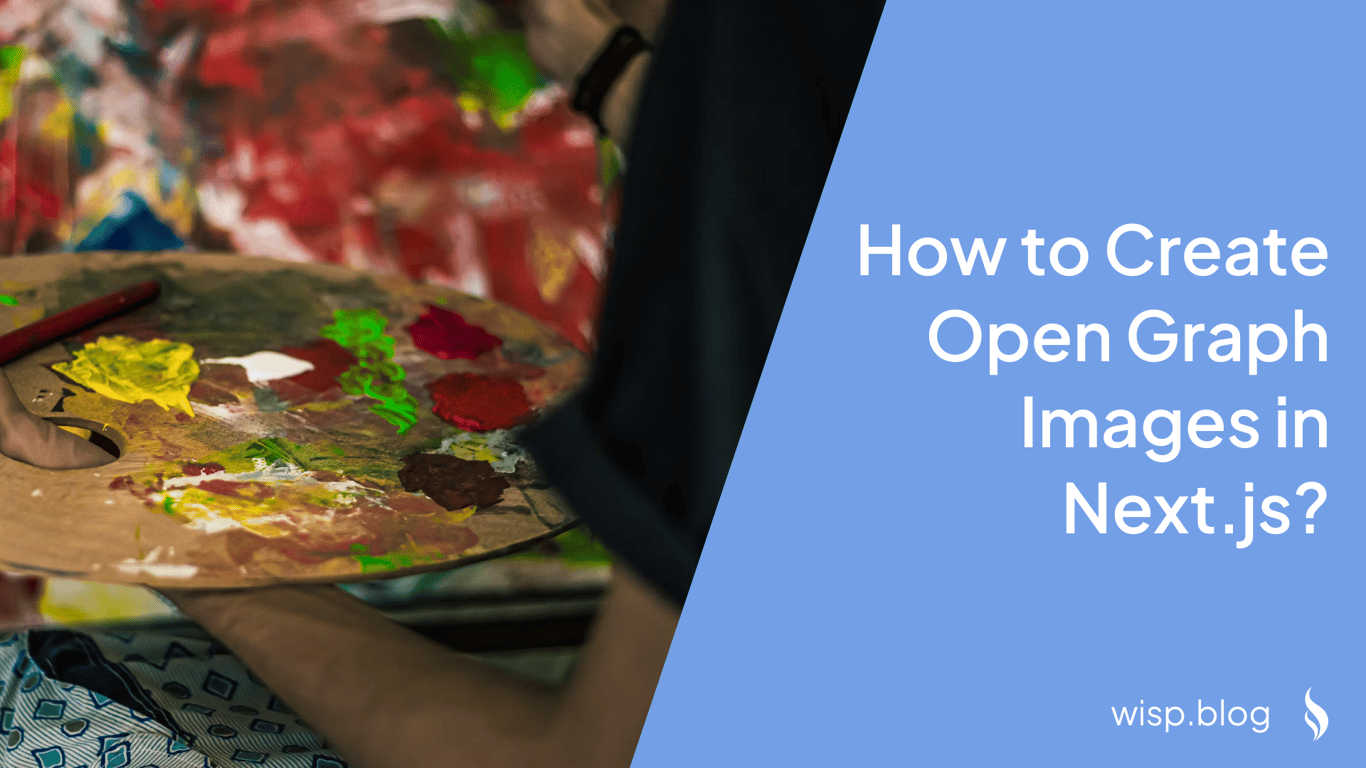
Are you struggling to get your Open Graph images working properly in your Next.js application? You're not alone. Many developers face challenges with images not updating after deployment, caching issues, and difficulties with dynamic content integration. In this comprehensive guide, we'll walk through everything you need to know about creating Open Graph images in Next.js, and introduce a tool that can make this process much simpler.
Why Open Graph Images Matter
Open Graph images are the visual previews that appear when your content is shared on social media platforms like Twitter, Facebook, and LinkedIn. They're crucial for:
Increasing click-through rates on social media shares
Making your content stand out in crowded feeds
Maintaining brand consistency across platforms
Providing visual context for your shared links
Common Challenges with Open Graph Images
Before diving into the solutions, let's address some common pain points that developers frequently encounter:
Deployment Updates Not Reflecting: Many developers report frustration with Open Graph images not updating after deploying their Next.js applications on Vercel.
Caching Issues: Vercel's caching mechanism can sometimes prevent updates from appearing, leading to outdated images being displayed.
Dynamic Content Integration: Incorporating user-generated content into Open Graph images can be particularly challenging, especially when dealing with real-time updates.
Edge Runtime Limitations: There are valid concerns about performance and capabilities when using Edge runtime for generating dynamic images.
Getting Started with Open Graph Images in Next.js
Next.js provides several approaches to implement Open Graph images. We'll explore both static and dynamic methods, starting with the simplest approach and progressing to more advanced implementations.
