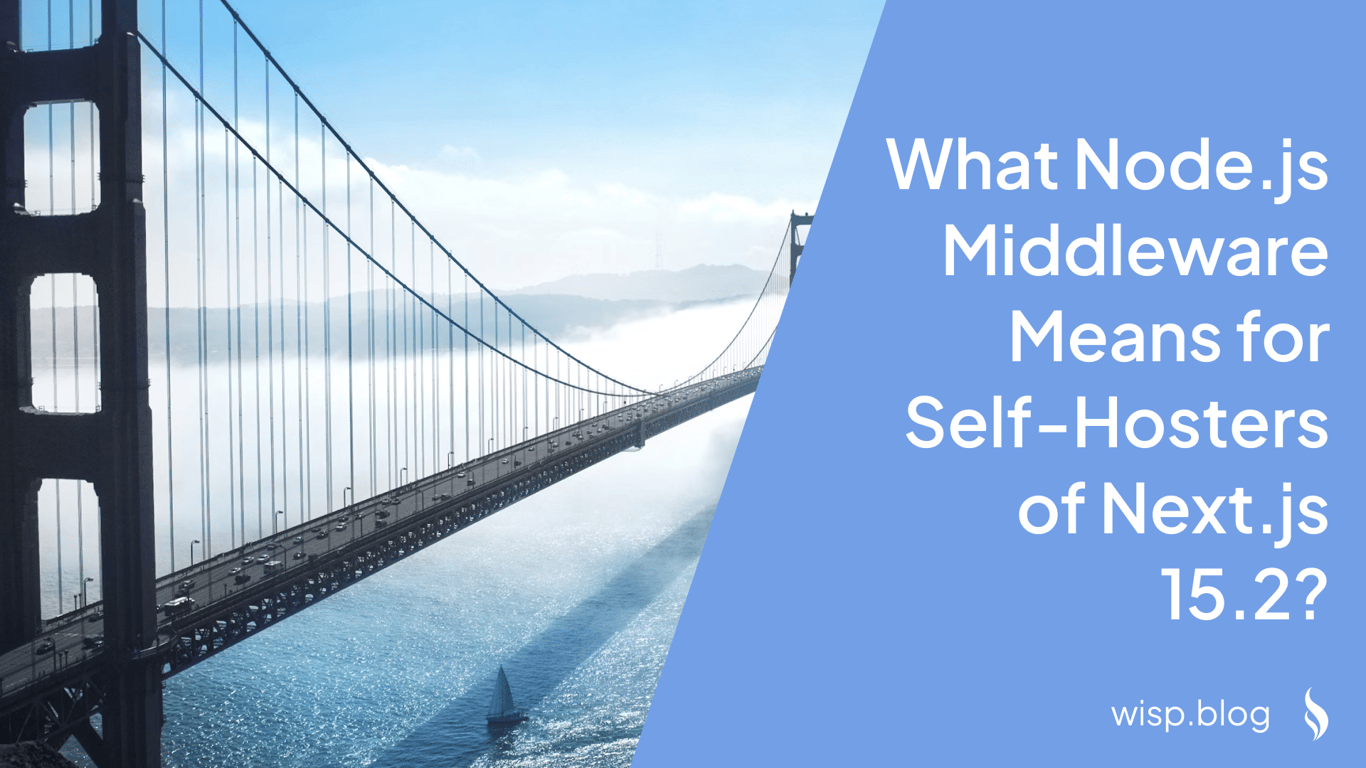
If you've been self-hosting Next.js applications, you're likely familiar with the challenges of managing middleware in your deployment setup. With the release of Next.js 15.2, a significant change has arrived that's causing quite a stir in the development community - the introduction of Node.js middleware support.
You might have already experienced the limitations of edge middleware or found yourself wondering "When should we use Node middleware vs edge?" This confusion is not uncommon, as evidenced by numerous discussions in the Next.js community. The good news is that this update brings powerful new capabilities that could transform how you handle server-side logic in your self-hosted applications.
The transition from edge middleware to Node.js middleware represents more than just a technical change - it's a fundamental shift in how we can approach request handling, authentication, and server-side operations in Next.js applications. For self-hosters, this means greater control and flexibility in implementing custom server-side logic, especially when dealing with complex authentication flows or when you need to leverage Node.js-specific libraries.
Why Node.js Middleware Matters Now
If you've been struggling with implementing certain server-side features or found yourself limited by edge middleware's capabilities, Node.js middleware opens up new possibilities. Here's why this matters:
Full Access to Node.js APIs: You can now use any Node.js library directly in your middleware. Need to use
crypto
for enhanced security or@opentelemetry/instrumentation-pino
for logging? Now you can.Improved Authentication Flows: Gone are the days of complex workarounds for handling authentication. With Node.js middleware, you can now automatically manage authorization tokens and handle 401 errors more elegantly.
Better Database Integration: Since middleware now runs closer to your database, you can optimize request handling and reduce latency - a crucial factor for self-hosted applications.
