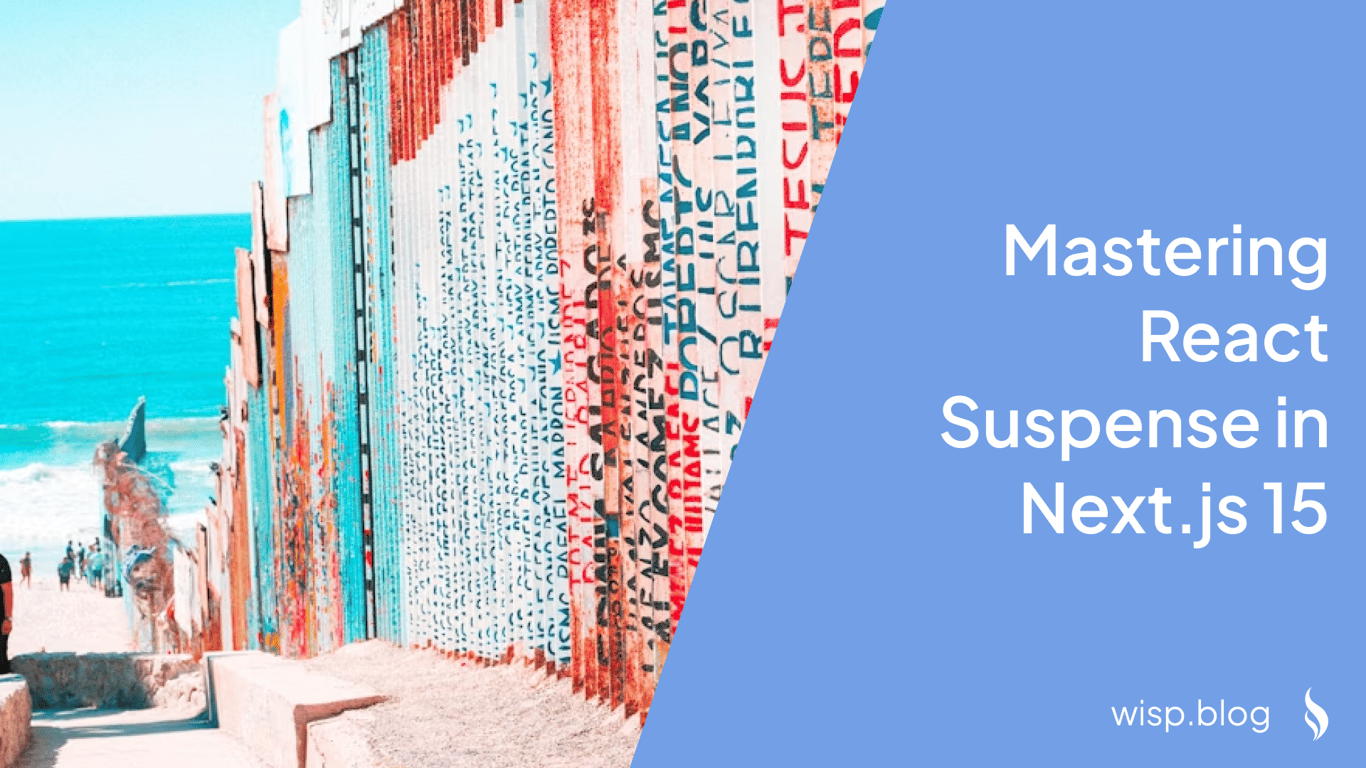
Are you tired of manually managing loading states across your React components? Finding yourself writing repetitive code with ternary operators for every async operation? React Suspense, especially when combined with Next.js 15, offers a more elegant solution to these common challenges.
Understanding React Suspense
React Suspense represents a paradigm shift in how we handle asynchronous operations in React applications. Instead of manually tracking loading states with boolean flags and conditional rendering, Suspense provides a declarative way to handle loading states while components wait for data or code to load.
As one developer noted on Reddit, "it's a lot easier and readable with less boilerplate and without setting loading states and props manually." This sentiment captures the core benefit of React Suspense - simplifying how we manage loading states in our applications.
Why Suspense Matters in Next.js 15
Next.js 15 brings exciting enhancements to React Suspense through its integration with Partial Prerendering (PPR). This combination offers several key advantages:
Unified Rendering Model: Next.js 15 implements a single React render tree where Suspense boundaries are respected throughout the rendering process, whether on the server or client.
Static Shell Generation: During build time, Next.js prerenders a static shell for each page, creating strategic "holes" for dynamic content defined by your Suspense boundaries.
Parallel Processing: When users visit your page, they immediately receive a fast static shell while the client and server work in parallel to populate dynamic content.
Here's a basic example of how Suspense works in Next.js 15:
import { Suspense } from 'react';
import { DynamicContent } from './DynamicContent';
export default function Page() {
return (
<main>
<h1>Welcome to My App</h1>
<Suspense fallback={<LoadingSpinner />}>
<DynamicContent />
</Suspense>
</main>
);
}
In this example, users see the heading and a loading spinner immediately, while the DynamicContent
component loads in the background. This approach eliminates the need for manual loading state management that traditionally looked like this:
// Old way - manual loading state management
const [isLoading, setIsLoading] = useState(true);
return (
<>
{isLoading ? <LoadingSpinner /> : <DynamicContent />}
</>
);
Setting Up React Suspense in Next.js 15
Let's walk through the process of implementing React Suspense in your Next.js 15 project.
1. Project Setup
First, ensure you're using the latest version of Next.js:
npm install next@canary react@rc react-dom@rc
Enable Partial Prerendering in your next.config.js
:
/** @type {import('next').NextConfig} */
const nextConfig = {
experimental: {
ppr: true
}
}
module default nextConfig
2. Implementing Suspense Boundaries
When implementing Suspense, it's crucial to consider where to place your Suspense boundaries. As highlighted by developers in the React community, one of the main advantages is that "the parent component doesn't need to understand how to figure out if everything in the child component is loaded and ready."
