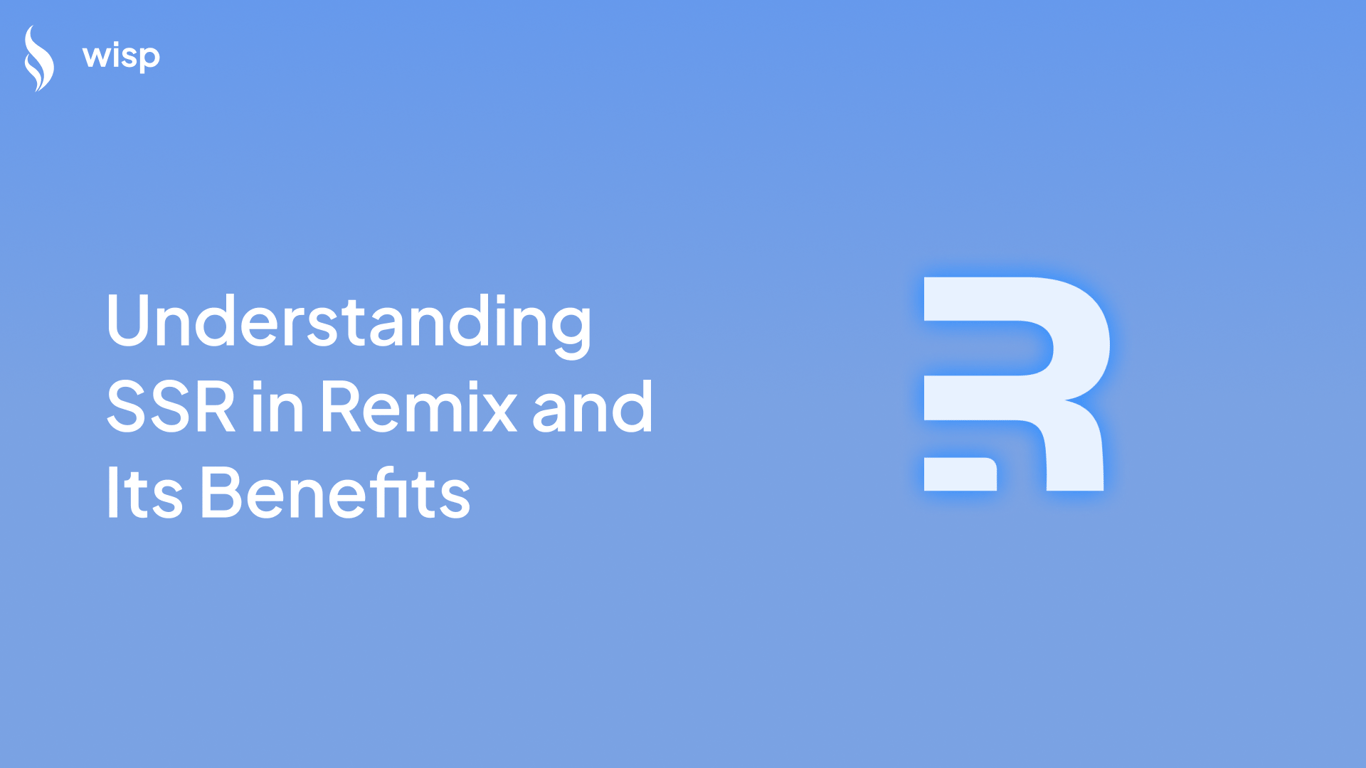
Server-Side Rendering (SSR) is a powerful technique in modern web development that helps to improve performance, SEO, and overall user experience. Remix, a full-stack web framework built on top of React, leverages SSR to deliver fast and seamless web applications. In this article, we will delve into the workings of SSR in Remix, its benefits, and how it compares to other frameworks like Next.js.
Understanding Remix
Key Features of Remix
Remix is designed to enhance both developer and user experiences through a variety of features and capabilities. Some of the key features include:
JavaScript and TypeScript Support: Remix is primarily written in JavaScript and supports TypeScript for type safety and better code quality.
Based on React: Remix utilizes the React library, making it familiar to developers who have experience with React.
Built-in Module Bundler: Remix includes a built-in module bundler (Remix and Vite), facilitating efficient bundling of JavaScript files.
Supports Static and Dynamic Pages: Remix supports both Static Site Generation (SSG) and Server-Side Rendering (SSR), making it versatile for various use cases.
Serverless Functions: Remix supports serverless functions, enabling on-demand execution without the need for server management.
Hot Reloading and Code Splitting: Instant preview of changes in the browser without refreshing, and splitting code into smaller chunks for improved performance.
Image Optimization and Critical CSS Extraction: Built-in optimization for images and critical CSS extraction to enhance page load times and performance.
Unique Concepts in Remix
Nested Routes: Remix supports nested routes that map different components and data dependencies to URL segments. This creates a hierarchy of route components for more efficient rendering and data handling.
Progressive Enhancement: Remix emphasizes the ability to function without JavaScript, ensuring essential tasks like form submissions are performed even if JavaScript is disabled.
Server/Client Model Philosophy: Remix focuses on optimizing data transfer over the network rather than just server efficiency, enhancing overall speed and performance.
Server-Side Rendering (SSR) in Remix
SSR Architecture in Remix
Remix's SSR architecture enhances performance by rendering HTML on the server and sending it to the client. Here are some key components:
Hydration and Initial Load Time: The
app/root.tsx
file controls the "hydrate" step, connecting static HTML with JavaScript to make it dynamic, ensuring fast initial load times.Routing and Layout Mechanisms: Nested routes and layouts avoid repetitive styles and optimize the structure. Remix maps portions of URLs to nested routes, enabling efficient data fetching and UI rendering.