Enhanced Development Experience: The new middleware implementation comes with improved debugging capabilities, making it easier to troubleshoot issues in your self-hosted environment.
The Shift from Edge to Node.js Middleware
One of the most significant changes in Next.js 15.2 is the move away from edge middleware. While this might seem daunting at first, especially if you've built your application around edge middleware capabilities, the transition brings several advantages:
More predictable behavior in self-hosted environments
Better compatibility with existing Node.js libraries
Improved debugging capabilities
Enhanced control over request/response handling
This shift addresses many of the pain points that developers have expressed in the community, particularly around authentication handling and middleware flexibility. As one developer noted in a recent discussion, "No more edge middleware is huge" - and they're right about the impact this change brings to self-hosted Next.js applications.
Getting Started with Node.js Middleware
Let's dive into how you can implement Node.js middleware in your self-hosted Next.js 15.2 application. The process is straightforward, but there are some important considerations to keep in mind.
Basic Setup
First, you'll need to upgrade your Next.js installation to the latest version and enable the experimental feature:
npm install next@canary
Then, update your next.config.js
:
const nextConfig = {
experimental: { nodeMiddleware: true },
};
export default nextConfig;
Creating Your First Node.js Middleware
Here's a basic example of how to implement Node.js middleware:
import { NextResponse } from 'next/server';
import bcrypt from 'bcrypt'; // Now you can use Node.js libraries directly!
export function middleware(request) {
// Example: API key validation with bcrypt
const apiKey = request.headers.get('x-api-key');
if (!apiKey) {
return NextResponse.redirect(new URL('/signin', request.url));
}
// You can now use Node.js specific features here
return NextResponse.next();
}
export const config = {
runtime: 'nodejs',
matcher: '/api/:path*'
};
Advanced Implementation Patterns
For more complex scenarios, you might want to implement middleware that handles multiple concerns:
import { NextResponse } from 'next/server';
import pino from 'pino'; // Logging library
import { verify } from 'jsonwebtoken'; // JWT verification
const logger = pino();
export async function middleware(request) {
// Logging
logger.info({
url: request.url,
method: request.method,
timestamp: new Date().toISOString()
});
// Authentication
const token = request.headers.get('authorization')?.split(' ')[1];
if (token) {
try {
const decoded = verify(token, process.env.JWT_SECRET);
// Attach user info to request
request.user = decoded;
} catch (error) {
return NextResponse.json(
{ error: 'Invalid token' },
{ status: 401 }
);
}
}
return NextResponse.next();
}
Common Use Cases and Patterns
When implementing Node.js middleware in your self-hosted Next.js application, several patterns have emerged as particularly useful:
Authentication and Authorization:
export async function middleware(request) {
const session = await getSession(request);
if (!session && !request.nextUrl.pathname.startsWith('/auth')) {
return NextResponse.redirect(new URL('/auth/login', request.url));
}
return NextResponse.next();
}
Request Logging and Monitoring:
import { createLogger } from 'winston';
const logger = createLogger(/* your config */);
export async function middleware(request) {
const startTime = Date.now();
const response = NextResponse.next();
logger.info({
path: request.nextUrl.pathname,
duration: Date.now() - startTime,
status: response.status
});
return response;
}
Best Practices for Self-Hosted Deployments
When implementing Node.js middleware in your self-hosted Next.js application, following these best practices will help ensure optimal performance and security:
1. Performance Optimization
import { NextResponse } from 'next/server';
import { cache } from 'react';
const getAuthStatus = cache(async (token) => {
// Your authentication logic here
return status;
});
export async function middleware(request) {
const token = request.headers.get('authorization');
const status = await getAuthStatus(token);
// Rest of your middleware logic
}
2. Error Handling
Implement robust error handling to ensure your middleware gracefully handles various scenarios:
export async function middleware(request) {
try {
// Your middleware logic
return NextResponse.next();
} catch (error) {
console.error('Middleware error:', error);
// Handle different types of errors
if (error.code === 'AUTH_REQUIRED') {
return NextResponse.redirect(new URL('/login', request.url));
}
return NextResponse.json(
{ error: 'Internal Server Error' },
{ status: 500 }
);
}
}
3. Security Considerations
When implementing security measures in your middleware:
import { rateLimit } from 'express-rate-limit';
import { NextResponse } from 'next/server';
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100 // limit each IP to 100 requests per windowMs
});
export async function middleware(request) {
// Implement rate limiting
const limitResult = await limiter(request);
if (limitResult.error) {
return NextResponse.json(
{ error: 'Too many requests' },
{ status: 429 }
);
}
// CORS headers
const response = NextResponse.next();
response.headers.set('Access-Control-Allow-Origin', '*');
response.headers.set('Access-Control-Allow-Methods', 'GET, POST, PUT, DELETE, OPTIONS');
response.headers.set('Access-Control-Allow-Headers', 'Content-Type, Authorization');
return response;
}
4. Monitoring and Logging
Implement comprehensive logging to track middleware performance and issues:
import { NextResponse } from 'next/server';
import pino from 'pino';
const logger = pino({
level: process.env.LOG_LEVEL || 'info',
transport: {
target: 'pino-pretty'
}
});
export async function middleware(request) {
