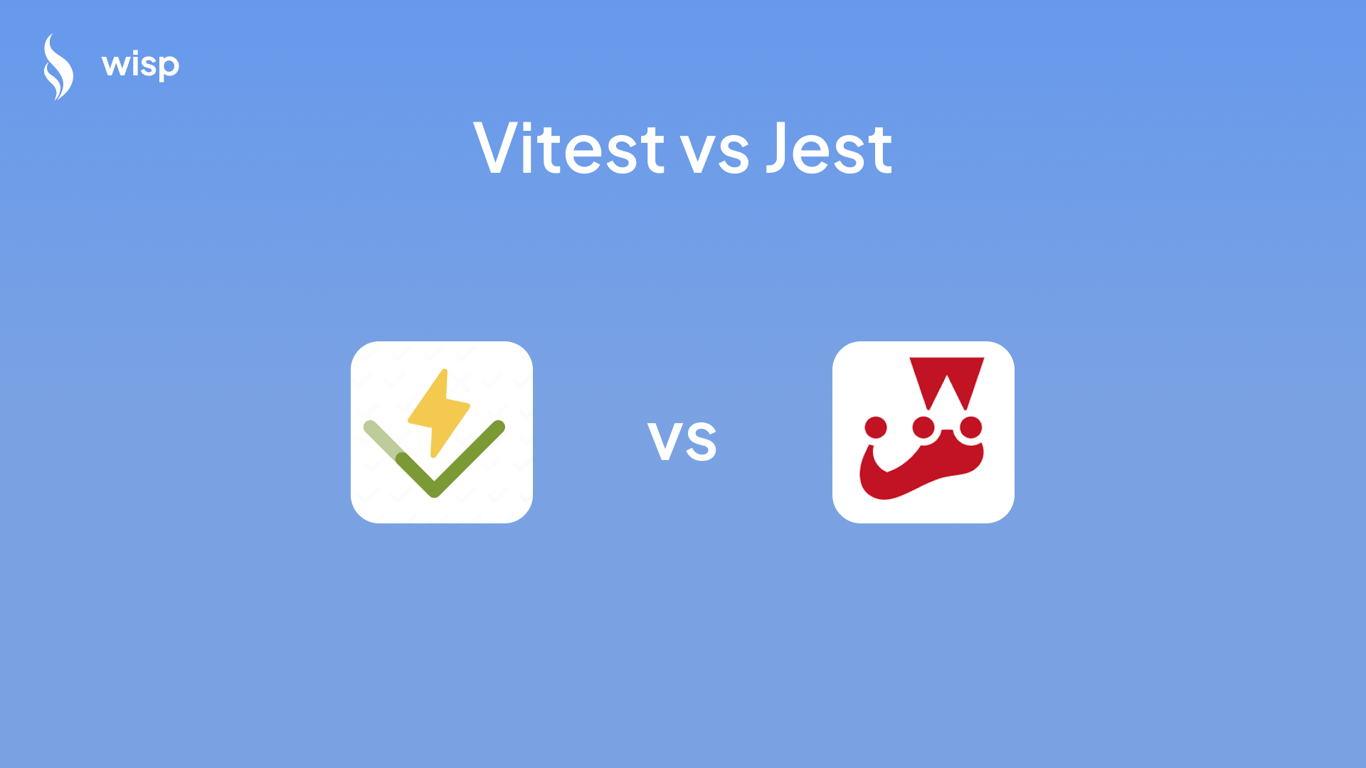
You're building a Next.js application and hit that crucial moment - choosing a testing framework. As you research, two names keep popping up: Jest and Vitest. While Jest has been the go-to testing framework for years, Vitest is the new challenger that's been gaining significant traction.
"In my experience, Vitest is faster and simpler," notes one developer in a recent Reddit discussion. Yet others maintain that "Jest has better ecosystem and support." This divide in the developer community has left many wondering which framework is truly the best choice for their Next.js projects.
The decision isn't always straightforward. While Vitest boasts impressive performance metrics, Jest's maturity and extensive ecosystem make it a compelling choice, especially for teams already familiar with its workflow. Let's dive deep into both frameworks to help you make an informed decision.
