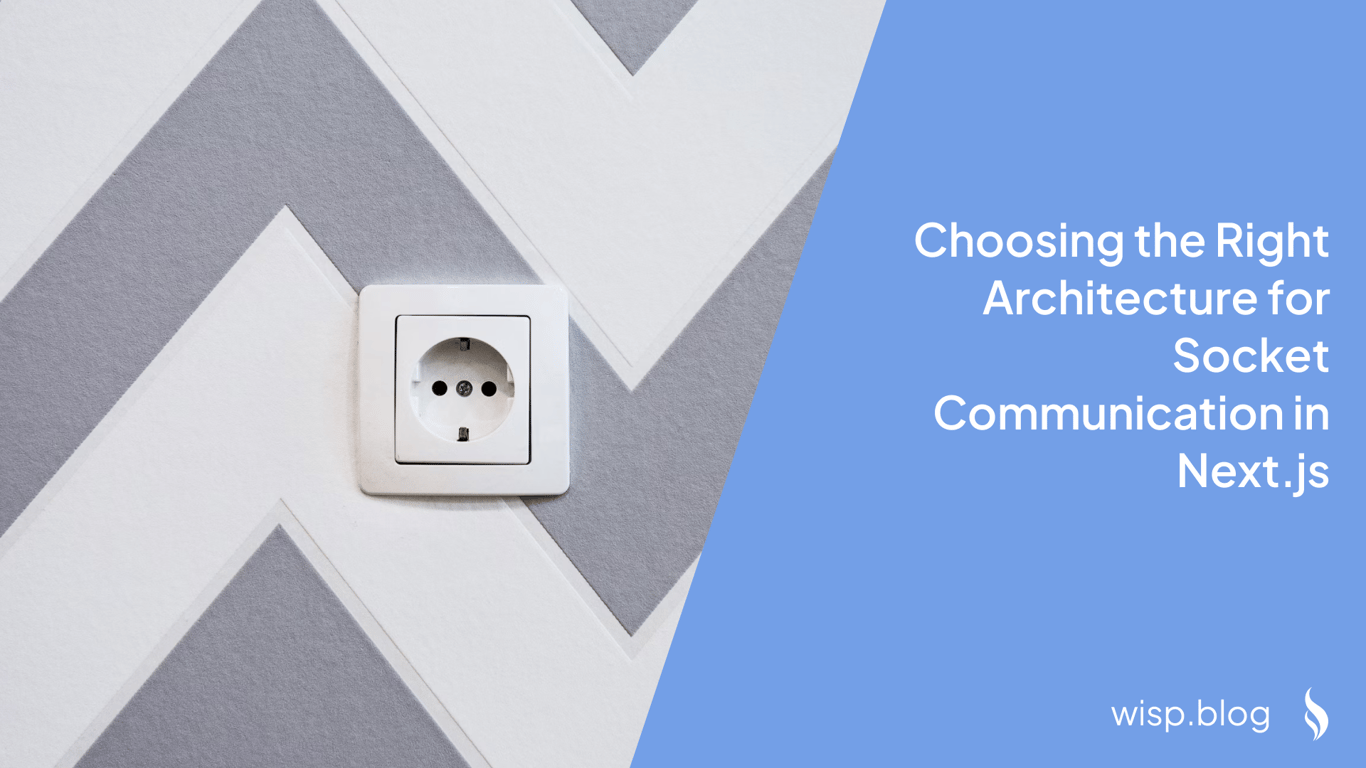
You're building a Next.js application that requires real-time communication - maybe it's a chat application, a game server interface, or a live monitoring dashboard. But as you dig deeper into implementation details, you're faced with a crucial architectural decision: should you use Next.js with a singleton for RCON connection and custom server for socket handling? Or perhaps Next.js combined with Express.js? Or should you just stick with vanilla React and Express?
The choice isn't always straightforward, especially when you're dealing with constraints like Vercel's hosting limitations or specific performance requirements. Let's dive deep into each approach and help you make an informed decision.
Understanding Your Options
Next.js with Singleton Pattern and Custom Server
This approach involves using Next.js as your primary framework while implementing a singleton pattern for managing RCON (Remote Console) connections and a custom server for handling WebSocket communications.
The singleton pattern ensures you maintain only one instance of your RCON connection throughout your application's lifecycle, which is particularly useful for managing server resources efficiently. Meanwhile, the custom server gives you the flexibility to handle WebSocket connections alongside your Next.js application.
Here's what makes this approach attractive:
Centralized Connection Management: The singleton pattern prevents multiple unnecessary connections to your RCON server, reducing resource usage and potential connection conflicts.
Full Control: Custom server implementation gives you complete control over how WebSocket connections are handled and managed.
Integrated Solution: Everything stays within your Next.js application, potentially simplifying deployment and maintenance.
