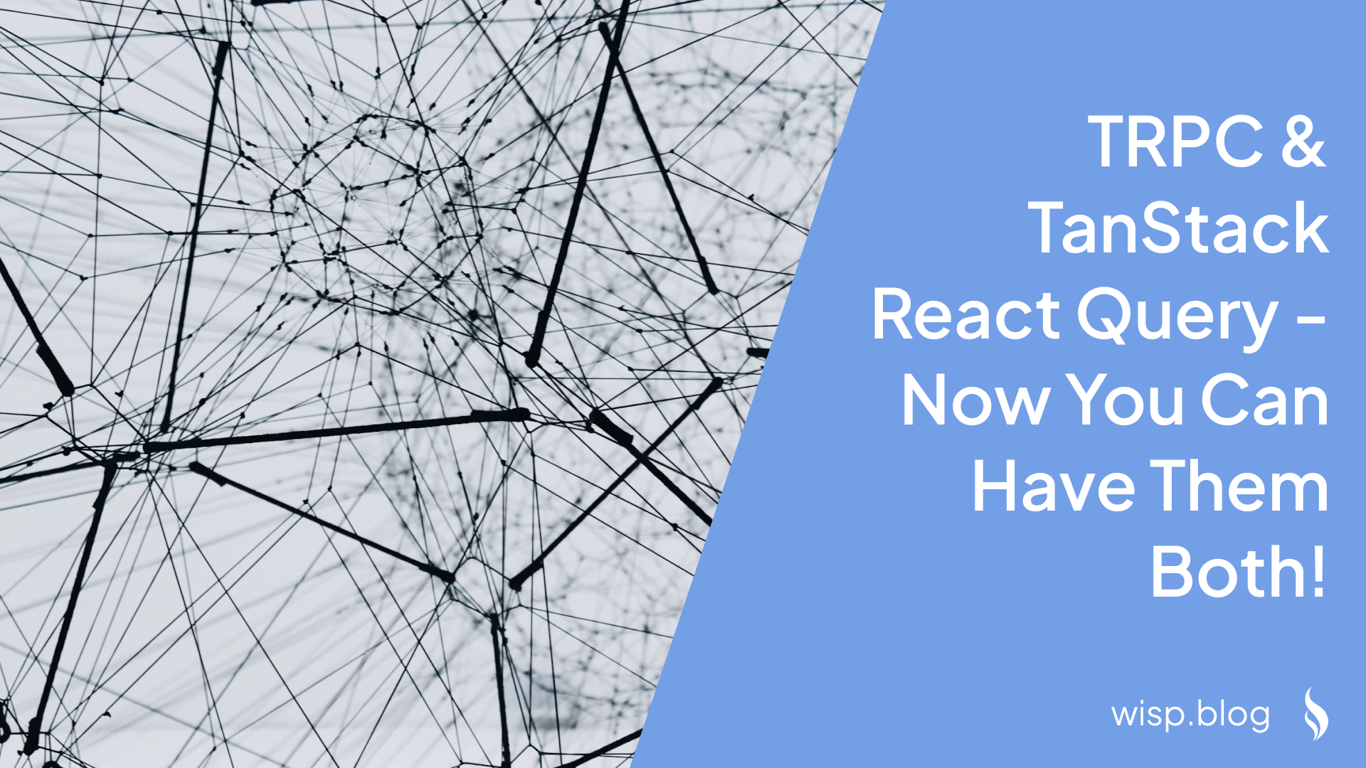
The development world is buzzing with excitement as tRPC releases its new TanStack React Query integration on February 17, 2025. This integration represents a significant milestone in how developers can manage API interactions and data fetching within React applications. If you've been following the React ecosystem, you know that both tRPC and TanStack Query have been game-changers in their own right. Now, they're joining forces to offer an even more powerful solution.
You've probably been in this situation: you're building a React application, and you need to manage server state effectively while maintaining type safety across your full-stack application. You might have used tRPC for its excellent TypeScript integration and end-to-end type safety, or TanStack Query (formerly React Query) for its powerful data synchronization capabilities. But what if you could have both?
The challenge has always been that while both libraries excel in their domains, developers often had to choose between tRPC's superior TypeScript integration and TanStack Query's robust data management features. Some developers even attempted to use both libraries together, resulting in complex wrapper code and reduced developer experience.
Here's the good news: the new integration changes everything. It brings together the best of both worlds, allowing you to leverage tRPC's type-safe APIs while taking full advantage of TanStack Query's powerful caching, background updates, and state management capabilities.
What Makes This Integration Special?
The new integration introduces several groundbreaking features that address common pain points developers have faced:
Native Interface Support: The integration now uses native QueryOptions and MutationOptions, eliminating the need for complex wrapper code. This means you can use familiar TanStack Query patterns while maintaining tRPC's type safety.
Improved Type Inference: One of the biggest headaches developers faced was accessing generated Query response types. The new integration makes this process more intuitive and straightforward.