Understanding Jest: The Established Champion
Jest, developed by Meta (formerly Facebook), has been the default choice for testing JavaScript applications for years. It's particularly well-integrated with the React ecosystem, making it a natural fit for Next.js applications.
Key Strengths of Jest:
Extensive Ecosystem
Vast collection of plugins and extensions
Rich documentation and community resources
Battle-tested in production environments
Built-in Features
Zero configuration required for most projects
Snapshot testing capabilities
Integrated code coverage reporting
Powerful mocking system
Community Support
Large, active community
Abundant learning resources
Quick issue resolution
Understanding Vitest: The Modern Challenger
Vitest emerged as a testing framework built specifically for Vite, bringing modern testing capabilities and impressive performance improvements. It's designed to be compatible with Jest's API while offering native ES module support and faster execution times.
Key Strengths of Vitest:
Performance
Significantly faster test execution
Native ES module support
Watch mode with instant feedback
Modern Architecture
Built on top of Vite
Better handling of modern JavaScript features
Simpler configuration process
Developer Experience
Improved error messages
Smart test file detection
Compatible with Jest's API
Real-World Performance Comparison
One of the most significant factors in choosing between Jest and Vitest is performance. According to user experiences and benchmarks:
Jest Performance
// Example Jest test execution time
Test Suites: 25 passed, 25 total
Tests: 100 passed, 100 total
Time: 15.5s
Vitest Performance
// Example Vitest test execution time
Test Suites: 25 passed, 25 total
Tests: 100 passed, 100 total
Time: 3.8s
As one developer noted, "The transition from Jest to Vitest is almost trivial... And it just runs way faster." This sentiment is echoed across multiple developer discussions.
Setting Up Testing in Your Next.js Project
Getting Started with Jest
Installation
npm install --save-dev jest @testing-library/react @testing-library/jest-dom
Configuration (jest.config.js)
module.exports = {
testEnvironment: 'jsdom',
setupFilesAfterEnv: ['<rootDir>/jest.setup.js'],
moduleNameMapper: {
'^@/components/(.*)$': '<rootDir>/components/$1',
},
testMatch: ['**/*.test.js', '**/*.test.tsx'],
};
Writing Your First Test
// components/Button.test.js
import { render, screen } from '@testing-library/react';
import Button from './Button';
describe('Button Component', () => {
test('renders button with text', () => {
render(<Button>Click me</Button>);
expect(screen.getByText('Click me')).toBeInTheDocument();
});
});
Getting Started with Vitest
Installation
npm install --save-dev vitest @testing-library/react @testing-library/jest-dom
Configuration (vite.config.js)
import { defineConfig } from 'vite';
export default defineConfig({
test: {
environment: 'jsdom',
globals: true,
setupFiles: './vitest.setup.js',
},
});
Writing Your First Test
// components/Button.test.js
import { render, screen } from '@testing-library/react';
import Button from './Button';
describe('Button Component', () => {
it('renders button with text', () => {
render(<Button>Click me</Button>);
expect(screen.getByText('Click me')).toBeInTheDocument();
});
});
Common Pain Points and Solutions
Jest Pain Points
TypeScript Integration Challenges
"I still have nightmares installing Jest in some TypeScript projects," shares one developer.
Solution: Use
ts-jest
and proper configuration:// jest.config.js module.exports = { preset: 'ts-jest', testEnvironment: 'jsdom', };
Slow Test Execution
Large test suites can become slow
Solution: Implement parallel test execution:
{ "scripts": { "test": "jest --maxWorkers=50%" } }
Vitest Pain Points
Command Line Issues
"If I run 'npm run vitest' it doesn't find any tests but if I run 'npx vitest' it all works," reports a user.
