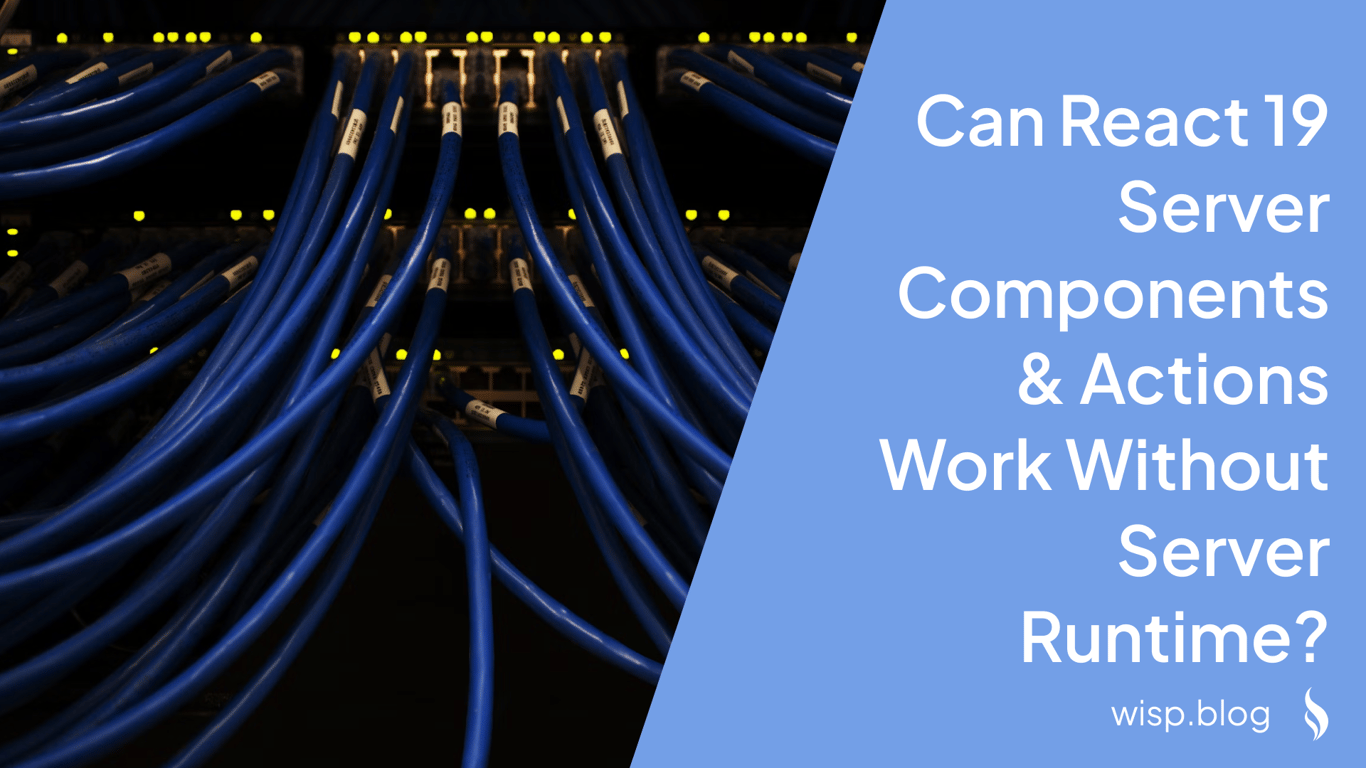
You've been exploring React's latest features - Server Components and Server Actions - and found yourself wondering if they can work without a dedicated server runtime. Perhaps you're maintaining a static site or working with a separate backend, and the thought of adding server-side JavaScript feels like unnecessary complexity.
The React community has been abuzz with skepticism about these features. As one developer noted, "Various articles and discussions constantly go in the direction of why server components are the wrong direction." Others question whether this is just another trend that adds more complexity than value.
Let's cut through the confusion and examine how React 19's Server Components and Server Actions actually work, their runtime dependencies, and most importantly - whether they can function without a traditional server environment.
Understanding the Fundamentals
Before diving deep, let's clarify what we're dealing with:
React Server Components (RSC) are components that execute exclusively on the server, sending only the rendered result to the client. They're designed to:
Reduce client-side JavaScript bundle size
Enable direct database access without API layers
Improve initial page load performance
Server Actions are functions marked with 'use server' that run on the server but can be triggered from client components. They're meant to:
Simplify client-server interactions
Provide type-safe server functions
Enable progressive enhancement for forms
Here's a basic example of a Server Component:
// ProductDetails.js
async function ProductDetails({ id }) {
const product = await db.query(`SELECT * FROM products WHERE id = ${id}`);
return (
<div>
<h1>{product.name}</h1>
<p>{product.description}</p>
<AddToCartButton id={product.id} />
</div>
);
}
And a Server Action:
// actions.js
'use server'
async function addToCart(productId) {
const user = await getCurrentUser();
await db.query(`INSERT INTO cart (user_id, product_id) VALUES (?, ?)`,
[user.id, productId]);
return { success: true };
}
The Runtime Requirement Reality
Here's the crucial point that many developers miss: Without an environment like Node.js, it would not be possible to execute this JavaScript on the server. This isn't just a limitation - it's a fundamental aspect of how Server Components and Actions work.
