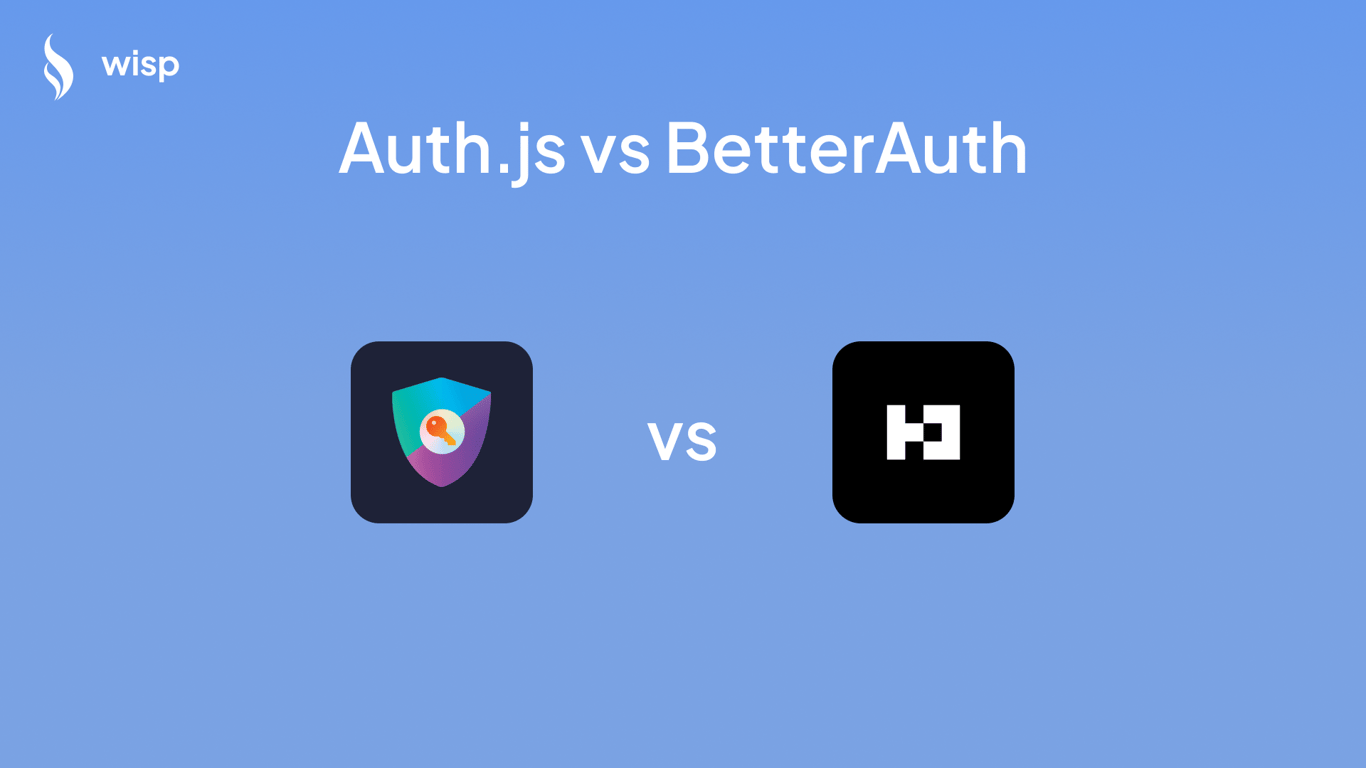
You've just started building your Next.js application and hit the inevitable authentication wall. After some research, you're torn between Auth.js (formerly NextAuth.js) and BetterAuth. The documentation for both looks promising, but you're worried about making the wrong choice and getting stuck with a problematic implementation down the line.
"I am really struggling with Auth.js," writes one developer on Reddit. "The docs was horrible, it offers little customizability, and the configuration just doesn't work." This sentiment echoes across many development forums, highlighting the common frustrations developers face when implementing authentication in Next.js applications.
But is BetterAuth truly the answer? Let's dive deep into both solutions to help you make an informed decision for your project.
Understanding the Landscape
Authentication in Next.js applications requires careful consideration of several factors:
Security and reliability
Ease of implementation
Documentation quality
Community support
Customization options
Integration capabilities
Both Auth.js and BetterAuth approach these requirements differently, each with their own strengths and trade-offs.
Auth.js: The Established Player
Auth.js, previously known as NextAuth.js, has been a go-to solution for Next.js authentication for years. It's backed by a large community and offers extensive customization options. However, recent user experiences have highlighted significant challenges:
Complex setup procedures, especially for credential-based authentication
Documentation that often leaves developers confused
Difficulties with implementing non-standard authentication flows
Challenges with services like Entra ID integration
As one developer noted, "If this were my first experience with web auth, I would have just thought auth ought to be this hard." This sentiment reflects a common frustration with Auth.js's learning curve.
BetterAuth: The Rising Alternative
BetterAuth has emerged as a compelling alternative, focusing on developer experience and security. Users particularly appreciate:
Straightforward setup process
Clear, comprehensive documentation
Responsive support community
Built-in security best practices
"Setup was a breeze. Way easier and better," reports a developer who switched from Auth.js to BetterAuth. This experience is consistently echoed across various developer forums and discussions.
