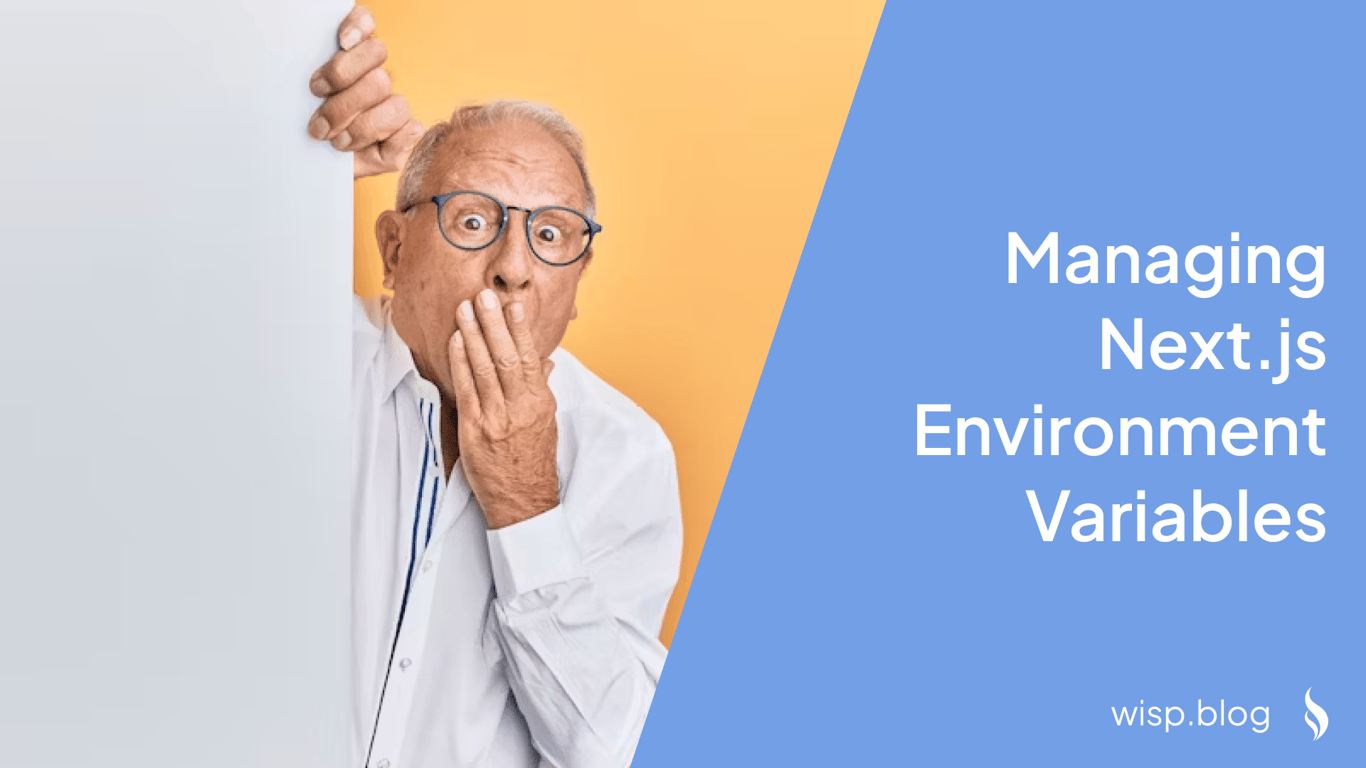
Environment variables play a crucial role in configuring and managing the operational settings of an application without hardcoding sensitive information into the codebase. When developing Next.js applications, effectively managing environment variables is essential for ensuring that your app runs smoothly across different environments, including local development, previews, and production deployments. This article explores how to manage environment variables in Next.js applications hosted on Vercel, providing comprehensive guidance from setup to deployment.
Understanding Environment Variables
What Are Environment Variables?
Environment variables are key-value pairs that define various settings or configurations for an application’s operating environment. They help segregate operational settings from application code, enhancing security and flexibility. For example, environment variables often store sensitive data like API keys, database credentials, or configuration URLs.