const startTime = performance.now();
try {
const response = await NextResponse.next();
logger.info({
path: request.nextUrl.pathname,
method: request.method,
duration: `${(performance.now() - startTime).toFixed(2)}ms`,
status: response.status
});
return response;
} catch (error) {
logger.error({
path: request.nextUrl.pathname,
method: request.method,
error: error.message,
stack: error.stack
});
throw error;
}
}
Common Challenges and Solutions
When working with Node.js middleware in Next.js 15.2, you might encounter several challenges. Here's how to address them:
1. Middleware and Server Actions Interaction
One common source of confusion is how middleware interacts with server actions. As noted in community discussions:
// Important: Middleware doesn't directly affect server actions
export async function middleware(request) {
// The next-action header determines which server action is called
const nextAction = request.headers.get('next-action');
if (nextAction) {
// Log server action calls
console.log(`Server action called: ${nextAction}`);
}
return NextResponse.next();
}
2. Route-Level Middleware Implementation
While Next.js doesn't support route-level middleware directly like Express.js, you can achieve similar functionality:
export function middleware(request) {
const { pathname } = request.nextUrl;
// Apply different middleware logic based on routes
if (pathname.startsWith('/api')) {
return handleApiMiddleware(request);
}
if (pathname.startsWith('/admin')) {
return handleAdminMiddleware(request);
}
return NextResponse.next();
}
async function handleApiMiddleware(request) {
// API-specific middleware logic
}
async function handleAdminMiddleware(request) {
// Admin-specific middleware logic
}
3. Handling Authentication State
Managing authentication state effectively in middleware:
import { NextResponse } from 'next/server';
import { verify } from 'jsonwebtoken';
export async function middleware(request) {
const token = request.cookies.get('auth-token');
try {
if (token) {
const decoded = verify(token.value, process.env.JWT_SECRET);
// Attach user info to headers for route handlers
const response = NextResponse.next();
response.headers.set('x-user-id', decoded.userId);
return response;
}
} catch (error) {
// Handle invalid tokens
request.cookies.delete('auth-token');
return NextResponse.redirect(new URL('/login', request.url));
}
return NextResponse.next();
}
4. Performance Optimization
When dealing with performance concerns:
import { NextResponse } from 'next/server';
import { cache } from 'react';
// Cache expensive operations
const getCachedData = cache(async (key) => {
// Your expensive operation here
return data;
});
export async function middleware(request) {
// Use caching for expensive operations
const data = await getCachedData('your-key');
const response = NextResponse.next();
response.headers.set('x-cached-data', JSON.stringify(data));
return response;
}
Future Considerations and Community Feedback
The introduction of Node.js middleware in Next.js 15.2 is just the beginning. Based on community feedback and discussions, here are some key areas to watch and consider for your self-hosted applications:
Emerging Patterns and Features
The Next.js community has been vocal about desired features and improvements:
Route-Level Middleware
Current workarounds using matchers and conditional logic
Potential future implementation similar to layout files
Community requests for more granular control
Enhanced Debugging Tools
Improved error views in development
Better integration with Node.js debugging tools
More detailed logging and monitoring capabilities
Authentication Patterns
Standardized approaches for token handling
Integration with popular authentication providers
Better session management solutions
Planning for the Future
When implementing Node.js middleware in your self-hosted Next.js application, consider:
Scalability
// Example of scalable middleware architecture
export function middleware(request) {
const middlewareChain = [
rateLimiting,
authentication,
logging,
// Easy to add new middleware functions
];
return executeMiddlewareChain(middlewareChain, request);
}
async function executeMiddlewareChain(chain, request) {
let response = null;
for (const middleware of chain) {
response = await middleware(request);
if (response) break;
}
return response || NextResponse.next();
}
Maintainability
// Separate middleware concerns
import { authMiddleware } from './middleware/auth';
import { loggingMiddleware } from './middleware/logging';
import { securityMiddleware } from './middleware/security';
export function middleware(request) {
// Easy to maintain and update individual middleware components
return compose([
authMiddleware,
loggingMiddleware,
securityMiddleware
])(request);
}
Conclusion
The introduction of Node.js middleware in Next.js 15.2 represents a significant step forward for self-hosted applications. While there are still areas for improvement, the current implementation provides powerful capabilities for handling complex server-side logic.
Key takeaways:
Node.js middleware offers greater flexibility and access to Node.js APIs
Proper implementation can significantly improve application security and performance
The community continues to shape the future of middleware functionality
Self-hosters have more control over their application's behavior
As you implement Node.js middleware in your self-hosted Next.js applications, remember to:
Follow best practices for security and performance
Stay updated with community feedback and feature releases
Plan for scalability and maintainability
Leverage the full power of Node.js libraries when needed
The future of Next.js middleware looks promising, with ongoing developments and improvements driven by community needs and feedback. Keep an eye on the Next.js blog and GitHub discussions for updates and new features as they become available.