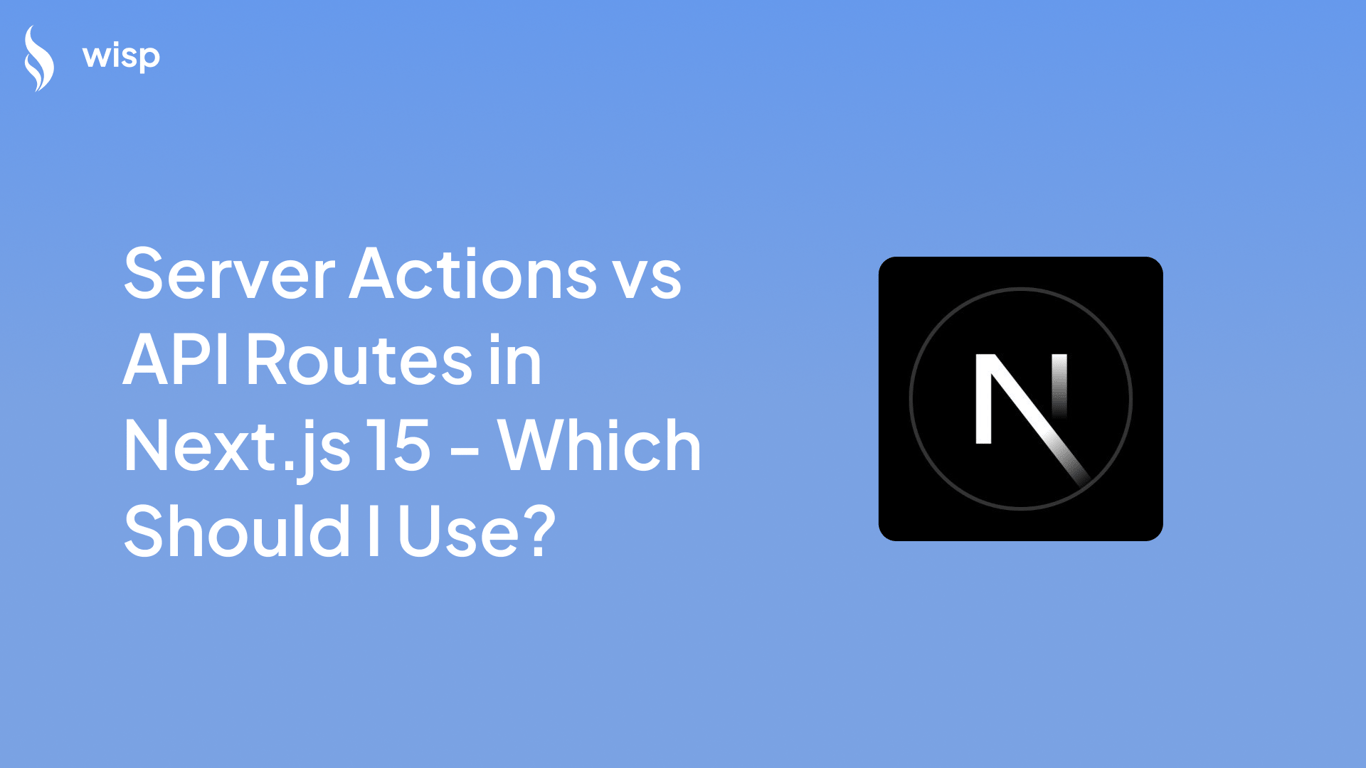
Over the years, Next.js has evolved into a robust framework for building React applications, providing a smoother developer experience and optimizing the performance of web applications. With the release of Next.js 15, developers are presented with new features, including enhanced Server Actions and API Routes.
This article aims to provide an authoritative and detailed comparison between Server Actions and API Routes in Next.js 15, helping you determine the best approach for your specific use case.
Overview of Next.js 15
Next.js 15 comes with a plethora of updates focused on improving security, performance, and the overall developer experience. Key highlights include:
Server Actions Security: Enhanced measures such as dead code elimination, secure action IDs, and public endpoint awareness.
API Routes: Significant changes to caching behavior and client router caching.
Async Request APIs: APIs now asynchronous for handling request-specific data.
React 19 Support: Compatibility with React 19, with backward compatibility for React 18.
Hydration Error Improvements: Enhanced error messages and visual indicators for static vs. dynamic routes.
Turbopack Dev Stabilization: Faster local server startup and improved development speeds.
Enhanced Component: Extends HTML
<form>
element features.Self-Hosting Improvements: Better cache-control directives and automated image optimization.
Bundling External Packages: New options for App Router and Pages Router.
ESLint 9 Support: Compatibility and new config format.
Server Components HMR: Improved hot module replacement for server components.
Faster Static Generation: Optimized static generation process.
Detailed Analysis of Server Actions
What Are Server Actions?
Server Actions, introduced in React 19, are asynchronous functions running on the server, used in both Server and Client Components. These are defined with the "use server"
directive and can be invoked from forms, event handlers, or third-party libraries. Their primary function is to handle form submissions and data mutations within Next.js applications.
Usage in Server Components
Server Components can define actions inline or at the module level with "use server"
. Here is an example:
async function createNoteAction() {
"use server";
await db.notes.create();
}
Usage in Client Components
Client Components can import Server Actions and use them within event handlers or form submissions.
"use client";
import { createNoteAction } from './actions';
function ClientComponent() {
return <button onClick={() => createNoteAction()}>Create Note</button>;
}
Passing Server Actions as Props
You can pass Server Actions as props to Client Components, enabling more flexible interactions.
