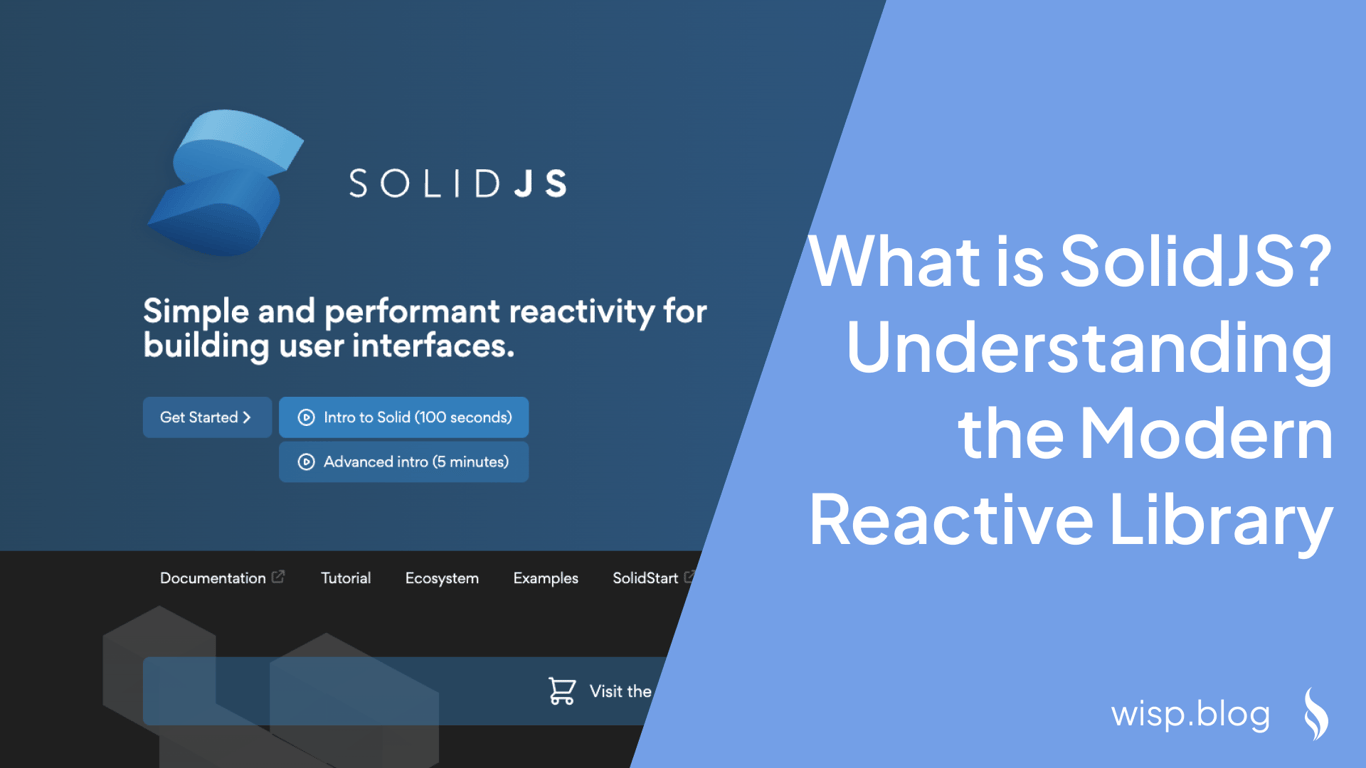
New libraries and frameworks emerge to offer innovative ways to build user interfaces. Among these, SolidJS stands out as a modern JavaScript library that promises high performance and a seamless developer experience. If you're familiar with React or Vue, you'll find SolidJS both familiar and refreshingly different. In this article, we'll explore what SolidJS is, its core features, how it works, and where it stands compared to other popular libraries.
What is SolidJS?
SolidJS is a declarative JavaScript library for building user interfaces, designed with a focus on performance and fine-grained reactivity. Developed by Ryan Carniato, SolidJS aims to combine the best aspects of reactive programming found in libraries like React and Vue while eliminating some of their inherent performance bottlenecks.
