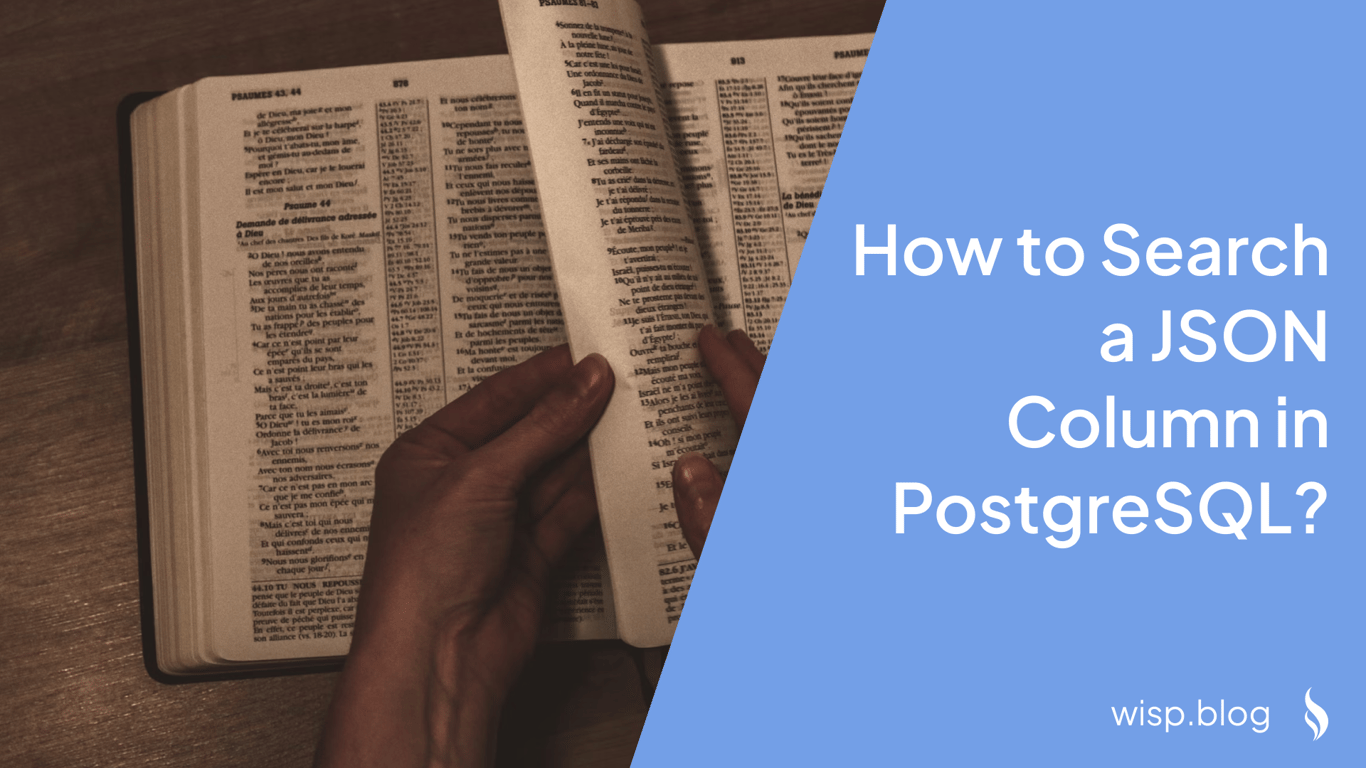
Are you working with JSON data in PostgreSQL and finding yourself puzzled about the most efficient way to search through it? Whether you're dealing with a few thousand records or scaling up to millions, understanding how to properly query JSON columns is crucial for maintaining performant applications.
In this comprehensive guide, we'll explore how to effectively search JSON columns in PostgreSQL, with a particular focus on the jsonb
data type that enables efficient searching and indexing.
