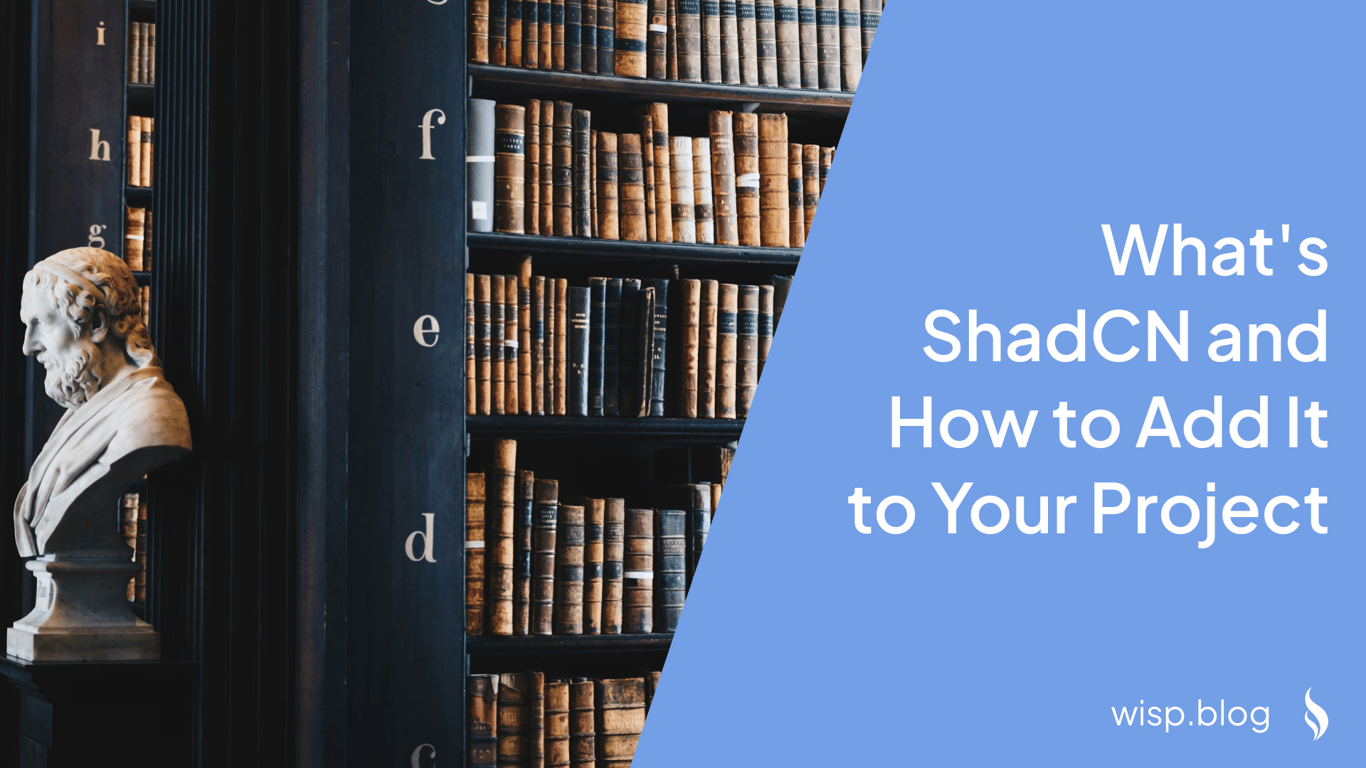
In the fast-paced world of web development, the demand for highly customizable and easily integrable components is ever-growing. Enter ShadCN – a revolutionary component library designed to streamline the development process and enhance productivity. This article delves into what ShadCN is, its origins, features, community reception, and how to seamlessly integrate it into your projects using the ShadCN CLI tool.
History and Origins
ShadCN was created with the vision of providing developers with beautifully designed components that can be directly integrated into web applications. Unlike traditional component libraries that often come with predefined styles and dependencies, ShadCN emphasizes flexibility and total control. The source code is open-source, inviting developers to contribute and customize components to fit their specific needs. This approach has resonated well within the developer community, making ShadCN a popular choice for building modern web applications.
Key Features and Benefits
ShadCN offers a variety of features designed to simplify and accelerate the development process:
Components
ShadCN provides a wide array of pre-designed components that can be easily customized and integrated into projects. This allows developers to maintain consistency and save time on design and implementation.
Blocks
Blocks are modular sections that combine multiple ShadCN components to build complex layouts and designs effortlessly. This feature is particularly useful for creating dashboards, authentication pages, and other intricate interfaces.
