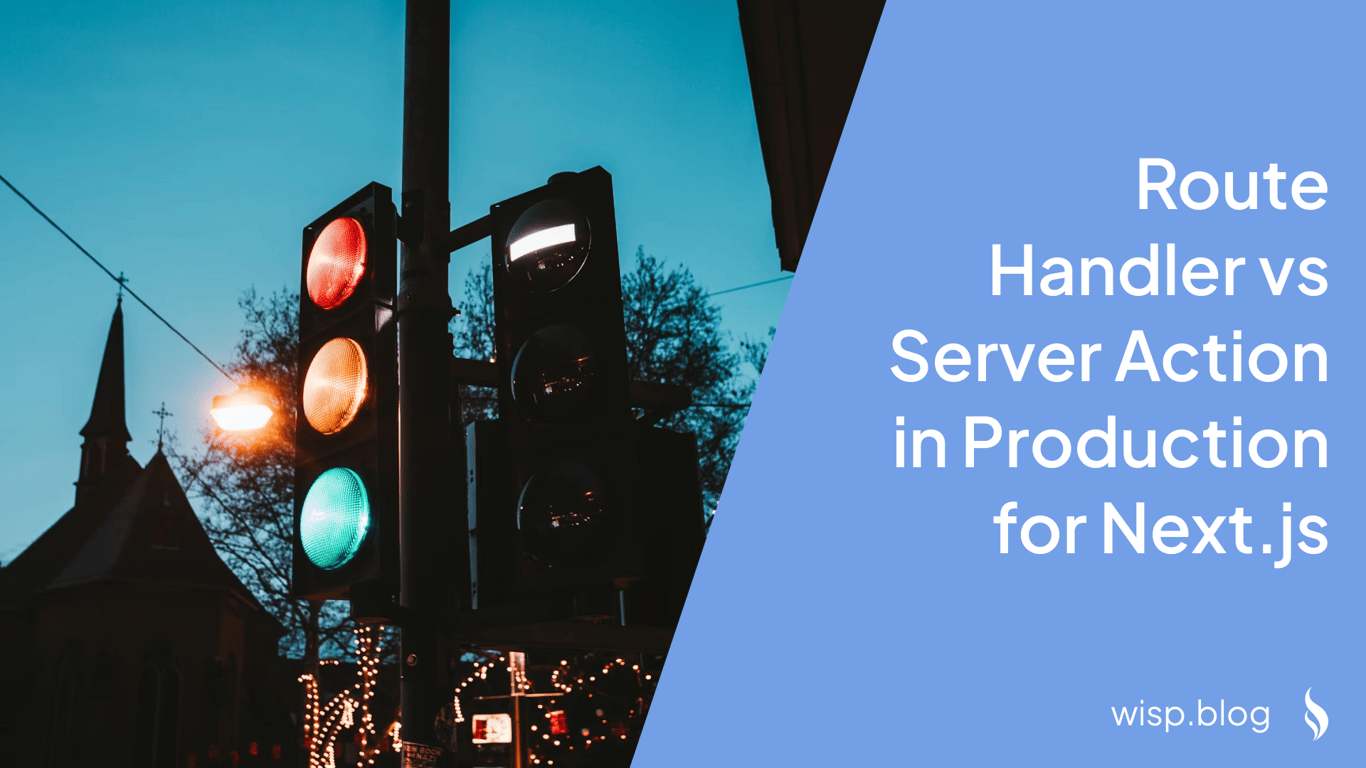
You've been building Next.js applications and now you're faced with a crucial decision: should you use Route Handlers or Server Actions for your server-side logic? This choice becomes especially critical when preparing for production deployment, where performance, maintainability, and scalability are paramount.
"I want a honest opinion from you guys there that which one is better from a professional's perspective in scale of small, medium and large projects," asks a developer in a recent Reddit discussion. This sentiment echoes throughout the Next.js community, particularly among developers preparing their first production deployment.
The confusion is understandable. Both approaches seem to solve similar problems, yet they serve distinctly different purposes. Some developers express strong preferences: "I personally hate to make REST API from that /api stuff when I can manage server actions for every type of CRUD." However, such blanket preferences might not always lead to the best architectural decisions.