"use client";
export default function ClientComponent({ updateItemAction }) {
return <form action={updateItemAction}>{/* ... */}</form>;
}
Error Handling and Security
Server Actions are designed to treat as public HTTP endpoints, where Next.js generates secure action IDs that are encrypted and periodically recalculated. For error handling, use the try/catch
blocks.
"use server";
export async function createUser() {
try {
// Mutate data
} catch (e) {
throw new Error('Failed to create user');
}
}
Revalidation and Caching
Use revalidatePath
or revalidateTag
to manage cache after Server Actions mutate data.
"use server";
import { revalidatePath } from 'next/cache';
export async function createPost() {
// Mutate data
revalidatePath('/posts');
}
Integration with Next.js
Server Actions can optimize data fetching and manage API routes, ensuring better performance for server-side rendering (SSR) and static site generation (SSG).
Understanding API Routes
Overview of API Routes
API Routes in Next.js allow you to create custom request handlers for given routes, using the Web and APIs. This feature is available only inside the app
directory and follows the convention of defining handlers within route.js
or route.ts
files.
Supported HTTP Methods
API Routes support multiple HTTP methods including GET
, POST
, PUT
, PATCH
, DELETE
, HEAD
, and OPTIONS
. By default, unsupported methods return a 405 Method Not Allowed
response.
Example of API Routes
Basic GET Handler// app/api/hello/route.js
export async function GET(request) {
return new Response('Hello, Next.js 15!');
}
Basic POST Handler// app/api/submit/route.js
export async function POST(request) {
const body = await request.json();
return new Response(`Received data: ${JSON.stringify(body)}`);
}
Advanced Use Cases
Fetching Data from APIs// app/api/product/route.js
export async function GET(request) {
const { searchParams } = new URL(request.url);
const id = searchParams.get('id');
const res = await fetch(`https://api.example.com/product/${id}`);
const product = await res.json();
return new Response(JSON.stringify(product), {
headers: { 'Content-Type': 'application/json' },
});
}
Handling Query Parameters// app/api/items/route.js
export async function GET(request) {
const { searchParams } = new URL(request.url);
const category = searchParams.get('category');
const res = await fetch(`https://api.example.com/items?category=${category}`);
const items = await res.json();n return new Response(JSON.stringify(items), {
headers: { 'Content-Type': 'application/json' },
});
}
Integrating with Databases// app/api/users/route.js
import { PrismaClient } from '@prisma/client';
const prisma = new PrismaClient();
export async function POST(request) {
try {
const body = await request.json();
const { name, email } = body;
const newUser = await prisma.user.create({
data: {
name,
email,
},
});
return new Response(JSON.stringify(newUser), {
headers: { 'Content-Type': 'application/json' },
status: 201,
});
} catch (error) {
return new Response(`Error: ${error.message}`, { status: 500 });
} finally {
await prisma.$disconnect();
}
}
Key Differences Between Server Actions and API Routes
Performance and Efficiency
Server Actions are inherently more efficient for data mutations that need to run on the server since they streamline the process by avoiding the client-server round-trip. API Routes, on the other hand, are ideal for creating more traditional RESTful APIs where multiple HTTP methods and complex routing logic are required.
Use Cases
Server Actions: Best suited for form submissions, server-side data mutations, and scenarios requiring secure, server-side processing within Server or Client Components.
API Routes: Ideal for building RESTful APIs, handling complex routing, and managing multiple HTTP methods for various endpoints.
Security
Both Server Actions and API Routes need to be treated with security in mind. Server Actions come with built-in secure action IDs and periodic recalculation, while API Routes necessitate careful implementation to avoid common security pitfalls.
Practical Examples
Server Action Example
// app/actions.ts
"use server";
export async function createUser(formData) {
const userData = {
name: formData.get('name'),
email: formData.get('email'),
};
// Perform user creation logic
}
// app/ui/form.tsx
"use client";
import { createUser } from './actions';
export function UserForm() {
return (
<form action={createUser}>
<input type="text" name="name" />
<input type="email" name="email" />
<button type="submit">Create User</button>
</form>
);
}
API Route Example
// app/api/create-user/route.js
import { PrismaClient } from '@prisma/client';
const prisma = new PrismaClient();
export async function POST(request) {
const body = await request.json();
const { name, email } = body;
const newUser = await prisma.user.create({
data: {
name,
email,
},
});
return new Response(JSON.stringify(newUser), {
headers: { 'Content-Type': 'application/json' },
status: 201,
});
}
Best Practices and Recommendations
Choose Server Actions when working within the context of a single component and needing efficient, server-side data mutations without additional HTTP overhead.
Opt for API Routes when building comprehensive APIs involving multiple endpoints and HTTP methods, or when a clear separation of concerns between client and server is needed.
Security: Always treat both Server Actions and API Routes as public endpoints, ensuring proper authentication and validation.
Performance: Consider the caching strategies and the asynchronous nature of these APIs to optimize performance.
Code Maintenance: Keep your codebase organized by clearly separating Server Actions and API Routes based on their purpose and usage context.
Conclusion
Next.js 15 has introduced powerful new features with Server Actions and API Routes, each serving distinct purposes and fitting different use cases. Understanding when to use each approach can significantly impact the performance, security, and maintainability of your application. By leveraging the strengths of both Server Actions and API Routes, you can build robust, efficient, and scalable applications with Next.js 15.