Solution: Ensure proper script configuration:
{ "scripts": { "test": "vitest", "test:watch": "vitest watch" } }
Intermittent Test Failures
Some users report unexplained test failures that resolve upon restart
Solution: Implement proper cleanup and isolation:
import { afterEach } from 'vitest'; import { cleanup } from '@testing-library/react'; afterEach(() => { cleanup(); });
Making the Decision: Comparative Analysis
Choose Jest If:
You Value Stability and Ecosystem
Your team is already familiar with Jest
You need extensive plugin support
You require comprehensive documentation
As one developer puts it, "We opted for Jest because our team is familiar with it. Plus, 300 tests only takes 15 seconds to run (in parallel). Along with extensive libraries/plugins, Jest seems to be the best option for us."
You Need Detailed Error Reporting
Jest provides comprehensive error messages
Better DOM structure visualization in test failures
More mature debugging tools
You're Working on a Large, Established Project
Proven reliability in large codebases
Extensive community support for troubleshooting
Well-established best practices
Choose Vitest If:
Performance is a Priority
You need faster test execution
You're working with modern JavaScript features
You want quick feedback during development
You're Starting a New Project
No legacy testing setup to maintain
Freedom to adopt modern testing practices
Ability to leverage latest features
You're Using Vite
Natural integration with Vite-based projects
Consistent development experience
Better handling of ES modules
Best Practices for Either Framework
Regardless of which testing framework you choose, following these best practices will help ensure successful test implementation:
Organize Tests Effectively
// Good organization
describe('UserProfile Component', () => {
describe('when user is logged in', () => {
it('displays user information', () => {
// Test implementation
});
it('shows logout button', () => {
// Test implementation
});
});
describe('when user is logged out', () => {
it('shows login prompt', () => {
// Test implementation
});
});
});
Use Proper Test Isolation
// Before each test
beforeEach(() => {
// Reset any shared state
jest.clearAllMocks(); // or vi.clearAllMocks() for Vitest
});
// After each test
afterEach(() => {
cleanup();
});
Implement Effective Mocking
// Jest example
jest.mock('axios', () => ({
get: jest.fn(() => Promise.resolve({ data: {} }))
}));
// Vitest equivalent
vi.mock('axios', () => ({
get: vi.fn(() => Promise.resolve({ data: {} }))
}));
Write Maintainable Tests
// Bad: Brittle test
test('renders correctly', () => {
const { container } = render(<Component />);
expect(container.innerHTML).toBe('<div>specific html</div>');
});
// Good: Resilient test
test('renders correctly', () => {
render(<Component />);
expect(screen.getByRole('button')).toBeInTheDocument();
expect(screen.getByText('Submit')).toBeVisible();
});
Migration Strategies
If you're considering switching from Jest to Vitest, here's a practical migration approach:
Gradual Migration
// Step 1: Add Vitest alongside Jest
{
"scripts": {
"test:jest": "jest",
"test:vitest": "vitest",
"test": "npm run test:jest && npm run test:vitest"
}
}
Update Test Files
// Before (Jest)
const sum = require('./sum');
test('adds numbers', () => {
expect(sum(1, 2)).toBe(3);
});
// After (Vitest)
import { expect, test } from 'vitest';
import sum from './sum';
test('adds numbers', () => {
expect(sum(1, 2)).toBe(3);
});
Update Configuration
// vite.config.js
import { defineConfig } from 'vite';
export default defineConfig({
test: {
globals: true,
environment: 'jsdom',
setupFiles: './src/test/setup.ts',
coverage: {
reporter: ['text', 'json', 'html'],
},
},
});
Conclusion
Both Jest and Vitest are excellent choices for testing Next.js applications, each with its own strengths. The decision ultimately comes down to your specific needs:
If you're starting a new project and value performance, Vitest is an excellent choice. Its modern architecture and impressive speed make it particularly attractive for greenfield projects.
If you have an established codebase or team familiarity with Jest, sticking with Jest might be the more pragmatic choice. Its mature ecosystem and extensive community support provide a solid foundation for large-scale applications.
Remember, as one developer wisely noted, "The transition from Jest to Vitest is almost trivial." This means you can start with either framework and switch later if needed, though it's always better to make an informed decision upfront to avoid unnecessary migrations.
Whichever framework you choose, focus on writing clean, maintainable tests that provide value to your project. The testing framework is just a tool - it's how you use it that matters most.