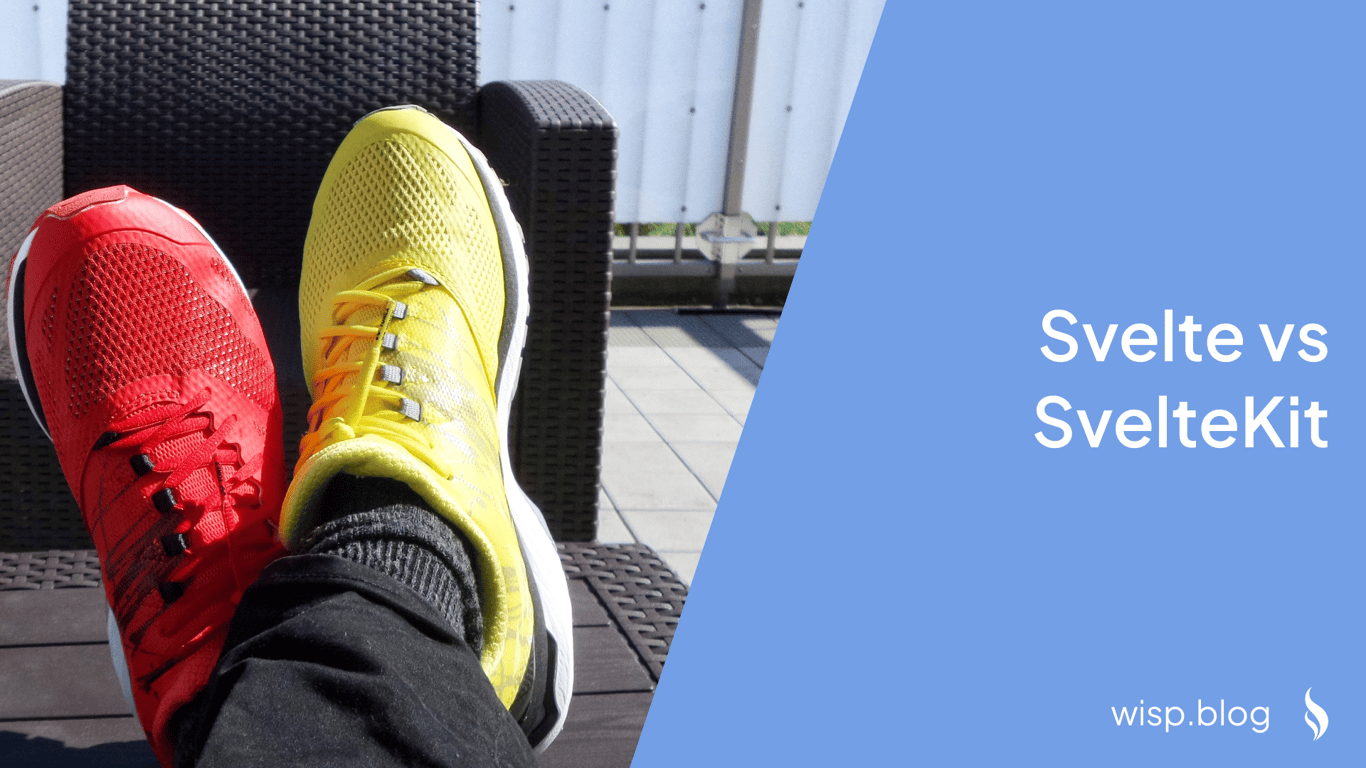
You've heard about Svelte's growing popularity in the frontend development world, and now there's SvelteKit too. But what exactly is SvelteKit, and when should you choose it over plain Svelte? If you're wrestling with these questions, you're not alone. Many developers express confusion about the distinction, with common questions like "I don't understand what SvelteKit is?" and "I don't see any advantages of SvelteKit other than the built-in router."
Let's clear up this confusion and help you make an informed decision for your next project.
Understanding Svelte: The Foundation
At its core, Svelte is a revolutionary UI framework that takes a unique approach to building web applications. Unlike traditional frameworks that do much of their work in the browser, Svelte shifts that work to the compile step. This means when you build your application, Svelte transforms your code into highly optimized JavaScript that updates the DOM directly.
Think of Svelte as a powerful but lightweight tool that:
Compiles your components into efficient, framework-free vanilla JavaScript
Provides reactive state management without complex state management libraries
Offers a clean, intuitive syntax that feels like writing enhanced HTML and JavaScript
Results in smaller bundle sizes compared to other frameworks
For instance, a simple Svelte component might look like this:
<script>
let count = 0;
function increment() {
count += 1;
}
</script>
<button on:click={increment}>
Clicks: {count}
</button>
This straightforward approach has made Svelte increasingly popular, especially for smaller applications or when you need to embed interactive components into existing websites. According to the State of JavaScript 2023 report, Svelte has consistently ranked high in developer satisfaction, particularly for its simplicity and performance.
