works perfectly in a Client Component
'use client';
function ClientComponent() {
const [count, setCount] = useState(0); // ✅ Works great!
return <button onClick={() => setCount(count + 1)}>{count}</button>;
}
The error in the first example isn't because hooks are harmful – it's because Server Components aren't designed to handle client-side interactivity. They're optimized for different purposes.
The Server Component Advantage
You might be wondering: "If hooks don't work in Server Components, why not make everything a Client Component?" This is where understanding Next.js's optimization strategy becomes crucial.
Server Components offer several significant advantages:
Reduced JavaScript Bundle Size: Server Components don't send any JavaScript to the client, resulting in smaller bundle sizes and faster page loads.
Improved SEO: Search engines can better index content rendered on the server.
Better Performance: Server-side rendering can be faster than client-side rendering, especially for data-heavy components.
Enhanced Security: Sensitive operations can be performed server-side without exposing logic to the client.
Consider this real-world example:
// ProductList.js (Server Component)
async function ProductList() {
const products = await fetchProducts(); // Runs on server
return (
<div>
{products.map(product => (
<ProductCard key={product.id} product={product} />
))}
</div>
);
}
// ProductCard.js (Client Component)
'use client';
function ProductCard({ product }) {
const [isInCart, setIsInCart] = useState(false);
return (
<div>
<h2>{product.name}</h2>
<button onClick={() => setIsInCart(!isInCart)}>
{isInCart ? 'Remove from Cart' : 'Add to Cart'}
</button>
</div>
);
}
In this pattern, the heavy lifting of data fetching happens on the server, while the interactive elements are handled by the client component with hooks. This is the ideal separation of concerns that Next.js encourages.
Understanding 'use client' and 'use server'
The 'use client' and 'use server' directives are Next.js's way of helping you define boundaries in your application. Here's how they work:
The 'use client' Directive
'use client';
// This component and all its children will be client-side
export default function InteractiveComponent() {
const [data, setData] = useState(null);
useEffect(() => {
// Client-side effects are fine here
fetchData().then(setData);
}, []);
return <div>{/* Interactive content */}</div>;
}
When you add 'use client' to a file:
All components in that file become Client Components
You can use hooks freely
The code runs in the browser
The component and its children are bundled together
The 'use server' Directive
// action.js
'use server';
export async function submitForm(formData) {
// This runs on the server
const result = await processFormData(formData);
return result;
}
The 'use server' directive:
Marks functions as server-side only
Allows secure data processing
Can be called from Client Components
Doesn't support hooks
Best Practices for Using Hooks in Next.js
To make the most of hooks while maintaining optimal performance, follow these guidelines:
1. Keep Server Components as the Default
Start with Server Components and only add 'use client' when you need interactivity or client-side features. This approach, known as the "server-first" pattern, ensures optimal performance.
// Layout.js (Server Component)
export default function Layout({ children }) {
const user = await getUser(); // Server-side operation
return (
<div>
<Header user={user} />
{children}
</div>
);
}
// Header.js (Client Component)
'use client';
export default function Header({ user }) {
const [isMenuOpen, setIsMenuOpen] = useState(false);
// Interactive menu logic here
}
2. Colocate Related Client Components
When you need multiple interactive components, group them together to minimize the number of Client Component boundaries:
// Bad: Multiple separate client components
'use client';
function Button() { /* ... */ }
'use client';
function Input() { /* ... */ }
// Good: Colocated client components
'use client';
function Button() { /* ... */ }
function Input() { /* ... */ }
3. Custom Hooks for Reusable Logic
Create custom hooks to encapsulate common client-side logic:
'use client';
function useFormValidation(initialValues) {
const [values, setValues] = useState(initialValues);
const [errors, setErrors] = useState({});
// Validation logic here
return { values, errors, setValues };
}
export function Form() {
const { values, errors, setValues } = useFormValidation({
email: '',
password: ''
});
// Form implementation
}
Common Pitfalls and How to Avoid Them
1. Hydration Errors
One of the most common issues developers face is hydration errors, which occur when the server-rendered content doesn't match what the client tries to render:
// ❌ Bad: This can cause hydration errors
function Component() {
const [mounted, setMounted] = useState(false);
useEffect(() => {
setMounted(true);
}, []);
return mounted ? <ClientOnlyComponent /> : null;
}
// ✅ Better: Use suspense for client-only components
import dynamic from 'next/dynamic';
const ClientOnlyComponent = dynamic(() => import('./ClientOnlyComponent'), {
ssr: false
});
function Component() {
return (
<Suspense fallback={<Loading />}>
<ClientOnlyComponent />
</Suspense>
);
}
2. Unnecessary Client Components
A common mistake is making entire pages client components when only small parts need interactivity:
// ❌ Bad: Making the whole page a client component
'use client';
export default function Page() {
return (
<div>
<StaticHeader /> {/* Doesn't need to be client-side */}
<StaticContent /> {/* Doesn't need to be client-side */}
<InteractiveForm /> {/* Needs to be client-side */}
</div>
);
}
// ✅ Better: Keep the page as a server component
export default function Page() {
return (
<div>
<StaticHeader />
<StaticContent />
<ClientForm /> {/* Only this component marked with 'use client' */}
</div>
);
}
3. Data Fetching in Client Components
While it's possible to fetch data in Client Components using hooks, it's often better to fetch data on the server:
// ❌ Bad: Fetching data in client component
'use client';
function ProductList() {
const [products, setProducts] = useState([]);
useEffect(() => {
fetch('/api/products').then(res => res.json()).then(setProducts);
}, []);
return <div>{/* Render products */}</div>;
}
// ✅ Better: Fetch in server component, pass to client
async function ProductList() {
const products = await fetch('/api/products').then(res => res.json());
return <ClientProductList products={products} />;
}
'use client';
function ClientProductList({ products }) {
// Handle interactivity here
return <div>{/* Render products */}</div>;
}
Performance Optimization Strategies
When using hooks in Next.js, consider these optimization strategies:
1. Lazy Loading Client Components
Use dynamic imports for large client components that aren't immediately needed:
import dynamic from 'next/dynamic';
const HeavyClientComponent = dynamic(() => import('./HeavyClientComponent'), {
loading: () => <LoadingSpinner />
});
2. Proper State Management
Choose the right state management approach based on your needs:
// Local state with useState
'use client';
function Counter() {
const [count, setCount] = useState(0);
return <button onClick={() => setCount(count + 1)}>{count}</button>;
}
// Global state with Context
'use client';
const GlobalState = createContext();
function Provider({ children }) {
const [state, setState] = useState(initialState);
return (
<GlobalState.Provider value={{ state, setState }}>
{children}
</GlobalState.Provider>
);
}
3. Caching Considerations
Understand how your use of hooks affects caching:
// Server Component with caching
export default async function CachedComponent() {
const cachedData = await fetch('https://api.example.com/data', {
next: { revalidate: 3600 } // Cache for 1 hour
});
return <ClientComponent initialData={cachedData} />;
}
// Client Component using cached data
'use client';
function ClientComponent({ initialData }) {
const [data, setData] = useState(initialData);
// Handle updates without affecting server cache
}
Making the Decision: Server vs. Client Components
When deciding whether to use hooks (and thus, Client Components), ask yourself:
Does this component need interactivity?
Does it require browser APIs?
Does it need to respond to user events?
Does it need to maintain client-side state?
If you answered "yes" to any of these questions, use a Client Component with hooks. If not, keep it as a Server Component.
Real-World Examples
Let's look at some common scenarios and how to handle them effectively:
Authentication Flow
// app/layout.js (Server Component)
export default async function RootLayout({ children }) {
const session = await getSession();
return (
<html>
<body>
<AuthProvider session={session}>
{children}
</AuthProvider>
</body>
</html>
);
}
// components/AuthProvider.js (Client Component)
'use client';
export function AuthProvider({ session, children }) {
const [currentSession, setCurrentSession] = useState(session);
useEffect(() => {
// Handle session updates
}, []);
return <SessionContext.Provider value={currentSession}>{children}</SessionContext.Provider>;
}
Data Grid with Sorting and Filtering
// app/products/page.js (Server Component)
export default async function ProductsPage() {
const initialProducts = await fetchProducts();
return <ProductsGrid initialProducts={initialProducts} />;
}
// components/ProductsGrid.js (Client Component)
'use client';
export function ProductsGrid({ initialProducts }) {
const [products, setProducts] = useState(initialProducts);
const [sortBy, setSortBy] = useState('name');
const [filters, setFilters] = useState({});
useEffect(() => {
// Handle sorting and filtering
const sortedProducts = sortProducts(products, sortBy);
const filteredProducts = applyFilters(sortedProducts, filters);
setProducts(filteredProducts);
}, [sortBy, filters]);
return (
<div>
<SortControls onSort={setSortBy} />
<FilterControls onFilter={setFilters} />
<Grid data={products} />
</div>
);
}
Form with Real-time Validation
// components/ContactForm.js (Client Component)
'use client';
export function ContactForm() {
const { values, errors, handleChange, handleSubmit } = useForm({
initialValues: {
name: '',
email: '',
message: ''
},
validate: (values) => {
const errors = {};
if (!values.email.includes('@')) {
errors.email = 'Invalid email';
}
return errors;
},
onSubmit: async (values) => {
'use server';
await submitForm(values);
}
});
return (
<form onSubmit={handleSubmit}>
<Input
value={values.email}
onChange={handleChange}
error={errors.email}
/>
{/* Other form fields */}
</form>
);
}
Conclusion
So, should you avoid using hooks in Next.js? The answer is a resounding no. Hooks are an essential part of building interactive React applications, and Next.js fully supports them in Client Components. The key is understanding where and how to use them effectively.
Remember these key points:
Server Components First: Start with Server Components and only add Client Components (and hooks) when needed for interactivity.
Strategic Component Split: Divide your application into Server and Client Components based on their responsibilities:
Use Server Components for data fetching and static content
Use Client Components (with hooks) for interactive features
Performance Optimization: Leverage Next.js's built-in optimizations:
Keep data fetching on the server when possible
Use dynamic imports for large Client Components
Implement proper caching strategies
Best Practices:
Colocate related Client Components
Create custom hooks for reusable logic
Avoid unnecessary Client Components
Handle hydration carefully
By following these guidelines, you can build performant, interactive applications that take full advantage of both Server Components and hooks in Next.js.
Additional Resources
Remember, the goal isn't to avoid hooks but to use them wisely in conjunction with Next.js's powerful server-side capabilities. This combination allows you to build fast, interactive, and maintainable applications that provide the best possible user experience.