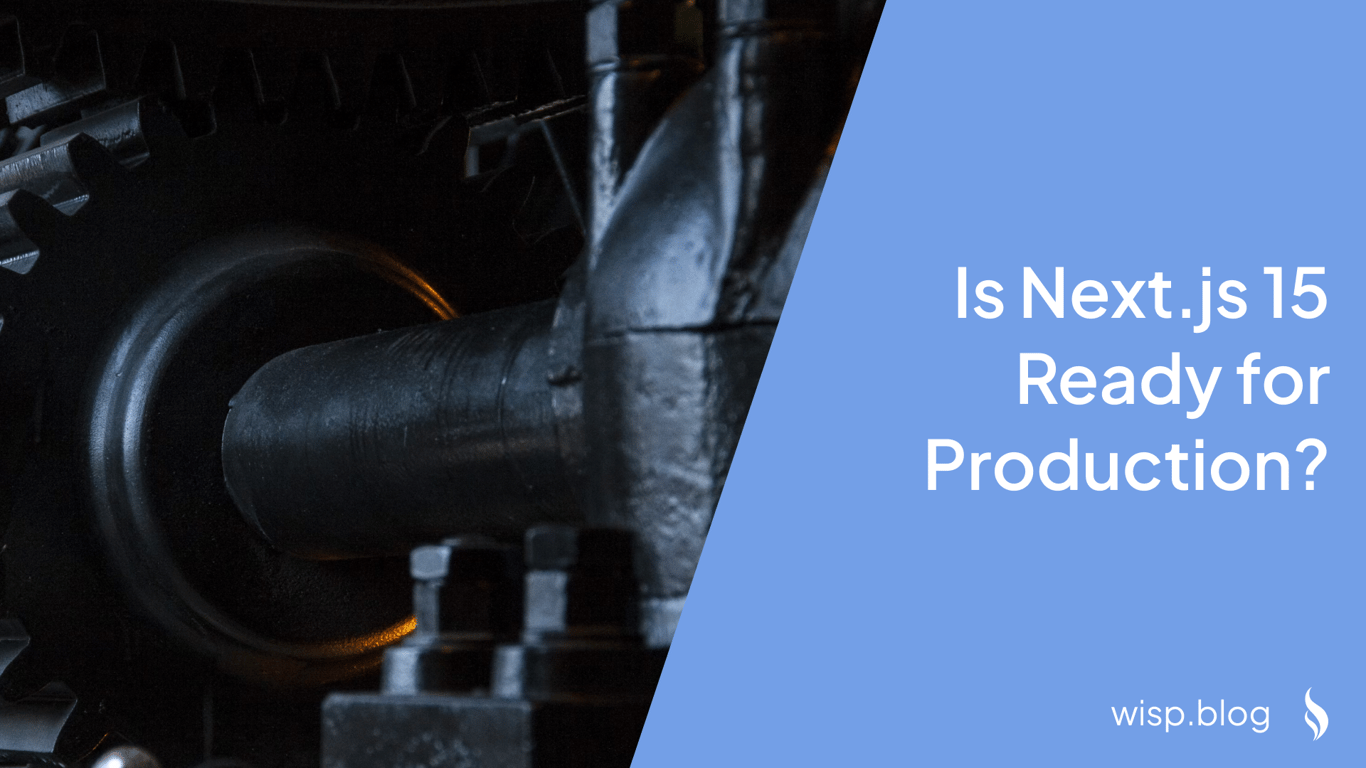
You've probably seen the buzz around Next.js 15's release, promising groundbreaking performance improvements and seamless React 19 integration. But as you scroll through Reddit discussions and GitHub issues, you're met with a mix of excitement and skepticism. Some developers praise the blazing-fast Turbopack improvements, while others express frustration over breaking changes and ecosystem compatibility.
"The problem isn't that the app router is unstable," one developer notes on Reddit. "The issue is that the surrounding ecosystem hasn't caught up. Dependencies you want to use may not support the app router, or their documentation still references the pages directory."
This sentiment echoes across the development community, leaving many wondering: Is Next.js 15 truly ready for production use? Let's dive deep into what's new, what's changed, and most importantly - whether you should make the leap.
The Promise of Next.js 15
Next.js 15 arrives with ambitious claims:
Up to 76.7% faster local server startup
96.3% faster code updates with Turbopack Dev
Seamless React 19 RC integration
Enhanced security features
Improved developer experience
But beneath these impressive numbers lies a more complex reality that deserves careful consideration.
Breaking Changes and Ecosystem Impact
The transition to Next.js 15 introduces several significant changes that might affect your existing projects:
React 19 Integration: While Next.js 15 supports React 19 RC (Release Candidate), this early adoption has raised eyebrows in the development community. As one developer pointed out on Reddit, "If one were to even go look at any large companies using Next.js, most of them are still on version 12 or 13."
Caching Behavior: GET route handlers are no longer cached by default, addressing previous confusion but potentially requiring updates to your caching strategy.
Server Components and Data Handling: The distinction between server-only queries and client components continues to be a source of confusion. As one developer expressed, "I couldn't use those queries if my form was on a client component."
These changes represent just the tip of the iceberg, and their impact varies depending on your project's complexity and requirements.
Real-World Developer Experiences
The Good: Performance and Security Improvements
Next.js 15's performance improvements are substantial and measurable. The Turbopack integration delivers on its promises:
Development Speed: Local development environment startup times have been dramatically reduced, with some developers reporting near-instant refreshes.