Enter SvelteKit: The Full-Stack Framework
While Svelte excels at building user interfaces, modern web applications often need more than just UI components. They require routing, server-side rendering, API endpoints, and more. This is where SvelteKit comes in.
SvelteKit is a full-featured application framework built on top of Svelte. It's to Svelte what Next.js is to React or Nuxt is to Vue. As one developer puts it, "If you need to use a server, then I use SvelteKit. If I'm creating a front-end only app calling APIs to other systems, I use Svelte."
What SvelteKit Brings to the Table
File-Based Routing
Automatically generates routes based on your file structure
Handles both static and dynamic routes
Supports layout files for shared UI elements
Server-Side Rendering (SSR)
Improves initial page load performance
Enhances SEO capabilities
Provides better user experience for content-heavy sites
API Routes
Create backend endpoints within your frontend project
Handle form submissions and data processing
Manage authentication and authorization
Advanced Data Loading
Load data on the server before rendering pages
Handle loading states elegantly
Manage errors consistently
Here's a simple example of how SvelteKit handles routing and data loading:
// src/routes/posts/[id]/+page.server.js
export async function load({ params }) {
const post = await fetchPost(params.id);
return {
post
};
}
// src/routes/posts/[id]/+page.svelte
<script>
export let data;
const { post } = data;
</script>
<h1>{post.title}</h1>
<div>{post.content}</div>
Making the Right Choice: Svelte vs SvelteKit
The decision between Svelte and SvelteKit isn't about which one is better - it's about choosing the right tool for your specific needs. Let's break down when to use each:
Choose Svelte When:
Building Standalone Components
Creating widgets or interactive elements for existing websites
Developing component libraries
Prototyping UI features
Working with Existing Backends
Integrating with established backend services
Adding interactive features to traditional server-rendered applications
Building frontend-only applications that consume external APIs
Prioritizing Simplicity
Learning the basics of component-based development
Creating small to medium-sized applications
Needing maximum flexibility in project structure
Choose SvelteKit When:
Building Full-Stack Applications
Creating complete web applications from scratch
Needing both frontend and backend functionality
Implementing server-side rendering for SEO
Requiring Advanced Routing
Managing complex navigation structures
Handling dynamic routes
Implementing nested layouts
Dealing with Data Loading
Fetching data on the server side to avoid CORS issues
Implementing progressive enhancement
Managing loading and error states consistently
Common Concerns and Solutions
CORS IssuesMany developers report struggling with CORS when making fetch requests from the client. SvelteKit's solution is elegant:
// Instead of client-side fetching: const response = await fetch('api/data'); // CORS error! // Use SvelteKit's load function: export async function load({ fetch }) { const response = await fetch('api/data'); // Works perfectly! return { data: await response.json() }; }
Directory StructureWhile some developers find SvelteKit's enforced directory structure restrictive, it promotes consistency and best practices in larger projects. The structure becomes more intuitive as you work with it:
src/ ├── routes/ │ ├── +layout.svelte │ ├── +page.svelte │ └── about/ │ └── +page.svelte
Best Practices and Tips
For Svelte Projects
Choose the Right RouterIf you're using plain Svelte and need routing, consider established options like:
Tinro for simple routing needs
Svelte Routing for more complex requirements
State Management
Use Svelte's built-in stores for simple state management
Consider Svelte State Management patterns for larger applications
For SvelteKit Projects
Optimize Performance
Utilize SvelteKit's preloading feature for faster page transitions
Implement proper caching strategies
Use static site generation where appropriate
Security Considerations
Implement proper CSRF protection
Use environment variables for sensitive data
Leverage SvelteKit's built-in security features
Conclusion
Both Svelte and SvelteKit are powerful tools in the modern web development ecosystem. Svelte shines in its simplicity and performance for UI components, while SvelteKit provides a comprehensive framework for building full-featured web applications.
As one developer wisely noted, "If you're aiming for a front-end only application, sticking with Svelte may be the right choice." However, if you're building a complete web application that needs server-side rendering, routing, and API endpoints, SvelteKit's additional features will save you significant development time and effort.
Remember, you can always start with Svelte and migrate to SvelteKit later if your project's needs grow. The important thing is to choose the tool that best fits your current requirements while keeping in mind your application's future scalability needs.
Whether you choose Svelte or SvelteKit, you're getting a modern, efficient framework that prioritizes developer experience without sacrificing performance. The key is understanding your project's requirements and choosing the tool that aligns with your specific needs.