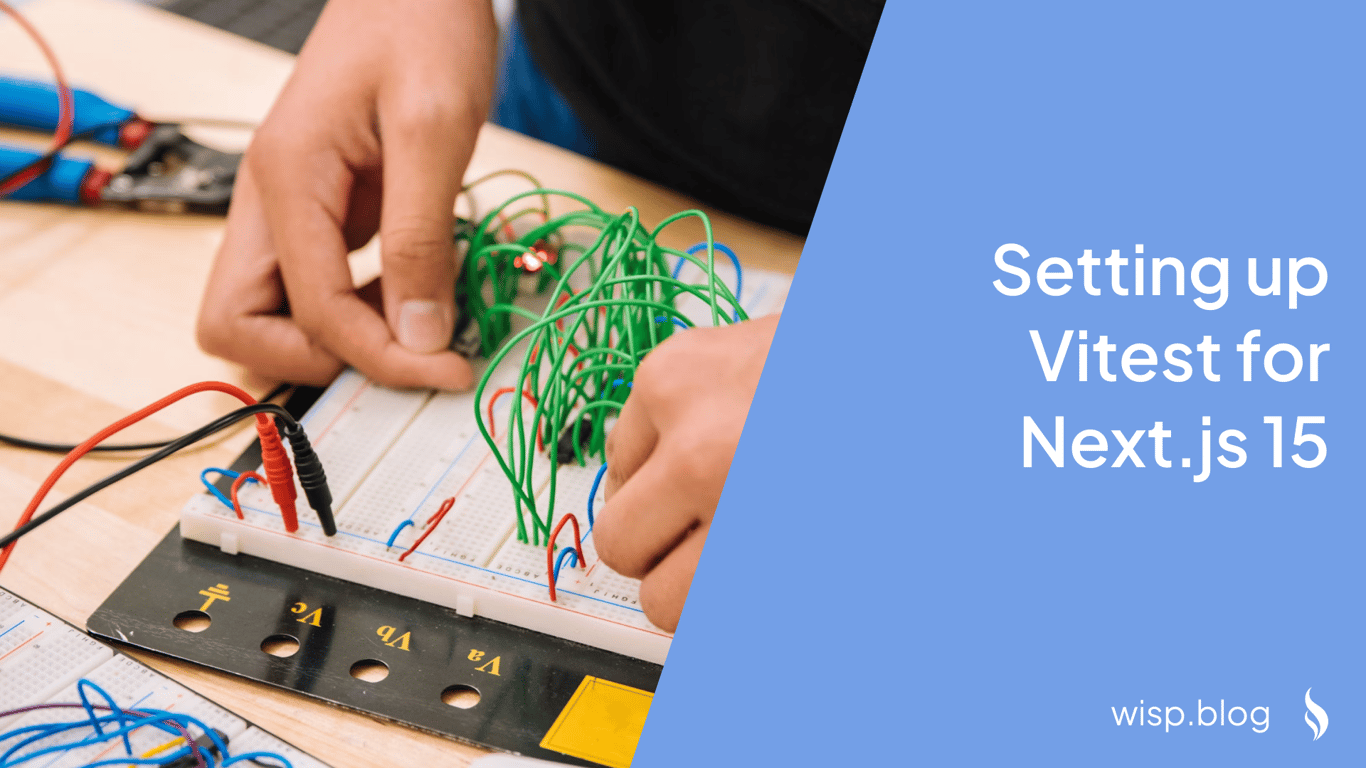
You've just upgraded to Next.js 15 and want to implement unit testing in your project. After some research, you discover Vitest - a modern testing framework that promises better performance and simpler setup. But as you dig deeper, the questions start piling up: Do you need Jest? What about React Testing Library? Why are there so many dependencies to consider?
If this sounds familiar, you're not alone. Many developers struggle with setting up testing frameworks, especially when transitioning from Jest to Vitest. The good news is that configuring Vitest for Next.js 15 is more straightforward than you might think.
What is Vitest and Why Choose It?
Vitest is a Vite-native test runner designed to provide a faster and more streamlined testing experience. While Jest has been the go-to testing framework for years, Vitest offers several compelling advantages:
Native Vite Integration: Leverages Vite's configuration and transforms for consistent behavior
Jest Compatibility: Supports most Jest APIs, making migration relatively painless
Lightning-Fast Performance: Utilizes Vite's dev server for improved test execution speed
Built-in TypeScript Support: No additional setup needed for TypeScript projects
As one developer noted on Reddit: "I've been having far fewer troubles with Vitest than with Jest." This sentiment is increasingly common among developers who have made the switch.
Prerequisites
Before we dive into the setup, ensure you have:
Node.js version 18 or higher installed
A Next.js 15 project ready for testing
Basic familiarity with React and Next.js
Quick Start Method
If you're starting a new project, the fastest way to get up and running is using the official Next.js template with Vitest:
npx create-next-app@latest --example with-vitest my-app-with-vitest
This command creates a new Next.js project with Vitest already configured, saving you the hassle of manual setup. However, if you're adding Vitest to an existing project, don't worry - we'll cover the manual setup process in detail next.