Let's dive deep into both approaches, understand their strengths and limitations, and develop a clear framework for choosing between them in production environments.
Understanding Route Handlers
Route Handlers in Next.js are specialized functions that handle HTTP requests at specific routes. They're defined in route.js
or route.ts
files within your application's directory structure and provide a way to create API endpoints.
Here's a basic example of a Route Handler:
// app/api/users/route.ts
import { NextRequest, NextResponse } from 'next/server';
export async function GET(request: NextRequest) {
const users = await prisma.user.findMany();
return NextResponse.json(users);
}
export async function POST(request: NextRequest) {
const data = await request.json();
const newUser = await prisma.user.create({
data: data
});
return NextResponse.json(newUser, { status: 201 });
}
Key characteristics of Route Handlers:
Full HTTP Method Support: They support all standard HTTP methods (GET, POST, PUT, DELETE, etc.)
API-First Design: Perfect for creating RESTful APIs
External Accessibility: Can be called from any client, not just your Next.js application
Flexible Response Types: Support various response formats including JSON, text, and streams
Understanding Server Actions
Server Actions represent a paradigm shift in how we handle server-side operations in Next.js applications. They're functions that execute on the server but can be called directly from your components, bridging the gap between client and server seamlessly.
Here's how a typical Server Action looks:
// app/actions/user.ts
'use server';
export async function createUser(formData: FormData) {
const name = formData.get('name');
const email = formData.get('email');
const newUser = await prisma.user.create({
data: {
name: name as string,
email: email as string,
}
});
revalidatePath('/users');
return newUser;
}
// Using in a component
export default function UserForm() {
return (
<form action={createUser}>
<input name="name" type="text" />
<input name="email" type="email" />
<button type="submit">Create User</button>
</form>
);
}
Key characteristics of Server Actions:
Progressive Enhancement: Works even without JavaScript enabled
Form Handling: Seamless integration with form submissions
Automatic Serialization: Handles data transfer between client and server
Built-in Validation: Can validate data before processing
Optimistic Updates: Supports optimistic UI updates for better UX
"Server actions should literally correspond to an action: a user does something and expects something to change in response," explains a developer in a community discussion. This perfectly encapsulates the primary use case for Server Actions.
Key Differences in Production
When deploying to production, understanding the fundamental differences between Route Handlers and Server Actions becomes crucial:
1. Request Method Support
Route Handlers:
Support all HTTP methods
Can handle complex request patterns
Ideal for RESTful API design
Server Actions:
Limited to POST requests
Optimized for form submissions and mutations
Simplified data handling
2. Caching and Performance
Route Handlers:
// app/api/cached-data/route.ts
export async function GET() {
const data = await fetchData();
return new Response(JSON.stringify(data), {
headers: {
'Cache-Control': 'max-age=3600',
},
});
}
Server Actions:
'use server';
export async function getData() {
// Automatically uses Next.js caching
const data = await fetch('https://api.example.com/data');
return data.json();
}
3. Error Handling and Validation
Route Handlers:
// app/api/protected/route.ts
import { NextResponse } from 'next/server';
export async function GET(request: Request) {
try {
const token = request.headers.get('authorization');
if (!token) {
return NextResponse.json(
{ error: 'Unauthorized' },
{ status: 401 }
);
}
const data = await fetchProtectedData(token);
return NextResponse.json(data);
} catch (error) {
return NextResponse.json(
{ error: 'Internal Server Error' },
{ status: 500 }
);
}
}
Server Actions:
'use server';
export async function submitForm(formData: FormData) {
try {
// Validation
const email = formData.get('email');
if (!email || !email.includes('@')) {
throw new Error('Invalid email');
}
// Processing
await processForm(formData);
revalidatePath('/dashboard');
return { success: true };
} catch (error) {
return { error: error.message };
}
}
4. Integration with External Services
Route Handlers:
Better suited for webhook endpoints
Can handle various content types
Easier to integrate with third-party services
Server Actions:
Optimized for internal application logic
Better for direct database operations
Tighter integration with Next.js features
When to Use Which in Production
Use Route Handlers When:
Building Public APIs