According to the official SolidJS website, the library distinguishes itself by prioritizing fine-grained reactivity and compile-time optimizations. Its core philosophy revolves around providing a highly efficient way to build complex, reactive interfaces without the overhead typically associated with virtual DOM diffing.
Brief History
SolidJS was initially conceived as an experiment to see how far a reactive system could be pushed in JavaScript. Over time, it evolved into a fully-fledged library that not only delivers on its performance promises but also offers an intuitive and enjoyable development experience.
Core Features of SolidJS
Fine-Grained Reactivity
One of SolidJS's standout features is its fine-grained reactivity system. Unlike libraries that rely on a virtual DOM to manage state changes, SolidJS updates the actual DOM directly and efficiently. This is achieved through reactive primitives such as createSignal
, which track dependencies and only update when necessary.
JSX Syntax
Just like React, SolidJS uses JSX (JavaScript XML) to render HTML. This makes it easy for developers who are already familiar with React to transition to SolidJS. The use of JSX allows for writing components in a way that is both declarative and expressive.
Compile-Time Optimization
SolidJS leverages compile-time optimizations to convert reactive code into highly efficient JavaScript. This means that a lot of the work typically done at runtime in other libraries is handled during the build process in SolidJS, resulting in smaller bundle sizes and faster execution.
No Virtual DOM
Unlike React, which relies heavily on a virtual DOM to manage changes, SolidJS updates the real DOM directly. This approach minimizes the performance overhead associated with diffing virtual DOM trees, making SolidJS exceptionally fast.
Component Architecture
Components in SolidJS are the building blocks of the UI, similar to other modern JavaScript libraries. They are simply functions that return JSX and can be composed to build complex interfaces. SolidJS components are highly efficient, rendering once and updating only as necessary.
Lifecycle Methods
SolidJS provides lifecycle methods such as onMount
and onCleanup
to manage component state and side effects. These methods offer fine-grained control over the component lifecycle, allowing developers to optimize performance and resource management.
Effects and Conditional Rendering
SolidJS makes managing reactive variables and conditional rendering straightforward with built-in components like Effect
and Show
. These components allow developers to reactively manage UI updates and define elements that should only be displayed under certain conditions.
How Does SolidJS Work?
SolidJS achieves its remarkable performance through a combination of fine-grained reactivity and compile-time optimizations. Understanding how SolidJS works involves delving into its core concepts and the innovative techniques it employs.
Fine-Grained Reactivity Explained
At the heart of SolidJS's performance lies its fine-grained reactivity system. This system consists of reactive primitives such as signals, computations, and memoizations, which together create a highly responsive user interface. Here's how they work:
Signals: These are the basic reactive primitive in SolidJS. Signals track values and notify observers of changes. You can create a signal using
createSignal
and update it when needed, triggering only the necessary updates in the DOM.import { createSignal } from 'solid-js'; const [count, setCount] = createSignal(0); function increment() { setCount(count() + 1); }
Computations: These are functions that run whenever the signals they depend on change. Computations are created using
createEffect
, and their primary role is to reactively update the UI or perform side effects based on signal changes.import { createEffect } from 'solid-js'; createEffect(() => { console.log('Count has changed to:', count()); });
Memoizations: These are used to optimize computed values. You can create a memoized value using
createMemo
, which recalculates only when its dependencies change.import { createMemo } from 'solid-js'; const doubleCount = createMemo(() => count() * 2);
Compilation and Optimization
SolidJS takes advantage of compile-time optimizations to convert reactive code into optimized JavaScript. This process eliminates much of the runtime overhead associated with virtual DOM diffing found in other libraries. Instead, SolidJS generates precise DOM manipulation instructions during the build process, resulting in smaller bundle sizes and faster execution.
Performance Characteristics
SolidJS is renowned for its exceptional performance, often outperforming other popular JavaScript libraries like React and Vue. Here are some of the techniques SolidJS uses to achieve this:
Granular Updates: By focusing on fine-grained reactivity, SolidJS minimizes the scope of DOM updates to only the necessary parts. This granular approach avoids the need for costly virtual DOM diffing.
