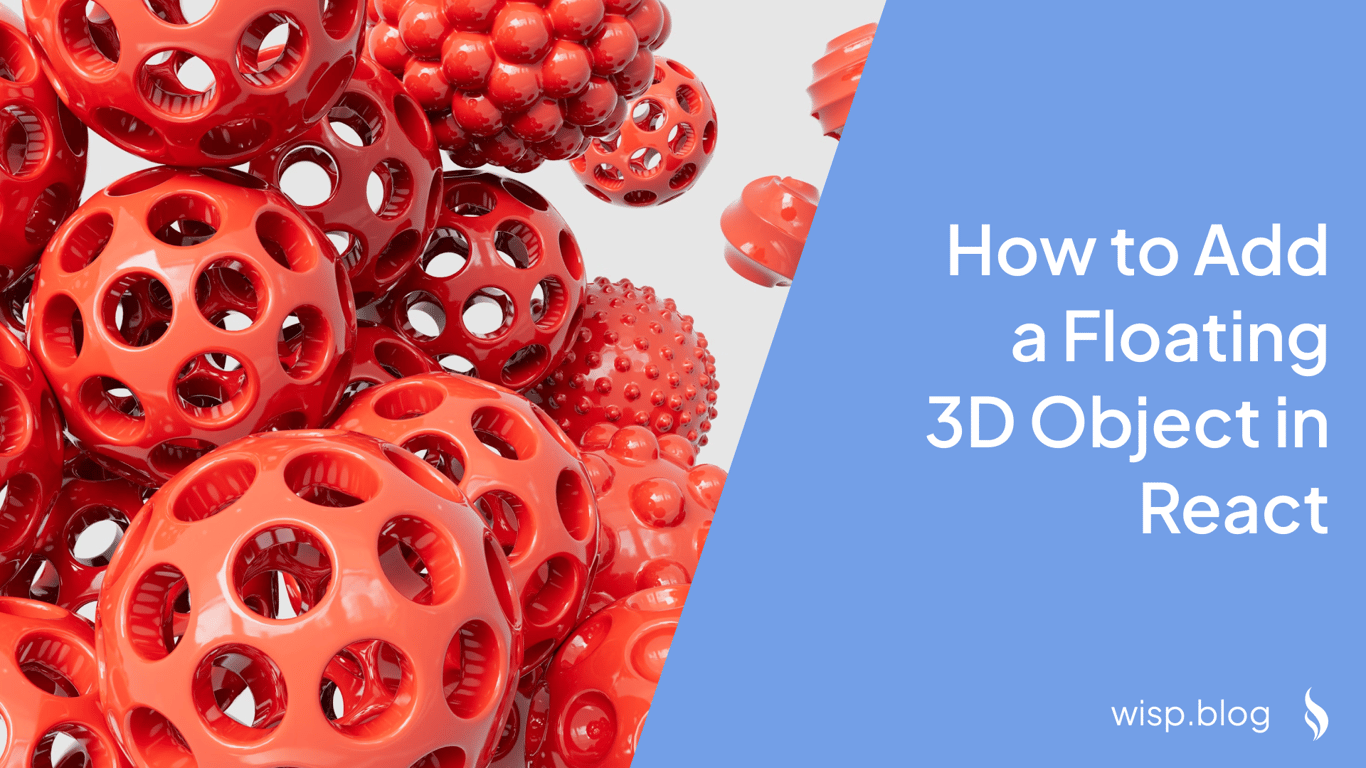
You've probably noticed the trend - sleek 3D objects floating and spinning on modern websites, creating an engaging and interactive experience. While these elements can be visually stunning, many developers struggle with implementing them effectively. Some worry about conversion rates, others about performance issues, and many find Three.js intimidating at first glance.
This comprehensive guide will walk you through adding a floating 3D object to your React application, addressing common concerns and providing practical solutions for optimal implementation.
Why Add 3D Objects to Your React Application?
Before diving into the technical details, let's address the elephant in the room. According to recent discussions on Reddit, developers often question whether 3D objects actually improve conversions or if they're just unnecessary eye candy that might distract visitors from the actual product.
The truth is, when implemented thoughtfully, 3D elements can:
Create a memorable and engaging user experience
Showcase products from multiple angles
Add a modern, professional touch to your website
Increase user interaction and time spent on page
However, the key lies in proper implementation. As one developer noted, "The jankiness of it moving is... something," highlighting the importance of smooth performance and optimization.
Prerequisites
Before we begin, make sure you have:
Node.js version 16.8 or later installed
Basic knowledge of React.js and TypeScript
A code editor of your choice
Familiarity with npm or yarn package manager
Setting Up Your Development Environment
Let's start by creating a new React application using Next.js, which provides an excellent foundation for our 3D implementation:
npx create-next-app@latest --typescript
Once your project is created, install the necessary dependencies:
npm install three @react-three/fiber @react-three/drei
These packages include:
three
: The core Three.js library@react-three/fiber
: A React renderer for Three.js@react-three/drei
: A collection of useful helpers and abstractions
Many developers have found React Three Fiber to be a game-changer in working with 3D graphics. As one developer shared on Reddit, "Three.js has been one of those things that I've struggled with for many years. Until it finally clicked thanks to React Three Fiber."
Creating Your First 3D Component
Let's create a simple floating 3D object component. We'll start with a basic structure and then add animation and interactivity.
