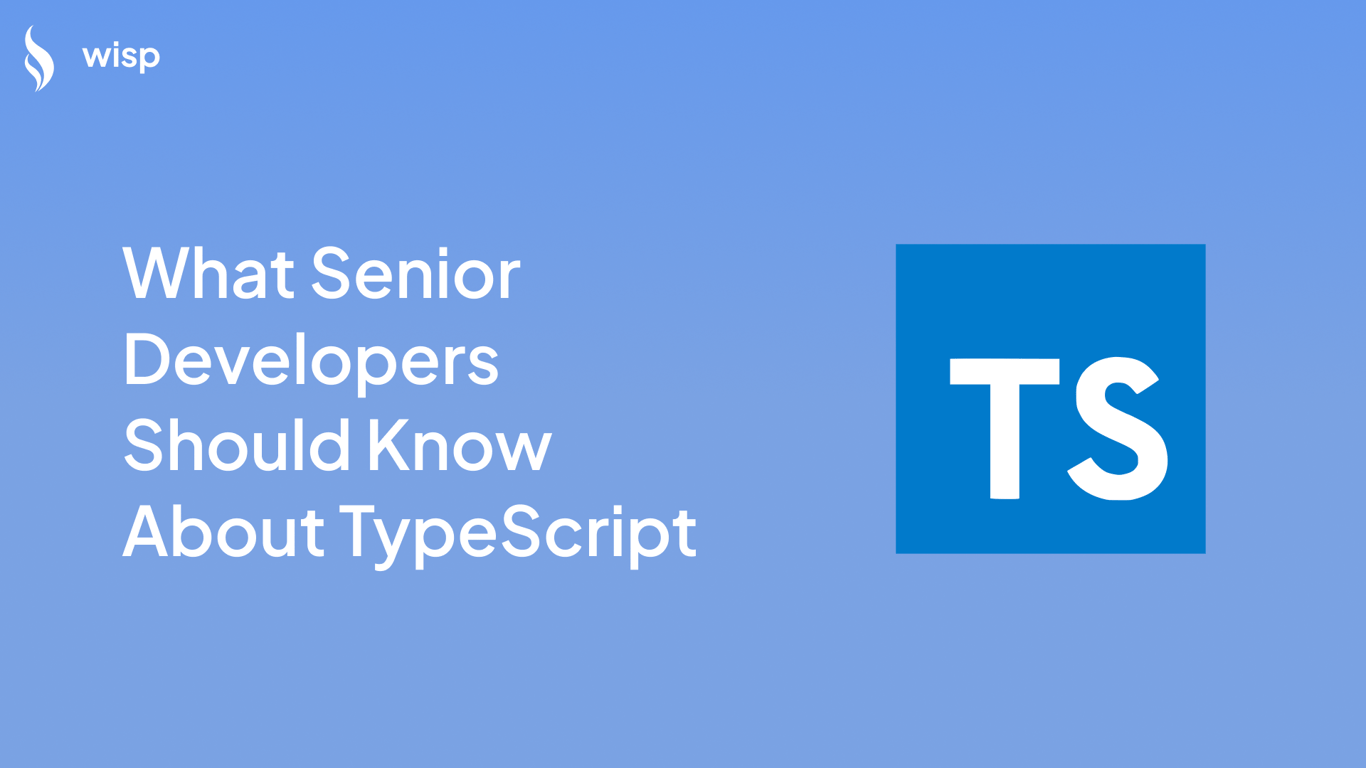
TypeScript has revolutionized the way developers write JavaScript, offering a static type system that enhances code quality and maintainability. For senior developers, mastering TypeScript can unlock new levels of efficiency and robustness in large-scale applications. This guide dives into advanced TypeScript features, best practices, and real-world examples to help you leverage TypeScript to its fullest potential.
Advanced TypeScript Features
Conditional Types
Conditional types allow for dynamic type transformations based on a condition, providing greater flexibility in type definitions.