As one developer aptly pointed out in a Reddit discussion: "How can you install libraries like Mongoose to communicate with the database if React, on its own, does not support these types of libraries, which are normally executed in the backend?"
This brings us to our core question: Can these features work without a server runtime?
Alternative Approaches
While you can't completely eliminate the need for a server runtime, there are several approaches to work with Server Components and Actions in different environments:
1. Build-time Generation
One approach is to execute Server Components during the build process rather than at runtime:
// This component's data will be fetched at build time
async function BlogPost({ slug }) {
const post = await fetchBlogPost(slug);
return (
<article>
<h1>{post.title}</h1>
{post.content}
</article>
);
}
This works well for:
Static content that doesn't need real-time updates
Sites with infrequent content changes
Projects where build-time data fetching is sufficient
2. Hybrid Solutions
You can combine static generation with client-side interactivity:
// StaticContent.js - Generated at build time
export default function StaticContent() {
return <div>Static content here...</div>;
}
// InteractiveWidget.js - Runs on the client
'use client'
export default function InteractiveWidget() {
return <button onClick={() => alert('Client-side interaction!')}>
Click me
</button>;
}
3. API-First Architecture
For applications with separate backends, you might want to skip Server Actions entirely:
// Using traditional API calls instead of Server Actions
function ProductList() {
const { data, error } = useSWR('/api/products', fetcher);
if (error) return <div>Failed to load</div>;
if (!data) return <div>Loading...</div>;
return <div>{/* Render products */}</div>;
}
Challenges and Considerations
When considering Server Components and Actions without a traditional runtime, several challenges emerge:
1. Scalability Concerns
A significant concern in the React community revolves around scalability. As one developer questioned: "If the code is so heavy, how would it work on backend? What if 1000 users are doing this heavy thing, you will pay a fortune to some cloud provider?"
To address these concerns:
Implement aggressive caching strategies
Utilize CDNs for static content delivery
Consider hybrid approaches that balance server and client processing
2. Development Complexity
Working without a server runtime can introduce additional complexity:
// Example of increased complexity in development setup
// webpack.config.js
module.exports = {
// ... other config
plugins: [
new ServerComponentsPlugin({
isServer: process.env.BUILD_TARGET === 'server',
runtime: 'static'
})
]
};
3. Limited Functionality
Without a server runtime, you lose access to key features:
Real-time data updates
Direct database access
File system operations
Environment-specific configurations
4. Build Process Overhead
Static generation of Server Components can significantly increase build times:
// Example of build-time data fetching
async function generateStaticParams() {
const products = await fetchAllProducts();
return products.map((product) => ({
id: product.id.toString()
}));
}
Best Practices and Recommendations
Based on community feedback and real-world experience, here are some recommended approaches:
1. Evaluate Your Use Case
Before implementing Server Components or Actions, consider:
Is your content primarily static or dynamic?
How frequently does your data change?
What are your scalability requirements?
Do you need real-time updates?
2. Choose the Right Tools
Several frameworks and tools can help manage Server Components without a traditional runtime:
Next.js: Offers the most mature implementation of Server Components
Gatsby: Excellent for static site generation with dynamic capabilities
Astro: Provides partial hydration and server-side rendering features
3. Implement Progressive Enhancement
Design your application to work without Server Components first, then enhance:
// Progressive enhancement example
function SubmitButton({ children }) {
const [isPending, startTransition] = useTransition();
return (
<button
disabled={isPending}
onClick={() => startTransition(() => {
// Fall back to client-side handling if server action isn't available
if (typeof submitForm === 'function') {
submitForm();
} else {
handleClientSide();
}
})}
>
{isPending ? 'Submitting...' : children}
</button>
);
}
Conclusion
While React 19's Server Components and Server Actions technically require a server runtime to function fully, there are ways to achieve similar benefits through alternative approaches. The key is understanding your specific needs and choosing the right architecture for your use case.
As the React ecosystem continues to evolve, we may see more solutions emerge for using these features in various environments. For now, consider these recommendations:
For static sites, leverage build-time generation
For dynamic applications, consider a hybrid approach
If you need full server capabilities, embrace a proper server runtime
Stay informed about the evolving React ecosystem
Remember what one developer wisely advised: "Wait for stability. Don't depend on Vercel to know what is and isn't stable." This approach will help you make informed decisions about implementing these features in your projects.
The future of React development is exciting, but it's essential to choose the right tools and approaches for your specific needs rather than following trends blindly. Whether you decide to use Server Components and Actions with or without a traditional runtime, ensure your choice aligns with your project's requirements and constraints.