Direct DOM Manipulation: SolidJS updates the actual DOM directly, bypassing the overhead of a virtual DOM. This direct approach results in faster rendering and more efficient updates.
Optimized Reactivity Management: SolidJS uses efficient data structures to manage reactivity, ensuring minimal memory allocations and optimal performance.
Cloning DOM Nodes: When dealing with larger templates, SolidJS can clone existing DOM nodes instead of creating new ones. This technique significantly boosts performance, especially with lists and tables.
No Virtual DOM: By avoiding the virtual DOM altogether, SolidJS eliminates the reconciliation costs associated with virtual DOM libraries.
Component Lifecycle Management: SolidJS handles component lifecycle using reactive computations, ensuring cleanups and avoiding memory leaks without additional abstractions.
For a detailed analysis of SolidJS's performance benchmarks, you can refer to Thinking Granular: How is SolidJS so Performant? by Ryan Carniato.
Comparison with React
SolidJS and React are often compared due to their similar goals of building efficient, declarative user interfaces. However, they differ significantly in their underlying mechanisms and performance characteristics. Let's take a closer look at how SolidJS stacks up against React.
Structure and Syntax
Both SolidJS and React use components as the building blocks of their UIs and employ JSX for rendering HTML. This makes transitioning between the two libraries relatively straightforward for developers. However, there are key differences in how these components are rendered and managed.
Reactivity Models and DOM Handling
React: React relies on a virtual DOM to manage state changes. When a component's state changes, React re-renders the entire component and updates the virtual DOM. React then compares the virtual DOM to the actual DOM (a process known as reconciliation) and applies the necessary updates. This process can be computationally expensive, especially for complex UIs with frequent state changes.
SolidJS: In contrast, SolidJS uses fine-grained reactivity to track dependencies and update only the necessary parts of the DOM. This approach eliminates the need for a virtual DOM and its associated overhead, resulting in more efficient updates and faster rendering.
State Management and Effects
React: In React, state is managed using hooks like
useState
and side effects are handled withuseEffect
. These hooks provide a powerful way to manage state and effects, but they also require developers to manually track dependencies and optimize performance.SolidJS: SolidJS introduces reactive primitives such as
createSignal
for state management andcreateEffect
for side effects. These primitives are designed to be more intuitive and efficient, automatically tracking dependencies and optimizing updates without additional effort from developers.
Performance
SolidJS's performance advantages stem from its fine-grained reactivity and direct DOM manipulation. By avoiding the virtual DOM and its reconciliation process, SolidJS can deliver faster updates and smaller bundle sizes. This makes SolidJS particularly well-suited for applications that require high performance and dynamic user interactions.
Advantages and Disadvantages
SolidJSAdvantages:
Avoids component re-rendering, resulting in better performance.
Direct manipulation of the DOM for efficient updates.
Smaller bundle sizes due to compile-time optimization.
Intuitive reactive primitives for managing state and effects.
Disadvantages:
Smaller community and ecosystem compared to React.
Learning curve for developers unfamiliar with reactive programming concepts.
Advantages:
Rich community and extensive ecosystem with numerous third-party libraries and tools.
Excellent documentation and widespread industry adoption.
Robust and flexible for a wide range of use cases.
Disadvantages:
Performance overhead due to the virtual DOM and reconciliation process.
Requires manual optimization for complex state management and effects.
For a detailed comparison of SolidJS and React, you can read the SolidJS vs. React: The Go-to Guide by Toptal.
Use Cases for SolidJS
SolidJS's unique combination of fine-grained reactivity and compile-time optimizations makes it an ideal choice for certain types of applications. Here are some scenarios where SolidJS shines:
High-Performance Web Applications
Due to its efficient DOM updates and minimal overhead, SolidJS is well-suited for high-performance web applications. Whether you're building a complex dashboard with real-time data updates or a highly interactive single-page application, SolidJS can handle the demands of dynamic and complex UIs with ease.
