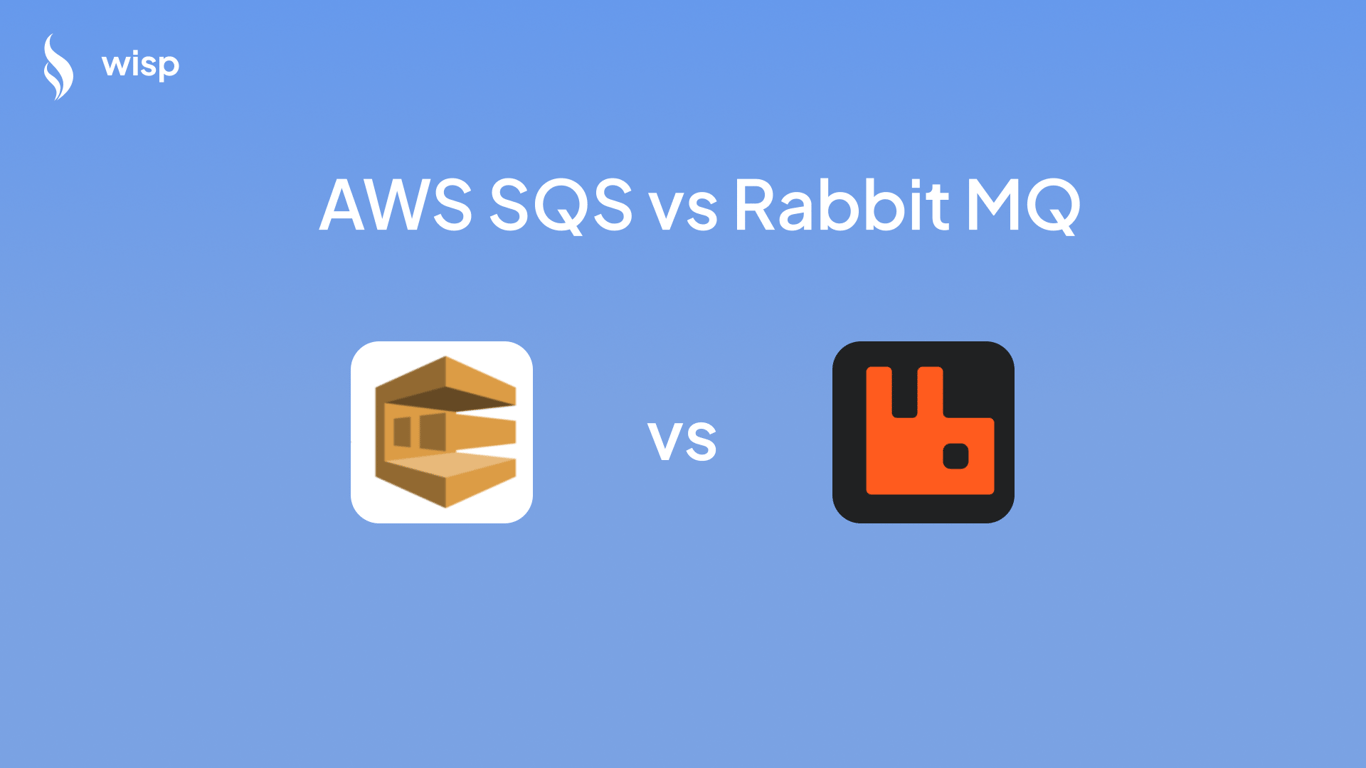
You've hit a crossroads in your system architecture journey. Your team needs a reliable message queue to handle communication between services, but you're torn between Amazon Simple Queue Service (SQS) and RabbitMQ. The constant back-and-forth between "Should we manage it ourselves?" and "Can we trust a managed service?" is keeping you up at night.
If you're wrestling with constant polling issues in SQS, concerned about RabbitMQ's maintenance overhead, or worried about message reliability - you're not alone. Many developers face these exact challenges when choosing between these two popular message queuing solutions.
Understanding the Basics
Before diving into the comparison, let's clarify what each service offers:
Amazon SQS (Simple Queue Service)
Amazon SQS is a fully managed message queuing service that enables you to decouple and scale microservices, distributed systems, and serverless applications. As part of the AWS ecosystem, it handles all the complexity of message delivery, including infrastructure management, scaling, and maintenance.
Key Features:
Fully managed service with no server management required
Automatic scaling based on demand
Standard and FIFO (First-In-First-Out) queue types
Native integration with AWS services
Pay-per-use pricing model
RabbitMQ
RabbitMQ is an open-source message broker that traditionally runs on your infrastructure (though managed options exist). It implements several messaging protocols, with Advanced Message Queuing Protocol (AMQP) being the primary one.
Key Features:
Multiple messaging protocols support (AMQP, MQTT, STOMP)
Flexible routing with exchanges and bindings
Rich plugin ecosystem
Clustering capabilities for high availability
Complete control over infrastructure and configuration
The Real-World Pain Points
From our research into developer experiences, several common challenges emerge when working with these systems:
SQS Polling Concerns: Many developers express frustration with SQS's requirement for constant polling. As one developer noted, "you have to constantly poll the Queue for new messages, and if request time outs after WaitTimeSeconds expires, you get back empty message if the Queue is empty."