However, there are some considerations:
Moving away from Next.js's built-in server can impact some of its optimization features
Custom server implementation requires additional setup and maintenance
You'll need to carefully manage server resources, especially in a serverless environment
Next.js + Express.js Architecture
This architecture separates your application into two distinct parts: a Next.js frontend and an Express.js backend server handling sockets and shell commands. According to recent discussions, this approach has gained popularity, especially for projects requiring robust real-time features.
Key benefits include:
Clear Separation of Concerns: Frontend and backend logic remain distinctly separated
Flexible Deployment Options: Your socket server can be hosted independently of your Next.js application
Scalability: Each part can be scaled independently based on needs
The Express.js backend can be hosted on platforms that support persistent connections, such as AWS EC2 or dedicated servers, while your Next.js frontend can still benefit from Vercel's optimized hosting and CDN capabilities.
Consider this architecture when:
Your application requires persistent WebSocket connections
You need to handle complex backend operations
You want to maintain separate scaling strategies for frontend and backend
Vanilla React + Express
The third option is to forgo Next.js entirely and opt for a traditional React frontend with an Express backend. This approach might seem counterintuitive given Next.js's popularity, but it has its merits:
Simplicity: No need to navigate Next.js-specific concepts and constraints
Familiar Territory: Many developers are already comfortable with this stack
Direct Control: Straightforward implementation of WebSocket connections without framework-specific considerations
Key Considerations for Your Choice
Real-time Communication Requirements
The nature of your real-time communication needs should heavily influence your architectural decision. According to developer discussions, consider:
Message Frequency: How often do you need to send/receive updates?
Connection Persistence: Do you need long-lived connections?
Scalability Requirements: How many concurrent connections do you expect?
Hosting and Deployment Constraints
Your hosting environment plays a crucial role in architectural decisions. For instance, if you're planning to use Vercel, you'll need to account for its limitations regarding WebSocket connections:
Vercel's serverless functions don't support WebSocket connections
You'll need a separate service for handling WebSocket connections
Consider the additional complexity in deployment and maintenance
Performance and Scalability
When it comes to performance and scalability, each architecture presents different challenges and opportunities:
Next.js with Singleton PatternMemory Management: The singleton pattern helps control memory usage by maintaining a single connection instance
Connection Pooling: Easier to implement connection pooling strategies
Resource Optimization: Better control over server resources
Independent Scaling: Frontend and backend can scale separately
Resource Distribution: Better distribution of computational resources
Flexible Deployment: Can optimize each component independently
Direct Control: More straightforward performance optimization
Less Overhead: Fewer layers of abstraction
Simplified Debugging: Easier to identify performance bottlenecks
Implementation Best Practices
1. Setting Up WebSocket Connections
Regardless of your chosen architecture, follow these best practices for WebSocket implementation:
// Example of WebSocket setup with Socket.IO
import { io } from 'socket.io-client';
const socket = io('your-websocket-server', {
reconnectionDelayMax: 10000,
reconnection: true,
reconnectionAttempts: 5
});
socket.on('connect', () => {
console.log('Connected to WebSocket server');
});
socket.on('disconnect', () => {
console.log('Disconnected from WebSocket server');
});
2. Managing RCON Connections
When implementing RCON connections, especially with the singleton pattern:
// Example of RCON singleton implementation
class RCONManager {
private static instance: RCONManager;
private connection: any;
private constructor() {
// Initialize RCON connection
}
public static getInstance(): RCONManager {
if (!RCONManager.instance) {
RCONManager.instance = new RCONManager();
}
return RCONManager.instance;
}
// Add methods for RCON operations
}
3. Error Handling and Recovery
Implement robust error handling and recovery mechanisms:
// Example of error handling in WebSocket connections
socket.on('error', (error) => {
console.error('WebSocket error:', error);
// Implement recovery logic
});
process.on('uncaughtException', (error) => {
console.error('Uncaught Exception:', error);
// Implement graceful shutdown
});
Making Your Decision
To choose the right architecture for your project, consider these factors:
Project Requirements
Scale of real-time communication needed
Complexity of backend operations
Development team expertise
Infrastructure Constraints
Hosting platform limitations
Budget considerations
Deployment requirements
Development Experience
Team familiarity with technologies
Development timeline
Maintenance requirements
Conclusion
The choice between Next.js with singleton pattern, Next.js + Express.js, or vanilla React + Express ultimately depends on your specific needs and constraints. Here's a quick decision guide:
Choose Next.js with Singleton Pattern if you need a tightly integrated solution and can manage the custom server implementation effectively.
Opt for Next.js + Express.js if you require clear separation of concerns and need to scale components independently.
Consider Vanilla React + Express if simplicity and direct control are your priorities.
Remember that there's no one-size-fits-all solution. The best architecture is the one that meets your specific requirements while considering your team's capabilities and your project's constraints.
For further reading and implementation details, check out these resources:
By carefully considering these aspects and following the best practices outlined above, you'll be well-equipped to implement a robust and scalable solution for your real-time communication needs in your Next.js application.