Build Optimization: Static site generation (SSG) and overall build times show significant improvements, especially for larger applications.
Security Enhancements: The introduction of unguessable action IDs and improved server-side security measures addresses previous vulnerabilities.
The Challenges: Documentation and Migration
However, developers are encountering several pain points:
Error Handling Opacity: One developer shared a particularly frustrating experience: "If you have an error outside a route handler function (e.g., while importing something), the route will just be 404 without any error logged anywhere. This lost me a few infuriating hours."
Documentation Gaps: "Some of the 'magic' specified in the doc just doesn't work," another developer notes, highlighting issues with features like favicon handling.
Manual Configuration Requirements: "You have to manually handle everything, the static generation, the canonicals, the fallbacks, everything..." This increased complexity has been a common complaint among developers transitioning to newer versions.
Making the Decision: Should You Upgrade?
The decision to upgrade to Next.js 15 should be based on several key factors:
Factors to Consider
Project Stage and Scale
For new projects: Starting with Next.js 15 might make sense if you're prepared to handle potential ecosystem incompatibilities
For existing projects: Consider the effort required to migrate and test all functionalities
Team Experience
Teams familiar with Next.js's app router will adapt more easily
Less experienced teams might struggle with the increased complexity and documentation gaps
Dependencies and Ecosystem
Check if your critical dependencies support Next.js 15
Evaluate the stability of alternative solutions if needed
Performance Requirements
If you need the performance improvements offered by Turbopack
Whether your application would benefit from React 19's features
Migration Strategy
If you decide to upgrade, here's a structured approach to minimize disruption:
1. Preparation Phase
# Create a new branch for the upgrade
git checkout -b next-15-upgrade
# Use the new automated upgrade CLI
npx @next/codemod@canary upgrade latest
# ...or upgrade manually
npm install next@latest react@rc react-dom@rc
2. Systematic Testing
Before proceeding with the upgrade in production:
Audit Dependencies
Review all dependencies for Next.js 15 compatibility
Look for alternative packages if needed
Test Critical Paths
Focus on server-side operations
Verify client component functionality
Check data fetching patterns
Common Issues and Solutions
1. Server-Side Data Management
One of the most discussed challenges is the confusion around server-only queries versus client components. Here's how to handle it:
// queries.ts (server-only)
import 'server-only'
export async function getData() {
// Your data fetching logic
}
// actions.ts
'use server'
export async function updateData(data: FormData) {
// Your mutation logic
}
2. Error Handling Improvements
To address the silent failures mentioned by developers:
// Add error boundary at the route level
export default function ErrorBoundary({
error,
reset,
}: {
error: Error
reset: () => void
}) {
console.error(error)
return (
<div>
<h2>Something went wrong!</h2>
<button onClick={() => reset()}>Try again</button>
</div>
)
}
3. Caching Strategy
With the changes to default caching behavior:
// Route handler with explicit caching
export async function GET() {
const res = await fetch('https://api.example.com/data', {
next: {
revalidate: 3600 // Cache for 1 hour
}
})
return Response.json(await res.json())
}
Performance Optimization Tips
When working with Next.js 15, consider these optimization strategies:
1. Intelligent Component Splitting
// Prefer this approach for dynamic imports
const DynamicComponent = dynamic(() => import('./Heavy'), {
loading: () => <p>Loading...</p>,
ssr: false // For client-only components
})
2. Route Optimization
// Configure route segments
export const dynamic = 'force-dynamic' // or 'force-static'
export const revalidate = 3600 // seconds
export default async function Page() {
// Your component logic
}
3. Image Optimization
// Implement responsive images
import Image from 'next/image'
export default function OptimizedImage() {
return (
<Image
src="/hero.jpg"
alt="Hero image"
width={1200}
height={600}
priority
sizes="(max-width: 768px) 100vw,
(max-width: 1200px) 50vw,
33vw"
/>
)
}
These optimizations can help mitigate some of the performance concerns developers have expressed about Next.js 15.
Conclusion: Is Next.js 15 Production-Ready?
The answer to whether Next.js 15 is production-ready isn't a simple yes or no. It depends heavily on your specific use case and requirements.
When to Adopt Next.js 15
✅ Consider Upgrading If:
You're starting a new project and can afford some initial setup complexity
Your team is experienced with Next.js and React
You need the performance improvements offered by Turbopack
Your project doesn't rely heavily on third-party packages that might have compatibility issues
❌ Consider Waiting If:
You have a complex existing application with many dependencies
Your team is new to Next.js or the app router
You require extensive third-party package support
You need rock-solid stability for critical applications
Final Thoughts
Next.js 15 represents a significant step forward in terms of performance and features. However, as one developer wisely noted on Reddit, "The surrounding ecosystem hasn't caught up." This means that while the core framework may be stable, the broader development experience might still have rough edges.
For those ready to embrace the changes, Next.js 15 offers exciting possibilities. But there's no shame in waiting for the ecosystem to mature further. As with any major upgrade, the key is to make an informed decision based on your specific circumstances rather than following the hype.
Remember: The best technology choice is the one that serves your project's needs while considering your team's capabilities and constraints.
This article is based on real developer experiences and discussions from the Next.js community. For the latest updates and detailed documentation, always refer to the official Next.js documentation.
(latest update on 2 Nov 2024)