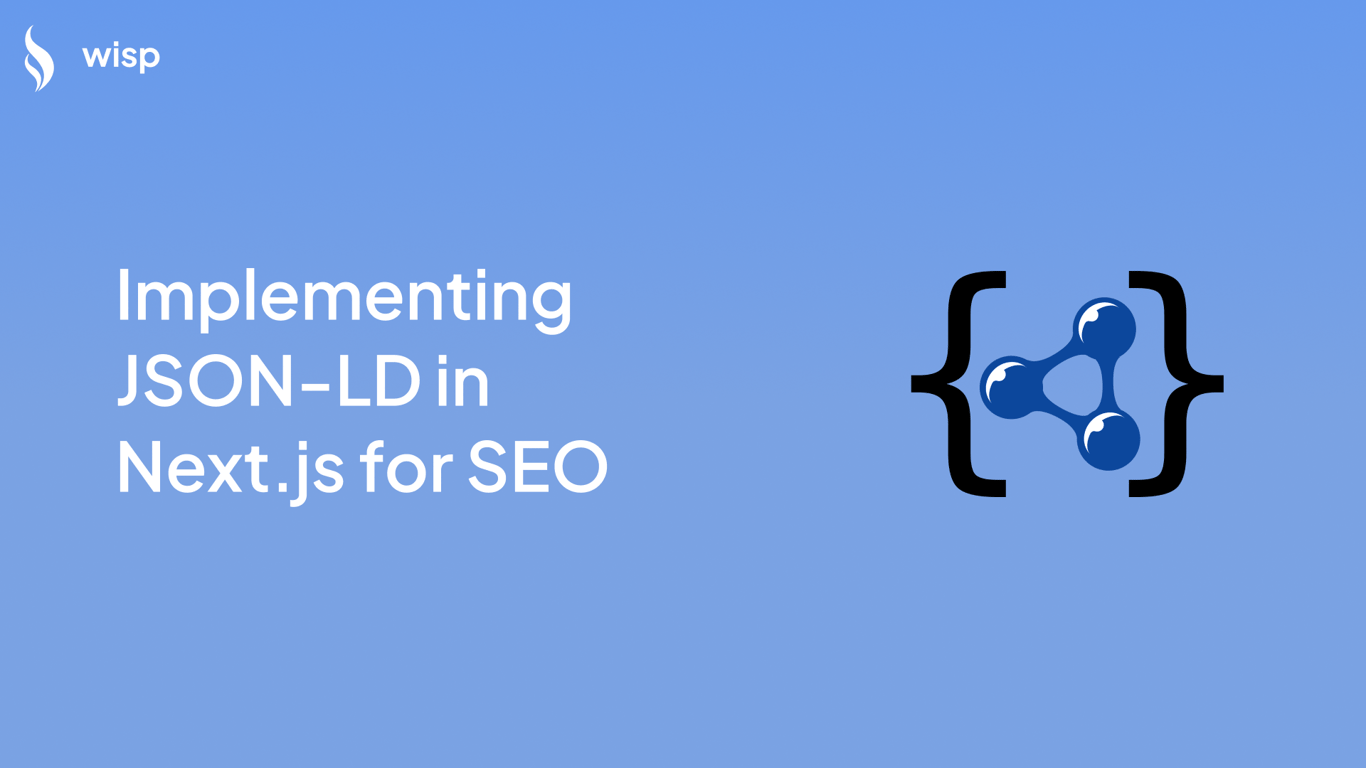
Are you struggling to implement JSON-LD structured data in your Next.js application? You're not alone. Many developers find themselves puzzled when their carefully crafted JSON-LD scripts don't work as expected in the head section, or worse, show up as duplicates in the page inspector.
"Adding it in the Head didn't work for me. I had to add it in the body instead," shares a frustrated developer on Reddit. This common pain point highlights the nuanced challenges of implementing structured data in Next.js applications.
But don't worry – you're about to discover exactly how to implement JSON-LD correctly in your Next.js project, avoiding the common pitfalls that trip up even experienced developers.
Why JSON-LD Matters for Your Next.js Application
Before diving into the implementation details, let's understand why JSON-LD is crucial for your website's SEO success.
JSON-LD (JavaScript Object Notation for Linked Data) is a lightweight format that helps search engines better understand your website's content. Unlike traditional meta tags, JSON-LD provides rich, structured data that can significantly improve your site's visibility in search results.
Here's why it's particularly important:
Enhanced Search Results: Your content can appear as rich snippets in search results, potentially increasing click-through rates by up to 30%
Better Content Understanding: Search engines can better comprehend your content's context and relevance
Future-Proof SEO: As search algorithms become more AI-driven, structured data becomes increasingly important for visibility
Common JSON-LD Implementation Challenges in Next.js
Many developers encounter several hurdles when implementing JSON-LD in Next.js:
Head Section Issues: Despite what traditional web development practices suggest, adding JSON-LD to the head section in Next.js often doesn't work as expected
Duplicate Entries: Developers frequently report seeing duplicate JSON-LD scripts when inspecting their pages
Documentation Gaps: The official Next.js documentation doesn't fully address common implementation scenarios
These challenges have led to numerous discussions in the developer community, with many seeking clearer guidance on proper implementation methods.
