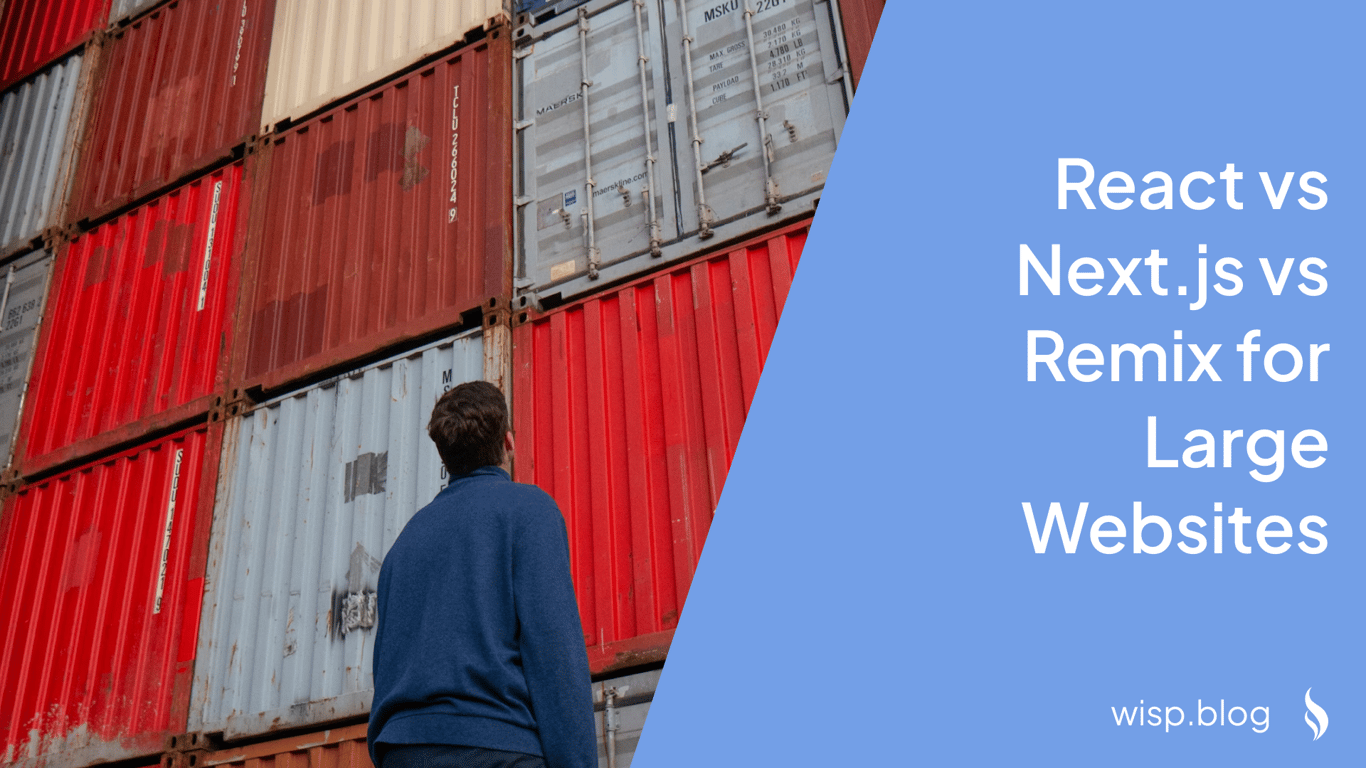
You're tasked with selecting a technology stack for a large-scale website project. As you browse through countless articles and developer discussions, you're bombarded with conflicting opinions about React, Next.js, and Remix. The stakes are high - your choice will impact development speed, performance, and maintenance costs for years to come.
"I'm facing a lot of issues with Next 15 SSR for infinite scroll!" laments one developer on Reddit. Another chimes in, "I'm so sick of App Router, I'd rather kill the app and move to simple React with Vite." These frustrations are real, and they highlight the complexity of choosing the right framework for large-scale applications.
The good news? You can make an informed decision by understanding each framework's strengths, limitations, and real-world performance characteristics. This guide will help you navigate these waters with confidence.
Understanding the Landscape
React: The Foundation
React remains the industry standard for building user interfaces, powering countless websites and applications. Its component-based architecture and vast ecosystem make it a compelling choice for large projects. However, as one developer notes, "React stopped for a long time at least on 18.2. Performance hasn't gotten better either." This stagnation has led many teams to explore enhanced frameworks built on top of React.
Next.js: The Enterprise Choice
Next.js has emerged as the "safe choice" for enterprise projects, offering a robust framework that extends React's capabilities. It provides built-in features like:
Server-side rendering (SSR)
Static site generation (SSG)
Incremental static regeneration (ISR)
Image optimization
API routes
As one developer puts it, "No one ever got fired for choosing Next.js." This sentiment reflects its position as a battle-tested solution for large-scale applications.