Data Dependencies and Actions: Data fetching and operations such as form submissions are managed with server-side functions (
loader
andaction
). TheuseLoaderData
anduseActionData
hooks retrieve and manage data efficiently.State Management and Prefetching: Instead of relying heavily on client-side state management tools like Redux, Remix uses cookies to store data like user tokens, simplifying state management and improving transition efficiency.
Benefits of Using SSR
Faster Initial Page Load: SSR sends fully rendered HTML pages to the client, reducing the time required for the browser to download and process JavaScript before rendering content.
Improved SEO: Search engines can easily crawl and index SSR-rendered pages since the complete HTML content is available upfront.
Enhanced User Experience: Users receive a pre-rendered page, enabling faster content visibility and smoother navigation.
Cross-Platform Capabilities: Consistent rendering across various devices and platforms, minimizing discrepancies that can occur with client-side rendering.
Comparative Analysis: Remix vs. Other Frameworks (e.g., Next.js)
When choosing a framework for Server-Side Rendering (SSR), it’s important to understand how Remix compares to other popular frameworks like Next.js. Here’s a breakdown of the key differences and similarities.
Routing
Next.js: Utilizes file-based routing, where the file structure defines the routes. This makes it simple to organize and understand the routing structure. For instance, creating a file
pages/[id].js
automatically sets up a dynamic route.Remix: Uses code-based routing, which offers more granular control. Routes are explicitly defined, allowing for sophisticated route management and transitions. Dynamic routes in Remix might look like
routes/:id.js
, giving you the ability to handle complex navigation scenarios.
Data Management & Loading
Next.js: Provides built-in data fetching methods like
getStaticProps
for static site generation andgetServerSideProps
for server-side rendering. It also supports client-side fetching with tools like SWR or Apollo.Remix: Uses a flexible data fetching model through the
load()
function. It supports server-side data fetching directly within route modules, which can simplify the process and reduce the client-side code.
Styling
Next.js: Supports various styling methods including CSS Modules, Styled JSX, and CSS-in-JS libraries like styled-components or emotion.
Remix: Offers a custom approach to styling, supporting global CSS stylesheets and CSS-in-JS libraries such as Emotion and Linaria. This flexibility allows for optimized styling of components and routes.
Image Optimization
Next.js: Features the
next/image
component for automatic image optimization, providing responsive images and lazy loading out of the box.Remix: Uses tools like the
sharp
library for image processing, offering flexibility and customization in how images are optimized.
SEO Optimization
Next.js: Built-in SEO features like customizable meta tags, server-side rendering, and automatic code splitting enhance the search engine visibility of your pages.
Remix: Allows custom metadata tags and fully supports server-side rendering to boost SEO. The framework’s agnostic nature provides flexibility for integrating SEO strategies.
Error Handling
Next.js: Robust error handling capabilities with support for custom error pages and Error Boundary components to catch and handle errors gracefully.
Remix: Provides fine-grained error handlers that allow developers to customize error rendering and handling, offering greater control over error management.
Deployment and Community Support
Next.js: Seamless deployment with support for platforms like Vercel, Netlify, and AWS. It has a large and active community with extensive documentation and examples.
Remix: Supports deployment on various platforms including Heroku, AWS, and edge providers like CloudFlare Workers. Remix also has growing community support with dedicated forums and active core team involvement.
Advantages of Using Remix for SSR
Simplified Development Process: Remix allows developers to write both frontend and backend code in the same file, making it easier to manage and debug. The co-location of data-fetching logic with components reduces the cognitive load on developers.
Enhanced Performance: By rendering HTML on the server and delivering it to the client, Remix improves initial load times and reduces the reliance on JavaScript for the initial view, ensuring a faster and more seamless user experience.
SEO Benefits: Server-side rendering ensures search engines can crawl fully rendered pages, enhancing SEO and discoverability.
Progressive Enhancement: Remix ensures that even users with disabled JavaScript or poor network conditions get a functioning app. This enhances functionality for users with better resources, providing a smooth and interactive experience.
Getting Started with Remix
Project Setup and Configuration
To start a new Remix project, you can use the following command:
npx create-remix@latest
For using an official Remix stack template, you can use:
npx create-remix@latest --template blues-stack
To run the project locally, navigate to your project directory and run:
cd your-project
npm run dev
Example Features
Nested Routes ExampleHere is an example of how nested routes can be implemented in Remix:
import { Outlet } from "@remix-run/react";
export default function SomeRoute() {
return (
<div>
<h1>My App</h1>
<main>
<Outlet />
</main>
</div>
);
}
Form Component and Action Function ExampleExample of form handling in Remix with server-side action:
import { Form } from "@remix-run/react";
import { redirect } from "@remix-run/node";
export const action = async ({ request }) => {
const formData = await request.formData();
const title = formData.get("title");
const slug = formData.get("slug");
await addPost({ title, slug });
return redirect("/somewhere");
};
export default function NewBlog() {
return (
<Form method="post">
<label>
Title: <input type="text" name="title" />
</label>
<label>
Slug: <input type="text" name="slug" />
</label>
<button type="submit">Add Post</button>
</Form>
);
}
Conclusion
Remix is a cutting-edge framework that leverages the best of web standards to deliver high-performing, dynamic web applications. Its focus on progressive enhancement, nested routes, and server/client-side data management sets it apart from other frameworks like Next.js. Whether for seasoned developers or newcomers, Remix offers substantial benefits that make it a compelling choice for modern web development.