Method 1: Static File Convention
The most straightforward way to add Open Graph images is using Next.js's file convention system. Here's how:
Create an image file named either
opengraph-image
ortwitter-image
in your route segmentUse supported formats:
.jpg
,.jpeg
,.png
, or.gif
For example, in your app directory:
app/
layout.tsx
opengraph-image.png # This will be used as the default OG image
page.tsx
about/
opengraph-image.jpg # This will be used for the /about route
This method automatically generates the necessary meta tags:
<meta property="og:image" content="<generated-image-url>" />
<meta name="twitter:image" content="<generated-image-url>" />
Method 2: Dynamic Generation with @vercel/og
For more flexibility, especially when dealing with dynamic content, you can use the @vercel/og
package to generate Open Graph images programmatically.
First, install the package:
npm install @vercel/og
# or
yarn add @vercel/og
Create a new API route for generating images. Create a file at
app/api/og/route.tsx
:
import { ImageResponse } from 'next/og'
export const runtime = 'edge'
export async function GET() {
return new ImageResponse(
(
<div
style={{
fontSize: 128,
background: 'white',
width: '100%',
height: '100%',
display: 'flex',
alignItems: 'center',
justifyContent: 'center',
}}
>
Hello World!
</div>
),
{
width: 1200,
height: 630,
},
)
}
Update your metadata configuration in your page or layout file:
import { Metadata } from 'next'
export const metadata: Metadata = {
openGraph: {
title: 'Your Title',
description: 'Your Description',
images: [{
url: '/api/og',
width: 1200,
height: 630,
}],
},
}
Method 3: Dynamic Images with User Content
For cases where you need to include dynamic content in your Open Graph images, you can modify the API route to accept parameters:
import { ImageResponse } from 'next/og'
export const runtime = 'edge'
export async function GET(request: Request) {
const { searchParams } = new URL(request.url)
const title = searchParams.get('title')
return new ImageResponse(
(
<div
style={{
fontSize: 64,
background: 'white',
width: '100%',
height: '100%',
display: 'flex',
flexDirection: 'column',
alignItems: 'center',
justifyContent: 'center',
padding: '40px',
}}
>
<h1 style={{ textAlign: 'center' }}>{title}</h1>
</div>
),
{
width: 1200,
height: 630,
},
)
}
Then update your metadata to include the dynamic parameters:
export async function generateMetadata({ params }): Promise<Metadata> {
const post = await getPost(params.slug)
return {
openGraph: {
title: post.title,
description: post.description,
images: [{
url: `/api/og?title=${encodeURIComponent(post.title)}`,
width: 1200,
height: 630,
}],
},
}
}
Troubleshooting Common Issues
1. Images Not Updating After Deployment
If you're experiencing issues with images not updating after deployment on Vercel, try these solutions:
Clear the Vercel cache:
Go to your project settings in the Vercel dashboard
Navigate to the "Cache" section
Click "Clear Cache"
Convert JPEG images to PNG:
Some developers have reported that replacing JPEG images with PNGs can force an update
Update your Next.js version:
Newer versions often include fixes for Open Graph-related issues
2. Caching Issues
To address caching problems:
Add cache control headers to your API route:
export async function GET() {
return new ImageResponse(
// ... your component
{
headers: {
'Cache-Control': 'public, max-age=3600, must-revalidate',
},
}
)
}
Use unique URLs for different image versions by adding a timestamp or version parameter:
`/api/og?title=${title}&v=${Date.now()}`
3. Edge Runtime Limitations
When using Edge runtime for dynamic image generation, keep in mind:
File size limitations
Memory constraints
Processing time restrictions
To optimize performance:
Minimize the complexity of your image generation
Cache generated images when possible
Consider using static images for non-dynamic content
Simplifying Open Graph Image Generation with Wisp
While the above methods are powerful, they can be complex to implement and maintain. This is where Wisp's OpenGraph Image Generator comes in handy. This tool offers a simpler alternative for creating professional Open Graph images without the technical overhead.
Key Features of Wisp's Tool:
No Account Required: Generate images instantly without signing up
Customization Options:
Title customization
Label addition
Brand text integration
Logo upload capability
Permanent URLs: Each generated image gets a permanent link for easy integration
High-Quality Templates: Professional designs ready for immediate use
Integration with Next.js
To use Wisp's generated images in your Next.js application:
Generate your image using the tool
Copy the permanent URL
Add it to your metadata configuration:
export const metadata: Metadata = {
openGraph: {
images: [{
url: 'your-wisp-generated-image-url',
width: 1200,
height: 630,
}],
},
}
Best Practices and Tips
Image Dimensions: Stick to the recommended 1200x630 pixels for optimal display across platforms
Testing: Always test your Open Graph images using:
Performance: Consider implementing:
Caching strategies for dynamic images
CDN distribution for static images
Lazy loading for optimal page performance
Conclusion
Creating effective Open Graph images in Next.js doesn't have to be complicated. While the framework provides powerful built-in capabilities for both static and dynamic image generation, tools like Wisp's OpenGraph Image Generator can significantly simplify the process.
Whether you choose to implement a custom solution or use a tool like Wisp, remember to:
Test your images across different platforms
Monitor performance and caching behavior
Keep your implementation as simple as possible while meeting your needs
By following this guide and choosing the right approach for your use case, you can ensure your content stands out on social media with professional, consistently rendered Open Graph images.