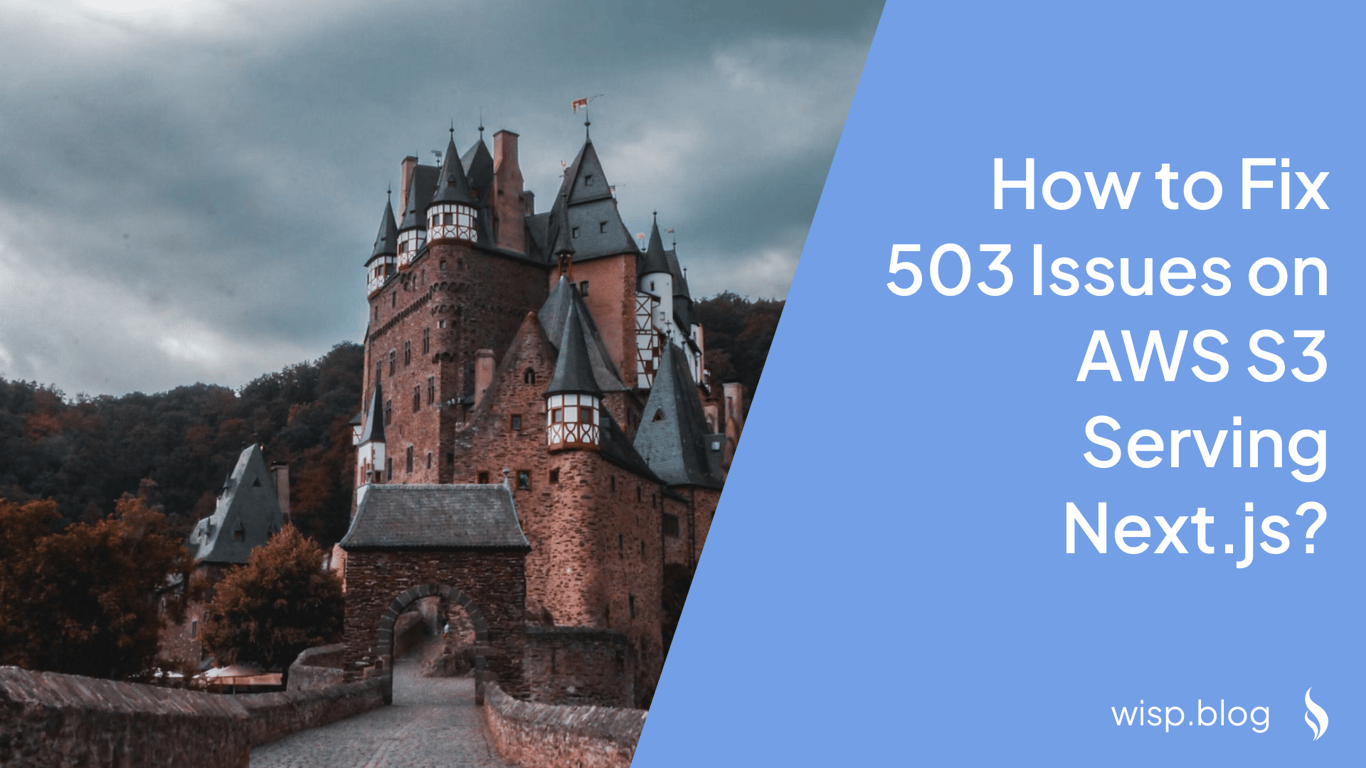
You've deployed your Next.js application using AWS S3 and CloudFront, expecting smooth sailing. But suddenly, your team reports frustrating experiences - blank pages appearing during navigation, especially after users complete external actions like Google sign-in or Stripe payments. Your CloudFront logs are filling up with 503 errors, and you're left wondering what went wrong.
These issues can be particularly maddening because they often appear inconsistently - working perfectly for some team members while others encounter blank pages and errors. If this sounds familiar, you're not alone. Many developers face similar challenges when serving Next.js applications through AWS infrastructure.
Understanding the Root Causes
Before diving into solutions, it's crucial to understand why these 503 errors occur. A 503 Service Unavailable error indicates that the server is temporarily unable to handle the request. In the context of AWS S3 and Next.js, several factors can trigger these errors:
1. Request Rate Limitations
AWS S3 has specific request rate limits that, when exceeded, can trigger 503 errors:
Up to 3,500 PUT/COPY/POST/DELETE requests per second
Up to 5,500 GET/HEAD requests per second
For AWS free tier users, there's a limit of 2,000 PUT requests total
When your application exceeds these limits, AWS responds with a 503 "Slow Down" error to protect its infrastructure.
2. Lambda@Edge Limitations
If you're using Lambda@Edge functions with CloudFront (common in Next.js deployments), you might encounter the dreaded 'LambdaLimitExceeded' error. This typically appears in your CloudFront logs as:
x-edge-detailed-result-type: LambdaLimitExceeded
This error occurs when the Lambda@Edge function hits its concurrent execution limits or times out.
3. Configuration Mismatches
Common configuration issues that can lead to 503 errors include:
Incorrect S3 bucket policies preventing proper access
Misconfigured CORS settings blocking necessary requests
Improper CloudFront cache settings causing request conflicts
Insufficient IAM permissions for Lambda@Edge or S3 access
4. External Service Integration Issues
The problem often becomes more pronounced when integrating with external services. Users frequently report increased occurrences of 503 errors and blank pages when:
Returning from OAuth authentication flows (like Google Sign-In)
Completing payment processes (such as Stripe checkout)
Handling file uploads through pre-signed URLs
Implementing the Solutions
Let's dive into practical solutions to resolve these 503 issues and improve your Next.js application's reliability on AWS.
