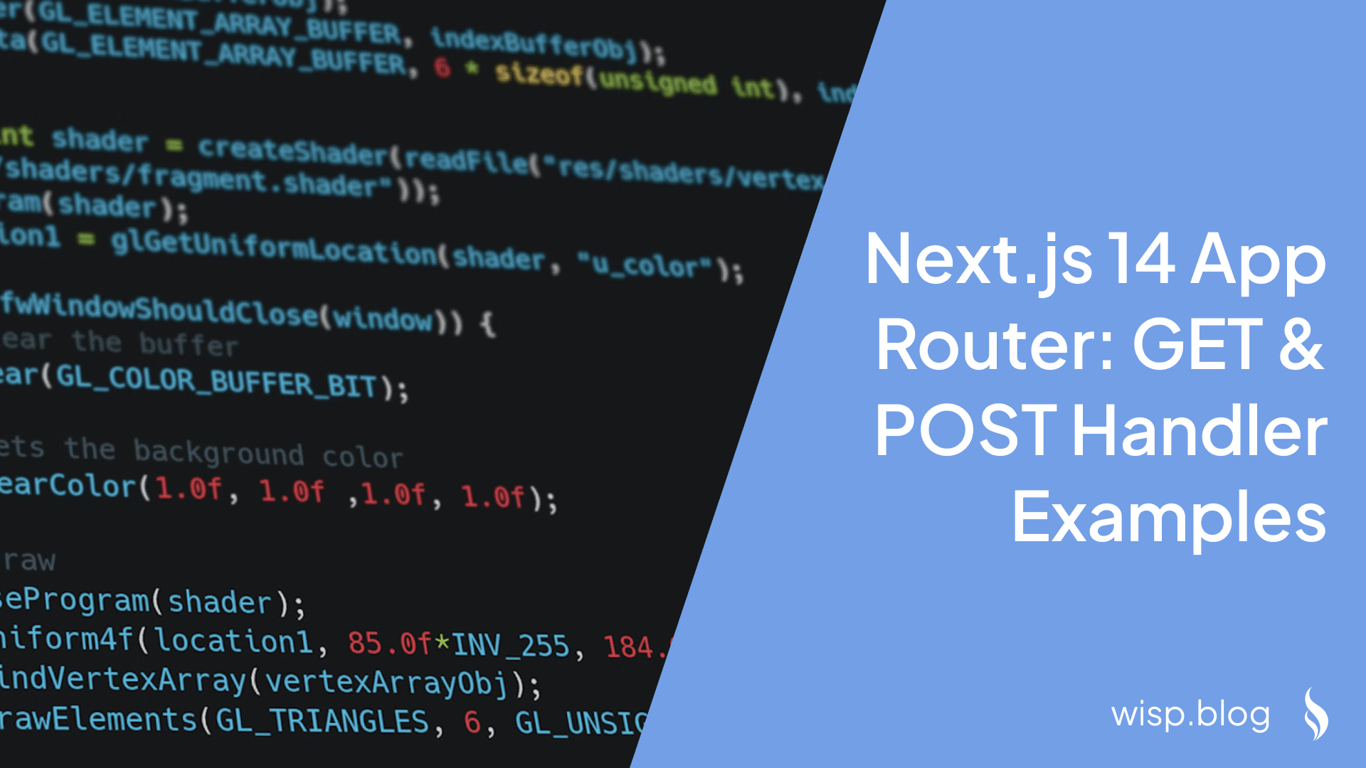
Next.js has rapidly become one of the most popular frameworks for building React applications. With the release of Next.js 14, the framework introduces the App Router, an innovative approach to handling routing in your applications. This article will explore the specifics of implementing GET and POST handlers using the new App Router, complete with comprehensive examples and best practices to enhance your development workflow.
Whether you're fetching data from an external API with GET requests or handling form submissions with POST requests, understanding how to utilize these new capabilities will be crucial for building robust and scalable applications.
1. Understanding Next.js 14 App Router
The Next.js 14 App Router is a revolutionary feature that allows developers to handle routing within their applications more efficiently. Unlike previous versions, where API routes were primarily defined in the pages
directory, the new App Router standardizes these routes inside the app
directory. This approach brings several enhancements including better performance, reduced initial load times, and improved support for dynamic routing.
Key Features
Centralized Routing: All routes are defined in the
app
directory, simplifying the structure and improving maintainability.Dynamic Routing: Enhanced support for dynamic routing with features like Catch-All and Dynamic Segments, making it easier to build complex applications.
Extended Request and Response APIs: Built-in enhancements to the native
Request
andResponse
objects, providing more functionality out-of-the-box.Improved Performance: Optimizations that reduce the overhead and improve the load times of your applications.
