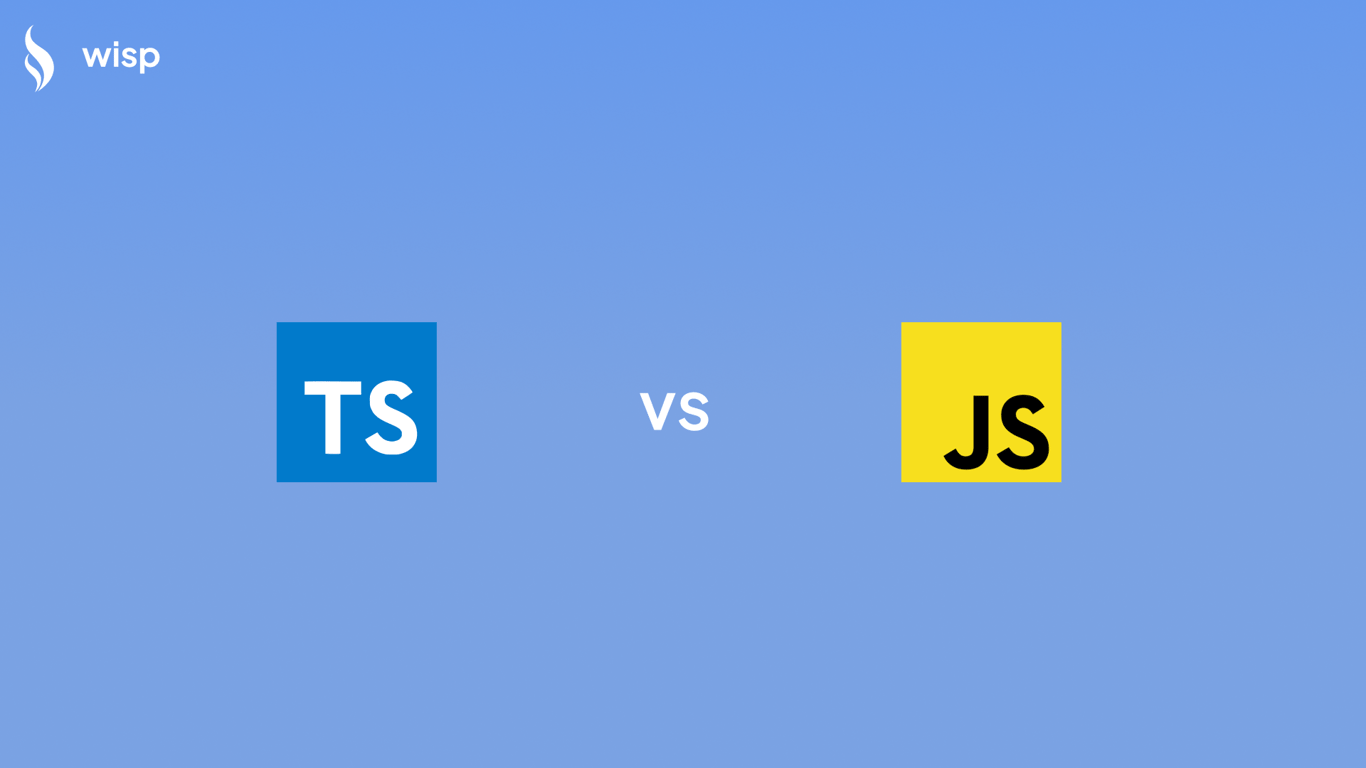
As a senior developer, choosing the right programming language for your project is critical. The debate between TypeScript and JavaScript is ongoing, and both languages have their strengths and weaknesses. This guide aims to provide an in-depth comparison between TypeScript and JavaScript, offering insights and code examples to help you make an informed decision.
Understanding JavaScript
JavaScript is a dynamic, interpreted programming language that forms the backbone of web development. It allows developers to create interactive web pages and is widely supported across all browsers.
Key Features of JavaScript
Interactivity with the DOM: JavaScript can access and modify the DOM of a webpage dynamically.
document.getElementById("demo").innerHTML = "Hello, JavaScript!";
Event Handling: JavaScript responds to user actions via event listeners.
document.getElementById("myButton").addEventListener("click", function() { alert("Button clicked!"); });
Asynchronous Programming: Supports asynchronous operations using callbacks, promises, and async/await.
async function fetchData() { let response = await fetch('https://api.example.com/data'); let data = await response.json(); console.log(data); } fetchData();
Dynamic Typing: Variables can change types at runtime.
let example = "Hello, world!"; console.log(typeof example); // "string" example = 42; console.log(typeof example); // "number"
Understanding TypeScript
TypeScript is a statically typed superset of JavaScript developed by Microsoft. It introduces static types to JavaScript, allowing for better tooling and error checking.