First, create a new file in your components directory:
// src/app/components/FloatingObject.tsx
"use client";
import { useRef } from "react";
import { Canvas, useFrame } from "@react-three/fiber";
import { OrbitControls } from "@react-three/drei";
import { Mesh } from "three";
function Box() {
const meshRef = useRef<Mesh>(null!);
useFrame((state, delta) => {
meshRef.current.rotation.y += delta;
meshRef.current.position.y = Math.sin(state.clock.elapsedTime) * 0.2;
});
return (
<mesh ref={meshRef}>
<boxGeometry args={[2, 2, 2]} />
<meshStandardMaterial color="orange" />
</mesh>
);
}
export function FloatingObject() {
return (
<div style={{ width: "100%", height: "500px" }}>
<Canvas>
<ambientLight intensity={0.5} />
<pointLight position={[10, 10, 10]} />
<OrbitControls />
<Box />
</Canvas>
</div>
);
}
Import and use the component in your page:
// src/app/page.tsx
import { FloatingObject } from "./components/FloatingObject";
export default function Home() {
return (
<main>
<h1>My 3D Object</h1>
<FloatingObject />
</main>
);
}
Understanding the Code
Let's break down the key elements of our 3D implementation:
Canvas Component: This is the container where Three.js will render our 3D scene. It creates a WebGL context and handles the rendering loop.
Mesh Component: Represents our 3D object. It consists of:
Geometry (the shape)
Material (the surface appearance)
Transform (position, rotation, scale)
Lighting: We've added two types of lights:
ambientLight
: Provides overall scene illuminationpointLight
: Creates directional shadows and highlights
OrbitControls: Enables user interaction with the 3D object:
Click and drag to rotate
Scroll to zoom
Right-click and drag to pan
Animation: The
useFrame
hook updates our object's position and rotation on every frame:useFrame((state, delta) => { meshRef.current.rotation.y += delta; meshRef.current.position.y = Math.sin(state.clock.elapsedTime) * 0.2; });
Adding a Custom 3D Model
While a simple box is great for learning, you'll likely want to use custom 3D models in your projects. Here's how to load a GLTF model:
// src/app/components/ModelViewer.tsx
"use client";
import { useLoader } from "@react-three/fiber";
import { GLTFLoader } from "three/examples/jsm/loaders/GLTFLoader";
function Model() {
const gltf = useLoader(GLTFLoader, "/path/to/your/model.gltf");
return <primitive object={gltf.scene} />;
}
Optimization and Performance
One of the biggest challenges developers face is optimizing 3D models for web use. As discussed on Reddit, large models can cause significant performance issues, with some reaching sizes of "400MB in .glb format and a little over 3GB in .obj format."
Here are essential optimization techniques:
Model Compression
Use Draco compression for your GLTF files
Reduce polygon count while maintaining visual quality
Optimize textures using tools like TextureCompressor
Code-level Optimization
// Implement lazy loading const Model = lazy(() => import("./Model")); // Use suspense for loading states <Suspense fallback={<LoadingSpinner />}> <Model /> </Suspense>
Performance Monitoring
// Add performance monitoring import Stats from "three/examples/jsm/libs/stats.module"; const stats = Stats(); document.body.appendChild(stats.dom); useFrame(() => { stats.update(); });
Common Issues and Solutions
Browser Compatibility Many developers worry about browser support. React Three Fiber works in all modern browsers that support WebGL. To handle older browsers:
// Check for WebGL support
if (!WebGL.isWebGLAvailable()) {
const warning = WebGL.getWebGLErrorMessage();
document.getElementById('container').appendChild(warning);
}
Mobile Performance For mobile devices, consider implementing level of detail (LOD):
import { LOD } from "three";
function AdaptiveModel() {
const lod = new LOD();
// Add different detail levels
lod.addLevel(highDetailModel, 0);
lod.addLevel(mediumDetailModel, 50);
lod.addLevel(lowDetailModel, 100);
return <primitive object={lod} />;
}
Best Practices and Tips
Loading States Always provide feedback during model loading:
function ModelViewer() {
return (
<Suspense fallback={
<div className="loading">
<span>Loading 3D Model...</span>
<ProgressBar />
</div>
}>
<Model />
</Suspense>
);
}
Error Handling Implement proper error boundaries for 3D content:
class ModelErrorBoundary extends React.Component {
componentDidCatch(error, errorInfo) {
// Log error to your analytics service
console.error(error);
// Show fallback UI
}
render() {
return this.state.hasError ? (
<div>Unable to load 3D content</div>
) : (
this.props.children
);
}
}
Accessibility Don't forget about users who may not be able to interact with 3D content:
<div role="region" aria-label="3D Model Viewer">
<Canvas>
<Model />
</Canvas>
<div className="controls" aria-label="Model controls">
{/* Alternative controls or description */}
</div>
</div>
Conclusion
Adding a floating 3D object to your React application can significantly enhance user engagement when implemented correctly. While there are legitimate concerns about performance and conversion rates, proper optimization and thoughtful implementation can address these issues effectively.
Remember to:
Start with simple shapes before moving to complex models
Implement proper loading states and error handling
Optimize your models using compression techniques
Consider mobile performance and browser compatibility
Test thoroughly across different devices and browsers
As the web continues to evolve, 3D content is becoming increasingly important for creating engaging user experiences. By following this guide and best practices, you can confidently add impressive 3D elements to your React applications while maintaining performance and accessibility.