2. Setting Up Your Project
Before delving into GET and POST handlers, it's essential to have a Next.js project set up. Here's a quick guide to get you started with a new Next.js project and set up the necessary directory structure.
Creating a New Next.js Project
You can create a new Next.js project using the following command:
npx create-next-app@latest my-next-app
Navigate into your project directory:
cd my-next-app
Directory Structure
Next.js 14 introduces a new structure for handling routes within the app
directory. Below is an example of how your project directory might look:
my-next-app/
├── app/
│ ├── api/
│ │ ├── hello/
│ │ │ └── route.js
│ ├── favicon.ico
│ ├── globals.css
│ ├── layout.js
│ └── page.js
├── public/
├── styles/
├── .gitignore
├── package.json
└── README.md
3. Basic Route Handler Configuration
What are Route Handlers?
Route handlers are functions that handle specific HTTP requests to particular routes in your application. They replace the traditional API routes and offer a more centralized and organized approach to handling requests in Next.js applications.
Creating a Simple GET Handler
To create a basic GET handler, you'll need to define a route.js
file within your desired route directory. Here is an example of a simple GET handler that returns a plain text response:
// app/api/hello/route.js
export async function GET(request) {
return new Response('Hello, Next.js 14!');
}
This handler listens for GET requests to the /api/hello
endpoint and responds with a plain text message.
Creating a Simple POST Handler
Similarly, you can create a POST handler to handle form submissions or other POST requests. Here’s an example:
// app/api/submit/route.js
export async function POST(request) {
const body = await request.json();
return new Response(`Received data: ${JSON.stringify(body)}`);
}
This POST handler listens for POST requests to the /api/submit
endpoint, reads the JSON body from the request, and responds with the received data.
4. Advanced GET Handlers
Fetching Data from APIs
One powerful use of GET handlers is to fetch data from external APIs. This allows you to integrate third-party data sources seamlessly into your Next.js application. Here’s an example GET handler that fetches product data from an external API:
// app/api/product/route.js
export async function GET(request) {
const { searchParams } = new URL(request.url);
const id = searchParams.get('id');
const res = await fetch(`https://api.example.com/product/${id}`);
const product = await res.json();
return new Response(JSON.stringify(product), {
headers: { 'Content-Type': 'application/json' },
});
}
In this example, the GET handler extracts a product ID from the query parameters, fetches the corresponding product data from an external API, and returns it as a JSON response.
Handling Query Parameters
Query parameters can be essential for filtering and fetching specific data. Here’s how you can handle them within your GET handlers:
// app/api/items/route.js
export async function GET(request) {
const { searchParams } = new URL(request.url);
const category = searchParams.get('category');
const res = await fetch(`https://api.example.com/items?category=${category}`);
const items = await res.json();
return new Response(JSON.stringify(items), {
headers: { 'Content-Type': 'application/json' },
});
}
This handler filters items based on the category
query parameter and returns the filtered list as a JSON response.
5. Advanced POST Handlers
Handling and Parsing JSON Body Data
When dealing with POST requests, it's common to handle and parse JSON body data. The following example demonstrates how to read and process JSON data from a POST request:
// app/api/data/route.js
export async function POST(request) {
const body = await request.json();
console.log('Received data:', body);
return new Response('Data received successfully', { status: 200 });
}
In this handler, the JSON body data is parsed using request.json()
and logged to the console. The handler then responds with a success message.
Validating and Processing Form Data
Validation is crucial when handling form data in POST requests. Below is an example demonstrating how to validate and process form data:
// app/api/form/route.js
export async function POST(request) {
const body = await request.json();
const { name, email } = body;
if (!name || !email) {
return new Response('Missing fields', { status: 400 });
}
// Process the valid form data (e.g., save to database)
return new Response(`Form submitted successfully. Name: ${name}, Email: ${email}`, { status: 200 });
}
This example checks for the presence of name
and email
fields in the request body. If any field is missing, it responds with an error message. Otherwise, it processes the data and returns a success response.
Integrating with Databases (e.g., Prisma)
Next.js route handlers can be used to integrate with databases such as Prisma to perform CRUD operations. Here’s an example of handling a POST request to save data to a database using Prisma:
// app/api/users/route.js
import { PrismaClient } from '@prisma/client';
const prisma = new PrismaClient();
export async function POST(request) {
try {
const body = await request.json();
const { name, email } = body;
const newUser = await prisma.user.create({
data: {
name,
email,
},
});
return new Response(JSON.stringify(newUser), {
headers: { 'Content-Type': 'application/json' },
status: 201,
});
} catch (error) {
return new Response(`Error: ${error.message}`, { status: 500 });
} finally {
await prisma.$disconnect();
}
}
In this example, the POST handler reads the request body, validates it, and uses Prisma to create a new user record in the database. Proper error handling and resource cleanup are also demonstrated.