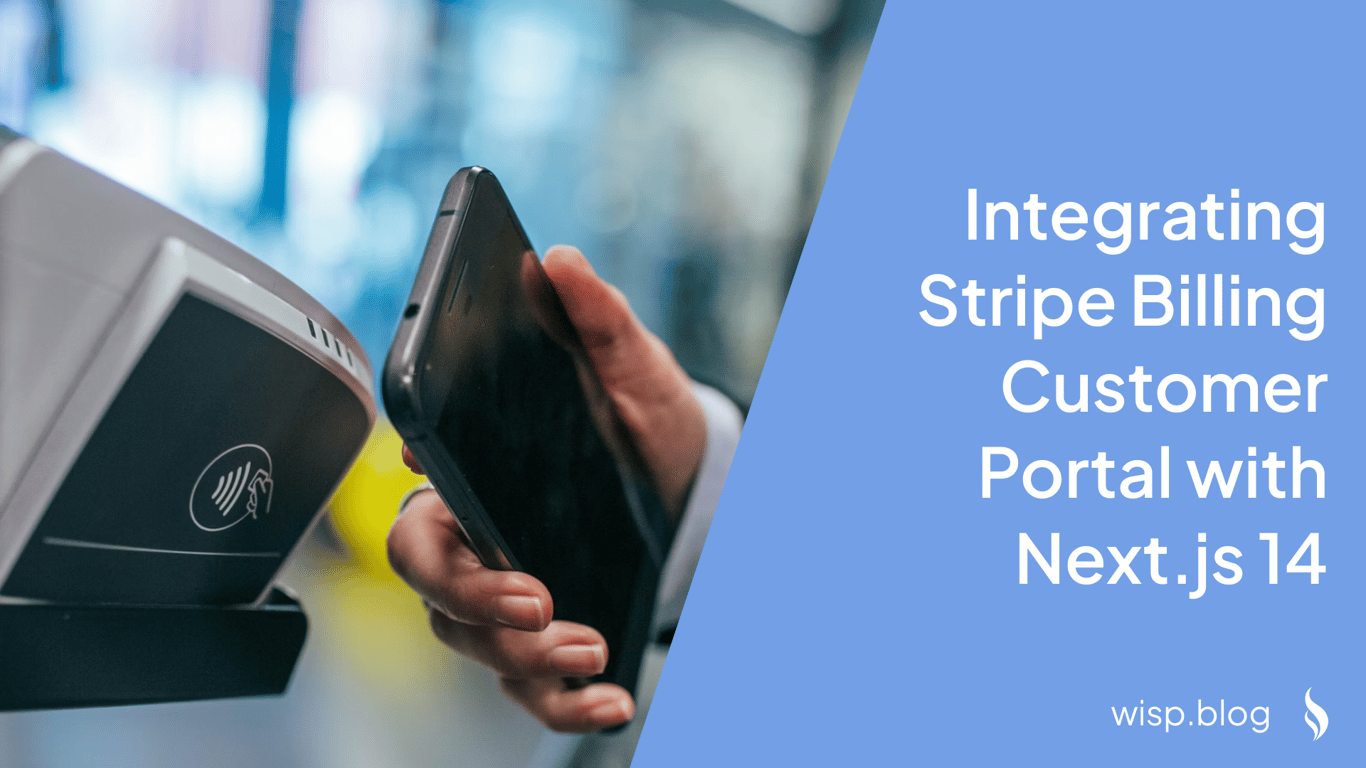
Integrating the Stripe Billing Customer Portal with Next.js 14 involves setting up Stripe, handling webhooks, and managing subscription data. This guide covers each step with detailed code snippets, best practices, and insights to ensure an efficient integration process.
Setting Up Stripe and Customer PortalCreate a Stripe Account
Sign up for a Stripe account.
Navigate to the Stripe Dashboard to create necessary products and configure the customer portal.
Create a pricing plan for your products.
Configure the Customer Portal
Go to Stripe Dashboard > Settings > Billing > Customer Portal.
Configure options like update billing information, cancel subscriptions, and switch between pricing plans.
Code Example: Adding a Customer Portal Button
import axios from 'axios'; import { useRouter } from 'next/router'; const ManageSubscriptionButton = () => { const router = useRouter(); const handleClick = async () => { const { data } = await axios.get('/api/portal'); router.push(data.url); }; return <button onClick={handleClick}>Manage Subscription</button>; }; export default ManageSubscriptionButton;
Setup API Route for Customer Portal Session
Create an API route to handle customer portal session creation.
import { NextApiRequest, NextApiResponse } from 'next'; import Stripe from 'stripe'; const stripe = new Stripe(process.env.STRIPE_SECRET_KEY, { apiVersion: '2022-08-27' }); export default async (req: NextApiRequest, res: NextApiResponse) => { const session = await stripe.billingPortal.sessions.create({ customer: req.body.customerId, return_url: 'https://your-website.com/account', }); res.send({ url: session.url }); };
Webhook Handling for Subscription Changes
Setup webhook endpoint to listen for subscription updates and cancellations.
import { NextApiRequest, NextApiResponse } from 'next'; import Stripe from 'stripe'; import { buffer } from 'micro'; const stripe = new Stripe(process.env.STRIPE_SECRET_KEY, { apiVersion: '2022-08-27' }); export const config = { api: { bodyParser: false } }; const webhookHandler = async (req: NextApiRequest, res: NextApiResponse) => { const buf = await buffer(req); const sig = req.headers['stripe-signature']; let event; try { event = stripe.webhooks.constructEvent(buf.toString(), sig, process.env.STRIPE_WEBHOOK_SECRET); } catch (err) { return res.status(400).send(`Webhook error: ${err.message}`); } if (event.type === 'customer.subscription.updated' || event.type === 'customer.subscription.deleted') { const subscription = event.data.object; // Handle subscription event (update your database, etc.) } res.json({ received: true }); }; export default webhookHandler;
Creating a Pricing Table Component
import React, { useState, useEffect } from 'react'; const StripePricingTable = () => { useEffect(() => { const script = document.createElement('script'); script.src = 'https://js.stripe.com/v3/pricing-table.js'; script.async = true; document.body.appendChild(script); return () => { document.body.removeChild(script); }; }, []); return ( <stripe-pricing-table pricing-table-id={process.env.NEXT_PUBLIC_STRIPE_PRICING_TABLE_ID} publishable-key={process.env.NEXT_PUBLIC_STRIPE_PUBLISHABLE_KEY} ></stripe-pricing-table> ); }; export default StripePricingTable;
Managing Subscriptions through Customer Portal
import axios from 'axios'; const handleManageSubscription = async () => { const { data } = await axios.get('/api/portal'); window.location.href = data.url; }; return <button onClick={handleManageSubscription}>Manage Subscription</button>;
By integrating Stripe Billing Customer Portal with Next.js 14, you can offer a seamless billing experience to your users. This guide covered detailed steps and provided code examples for both frontend and backend integration processes.