Feature Comparison
Let's break down the key features of both solutions to understand their capabilities better.
Auth.js Features
Authentication Providers
Built-in support for OAuth providers (Google, GitHub, etc.)
Custom credential providers
Email/passwordless authentication
Support for multiple providers simultaneously
Session Management
JWT and database session strategies
Customizable session handling
Session rotation and security features
Customization
Extensive configuration options
Custom pages and layouts
Event callbacks for authentication flows
BetterAuth Features
Core Authentication
Streamlined credential-based authentication
Built-in MFA support
Passwordless options
Social login integrations
Security Features
Advanced rate limiting
Automatic security headers
CSRF protection
Built-in password policies
Developer Experience
Type-safe APIs
Intuitive configuration
Comprehensive error handling
Real-time webhook support
Implementation Comparison
Let's look at how both solutions handle common authentication scenarios.
Setting Up Auth.js
First, install the necessary packages:
npm install next-auth
# or
yarn add next-auth
Create an API route for authentication:
// pages/api/auth/[...nextauth].ts
import NextAuth from "next-auth"
import CredentialsProvider from "next-auth/providers/credentials"
export default NextAuth({
providers: [
CredentialsProvider({
name: "Credentials",
credentials: {
username: { label: "Username", type: "text" },
password: { label: "Password", type: "password" }
},
async authorize(credentials, req) {
// Add your authentication logic here
const user = await authenticateUser(credentials)
if (user) {
return user
}
return null
}
})
],
session: {
strategy: "jwt"
},
callbacks: {
async session({ session, token }) {
// Add custom session handling
return session
}
}
})
Set up the provider in your app:
// pages/_app.tsx
import { SessionProvider } from "next-auth/react"
function MyApp({ Component, pageProps: { session, ...pageProps } }) {
return (
<SessionProvider session={session}>
<Component {...pageProps} />
</SessionProvider>
)
}
export default MyApp
Setting Up BetterAuth
Install BetterAuth:
npm install better-auth
# or
yarn add better-auth
Create your auth configuration:
// lib/auth.ts
import { createAuth } from "better-auth"
export const auth = createAuth({
secret: process.env.AUTH_SECRET,
database: {
type: "postgres",
url: process.env.DATABASE_URL
},
session: {
strategy: "jwt"
}
})
Set up the API route:
// pages/api/auth/[...auth].ts
import { auth } from "@/lib/auth"
import { createHandler } from "better-auth/next"
export default createHandler(auth)
Real-World Implementation Examples
Let's explore some common authentication scenarios and how they're handled in both libraries.
User Login with Auth.js
// components/LoginForm.tsx
import { signIn } from "next-auth/react"
import { useState } from "react"
export default function LoginForm() {
const [credentials, setCredentials] = useState({
email: "",
password: ""
})
const handleSubmit = async (e) => {
e.preventDefault()
try {
const result = await signIn("credentials", {
redirect: false,
email: credentials.email,
password: credentials.password
})
if (result?.error) {
// Handle error
console.error(result.error)
}
} catch (error) {
console.error("Login failed:", error)
}
}
return (
<form onSubmit={handleSubmit}>
<input
type="email"
value={credentials.email}
onChange={(e) => setCredentials({
...credentials,
email: e.target.value
})}
/>
<input
type="password"
value={credentials.password}
onChange={(e) => setCredentials({
...credentials,
password: e.target.value
})}
/>
<button type="submit">Login</button>
</form>
)
}
User Login with BetterAuth
// components/LoginForm.tsx
import { useAuth } from "better-auth/react"
import { useState } from "react"
export default function LoginForm() {
const { login } = useAuth()
const [credentials, setCredentials] = useState({
email: "",
password: ""
})
const handleSubmit = async (e) => {
e.preventDefault()
try {
await login({
email: credentials.email,
password: credentials.password
})
// Successful login will automatically update auth state
} catch (error) {
console.error("Login failed:", error)
}
}
return (
<form onSubmit={handleSubmit}>
<input
type="email"
value={credentials.email}
onChange={(e) => setCredentials({
...credentials,
email: e.target.value
})}
/>
<input
type="password"
value={credentials.password}
onChange={(e) => setCredentials({
...credentials,
password: e.target.value
})}
/>
<button type="submit">Login</button>
</form>
)
}
Protected Routes and Session Management
Auth.js Protected Routes
// middleware.ts
export { default } from "next-auth/middleware"
export const config = {
matcher: ["/protected/:path*"]
}
// pages/protected/dashboard.tsx
import { useSession } from "next-auth/react"
import { useRouter } from "next/router"
export default function Dashboard() {
const { data: session, status } = useSession()
const router = useRouter()
if (status === "loading") {
return <div>Loading...</div>
}
if (!session) {
router.push("/login")
return null
}
return (
<div>
<h1>Welcome {session.user.name}</h1>
{/* Dashboard content */}
</div>
)
}
BetterAuth Protected Routes
// middleware.ts
import { createMiddleware } from "better-auth/next"
import { auth } from "@/lib/auth"
export default createMiddleware(auth)
export const config = {
matcher: ["/protected/:path*"]
}
// pages/protected/dashboard.tsx
import { useAuth } from "better-auth/react"
export default function Dashboard() {
