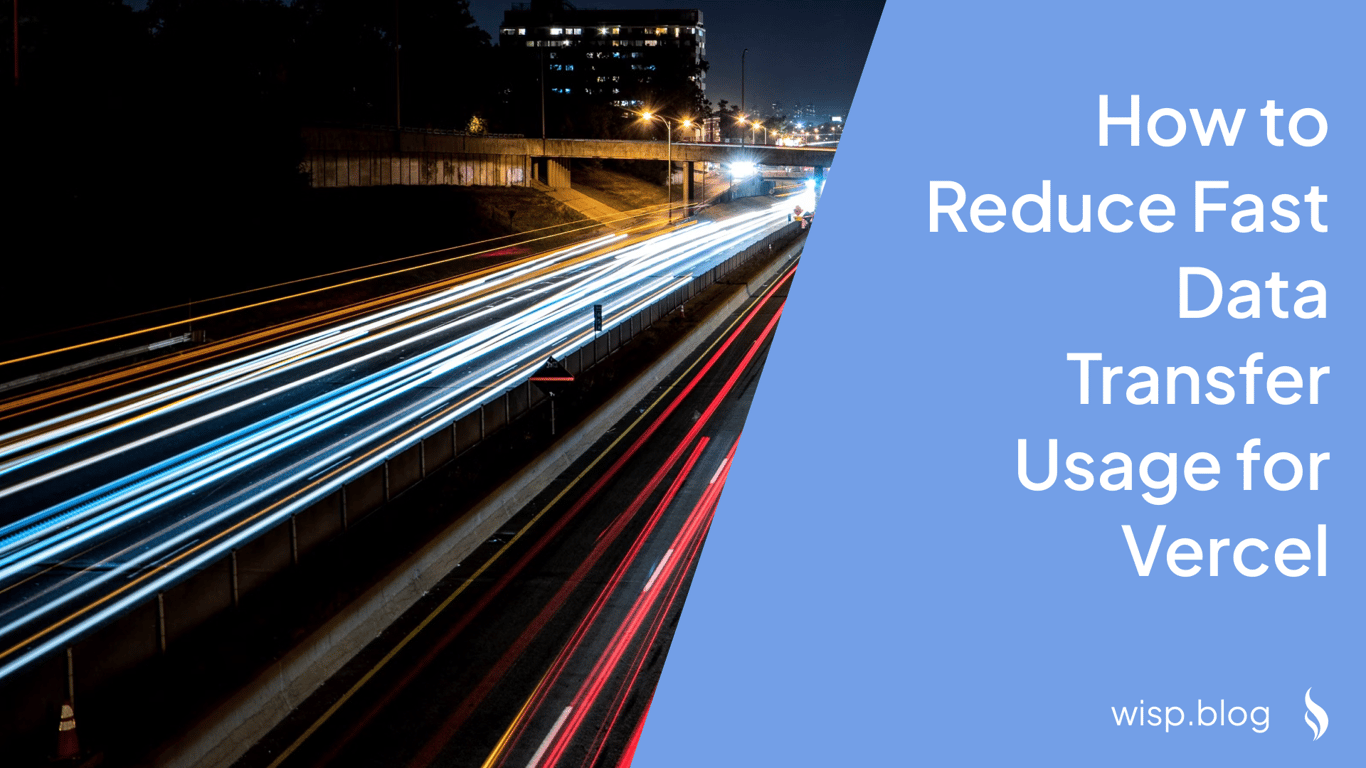
You've just checked your Vercel dashboard and your heart sinks - your Fast Data Transfer (FDT) usage is skyrocketing, consuming gigabytes of data every few minutes. What's worse, you're rapidly approaching Vercel's 1TB limit, and the thought of additional charges at $0.15 per GB is keeping you up at night.
If you're seeing messages like "Fast Data Transfer outgoing usage extremely high" or watching your usage climb by several gigabytes every 15 minutes, you're not alone. Many developers have found themselves in this predicament, especially when dealing with image-heavy Next.js applications.
The situation becomes even more stressful when you realize you might need to shut down your site within days if you can't find a solution. Perhaps you thought the Next.js Image component's lazy loading would prevent this issue, but the reality is more complex - especially when dealing with animated GIFs and other large media files.
But don't worry - there's hope. By implementing the right optimization strategies and understanding how Vercel handles data transfer, you can significantly reduce your FDT usage while maintaining your site's performance and visual quality.
Understanding Fast Data Transfer (FDT) on Vercel
Before diving into solutions, it's crucial to understand what Fast Data Transfer actually is and why it matters:
Fast Data Transfer measures the amount of data transferred between Vercel's Edge Network and your end users. This includes:
Images served through the Next.js Image component
Static files and assets
API responses
HTML, CSS, and JavaScript bundles
Under Vercel's pricing structure:
Hobby Plan users get 100 GB included
Pro Plan users get 1 TB included
Beyond these limits, you're charged $0.15 per GB
The costs can add up quickly, especially if you're serving large images or animated GIFs without proper optimization. For instance, a single unoptimized 3MB image viewed by 1000 users could consume 3GB of your FDT allowance - and that's just one image!