Types of Environment Variables
Local: For development settings, typically stored in
.env.local
.Preview: For deployment previews, configured in Vercel’s project settings.
Production: For production deployments, also configured in Vercel’s project settings.
Setting Up Environment Variables in Next.js
Creating Environment Files
Next.js supports multiple environment files for different stages of development. These files should be placed in the root directory of your project:
.env.local
: Used for local development settings and should not be committed to version control..env.development
: Loaded when runningnext dev
..env.production
: Loaded duringnext build
ornext start
.
Example of .env.local
:
DATABASE_USER=janedoe
DATABASE_PASSWORD=securepassword123
NEXT_PUBLIC_API_URL=http://localhost:3000/api
Accessing Environment Variables in Next.js
Environment variables in Next.js can be accessed via process.env
. Any variable defined in the .env
files will be available in process.env
.
All environment variables are accessible on the server-side code.
export async function getStaticProps() {
const dbUser = process.env.DATABASE_USER;
const dbPassword = process.env.DATABASE_PASSWORD;
return { props: { dbUser, dbPassword } };
}
Client-Side AccessVariables that need to be accessed on the client-side must be prefixed with NEXT_PUBLIC_
. This ensures that sensitive information is not exposed to the client.
const apiUrl = process.env.NEXT_PUBLIC_API_URL;
fetch(apiUrl + '/data')
.then(response => response.json())
.then(data => console.log(data));
Best Practices for Managing Environment Variables
Security Tips
Do not commit
.env.local
and other sensitive environment files to version control. Add these files to.gitignore
to prevent accidental exposure.Use descriptive names: Ensure the names of your environment variables are descriptive and use uppercase letters with underscores (e.g.,
DATABASE_USER
).Public Variables: Prefix variables that need to be exposed to the client with
NEXT_PUBLIC_
.
Example of .gitignore
entry:
# .gitignore
.env*
!*.example
Configuring Environment Variables on Vercel
Steps to Configure Environment Variables
Navigate to the Environment Variables page in Vercel’s Project Settings.
Click on “Add New” and enter the name and value for the environment variable.
Select the environments (Production, Preview, Development) that this variable should apply to.
Click Save.
Vercel CLI Commands
Sync Local Variables: Use
vercel env pull .env.local
to download development environment variables to.env.local
.
Environment Variable Limits
Total Size Limit: Vercel allows up to 64KB of environment variables per deployment.
Edge Functions and Edge Middleware: Limited to 5KB per variable.
Using Environment Variables in Different Next.js Features
Static Generation
Environment variables must be set before running the build command for them to be available during static generation.
export async function getStaticProps() {
const siteTitle = process.env.NEXT_PUBLIC_SITE_TITLE || 'Default Site Title';
return { props: { siteTitle } };
}
Server-Side Rendering
Environment variables are read at runtime, suitable for dynamic content.
export async function getServerSideProps() {
const apiKey = process.env.API_KEY;
return { props: { apiKey } };
}
Advanced Usage and Troubleshooting Tips
Dynamic Configurations with next.config.js
Modify or add environment variables programmatically based on custom logic.
module.exports = {
env: {
CUSTOM_VAR: process.env.NODE_ENV === 'production' ? 'valueProd' : 'valueDev'
},
webpack(config, { isServer }) {
if (!isServer) {
config.plugins.push(new webpack.DefinePlugin({
'process.env.CUSTOM_VAR': JSON.stringify(process.env.CUSTOM_VAR),
}));
}
return config;
}
};
Common Pitfalls and Solutions
Variable Naming: Ensure variables are named correctly and consistently.
Sync Issues: Use
vercel env pull
to ensure local variables are up to date.Size Limits: Monitor the size of environment variables to stay within Vercel’s limits.
Conclusion
Effectively managing environment variables is crucial for the security and efficiency of your Next.js applications. By following best practices and properly configuring variables for different environments, you can ensure smooth and secure deployments on Vercel.