Remix: The New Contender
Remix takes a fresh approach to web development, focusing on web fundamentals and optimal user experience. It excels in:
Server-side rendering
Nested routing
Data loading
Error handling
Progressive enhancement
Key Considerations for Large Websites
1. Performance and Scalability
ReactPros: Lightweight core library, flexible architecture
Cons: Requires additional setup for SSR and optimization
Real-world insight: "Most apps don't need mad performance. Most apps aren't big tech," notes one developer. However, for large websites, performance optimization becomes crucial.
Pros: Built-in performance optimizations, automatic code splitting
Cons: Some developers report SSR implementation challenges
Real-world insight: "Strictly we want the first render to be plain HTML, even though Google now supports hydration." Next.js handles this requirement well through its various rendering strategies.
Pros: Optimized for performance on slow networks
Cons: Smaller ecosystem compared to Next.js
Real-world insight: Remix's approach to data loading and progressive enhancement can significantly improve perceived performance.
2. Developer Experience and Learning Curve
React// Simple React component example
function ProductList({ products }) {
return (
<div>
{products.map(product => (
<ProductCard key={product.id} {...product} />
))}
</div>
);
}
Next.js// Next.js page with SSR
export async function getServerSideProps() {
const products = await fetchProducts();
return {
props: { products }
};
}
Remix// Remix loader example
export async function loader() {
const products = await getProducts();
return json({ products });
}
3. Cost Considerations
A critical factor often overlooked is the total cost of ownership. As one developer shares, "I'm hoping we can come together as a community to share our experiences and recommendations to create a cost-effective, yet powerful, tech stack."
Framework-Specific Challenges and Solutions
React Challenges
Server-Side Rendering
Challenge: Implementing SSR requires additional setup and configuration
Solution: Consider using frameworks like Next.js or Remix that provide SSR out of the box
Performance Optimization
Challenge: "Performance hasn't gotten better either," as noted by developers
Solution: Implement code splitting, lazy loading, and proper state management
Next.js Pain Points
App Router Complexity
Challenge: "I'm fucking sick of App Router," expresses one frustrated developer
Solution: Consider using the Pages Router for simpler applications or when team familiarity is a concern
SSR Implementation
Challenge: "Facing a lot of issues with Next 15 SSR for infinite scroll!"
Solution:
// Implement efficient infinite scroll using React Query import { useInfiniteQuery } from 'react-query'; function ProductList() { const { data, fetchNextPage, hasNextPage, } = useInfiniteQuery('products', fetchProductPage); }
Remix Considerations
Ecosystem Size
Challenge: Smaller community compared to Next.js
Solution: Evaluate if the built-in features compensate for fewer third-party packages
Database Integration
Challenge: Different approach to data loading
Solution: Leverage Remix's loader pattern for efficient data fetching
// Efficient data loading in Remix export async function loader({ request }) { const products = await db.product.findMany(); return json({ products }); }
Making the Decision
Choose React When:
You need maximum flexibility
Your team has strong React expertise
You're building a single-page application
You want to maintain full control over your architecture
Choose Next.js When:
You need robust SSR and SSG capabilities
You want a battle-tested enterprise solution
You require strong SEO optimization
You need a hybrid rendering approach
Choose Remix When:
Network performance is crucial
You prefer a more opinionated framework
You want excellent error handling
Your application requires nested routing
Cost-Effective Implementation Strategies
Authentication and Authorization
As highlighted in community discussions, authentication services can be a significant cost factor. "Clerk is the biggest cost. That's something to think about.." Consider alternatives like:
Supabase for authentication and database needs
Self-hosted solutions for better cost control
Open-source alternatives when appropriate
Performance Optimization Tips
Image Optimization
// Next.js Image component for automatic optimization import Image from 'next/image'; export default function ProductImage({ src, alt }) { return ( <Image src={src} alt={alt} width={500} height={300} loading="lazy" /> ); }
Code Splitting
// Dynamic imports for better performance const ProductDetails = dynamic(() => import('./ProductDetails'), { loading: () => <LoadingSpinner /> });
Conclusion
The choice between React, Next.js, and Remix for large websites depends on various factors including:
Team expertise and preferences
Project requirements and constraints
Performance needs
Budget considerations
SEO requirements
While Next.js remains a "safe choice" with its robust feature set and strong community support, both React and Remix offer compelling alternatives depending on your specific needs. Remember that "most apps don't need mad performance," but choosing the right framework can significantly impact your project's success.
Consider starting with a proof of concept (POC) using your framework of choice to validate your decision before committing to a full implementation. This approach allows you to identify potential challenges early and adjust your strategy accordingly.
Additional Resources
Remember, there's no one-size-fits-all solution. The best choice is the one that aligns with your team's capabilities, project requirements, and business goals while providing a sustainable path forward for your large-scale website.