Management Overhead: Teams often struggle with RabbitMQ's maintenance requirements. A DevOps team shared their experience: "We've been using RabbitMQ for sending/consuming queues, but our DevOps team want to switch to some managed solution in order to guarantee High Availability (99.99%+ uptime)."
Message Reliability: There are concerns about message duplication in SQS under certain circumstances, leading some teams to choose RabbitMQ for guaranteed message delivery without duplication.
Deep Dive: SQS vs RabbitMQ Feature Comparison
Architecture and Message Handling
SQSMessage Delivery: Uses a pull-based model where consumers must poll for messages
Scalability: Automatically scales with no upper limit on throughput
Message Retention: Messages can be retained for up to 14 days
Message Size: Limited to 256KB per message
Ordering: Standard queues provide best-effort ordering; FIFO queues guarantee ordering
Message Delivery: Push-based model with immediate message delivery
Scalability: Manual scaling through clustering
Message Retention: Limited by available storage
Message Size: No built-in size limitation (practical limits apply)
Ordering: Natural FIFO ordering within queues
Integration and Management
SQSAWS Integration: Seamless integration with AWS services like Lambda, EC2, and CloudWatch
Management: Minimal management required; AWS handles infrastructure
Monitoring: Built-in CloudWatch metrics and AWS Console visibility
Security: IAM roles and policies for access control
Protocol Support: Multiple protocols (AMQP, MQTT, STOMP, etc.)
Management: Requires hands-on management of infrastructure
Monitoring: Web-based management UI and extensive monitoring tools
Security: Plugin-based security with LDAP support
Cost Considerations
SQSPay-per-request pricing model
No upfront costs or minimum fees
Costs can scale with usage
Additional charges for data transfer
Infrastructure costs (servers, storage)
Operational costs (monitoring, maintenance)
Potential cost savings at high volumes
More predictable pricing for consistent workloads
Real-World Performance Insights
From developer experiences:
Message Processing
SQS users report occasional message duplication: "I heard that SQS can send the same message more than once in certain circumstances. That's the main reason I went with RabbitMQ."
RabbitMQ offers more precise control over message handling but requires careful configuration
Visibility and Debugging
SQS has limited visibility into failed messages: "If queue visibility is important than don't use SQS, basically you can just see messages in flight. You don't see stuff like what failed, why it failed, etc..."
RabbitMQ provides detailed insights into message flow and failure scenarios
Making the Right Choice: Use Case Analysis
When to Choose SQS
AWS-Native Applications
If your application is already running on AWS
When you need seamless integration with other AWS services
For serverless architectures using Lambda
Minimal Management Overhead
When you have limited DevOps resources
If you prefer focusing on application logic rather than infrastructure
When you need automatic scaling without intervention
Simple Queuing Needs
For basic producer-consumer patterns
When message ordering is not critical (using standard queues)
If you can work within the 256KB message size limit
When to Choose RabbitMQ
Complex Routing Requirements
When you need advanced message routing patterns
If you require pub/sub functionality
For applications using multiple messaging protocols
Complete Control
When you need full control over the messaging infrastructure
If you require specific optimizations for your use case
When you want to avoid vendor lock-in
High-Performance Requirements
For applications requiring minimal latency
When you need guaranteed message ordering
If you need to handle messages larger than 256KB
Implementation Best Practices
SQS Implementation Tips
Handling Duplicate Messages
# Implement idempotency using a unique message ID def process_message(message): message_id = message['MessageId'] if not is_message_processed(message_id): # Process the message mark_message_as_processed(message_id)
Optimal Polling
Use long polling (20 seconds) to reduce API calls
Implement exponential backoff for empty queues
Consider using the AWS SDK's built-in batch processing
Cost Optimization
Delete messages promptly after processing
Use batch operations when possible
Monitor CloudWatch metrics to optimize polling frequency
RabbitMQ Implementation Tips
Connection Management
# Implement proper connection handling def get_connection(): try: connection = pika.BlockingConnection(parameters) return connection except pika.exceptions.AMQPConnectionError: # Implement retry logic return handle_connection_error()
Error Handling
Implement dead letter queues for failed messages
Use proper acknowledgment modes
Handle "poison pill" messages appropriately
High Availability Setup
Configure clustering for redundancy
Implement proper monitoring
Use mirrored queues for critical data
Common Pitfalls to Avoid
SQS Pitfalls
Relying on exact message ordering in standard queues
Not handling duplicate messages
Inefficient polling strategies
RabbitMQ Pitfalls
Inadequate monitoring setup
Poor connection management
Insufficient cluster configuration
Conclusion
The choice between SQS and RabbitMQ ultimately depends on your specific needs:
Choose SQS if you want a managed service with minimal operational overhead and are already invested in the AWS ecosystem. It's particularly suitable for teams that want to focus on application development rather than infrastructure management.
Choose RabbitMQ if you need complex routing capabilities, want to avoid vendor lock-in, or require complete control over your messaging infrastructure. It's ideal for teams with strong DevOps capabilities and specific performance requirements.
Remember that these solutions aren't mutually exclusive. Many organizations use both services for different use cases within their architecture.
Additional Resources
By carefully considering your requirements and understanding these solutions' strengths and limitations, you can make an informed decision that best serves your application's needs.