Step-by-Step Implementation Guide
Let's walk through the process of correctly implementing JSON-LD in your Next.js application.
1. Setting Up Your Environment
First, ensure you're using the latest version of Next.js (recommended v14.1 or later). You'll also need to install the required package for type support:
npm install schema-dts
# or
yarn add schema-dts
# or
pnpm install schema-dts
2. Creating Your JSON-LD Component
Instead of placing your JSON-LD in the head section (which can cause issues), create a component that will render the structured data in the body:
export default async function Page({ params }) {
const product = await getProduct(params.id);
const jsonLd = {
'@context': 'https://schema.org',
'@type': 'Product',
name: product.name,
image: product.image,
description: product.description,
};
return (
<section>
<script
type="application/ld+json"
dangerouslySetInnerHTML={{ __html: JSON.stringify(jsonLd) }}
/>
{/* Your page content */}
</section>
);
}
3. Implementing Different Schema Types
Different types of content require different schema structures. Here are some common examples:
For Blog Posts:const blogPostSchema = {
'@context': 'https://schema.org',
'@type': 'BlogPosting',
headline: 'Your Blog Title',
datePublished: '2024-02-20',
author: {
'@type': 'Person',
name: 'Author Name'
}
};
For E-commerce Products:const productSchema = {
'@context': 'https://schema.org',
'@type': 'Product',
name: 'Product Name',
description: 'Product Description',
offers: {
'@type': 'Offer',
price: '19.99',
priceCurrency: 'USD'
}
};
Best Practices and Common Pitfalls
Validation and Testing
Before deploying your JSON-LD implementation, it's crucial to validate your structured data:
Use Google's Rich Results Test:
Enter your URL or paste your code directly
Review any errors or warnings
Monitor in Google Search Console:
Regularly check the "Enhancements" section
Look for any structured data errors
Track the performance of your rich results
Avoiding Common Mistakes
Duplicate Entries If you're seeing duplicate JSON-LD entries in your page inspector, check for:
Multiple rendering of the same component
JSON-LD being added in both server and client components
Incorrect placement in layout files
Invalid Schema Structure Ensure your schema follows the official Schema.org vocabulary:
// Incorrect
const invalidSchema = {
'@context': 'https://schema.org',
'@type': 'Product',
productName: 'Example Product' // Wrong property name
};
// Correct
const validSchema = {
'@context': 'https://schema.org',
'@type': 'Product',
name: 'Example Product' // Correct property name
};
Missing Required Properties Each schema type has required properties. For example, a Product schema must include:
name
image
description
offers (if you're selling the product)
Performance Optimization
While implementing JSON-LD, keep these performance considerations in mind:
Minimize Data Size:
// Only include necessary properties
const optimizedSchema = {
'@context': 'https://schema.org',
'@type': 'Product',
name: product.name,
description: product.description
// Avoid including unnecessary metadata
};
Use Server Components:
// pages/products/[id].js
export default async function ProductPage({ params }) {
// Fetch product data server-side
const product = await getProduct(params.id);
return (
<ProductComponent product={product} />
);
}
Advanced Implementation Techniques
Dynamic Schema Generation
For larger applications, consider creating a schema generator utility:
// utils/schemaGenerator.js
export const generateSchema = (type, data) => {
switch (type) {
case 'product':
return {
'@context': 'https://schema.org',
'@type': 'Product',
name: data.name,
description: data.description,
// ... other properties
};
case 'article':
return {
'@context': 'https://schema.org',
'@type': 'Article',
headline: data.title,
author: {
'@type': 'Person',
name: data.author
},
// ... other properties
};
default:
return null;
}
};
Integration with Content Management Systems
When working with a CMS, automate your JSON-LD generation:
// pages/[slug].js
export default function ContentPage({ pageData }) {
const schemaData = {
'@context': 'https://schema.org',
'@type': pageData.type,
name: pageData.title,
description: pageData.description,
datePublished: pageData.publishDate,
author: {
'@type': 'Person',
name: pageData.author
}
};
return (
<>
<script
type="application/ld+json"
dangerouslySetInnerHTML={{ __html: JSON.stringify(schemaData) }}
/>
{/* Page content */}
</>
);
}
Conclusion
Implementing JSON-LD in Next.js doesn't have to be a source of frustration. By following the best practices outlined in this guide and avoiding common pitfalls, you can ensure your structured data implementation is both effective and maintainable.
Remember these key takeaways:
Place JSON-LD scripts in the body section rather than the head
Always validate your structured data before deployment
Monitor your implementation through Google Search Console
Keep your schema structure clean and optimized
For more detailed information, refer to the official Next.js documentation and Schema.org guidelines.
By implementing JSON-LD correctly, you're not just improving your website's SEO – you're making your content more accessible and understandable for search engines and users alike.