// Example of Conditional Types
interface Dog { bark: () => void; }
interface Cat { meow: () => void; }
type Pet = Dog | Cat;
type PetSoundFunction<T extends Pet> = T extends Dog ? T['bark'] : T['meow'];
function getSound<T extends Pet>(pet: T): PetSoundFunction<T> {
if ('bark' in pet) {
return pet.bark;
} else {
return pet.meow;
}
}
Mapped Types
Mapped types transform properties of an existing type into a new type. They are particularly useful for creating variations of existing types.
// Example of Mapped Types
type ReadOnly<T> = { readonly [P in keyof T]: T[P]; }
interface User { name: string; age: number; }
const readOnlyUser: ReadOnly<User> = { name: 'John Doe', age: 30 };
// readOnlyUser.name = 'Jane Doe'; // Error: Cannot assign to 'name' because it is a read-only property
Template Literal Types
Template literal types build new string types by combining string literals and allowing dynamic expressions.
// Example of Template Literal Types
type Endpoint = `/users/${number}`;
const validEndpoint: Endpoint = '/users/123';
// const invalidEndpoint: Endpoint = '/posts/456'; // Error: Type '"/posts/456"' is not assignable to type 'Endpoint'
Higher-Order Types
Higher-order types are types that use other types as parameters or return values, enabling higher abstraction levels.
// Example of Higher-Order Types
type IsStringLiteral<T> = T extends string ? true : false;
function logIfString<T>(value: T): T extends string ? void : T {
if (typeof value === 'string') {
console.log(value);
}
return value;
}
Recursive Types
Recursive types are self-referencing and enable the definition of complex data structures.
// Example of Recursive Types
interface LinkedList<T> {
value: T;
next?: LinkedList<T>;
}
const numbersList: LinkedList<number> = {
value: 1,
next: {
value: 2,
next: {
value: 3,
next: null
}
}
};
Type Guards
Type guards allow you to narrow down the type of a variable within a block of code, improving type safety.
// Example of Type Guards
type NumberOrString = number | string;
function isNumber(value: NumberOrString): value is number {
return typeof value === 'number';
}
const value: NumberOrString = 123;
if (isNumber(value)) {
console.log('Value is a number:', value);
} else {
console.log('Value is a string:', value);
}
TypeScript Best Practices for Senior Developers
Type Inference vs. Explicit Types
TypeScript's type inference capabilities are powerful, but knowing when to use explicit types can lead to more robust and readable code.
// Example of Type Inference
let inferredString = 'Hello World'; // inferred as string
// Example of Explicit Types
let explicitString: string = 'Hello World';
Union and Intersection Types
Union types combine multiple types into one, while intersection types merge multiple types, providing flexibility and precision.
// Example of Union and Intersection Types
type NumberOrString = number | string;
type Point = { x: number; y: number; };
type LabeledPoint = Point & { label: string; };
const value: NumberOrString = 123; // or value = "hello"
const labeledPoint: LabeledPoint = { x: 0, y: 0, label: 'origin' };
Leveraging Utility Types
Utility types help manipulate type definitions and enhance code reusability.
// Examples of Built-In Utility Types
type User = { id: number; name: string; email: string; };
type PartialUser = Partial<User>;
type RequiredUser = Required<User>;
type ReadonlyUser = Readonly<User>;
// Custom Utility Type
type Nullable<T> = { [P in keyof T]: T[P] | null };
const nullableUser: Nullable<User> = { id: 1, name: null, email: 'user@example.com' };
Effective Use of Generics
Generics provide a way to create reusable components and functions, enhancing type safety and flexibility.
// Example of Generics
function identity<T>(arg: T): T {
return arg;
}
const num = identity<number>(42);
const str = identity<string>('Hello');
Code Maintainability and Reusability
Modular Code
Organizing your code into modules is essential for maintaining a clean and manageable codebase. It helps in isolating functionalities and encourages reusability.