Here's a practical example of implementing nested Suspense boundaries:
import { Suspense } from 'react';
import { ErrorBoundary } from 'react-error-boundary';
export default function Dashboard() {
return (
<div className="dashboard">
{/* Critical UI elements load first */}
<header>
<h1>Dashboard</h1>
<Suspense fallback={<NavSkeleton />}>
<Navigation />
</Suspense>
</header>
<div className="dashboard-content">
{/* Wrap content that can load later */}
<ErrorBoundary fallback={<ErrorUI />}>
<Suspense fallback={<WidgetsSkeleton />}>
<DashboardWidgets />
</Suspense>
</ErrorBoundary>
{/* Separate boundary for less critical content */}
<Suspense fallback={<AnalyticsSkeleton />}>
<AnalyticsPanel />
</Suspense>
</div>
</div>
);
}
3. Data Fetching with Suspense
Next.js 15 makes data fetching with Suspense particularly elegant. Here's how you can implement it:
// app/api/fetch.ts
async function fetchData() {
const res = await fetch('https://api.example.com/data');
if (!res.ok) throw new Error('Failed to fetch data');
return res.json();
}
// app/components/DataComponent.tsx
async function DataComponent() {
const data = await fetchData();
return (
<div>
{data.map(item => (
<div key={item.id}>{item.name}</div>
))}
</div>
);
}
// app/page.tsx
export default function Page() {
return (
<Suspense fallback={<LoadingSkeleton />}>
<DataComponent />
</Suspense>
);
}
Advanced Patterns and Best Practices
Parallel Data Fetching
One of the most powerful features of Suspense in Next.js 15 is the ability to fetch data in parallel while showing appropriate loading states. Here's how you can implement this:
async function ParallelDataFetch() {
// These requests will load in parallel
const [userData, productData] = await Promise.all([
fetch('/api/user').then(res => res.json()),
fetch('/api/products').then(res => res.json())
]);
return (
<div>
<UserProfile data={userData} />
<ProductList data={productData} />
</div>
);
}
export default function Page() {
return (
<div>
<h1>Dashboard</h1>
{/* Each Suspense boundary can load independently */}
<Suspense fallback={<UserProfileSkeleton />}>
<UserSection />
</Suspense>
<Suspense fallback={<ProductsSkeleton />}>
<ParallelDataFetch />
</Suspense>
</div>
);
}
Error Handling
Proper error handling is crucial when working with Suspense. Here's a robust error boundary implementation:
import { ErrorBoundary } from 'react-error-boundary';
function ErrorFallback({ error, resetErrorBoundary }) {
return (
<div role="alert">
<p>Something went wrong:</p>
<pre>{error.message}</pre>
<button onClick={resetErrorBoundary}>Try again</button>
</div>
);
}
export default function Page() {
return (
<ErrorBoundary
FallbackComponent={ErrorFallback}
onReset={() => {
// Reset application state here
}}
>
<Suspense fallback={<Loading />}>
<DataComponent />
</Suspense>
</ErrorBoundary>
);
}
Performance Optimization
To maximize the benefits of Suspense in Next.js 15, consider these performance optimization techniques:
Strategic Boundary Placement: Place Suspense boundaries around components that can load independently:
function HomePage() {
return (
<Layout>
{/* Critical content loads first */}
<Header />
{/* Less critical content can load later */}
<Suspense fallback={<ContentSkeleton />}>
<MainContent />
</Suspense>
{/* Lowest priority content */}
<Suspense fallback={<FooterSkeleton />}>
<Footer />
</Suspense>
</Layout>
);
}
Streaming with Suspense: Next.js 15 supports streaming, which works seamlessly with Suspense:
import { experimental_useFormStatus as useFormStatus } from 'react-dom';
function SubmitButton() {
const { pending } = useFormStatus();
return (
<button disabled={pending}>
{pending ? 'Submitting...' : 'Submit'}
</button>
);
}
Common Challenges and Solutions
1. Handling Race Conditions
When dealing with multiple async operations, race conditions can occur. Here's how to handle them effectively:
function useData(id) {
const [data, setData] = useState(null);
const latestId = useRef(id);
useEffect(() => {
latestId.current = id;
fetchData(id).then(result => {
// Only update if this is still the latest request
if (latestId.current === id) {
setData(result);
}
});
}, [id]);
return data;
}
2. Avoiding Waterfall Requests
To prevent sequential loading of data, use parallel data fetching patterns:
// Instead of this (waterfall):
const user = await fetchUser(id);
const posts = await fetchUserPosts(user.id);
// Do this (parallel):
const [user, posts] = await Promise.all([
fetchUser(id),
fetchUserPosts(id)
]);
Looking Ahead
React Suspense in Next.js 15 represents a significant step forward in how we handle asynchronous operations and loading states in React applications. As noted by the community, "This leads to much more readable code and probably better UI."
The integration with Partial Prerendering in Next.js 15 further enhances these capabilities, providing a powerful foundation for building fast, responsive applications with clean, maintainable code.
Remember these key takeaways:
Suspense eliminates the need for manual loading state management
Strategic placement of Suspense boundaries can significantly improve user experience
Proper error handling is crucial for robust applications
Parallel data fetching can prevent loading waterfalls
Next.js 15's PPR integration makes Suspense even more powerful
As you implement React Suspense in your Next.js 15 applications, focus on creating intuitive loading experiences that enhance rather than detract from your user interface. The future of React development is here, and it's more elegant and efficient than ever.