Manual Setup Steps
Let's break down the manual setup process into clear, manageable steps:
1. Install Required Dependencies
First, install Vitest and its required dependencies. One common source of confusion is knowing exactly which packages you need. Here's the complete list:
npm install -D vitest @vitejs/plugin-react jsdom @testing-library/react @testing-library/dom vite-tsconfig-paths
Each package serves a specific purpose:
vitest
: The core testing framework@vitejs/plugin-react
: Enables React support in Vitestjsdom
: Provides a browser-like environment for tests@testing-library/react
: Helps test React componentsvite-tsconfig-paths
: Enables path aliases from your tsconfig
2. Create Configuration File
Create a new file called vitest.config.mts
(or vitest.config.js
for JavaScript projects) in your project root:
import { defineConfig } from 'vitest/config';
import react from '@vitejs/plugin-react';
import tsconfigPaths from 'vite-tsconfig-paths';
export default defineConfig({
plugins: [tsconfigPaths(), react()],
test: {
environment: 'jsdom',
include: ['**/*.{test,spec}.{js,mjs,cjs,ts,mts,cts,jsx,tsx}'],
globals: true
},
});
3. Update Package.json
Add the test script to your package.json
:
{
"scripts": {
"test": "vitest",
"test:ui": "vitest --ui",
"test:coverage": "vitest run --coverage"
}
}
4. Create Test Setup File (Optional)
For additional setup like global mocks or custom matchers, create a setup.ts
file:
import '@testing-library/jest-dom';
import { cleanup } from '@testing-library/react';
import { afterEach } from 'vitest';
// Automatically cleanup after each test
afterEach(() => {
cleanup();
});
Writing Your First Test
Let's create a simple test to verify our setup. We'll test a basic Next.js page component:
Example Component and Test
First, create a simple page component (
app/pages/hello.tsx
):
export default function HelloPage() {
return (
<div>
<h1>Hello, Vitest!</h1>
<p data-testid="greeting">Welcome to testing with Vitest</p>
</div>
);
}
Create a corresponding test file (
__tests__/hello.test.tsx
):
import { describe, expect, it } from 'vitest';
import { render, screen } from '@testing-library/react';
import HelloPage from '../app/pages/hello';
describe('HelloPage', () => {
it('renders hello message', () => {
render(<HelloPage />);
expect(screen.getByRole('heading')).toHaveTextContent('Hello, Vitest!');
expect(screen.getByTestId('greeting')).toBeInTheDocument();
});
});
Running Tests
Now you can run your tests using any of these commands:
# Run tests in watch mode
npm test
# Run tests once
npm test run
# Run tests with UI
npm run test:ui
# Generate coverage report
npm run test:coverage
Common Challenges and Solutions
1. Dependency Confusion
Many developers express confusion about which dependencies they need. As one developer asked on Reddit: "Do I need both Jest and Vitest?"
The answer is no - Vitest and Jest are both test runners, and you should choose one or the other. Vitest is designed to work with React Testing Library, which handles the actual component testing.
2. Configuration Issues
If you're encountering configuration problems, check these common issues:
Ensure all peer dependencies are installed
Verify your
vitest.config
file is in the root directoryCheck that file extensions in your test patterns match your project
Confirm your TypeScript configuration is properly set up
3. Performance Optimization
While some developers claim that "Jest is still faster than Vitest," many find the opposite to be true. To optimize Vitest performance:
Use the
--threads
flag for parallel testingImplement proper test isolation
Utilize the watch mode effectively
Consider using the UI mode for better test debugging
Best Practices and Tips
1. Organizing Tests
Structure your tests to mirror your source code:
src/
components/
Button.tsx
Button.test.tsx
pages/
index.tsx
index.test.tsx
2. Using Test Utilities
Create a test-utils.ts
file for common testing patterns:
import { render } from '@testing-library/react';
const customRender = (ui: React.ReactElement, options = {}) =>
render(ui, {
// wrap provider(s) here if needed
...options,
});
export * from '@testing-library/react';
export { customRender as render };
3. Mocking Next.js Features
When testing Next.js-specific features, you might need to mock certain modules:
vi.mock('next/router', () => ({
useRouter: () => ({
push: vi.fn(),
pathname: '/',
}),
}));
Additional Resources
Conclusion
Setting up Vitest for Next.js 15 doesn't have to be complicated. While the initial configuration might seem daunting, the benefits of faster test execution and simpler maintenance make it worth the effort. As one developer mentioned, "the transition from Jest to Vitest is almost trivial," and with this guide, you should be well-equipped to make that transition smoothly.
Remember that testing is an essential part of professional development. As noted in a Reddit discussion: "Pretty much all of the companies I worked at half-assed testing until the most recent one, and it made a hell of a lot of difference."
Start small, focus on writing meaningful tests, and gradually build up your test coverage. With Vitest and Next.js 15, you have a powerful combination that can help ensure your application's reliability and maintainability.