Charts
With a focus on data visualization, ShadCN includes customizable chart components built on top of Recharts. These components allow developers to present data in a visually appealing and informative manner.
Themes
ShadCN offers a flexible theming system that enables developers to create and switch between different themes using CSS variables. This ensures a consistent design aesthetic across the entire application.
Examples
To help developers get started quickly, ShadCN provides several example implementations that demonstrate how to effectively use its components. These examples cover a range of applications, from simple forms to complex dashboards.
Colors
A comprehensive color palette is available to ensure visual consistency throughout the application. This palette can be tailored to fit the specific branding requirements of any project.
Core Technologies
ShadCN leverages several core technologies to deliver its powerful features:
Radix UI: Provides headless, accessible UI components that give structure and behavior without tying down the styling.
Tailwind CSS: Uses a utility-first approach to CSS, enabling rapid UI development.
TypeScript: Ensures strong typing and an improved development experience.
ShadCN CLI Tool
One of the standout features of ShadCN is its Command Line Interface (CLI) tool. This tool significantly enhances the extensibility and usability of ShadCN by allowing developers to add components, themes, hooks, utilities, and more directly from the command line. Here’s an in-depth look at how to use the ShadCN CLI and the benefits it offers.
Getting Started with ShadCN CLI
The ShadCN CLI can be used to quickly bootstrap and integrate ShadCN components into your existing projects. Here’s a step-by-step guide on how to get started:
Initializing a Project: Navigate to your project directory and run the initialization command. This sets up ShadCN in your project.
npx shadcn@latest init
Adding Components: Once your project is set up, you can start adding components. For example, to add a button component, you would run:
npx shadcn@latest add button
Custom URLs: The CLI tool also supports adding components from any URL. This is particularly useful for integrating externally hosted components or custom creations.
npx shadcn@latest add --url <component-url>
Example: Adding a Component from Shadcn
To demonstrate how easy it is to add components with Shadcn, let's try adding a button to our app:
You can now start adding components to your project.
npx shadcn@latest add button
The command above will add the
Button
component to your project. You can then import it like this:import { Button } from "@/components/ui/button" export default function Home() { return ( <div> <Button>Click me</Button> </div> ) }
The list of components that are offered by Shadcn CLI is available in their component documentations.
Example: Adding a Marquee from 3rd Party
To further demonstrate the CLI tool’s flexibility, let’s walk through adding an infinite scrolling component that can be used to display text, images, or videos into a Next.js project:
Add the Component: Use the ShadCN CLI to add the Marquee component from MagicUI. For this example, we’ll use a pre-created component URL.
shadcn init npx shadcn@latest add "https://magicui.design/r/marquee"
Integrate the Component: Import and use the component in your Next.js application.
// pages/index.js import { cn } from "@/lib/utils"; import Marquee from "@/components/magicui/marquee"; const reviews = [ { name: "Jack", username: "@jack", body: "I've never seen anything like this before. It's amazing. I love it.", img: "https://avatar.vercel.sh/jack", }, { name: "Jill", username: "@jill", body: "I don't know what to say. I'm speechless. This is amazing.", img: "https://avatar.vercel.sh/jill", }, { name: "John", username: "@john", body: "I'm at a loss for words. This is amazing. I love it.", img: "https://avatar.vercel.sh/john", }, { name: "Jane", username: "@jane", body: "I'm at a loss for words. This is amazing. I love it.", img: "https://avatar.vercel.sh/jane", }, { name: "Jenny", username: "@jenny", body: "I'm at a loss for words. This is amazing. I love it.", img: "https://avatar.vercel.sh/jenny", }, { name: "James", username: "@james", body: "I'm at a loss for words. This is amazing. I love it.", img: "https://avatar.vercel.sh/james", }, ]; const firstRow = reviews.slice(0, reviews.length / 2); const secondRow = reviews.slice(reviews.length / 2); const ReviewCard = ({ img, name, username, body, }: { img: string; name: string; username: string; body: string; }) => { return ( <figure className={cn( "relative w-64 cursor-pointer overflow-hidden rounded-xl border p-4", // light styles "border-gray-950/[.1] bg-gray-950/[.01] hover:bg-gray-950/[.05]", // dark styles "dark:border-gray-50/[.1] dark:bg-gray-50/[.10] dark:hover:bg-gray-50/[.15]", )} > <div className="flex flex-row items-center gap-2"> <img className="rounded-full" width="32" height="32" alt="" src={img} /> <div className="flex flex-col"> <figcaption className="text-sm font-medium dark:text-white"> {name} </figcaption> <p className="text-xs font-medium dark:text-white/40">{username}</p> </div> </div> <blockquote className="mt-2 text-sm">{body}</blockquote> </figure> ); }; export function MarqueeDemo() { return ( <div className="relative flex h-[500px] w-full flex-col items-center justify-center overflow-hidden rounded-lg border bg-background md:shadow-xl"> <Marquee pauseOnHover className="[--duration:20s]"> {firstRow.map((review) => ( <ReviewCard key={review.username} {...review} /> ))} </Marquee> <Marquee reverse pauseOnHover className="[--duration:20s]"> {secondRow.map((review) => ( <ReviewCard key={review.username} {...review} /> ))} </Marquee> <div className="pointer-events-none absolute inset-y-0 left-0 w-1/3 bg-gradient-to-r from-white dark:from-background"></div> <div className="pointer-events-none absolute inset-y-0 right-0 w-1/3 bg-gradient-to-l from-white dark:from-background"></div> </div> ); }
Benefits of ShadCN CLI
The ShadCN CLI offers several benefits that enhance the development workflow:
Speed and Efficiency: Quickly add and configure components without manually copying files or code snippets.