Understanding JSON Data Types in PostgreSQL
Before diving into search techniques, it's essential to understand that PostgreSQL offers two distinct JSON data types:
JSON: Stores an exact copy of the input text
JSONB: Stores data in a decomposed binary format
While both types enforce valid JSON syntax, jsonb
is generally the preferred choice for searching and indexing. Here's why:
Feature | JSON | JSONB |
---|---|---|
Storage Format | Text | Binary |
Processing Overhead | Lower on input | Lower on processing |
Indexing Support | No | Yes (GIN indexes) |
Search Performance | Slower | Faster |
Setting Up Your JSON Column
Let's create a sample table to demonstrate JSON searching capabilities:
CREATE TABLE customer (
id SERIAL PRIMARY KEY,
data JSONB NOT NULL
);
And insert some sample data:
INSERT INTO customer (data) VALUES
('{
"first_name": "John",
"last_name": "Doe",
"email": "john.doe@example.com",
"phone_numbers": [
{"type": "home", "number": "555-1234"},
{"type": "work", "number": "555-5678"}
]
}');
Basic JSON Search Operations
PostgreSQL provides several operators for querying JSON data. Here are the most commonly used ones:
The Arrow Operators
->
: Gets JSON object field as JSON->>
: Gets JSON object field as text
Let's see these in action:
-- Get first name as text
SELECT data->>'first_name' FROM customer;
-- Get phone numbers array as JSON
SELECT data->'phone_numbers' FROM customer;
Searching for Specific Values
To search for customers with a specific first name:
SELECT * FROM customer
WHERE data->>'first_name' = 'John';
Searching Nested Objects
For nested data like phone numbers:
SELECT *
FROM customer AS c,
jsonb_array_elements(c.data->'phone_numbers') AS phones
WHERE phones->>'type' = 'home';
Case-Insensitive Search
For partial matches ignoring case:
SELECT * FROM customer
WHERE data->>'first_name' ILIKE '%joh%';
Optimizing Performance with Indexes
When dealing with large datasets, proper indexing becomes crucial. Based on user experiences from production environments, implementing the right indexes can lead to significant performance improvements.
Creating Indexes
For basic text searches on specific fields:
CREATE INDEX idx_customer_firstname
ON customer USING GIN ((data->>'first_name'));
CREATE INDEX idx_customer_lastname
ON customer USING GIN ((data->>'last_name'));
For searching across the entire JSON document:
CREATE INDEX idx_customer_data
ON customer USING GIN (data);
Performance Considerations
When working with JSON columns in PostgreSQL, keep these performance factors in mind:
Data Volume: Users working with millions of records report that proper indexing can make queries up to 6 times faster.
Query Complexity: Complex nested queries might require multiple indexes or consideration of alternative solutions like Elasticsearch for better performance.
Write vs. Read Operations: JSONB indexes can slow down write operations while significantly improving read performance.
Testing Performance
Before deploying to production, it's crucial to test performance with realistic data volumes. Here's a recommended approach:
Create a test dataset with millions of records
Run EXPLAIN ANALYZE on your common queries
Monitor query execution times with and without indexes
Test different index types and combinations
EXPLAIN ANALYZE
SELECT * FROM customer
WHERE data->>'first_name' ILIKE '%John%';
Best Practices and Recommendations
Based on real-world experiences shared in the PostgreSQL community, here are some best practices for working with JSON searches:
Choose JSONB Over JSON
Always use JSONB for searchable content
The binary format provides better query performance
Enables index support for faster searches
Index Strategically
Create indexes only for frequently searched fields
Monitor index usage and remove unused indexes
Consider partial indexes for specific use cases
Consider Alternatives
For search-heavy applications, consider using Elasticsearch alongside PostgreSQL
Use normalized tables for frequently queried structured data
Implement caching for commonly accessed data
When to Consider Alternative Solutions
While PostgreSQL's JSON capabilities are powerful, there are scenarios where you might want to consider alternatives:
High-Volume Search Operations
If your application primarily focuses on search functionality
When dealing with complex full-text search requirements
When handling hundreds of millions of records
Complex Search Requirements
Need for advanced text analysis and scoring
Requirement for fuzzy matching
Complex aggregations on JSON data
Conclusion
PostgreSQL's JSON search capabilities provide a robust solution for many use cases, especially when properly configured with JSONB and appropriate indexing. The key is to understand your specific requirements and test thoroughly with realistic data volumes.
For applications dealing with millions of records, proper indexing and regular performance testing are crucial. While PostgreSQL can handle JSON searches efficiently, consider supplementing with specialized search solutions like Elasticsearch for search-heavy applications.
Remember to always test your queries with EXPLAIN ANALYZE and monitor performance as your data grows. This proactive approach will help ensure your application maintains optimal performance as it scales.