Applications with Complex UI State
SolidJS's fine-grained reactivity makes it particularly effective for applications with complex UI states and interactions. By efficiently managing and updating only the parts of the DOM that need to change, SolidJS ensures smooth and responsive user experiences even in the most demanding scenarios.
React Alternative
For developers familiar with React, SolidJS offers a compelling alternative that retains the declarative nature and component-based architecture of React while providing significant performance improvements. If you're looking to boost the performance of your existing React projects or explore new possibilities, SolidJS is worth considering.
Real-World Applications
SolidJS is already being used in various real-world applications, demonstrating its versatility and reliability. Here are a few examples:
Complex Dashboards: Applications that require real-time data visualization and updates can benefit from SolidJS's efficient reactivity and performance.
Interactive SPAs: Single-page applications with heavy user interactions and dynamic content updates can leverage SolidJS for a smoother user experience.
E-commerce Platforms: High-performance e-commerce platforms with complex state management needs can utilize SolidJS to enhance speed and responsiveness.
Content Management Systems (CMS): Integrate SolidJS with a CMS like Wisp to build high-performance content-driven websites with ease.
Code Examples
To give you a practical understanding of SolidJS, here are a few code examples demonstrating its core concepts and features.
Basic Component
Creating a basic component in SolidJS is straightforward. Here's an example of a simple HelloWorld
component:
import { render } from 'solid-js/web';
function HelloWorld() {
return <h1>Hello World</h1>;
}
render(() => <HelloWorld />, document.getElementById('app'));
Nesting Components
You can easily compose components in SolidJS by nesting them within each other. Here’s an example:
function Header() {
return <h1>Header</h1>;
}
function App() {
return (
<>
<Header />
<h1>Hello World</h1>
</>
);
}
render(() => <App />, document.getElementById('app'));
Reactivity Example
SolidJS shines with its reactivity system. Here's an example showcasing reactive state management using createSignal
:
import { render } from 'solid-js/web';
import { createSignal } from 'solid-js';
function DisplayName() {
const [name, setName] = createSignal('fuzzysid');
const changeName = () => setName('siddhant');
return (
<>
<h1>{name()}</h1>
<button onClick={changeName}>Change Name</button>
</>
);
}
render(() => <DisplayName />, document.getElementById('app'));
Getting Started with SolidJS
If you're excited about the potential of SolidJS and want to start building with it, here's a quick guide to get you up and running.
Installation and Setup
To get started with SolidJS, you can use the official SolidJS template or set up a new project manually. The easiest way to create a new SolidJS project is by using the SolidJS CLI:
npx degit solidjs/templates/js my-solid-app
cd my-solid-app
npm install
npm start
Basic Tutorial
The official SolidJS tutorial provides a comprehensive introduction to the library's core features and concepts. Here’s a brief overview of what you'll learn:
Creating Your First Component: Learn how to set up a basic SolidJS component and render it in the DOM.
State Management: Understand how to use
createSignal
to manage state reactively.Effects and Computations: Discover how to handle side effects and computations using
createEffect
andcreateMemo
.Conditional Rendering: Learn how to conditionally render components using
Show
and other built-in helpers.Lifecycle Methods: Explore lifecycle methods such as
onMount
andonCleanup
to manage component state and effects effectively.
Conclusion
SolidJS presents a compelling option for developers looking to build high-performance web applications with efficient reactivity. Its fine-grained reactivity and compile-time optimizations set it apart from other libraries like React, offering significant performance gains and a smoother development experience.
By understanding the core concepts of SolidJS and exploring its features through practical examples, you can harness the power of this innovative library in your projects. Whether you're building complex dashboards, interactive SPAs, or e-commerce platforms, SolidJS provides the tools you need to create responsive and performant user interfaces.
For developers looking to integrate SolidJS with a robust CMS, consider using Wisp. With Wisp, you can easily manage your content and build high-performance websites that leverage the power of SolidJS.
Ready to dive into SolidJS? Start with the official SolidJS tutorial and begin your journey towards building fast, reactive web applications today.