Customizability: Easily integrate components from various sources, ensuring your project meets specific requirements.
Extensibility: Inject dependencies, pages, CSS, and more, creating a highly customizable development environment.
Community Reaction and Sentiment
The ShadCN component library has garnered significant attention and positive feedback from the developer community. Here are some notable reactions and sentiments:
Ease of Use: Many developers appreciate the straightforward setup and integration process.
Flexibility: The ability to customize components extensively has been highlighted as a major advantage.
Innovative CLI Tool: The CLI tool has received praise for its functionality and the flexibility it brings to the development workflow.
No External Dependencies: Developers value the fact that ShadCN components live directly in the codebase, eliminating version conflicts and update headaches.
Example Community Comments:
“ShadCN UI provides pre-built components that can be entirely customized to match your application's design system. This means you're not limited to a predefined style, as you would be with other component libraries.”
“Components live directly in your codebase, eliminating version conflicts and update headaches.”
“Easily adapt components to your existing design system without constraints.”
“ShadCN UI is the perfect React component library for anyone building frontend applications in 2024.”
Comparison with Other Libraries
ShadCN stands out from other traditional component libraries in several key ways:
No External Dependencies: Unlike traditional libraries that require installation as npm dependencies, ShadCN components are directly copied into the codebase, providing full control and eliminating version management issues.
Tailored Customization: ShadCN allows for extensive customization, enabling developers to adapt components to their existing design systems without constraints.
Seamless Integration: Works seamlessly with existing tools and familiar technologies like React and Tailwind CSS, offering a smooth development experience.
Future-Proof Development: ShadCN components evolve with the project, independent of third-party roadmaps, ensuring long-term maintainability.
Practical Applications and Use Cases
ShadCN is particularly well-suited for a variety of development scenarios. Here are some ideal use cases and examples of projects that benefit from using ShadCN:
Ideal For:
Junior to Mid-Level Developers: Perfect for developers looking to improve their React and component design skills. The library’s flexibility allows for learning and experimentation without the constraints of predefined styles.
Early-Stage Products: Ideal for MVPs, hackathon projects, or startups that need to iterate rapidly. ShadCN's easy integration and customization capabilities speed up development timelines.
Accessibility-Driven Applications: Designed to meet strict accessibility guidelines, making it a good choice for projects where accessibility is a priority.
Developers Frustrated with Opinionated Libraries: Enables developers to implement their own design systems without being locked into the opinions of a third-party library.
Real-World Examples
Dashboard Applications: ShadCN’s blocks and customizable components make it easy to create comprehensive and visually consistent dashboards.
Authentication Pages: Theming and example implementations simplify the creation of secure, user-friendly authentication systems.
Data Visualization Tools: Built-in chart components facilitate the development of interactive and informative data visualization applications.
E-commerce Sites: ShadCN's extensive component library helps in quickly building feature-rich e-commerce platforms with consistent design aesthetics.
Integration with Vercel’s v0
ShadCN integrates seamlessly with Vercel's AI-assisted development platform, v0.dev. This integration enhances the capabilities of v0.dev, streamlining the design process by combining AI-driven design with the flexibility of ShadCN components. This synergy allows developers to build and deploy sophisticated applications rapidly.
Conclusion
ShadCN is a powerful, flexible, and highly customizable component library that offers numerous benefits over traditional libraries. Its extensibility through the CLI tool, combined with community-driven development and extensive customization options, makes it an invaluable resource for modern web development. Whether you are building an MVP, enhancing the accessibility of your application, or developing complex data visualizations, ShadCN provides the tools and flexibility needed for success.