Best Practices for File Structuring:
Group related files together (e.g., components, utilities, types).
Use consistent naming conventions for files and directories.
Avoid deep nesting of directories.
Example Code:
// File: src/components/Button.tsx
import React from 'react';
interface ButtonProps {
label: string;
onClick: () => void;
}
const Button: React.FC<ButtonProps> = ({ label, onClick }) => (
<button onClick={onClick}>{label}</button>
);
export default Button;
// File: src/utils/math.ts
export const add = (a: number, b: number): number => a + b;
// File: src/types/index.ts
export interface User {
id: number;
name: string;
email: string;
}
Reusable Components and Hooks
Creating reusable components and hooks not only improves code reusability but also enhances maintainability and consistency across your application.
Creating Reusable React Components:
// File: src/components/TextInput.tsx
import React, { FC } from 'react';
interface TextInputProps {
value: string;
onChange: (value: string) => void;
placeholder?: string;
}
const TextInput: FC<TextInputProps> = ({ value, onChange, placeholder }) => (
<input
type="text"
value={value}
onChange={(e) => onChange(e.target.value)}
placeholder={placeholder}
/>
);
export default TextInput;
Example Custom Hook:
// File: src/hooks/useFetch.ts
import { useState, useEffect } from 'react';
interface FetchState<T> {
data: T | null;
loading: boolean;
error: Error | null;
}
const useFetch = <T, >(url: string): FetchState<T> => {
const [data, setData] = useState<T | null>(null);
const [loading, setLoading] = useState<boolean>(true);
const [error, setError] = useState<Error | null>(null);
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch(url);
const result = await response.json();
setData(result);
} catch (err) {
setError(err);
} finally {
setLoading(false);
}
};
fetchData();
}, [url]);
return { data, loading, error };
};
export default useFetch;
Design Patterns in TypeScript
Incorporating design patterns into your TypeScript projects can lead to more organized and maintainable code. Understanding and implementing common design patterns can greatly enhance your ability to solve complex problems efficiently.
Common Design Patterns and Their Implementations:
Singleton Pattern: Ensures a class has only one instance and provides a global point of access to it.
class Singleton {
private static instance: Singleton;
private constructor() {}
public static getInstance(): Singleton {
if (!Singleton.instance) {
Singleton.instance = new Singleton();
}
return Singleton.instance;
}
}
const singletonInstance = Singleton.getInstance();
Observer Pattern: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
interface Observer {
update(message: string): void;
}
class Subject {
private observers: Observer[] = [];
public addObserver(observer: Observer): void {
this.observers.push(observer);
}
public removeObserver(observer: Observer): void {
this.observers = this.observers.filter(obs => obs !== observer);
}
public notifyObservers(message: string): void {
this.observers.forEach(observer => observer.update(message));
}
}
Factory Pattern: Creates an instance of several derived classes.
interface Product {
create(): void;
}
class ConcreteProductA implements Product {
public create() {
console.log('ConcreteProductA created');
}
}
class ConcreteProductB implements Product {
public create() {
console.log('ConcreteProductB created');
}
}
class Factory {
public static createProduct(type: string): Product {
if (type === 'A') {
return new ConcreteProductA();
} else if (type === 'B') {
return new ConcreteProductB();
}
throw new Error('Invalid product type');
}
}
const productA = Factory.createProduct('A');
productA.create();
Handling Complex Types
Type Definition Files
Type definition files (.d.ts
files) are essential for providing type information about JavaScript libraries to TypeScript. They describe the shape of existing JavaScript code, allowing TypeScript to offer type checking and better tooling.
What They Are and How to Use Them:
Type definition files define the types of functions, classes, and other objects.
TypeScript uses these definitions to provide intellisense and compile-time type checking.
Example Code:
// File: myLibrary.d.ts
export function greet(name: string): string;
// Usage in TypeScript file
import { greet } from './myLibrary';
const greeting = greet('World');
Discriminated Unions
Discriminated unions, also known as tagged unions or algebraic data types, are a powerful feature that allows you to model complex data structures in a type-safe way.
