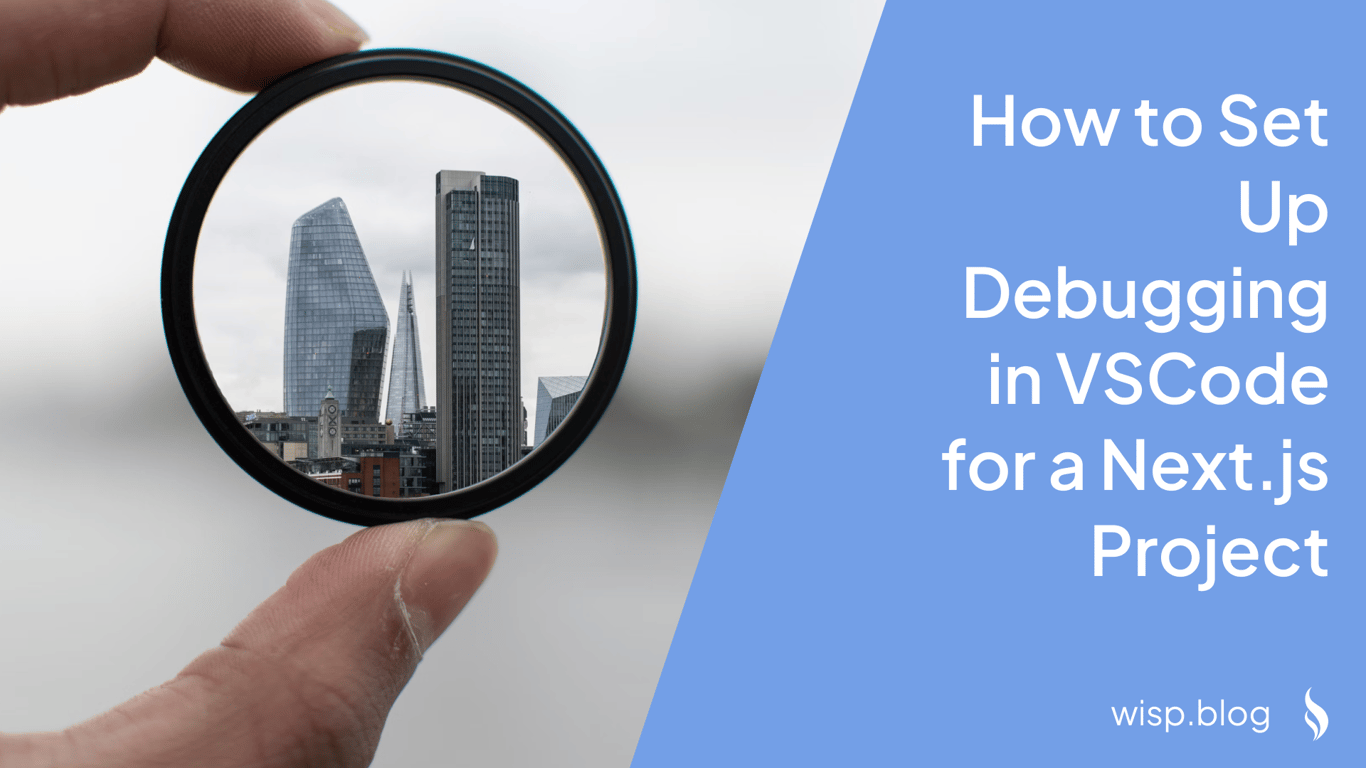
Are you struggling to set up debugging for your Next.js project in Visual Studio Code? You're not alone. Many developers spend hours trying to configure their debugging environment correctly, often reverting to console.log statements out of frustration. But there's a better way.
This comprehensive guide will walk you through the process of setting up debugging in VSCode for your Next.js project, helping you avoid common pitfalls and save precious development time.