// app/api/v1/products/route.ts export async function GET(request: Request) { const { searchParams } = new URL(request.url); const category = searchParams.get('category'); const products = await prisma.product.findMany({ where: { category: category || undefined } }); return NextResponse.json(products); }
Handling Webhooks
// app/api/webhook/stripe/route.ts export async function POST(request: Request) { const payload = await request.text(); const sig = request.headers.get('stripe-signature'); try { const event = stripe.webhooks.constructEvent( payload, sig, process.env.STRIPE_WEBHOOK_SECRET ); // Process webhook return new Response('OK', { status: 200 }); } catch (err) { return new Response('Webhook Error', { status: 400 }); } }
Use Server Actions When:
Handling Form Submissions
// app/actions/contact.ts 'use server'; export async function submitContactForm(formData: FormData) { const email = formData.get('email'); const message = formData.get('message'); await sendEmail({ to: 'support@example.com', from: email as string, message: message as string }); revalidatePath('/contact'); }
Managing User Authentication
// app/actions/auth.ts 'use server'; export async function login(formData: FormData) { const email = formData.get('email'); const password = formData.get('password'); const user = await authenticate(email, password); if (user) { cookies().set('session', user.sessionToken); redirect('/dashboard'); } return { error: 'Invalid credentials' }; }
Best Practices for Production
Error Handling
// Shared error handling utility const handleError = (error: unknown) => { console.error(error); if (error instanceof ValidationError) { return { error: error.message, status: 400 }; } return { error: 'An unexpected error occurred', status: 500 }; }; // In Route Handler export async function POST(request: Request) { try { const data = await request.json(); // Process data return NextResponse.json({ success: true }); } catch (error) { const { error: message, status } = handleError(error); return NextResponse.json({ error: message }, { status }); } } // In Server Action export async function processData(data: FormData) { try { // Process data return { success: true }; } catch (error) { return handleError(error); } }
Performance Optimization
For Route Handlers:
// app/api/cached-data/route.ts
import { NextResponse } from 'next/server';
export async function GET() {
const data = await fetchExpensiveData();
return NextResponse.json(
{ data },
{
headers: {
'Cache-Control': 'public, s-maxage=3600, stale-while-revalidate=86400',
},
}
);
}
For Server Actions:
'use server';
import { unstable_cache } from 'next/cache';
export const getCachedData = unstable_cache(
async () => {
const data = await fetchExpensiveData();
return data;
},
['cached-data'],
{
revalidate: 3600,
tags: ['cached-data'],
}
);
Security Considerations
// Middleware for Route Handlers
// middleware.ts
export function middleware(request: NextRequest) {
const token = request.headers.get('authorization');
if (!token && request.nextUrl.pathname.startsWith('/api/')) {
return NextResponse.json(
{ error: 'Unauthorized' },
{ status: 401 }
);
}
return NextResponse.next();
}
// Security for Server Actions
'use server';
import { getServerSession } from 'next-auth/next';
export async function protectedAction(formData: FormData) {
const session = await getServerSession();
if (!session) {
throw new Error('Unauthorized');
}
// Proceed with protected operation
}
Making the Decision
When deciding between Route Handlers and Server Actions in production, consider these factors:
External Accessibility
Need external API access? → Route Handlers
Internal application logic only? → Server Actions
Data Operations
Complex data aggregation? → Route Handlers
Simple CRUD operations? → Server Actions
Client Integration
Third-party service integration? → Route Handlers
Form submissions and UI updates? → Server Actions
Performance Requirements
Need fine-grained caching control? → Route Handlers
Automatic caching sufficient? → Server Actions
Conclusion
The choice between Route Handlers and Server Actions in Next.js isn't about which is "better" – it's about using the right tool for the job. Route Handlers excel at creating robust APIs and handling external integrations, while Server Actions shine in managing internal application state and form submissions.
As one developer noted in the community, "Modern software is having a lot of functionality and one way for us coders to understand code it faster is to keep functionality closely related in the file structure." This principle should guide your decision-making process.
Remember:
Route Handlers for public APIs and complex integrations
Server Actions for internal operations and form handling
Consider using both when appropriate – they're complementary, not competitive
By understanding these distinctions and following the best practices outlined above, you can build more maintainable, scalable, and efficient Next.js applications in production.