const { user, isLoading } = useAuth()
if (isLoading) {
return <div>Loading...</div>
}
return (
<div>
<h1>Welcome {user.name}</h1>
{/* Dashboard content */}
</div>
)
}
Performance and Security Considerations
Auth.js Security Features
JWT Handling
// pages/api/auth/[...nextauth].ts
export default NextAuth({
jwt: {
secret: process.env.JWT_SECRET,
maxAge: 60 * 60 * 24 * 30, // 30 days
encryption: true
},
security: {
csrf: true,
cookieSecure: process.env.NODE_ENV === "production"
}
})
Custom Authorization Logic
// lib/auth-checks.ts
export const checkUserPermissions = async (session) => {
if (!session) return false
// Add your custom authorization logic
const userRoles = await fetchUserRoles(session.user.id)
return userRoles.includes("admin")
}
BetterAuth Security Features
Built-in Rate Limiting
// lib/auth.ts
import { createAuth } from "better-auth"
export const auth = createAuth({
rateLimit: {
enabled: true,
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100 // limit each IP to 100 requests per windowMs
},
security: {
passwordPolicy: {
minLength: 8,
requireNumbers: true,
requireSpecialChars: true
}
}
})
MFA Implementation
// pages/api/auth/enable-mfa.ts
import { auth } from "@/lib/auth"
export default async function handler(req, res) {
const { user } = await auth.getUserSession(req)
if (!user) {
return res.status(401).json({ error: "Unauthorized" })
}
const secret = await auth.mfa.generateSecret()
const qrCode = await auth.mfa.generateQRCode(secret)
return res.json({ secret, qrCode })
}
Migration Considerations
Migrating from Auth.js to BetterAuth
If you're considering migrating from Auth.js to BetterAuth, here's a step-by-step approach:
Backup Your Data
# Export your users and sessions
pg_dump -t users -t sessions > auth_backup.sql
Update Dependencies
npm remove next-auth
npm install better-auth
Update Configuration
// Before (Auth.js)
export default NextAuth({
providers: [...],
callbacks: {...},
session: {...}
})
// After (BetterAuth)
export const auth = createAuth({
providers: {...},
session: {...},
callbacks: {...}
})
Update Components
// Before (Auth.js)
import { useSession } from "next-auth/react"
// After (BetterAuth)
import { useAuth } from "better-auth/react"
Making the Decision: Which One Should You Choose?
After analyzing both solutions in detail, here's a framework to help you make the decision:
Choose Auth.js If:
You Need Maximum Flexibility
You require extensive customization of authentication flows
You're working with multiple OAuth providers
You need to implement complex authorization schemes
You're Comfortable with Complexity
You have experience with authentication implementations
You're willing to invest time in understanding the documentation
You have the resources to handle potential issues
You Value Community Resources
You prefer solutions with extensive community tutorials
You want access to a large number of examples and implementations
You need integration with many third-party services
Choose BetterAuth If:
You Prioritize Developer Experience
You want a more straightforward setup process
You prefer clear, comprehensive documentation
You value responsive support
Security is a Top Concern
You need built-in security features
You want automatic implementation of security best practices
You require built-in MFA support
You're Building a New Project
You're starting from scratch
You want to avoid technical debt
You need quick implementation
Real-World Success Stories
From the developer community:
"The Auth.js Discord was deader than courdroy disco, so I tried switching to Better Auth... Better Auth worked, I was pointed to documentation that helped with my newbie questions." - Reddit user
"Setup was a breeze. Way easier and better. I've been using it for many months and I've never loved any auth framework so much." - Developer testimonial
These experiences highlight the importance of considering not just the technical capabilities but also the overall developer experience when choosing an authentication solution.
Conclusion and Best Practices
The choice between Auth.js and BetterAuth ultimately depends on your specific needs and circumstances. Here are some final recommendations to ensure success with either solution:
Best Practices for Auth.js
Documentation First
Thoroughly read the documentation before implementation
Keep track of version changes and updates
Join the GitHub discussions for complex issues
Testing Strategy
Implement comprehensive testing for authentication flows
Test edge cases and error scenarios
Use the provided testing utilities
Security Considerations
Regularly update dependencies
Implement proper error handling
Use secure session configurations
Best Practices for BetterAuth
Implementation Strategy
Follow the quick start guide step by step
Utilize the built-in security features
Take advantage of the type-safe APIs
Monitoring and Maintenance
Set up proper logging and monitoring
Keep the library updated
Use the provided debugging tools
Community Engagement
Participate in the community forums
Report issues and contribute feedback
Share your implementation experiences
Additional Resources
Remember, authentication is a critical component of your application's security infrastructure. Take the time to make an informed decision based on your specific requirements, team expertise, and project constraints. Whether you choose Auth.js or BetterAuth, ensure you follow security best practices and keep your implementation up to date with the latest security standards.