Key Features of TypeScript
Static Type Checking: TypeScript adds static type checking, catching errors at compile time.
let message: string = "Hello, TypeScript!"; // message = 123; // This line would cause a compile-time error
Interfaces: Allows defining the shape of objects.
interface User { name: string; age: number; } const user: User = { name: "Alice", age: 30 };
Classes and Inheritance: Supports classes and inheritance with additional features like access modifiers.
class Animal { name: string; constructor(name: string) { this.name = name; } move(distanceInMeters: number = 0) { console.log(`${this.name} moved ${distanceInMeters} m.`); } } class Snake extends Animal { constructor(name: string) { super(name); } move(distanceInMeters = 5) { console.log("Slithering..."); super.move(distanceInMeters); } }
Generics: Allows creation of reusable components that work with multiple types.
function identity<T>(arg: T): T { return arg; } let output1 = identity<string>("myString"); let output2 = identity<number>(68);
Enums: Adds support for defining a set of named constants.
enum Color { Red, Green, Blue, } let c: Color = Color.Green;
Advanced Types: Supports union types, intersection types, and type guards for more flexible type manipulation.
type StringOrNumber = string | number; function logMessage(message: StringOrNumber): void { if (typeof message === "string") { console.log("String message: " + message); } else { console.log("Number message: " + message); } }
Differences Between TypeScript and JavaScript
Static vs Dynamic Typing
TypeScript is statically typed, meaning types are checked at compile time. JavaScript is dynamically typed, meaning types are resolved at runtime.
Example - TypeScript:let myString: string = "this is ts";
myString = 42; // TypeScript will warn this is a mistake
Example - JavaScript:let myString = "this is js";
myString = 42; // JavaScript will allow this
Compile-Time Type Checking
TypeScript: Allows for catching type-related errors at compile time, reducing runtime errors.
JavaScript: Lacks this feature, meaning type-related errors are caught only at runtime.
Class and Interface Support
TypeScript: Supports modern OOP features like classes and interfaces, enabling more structured and maintainable code.
JavaScript: Supports classes but TypeScript’s implementation is more robust and includes interfaces.
Tooling and IDE Support
TypeScript: Static typing enables superior tooling support with features like auto-completion, navigation, and advanced refactoring.
JavaScript: Has good tooling support but lacks some advanced features due to its dynamic nature.
Community and Ecosystem
JavaScript: Vast, established community and a massive library ecosystem.
TypeScript: Rapidly growing, with increasing library support and community engagement.
Learning Curve
JavaScript: Generally easier for beginners due to its simplicity and flexibility.
TypeScript: Requires understanding of types and additional syntax, posing a steeper learning curve.
Benefits and Drawbacks
TypeScript Benefits:
Error prevention during development.
Improved development experience with better IDE support.
Safer code, especially in collaborative environments.
TypeScript Drawbacks:
Requires more initial coding effort and learning.
Some developers may find it verbose or cumbersome.
JavaScript Benefits:
Faster to write and test small projects.
Flexible and less strict about types.
Larger community and more resources for learning.
JavaScript Drawbacks:
Higher potential for runtime errors.
Difficult to manage in large, complex projects.
When to Use TypeScript vs JavaScript
TypeScript
Large projects: Better for maintaining large codebases and teams.
External libraries: Useful when using libraries with type definitions.
Safety and maintenance: Reduces long-term errors and improves code safety.
JavaScript
Quick scripts or personal projects: Faster for simple or smaller projects.
Speed and flexibility: Allows rapid development and flexibility at the cost of potential runtime errors.
Code Examples
JavaScript - Adding Numbers
function addNumbers(a, b) {
return a + b;
}
console.log(addNumbers(5, 10)); // Output: 15
TypeScript - Adding Numbers with Type Safety
function addNumbers(a: number, b: number): number {
return a + b;
}
console.log(addNumbers(5, 10)); // Output: 15
Conclusion
Choosing between TypeScript and JavaScript depends on the project requirements and the team's familiarity with the languages. While JavaScript offers flexibility and simplicity, TypeScript provides type safety and improved tooling, making it suitable for larger projects. Understanding the strengths and weaknesses of both can help senior developers make the best choice for their specific needs.