Definition and Use Cases:
Discriminated unions use a common property (the discriminant) to differentiate between different types.
Useful for handling different shapes of data that a variable might represent.
Example Code:
interface Square { kind: 'square'; size: number; }
interface Rectangle { kind: 'rectangle'; width: number; height: number; }
interface Circle { kind: 'circle'; radius: number; }
type Shape = Square | Rectangle | Circle;
function area(shape: Shape): number {
switch (shape.kind) {
case 'square': return shape.size * shape.size;
case 'rectangle': return shape.width * shape.height;
case 'circle': return Math.PI * shape.radius * shape.radius;
}
}
Advanced Type Guards
Advanced type guards go beyond simple checks and allow for more complex type inference within your code.
Creating and Using Custom Type Guards:
interface Bird { fly(): void; layEggs(): void; }
interface Fish { swim(): void; layEggs(): void; }
type Pet = Bird | Fish;
function isBird(pet: Pet): pet is Bird {
return (pet as Bird).fly !== undefined;
}
function getPet(): Pet {
// Imagine some complex logic here
return { fly: () => console.log('Flying!'), layEggs: () => console.log('Laying eggs!') } as Bird;
}
const pet = getPet();
if (isBird(pet)) {
pet.fly(); // TypeScript knows pet is Bird
} else {
pet.swim(); // TypeScript knows pet is Fish
}
Real-World Examples and Case Studies
Example 1: Building a Type-Safe Form Handling Library
Problem Definition: Handling form state can become complex and error-prone, especially with dynamic forms.
TypeScript Implementation: A simple type-safe form handling hook using TypeScript.
Example Code:
// File: src/hooks/useForm.ts
import { useState } from 'react';
function useForm<T>(initialValues: T) {
const [values, setValues] = useState<T>(initialValues);
const handleChange = (e: React.ChangeEvent<HTMLInputElement>) => {
const { name, value } = e.target;
setValues({ ...values, [name]: value });
};
return { values, handleChange };
}
export default useForm;
Benefits and Challenges:
Benefits: Type safety ensures that only valid form fields are updated.
Challenges: Requires careful type management especially with deeply nested form structures.
Example 2: Refactoring a JavaScript Codebase to TypeScript
Step-by-Step Process:
Setup TypeScript: Initialize TypeScript in the project.
npm install typescript --save-dev
npx tsc --init
Rename Files: Change file extensions from
.js
to.ts
or.tsx
.Add Type Definitions: Gradually add type definitions starting from simple types and moving to more complex interfaces.
Fix Errors: Resolve TypeScript errors and warnings iteratively.
Lessons Learned:
Start small and gradually convert the codebase.
Utilize community TypeScript definitions from DefinitelyTyped.
Continuously run tests to ensure functionality remains intact.
Example 3: Using TypeScript in a Large-Scale React Application
Overview of the Application: A comprehensive e-commerce application with complex state management and dynamic features.
TypeScript Benefits Observed:
Improved developer experience with better tooling and autocomplete.
Early detection of bugs through static type checking.
Enhanced code maintainability and readability.
Common Pitfalls and How to Avoid Them
Misunderstanding Type Inference
Relying too much on type inference can lead to subtle bugs. Explicit types should be used when the inference is not obvious.
Overusing Type Assertions
Using type assertions (
as
keyword) to override TypeScript's type system can cause runtime errors if done incorrectly. Use assertions sparingly and only when necessary.
Ignoring Narrowing and Guards
Ignoring type narrowing and guards can lead to type safety issues. Always use type guards to validate types in complex conditions.
Conclusion
TypeScript offers a robust set of tools and features that can significantly enhance code quality and maintainability, especially in large-scale applications. By understanding and utilizing advanced TypeScript features, following best practices, and continually learning, senior developers can master TypeScript to build more reliable and scalable software.