Key Strategies to Reduce FDT Usage
1. Optimize Your Image Component Implementation
The Next.js Image component is powerful but needs to be configured correctly to maximize its benefits:
import Image from 'next/image';
// Good Implementation
const OptimizedImage = () => {
return (
<Image
src="/path/to/image.jpg"
width={600}
height={400}
quality={75} // Adjust based on your needs
priority={false} // Only set to true for above-the-fold images
loading="lazy"
/>
);
};
Key considerations:
Set appropriate
quality
values (default is 75)Use
priority
only for critical above-the-fold imagesEnsure proper
width
andheight
attributes to prevent layout shiftLeverage the automatic WebP/AVIF conversion
2. Implement Effective Caching Strategies
One of the most effective ways to reduce FDT usage is through proper caching. Here's how to implement it:
// In your Next.js API routes or middleware
export async function middleware(request) {
const response = await fetch(request);
// Set cache headers
const headers = new Headers(response.headers);
headers.set('Cache-Control', 'public, max-age=86400, s-maxage=86400, stale-while-revalidate=59');
return new Response(response.body, {
status: response.status,
headers
});
}
For images specifically, you can configure caching in your next.config.js
:
module.exports = {
images: {
minimumCacheTTL: 60, // Cache images for at least 60 seconds
deviceSizes: [640, 750, 828, 1080, 1200], // Limit size variations
imageSizes: [16, 32, 48, 64, 96], // Limit size variations
},
};
3. Special Handling for GIFs
GIFs present a unique challenge because they're not optimized by the Next.js Image component. Here's how to handle them effectively:
Option 1: Use a Cloudflare Worker// Cloudflare Worker code
const CACHE_TIME = 60 * 60 * 24; // 24 hours
const CACHE_HEADERS = {
'Cache-Control': `public, max-age=${CACHE_TIME}, immutable`,
};
export default {
async fetch(request) {
const url = new URL(request.url);
const imageUrl = url.searchParams.get('url');
const response = await fetch(imageUrl, {
method: 'GET',
cf: {
cacheEverything: true,
cacheTtl: CACHE_TIME,
},
});
return new Response(response.body, {
status: response.status,
headers: {
...response.headers,
...CACHE_HEADERS,
},
});
},
};
Option 2: Use Traditional HTML TagsFor GIFs, sometimes the simplest solution is the best:
// Instead of Next.js Image component
<img
src="/path/to/your.gif"
alt="Description"
loading="lazy"
/>
4. Implement Image Compression Before Upload
Before your images even reach your Next.js application, ensure they're properly compressed:
Use tools like TinyJPG to compress images
Convert large GIFs to video formats when possible
Consider using modern formats like WebP with fallbacks:
<picture>
<source srcSet="/path/to/image.webp" type="image/webp" />
<source srcSet="/path/to/image.jpg" type="image/jpeg" />
<img src="/path/to/image.jpg" alt="Description" loading="lazy" />
</picture>
5. Monitor and Analyze Your FDT Usage
Regular monitoring is crucial for maintaining optimal FDT usage:
Use Vercel's Dashboard:
Monitor the Fast Data Transfer chart
Pay special attention to paths ending in
_next/image
Set up alerts for unusual spikes in usage
Implement logging for large transfers:
// Custom middleware to log large transfers
export async function middleware(request) {
const response = await fetch(request);
const contentLength = response.headers.get('content-length');
if (contentLength > 1000000) { // Log transfers over 1MB
console.warn(`Large transfer detected: ${request.url} (${contentLength} bytes)`);
}
return response;
}
Best Practices and Common Pitfalls
To avoid common issues that lead to excessive FDT usage:
Don't serve original GIFs through the Image component
Use video formats for animated content
Implement Cloudflare caching for GIFs when necessary
Optimize your cache strategy
Use appropriate Cache-Control headers
Implement stale-while-revalidate where suitable
Consider using a CDN for static assets
Regular monitoring and optimization
Review your FDT usage weekly
Identify and address spikes promptly
Keep your Next.js and dependencies updated
Conclusion
Managing Fast Data Transfer usage on Vercel doesn't have to be a constant source of stress. By implementing proper image optimization, effective caching strategies, and special handling for GIFs, you can significantly reduce your FDT usage while maintaining excellent performance.
Remember to:
Properly configure the Next.js Image component
Implement appropriate caching strategies
Handle GIFs separately with Cloudflare Workers or traditional tags
Compress images before upload
Monitor your usage regularly
With these strategies in place, you can keep your FDT usage well within limits while providing an excellent user experience for your visitors.