1. Optimize Request Patterns
To avoid hitting S3's request limits:
// Example of implementing exponential backoff
const fetchWithRetry = async (url, options = {}, maxRetries = 3) => {
for (let i = 0; i < maxRetries; i++) {
try {
const response = await fetch(url, options);
if (response.status !== 503) return response;
// Wait with exponential backoff
await new Promise(resolve =>
setTimeout(resolve, Math.pow(2, i) * 1000)
);
} catch (error) {
if (i === maxRetries - 1) throw error;
}
}
};
Additionally:
Implement request batching for multiple small files
Use CloudFront caching effectively to reduce direct S3 requests
Consider implementing a queue system for large upload operations
2. Configure S3 Bucket Properly
Ensure your S3 bucket has the correct permissions and CORS configuration:
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "PublicReadGetObject",
"Effect": "Allow",
"Principal": "*",
"Action": [
"s3:GetObject"
],
"Resource": "arn:aws:s3:::your-bucket-name/*"
}
]
}
For CORS configuration:
[
{
"AllowedHeaders": [
"*"
],
"AllowedMethods": [
"GET",
"HEAD"
],
"AllowedOrigins": [
"https://your-domain.com"
],
"ExposeHeaders": [
"ETag"
],
"MaxAgeSeconds": 3600
}
]
3. Optimize CloudFront Configuration
To minimize 503 errors and improve performance:
Adjust cache behaviors:
// Next.js API route example with cache control
export default function handler(req, res) {
res.setHeader('Cache-Control', 's-maxage=60, stale-while-revalidate');
// Your API logic here
}
Configure CloudFront to handle errors gracefully:
Set up custom error responses
Implement fallback content for 503 errors
Use origin failover for critical content
4. Implement Robust Error Handling
Add comprehensive error handling in your Next.js application:
// pages/_app.js
import { useEffect } from 'react';
import { useRouter } from 'next/router';
function MyApp({ Component, pageProps }) {
const router = useRouter();
useEffect(() => {
const handleRouteChangeError = (err, url) => {
if (err.cancelled) return;
// Handle 503 errors during navigation
if (err.status === 503) {
console.error(`Navigation to ${url} failed: Service Unavailable`);
// Implement retry logic or show user-friendly message
}
};
router.events.on('routeChangeError', handleRouteChangeError);
return () => {
router.events.off('routeChangeError', handleRouteChangeError);
};
}, [router]);
return <Component {...pageProps} />;
}
export default MyApp;
5. Monitor and Alert
Set up comprehensive monitoring to catch issues before they affect users:
Configure CloudWatch Alarms:
aws cloudwatch put-metric-alarm \
--alarm-name "S3-503-Errors" \
--alarm-description "Alert on high 503 error rate" \
--metric-name "5xxErrorRate" \
--namespace "AWS/CloudFront" \
--statistic "Average" \
--period 300 \
--threshold 5 \
--comparison-operator "GreaterThanThreshold" \
--evaluation-periods 2 \
--alarm-actions "arn:aws:sns:region:account-id:topic-name"
Implement application-level logging:
// utils/logger.js
const logError = async (error, context) => {
console.error(`[${context}] Error:`, error);
// Send to your logging service
await fetch('/api/log', {
method: 'POST',
body: JSON.stringify({
error: error.message,
context,
timestamp: new Date().toISOString()
})
});
};
6. Optimize for External Service Integration
When dealing with external service redirects:
// pages/api/auth/[...nextauth].js
import NextAuth from 'next-auth';
export default NextAuth({
callbacks: {
async redirect({ url, baseUrl }) {
// Ensure proper handling of redirects
if (url.startsWith(baseUrl)) return url;
if (url.startsWith('/')) return new URL(url, baseUrl).toString();
return baseUrl;
}
},
// Other configuration...
});
Best Practices and Preventive Measures
To maintain a healthy Next.js application on AWS S3 and prevent 503 errors:
1. Regular Maintenance
Perform regular CloudFront cache invalidations when deploying updates
Monitor S3 request metrics to identify potential bottlenecks
Review and update IAM permissions as your application evolves
2. Performance Optimization
Implement proper caching strategies:
Use stale-while-revalidate for dynamic content
Set appropriate cache TTLs for static assets
Configure browser caching headers
Optimize asset delivery:
Use compression for text-based assets
Implement image optimization
Utilize CDN edge locations effectively
3. Scalability Considerations
Implement request rate limiting in your application
Use multiple S3 prefixes for better performance distribution
Consider implementing a queue system for heavy upload operations
Conclusion
503 errors in Next.js applications served through AWS S3 can be frustrating, but they're manageable with the right approach. By implementing proper error handling, monitoring, and optimization strategies, you can significantly reduce their occurrence and impact on your users.
Remember to:
Monitor your application's request patterns
Implement robust error handling
Configure your AWS services correctly
Maintain proper caching strategies
Stay within AWS service limits
These practices will help ensure a smoother experience for your users and easier maintenance for your team.
Additional Resources
By following these guidelines and implementing the solutions outlined above, you can maintain a robust Next.js application on AWS S3 while minimizing the occurrence of 503 errors and providing a better experience for your users.