Why Proper Debugging Setup Matters
While console.log
might seem like a quick solution, proper debugging setup allows you to:
Inspect variables and state in real-time
Set breakpoints to pause code execution at specific points
Step through your code line by line
Examine the call stack and scope variables
Debug both client-side and server-side code effectively
Prerequisites
Before we begin, ensure you have:
Visual Studio Code installed
A Next.js project set up
Debugger for Chrome extension installed in VSCode
Setting Up the Debug Configuration
1. Create the launch.json File
First, we need to create a debug configuration file:
Open your Next.js project in VSCode
Press
Ctrl+Shift+D
(Windows/Linux) orCmd+Shift+D
(Mac) to open the Run and Debug viewClick on "create a launch.json file" and select "Node.js"
Here's a working configuration that you can copy directly into your launch.json
:
{
"version": "0.2.0",
"configurations": [
{
"name": "Next.js: debug server-side",
"type": "node",
"request": "launch",
"program": "${workspaceFolder}/node_modules/.bin/next",
"args": ["dev"],
"console": "integratedTerminal",
"skipFiles": ["<node_internals>/**"],
"outFiles": ["${workspaceFolder}/.next/**/*.js"],
"sourceMaps": true,
"trace": true
}
]
}
This configuration is based on the official Next.js repository's setup and has been tested to work reliably across different Next.js versions.
2. Configure Environment Variables
If you're working across different platforms (especially Windows), you might need to add cross-env to handle environment variables consistently:
Install cross-env:
npm install --save-dev cross-env
Update your package.json scripts:
{
"scripts": {
"dev": "cross-env NODE_OPTIONS='--inspect' next dev"
}
}
Common Debugging Issues and Solutions
1. Breakpoints Not Working
A frequent issue, especially after upgrading to Next.js 14, is breakpoints not functioning properly. Here's how to fix it:
Disable Turbo Mode: If you're using Turbopack and breakpoints aren't working, try disabling it temporarily:
next dev --no-turbo
Update Pattern Matching: For Next.js 14+, update your launch.json pattern to match the new server start format:
{
"serverReadyAction": {
"pattern": "Local:\\s*(https?://.+)"
}
}
2. Module Not Found Errors
If you encounter errors like "Cannot find module", ensure that:
Your project dependencies are properly installed
The paths in your launch.json are correct for your operating system
Node.js is properly installed and accessible
3. Client-Side Debugging
For comprehensive debugging that includes both server and client-side code:
Add a Chrome configuration to your launch.json:
{
"name": "Next.js: debug client-side",
"type": "chrome",
"request": "launch",
"url": "http://localhost:3000",
"webRoot": "${workspaceFolder}",
"sourceMapPathOverrides": {
"webpack://_N_E/*": "${webRoot}/*"
}
}
Using the Debugger Effectively
Now that your debugger is set up, here's how to make the most of it:
1. Setting Breakpoints
Click the left margin of your code editor to set a breakpoint
Use conditional breakpoints by right-clicking the breakpoint and setting conditions
Use the Debug toolbar to control execution:
Continue (F5)
Step Over (F10)
Step Into (F11)
Step Out (Shift+F11)
2. Inspecting Variables
Use the Variables panel to inspect local and global variables
Add variables to the Watch panel for continuous monitoring
Use the Debug Console to evaluate expressions
3. Error Handling
For better error tracking, create a custom error page:
// pages/_error.js
function Error({ statusCode }) {
return (
<p>
{statusCode
? `An error ${statusCode} occurred on server`
: 'An error occurred on client'}
</p>
);
}
Error.getInitialProps = ({ res, err }) => {
const statusCode = res ? res.statusCode : err ? err.statusCode : 404;
return { statusCode };
};
export default Error;
Additional Tips and Best Practices
Use Source Maps: Ensure source maps are enabled for better debugging experience:
// next.config.js
module.exports = {
productionBrowserSourceMaps: true
}
React Developer Tools: Install the React Developer Tools browser extension for component debugging
Regular Testing: Test your debugging setup regularly, especially after Next.js updates
Conclusion
While setting up debugging in VSCode for Next.js projects can be challenging, following this guide should help you establish a reliable debugging environment. Remember that proper debugging setup is an investment that pays off in faster development and fewer frustrating debugging sessions.
For more information and updates, refer to:
Next.js GitHub repository for the latest configurations