Enhanced Query Key Management: Previous limitations in query key usage often forced developers to resort to vanilla client calls. The new integration provides better control over query keys, making caching and data invalidation more predictable.
Here's a simple example of how clean the new API looks:
import { useQuery } from '@tanstack/react-query';
import { useTRPC } from './trpc';
export function UserProfile() {
const trpc = useTRPC();
const userQuery = useQuery(
trpc.users.get.queryOptions({ id: '123' })
);
if (userQuery.isLoading) return <div>Loading...</div>;
return <div>Hello, {userQuery.data.name}!</div>;
}
Understanding the Core Components
tRPC: The Type-Safe RPC Framework
tRPC has revolutionized how we handle API interactions in TypeScript applications. At its core, it provides:
End-to-end Type Safety: No more guessing about API response shapes or manually maintaining type definitions.
Simplified API Layer: Eliminate the need for traditional REST endpoints and complex API documentation.
Zod Integration: Built-in schema validation ensures data integrity across your application.
According to the official documentation, tRPC transforms your API into a set of strongly-typed functions that can be called from your frontend code as if they were local functions.
TanStack Query: The Data Management Powerhouse
TanStack Query (formerly React Query) has established itself as the go-to solution for managing server state in React applications. Its key features include:
Automatic Background Updates: Keep your UI in sync with server data without manual intervention.
Smart Caching: Reduce unnecessary network requests and improve application performance.
Optimistic Updates: Provide instant feedback to users while handling server operations.
The Integration in Action
Let's look at some practical examples of how the new integration simplifies common tasks:
1. Basic Query with Type Safety
// Previous approach with separate type definitions
type User = {
id: string;
name: string;
email: string;
};
// New approach with automatic type inference
const userQuery = useQuery(
trpc.users.get.queryOptions({ id: '123' })
);
// userQuery.data is automatically typed as User
2. Mutations with Error Handling
const mutation = useMutation(
trpc.users.update.mutationOptions({
onSuccess: (data) => {
// Type-safe access to the updated user data
console.log(data.name);
},
onError: (error) => {
// Strongly typed error handling
console.error(error.message);
}
})
);
3. Query Invalidation and Cache Management
const queryClient = useQueryClient();
const mutation = useMutation(
trpc.posts.create.mutationOptions({
onSuccess: () => {
// Type-safe query invalidation
queryClient.invalidateQueries({
queryKey: trpc.posts.list.queryKey()
});
}
})
);
Migration and Best Practices
If you're considering adopting this integration or migrating an existing project, here are some key points to consider:
For New Projects
Start with the New Integration: If you're starting a new project, it's recommended to use the new integration from the beginning. This ensures you get all the benefits of both libraries without technical debt.
Proper Setup: Initialize your project with both libraries:
npm install @trpc/client @trpc/server @tanstack/react-query
Configure Once, Use Everywhere: Set up your tRPC client with TanStack Query integration:
import { createTRPCClient } from '@trpc/client';
import { QueryClient } from '@tanstack/react-query';
const queryClient = new QueryClient();
const trpcClient = createTRPCClient({
// Your configuration here
});
For Existing Projects
The integration team has thoughtfully considered the migration path for existing projects. You can:
Gradually Migrate: Both the classic tRPC API and the new TanStack integration can coexist in the same project.
Use the Migration Helper: The team has provided a codemod to help automate the migration process:
npx @trpc/migrate-to-tanstack-query@latest
Update Query Keys: Review and update your query key strategy to take advantage of the new integration's improved caching capabilities.
Common Challenges and Solutions
Based on user feedback and common issues reported in the React community, here are some challenges you might encounter and their solutions:
1. Accessing Generated Query Response Types
One of the most common confusions has been around accessing generated query response types. Here's how to handle it:
// Get the type of a query response
type UserData = inferQueryResponse<typeof trpc.users.get>;
// Use it in your components
const UserComponent = () => {
const query = useQuery(trpc.users.get.queryOptions());
const userData: UserData = query.data;
// ...
};
2. Managing Query Keys
The new integration provides better control over query keys, addressing previous limitations:
// Define custom query keys
const queryKey = trpc.users.list.queryKey({
status: 'active',
role: 'admin'
});
// Use in queries
const query = useQuery({
...trpc.users.list.queryOptions(),
queryKey
});
Performance and Optimization Tips
To get the most out of this integration, consider these performance optimization strategies:
1. Intelligent Query Invalidation
// Instead of invalidating all queries
queryClient.invalidateQueries();
// Invalidate specific queries
queryClient.invalidateQueries({
queryKey: trpc.users.list.queryKey(),
exact: true // Only invalidate this exact query
});
2. Prefetching Data
// Prefetch data for better user experience
await queryClient.prefetchQuery(
trpc.users.get.queryKey({ id: '123' }),
() => trpc.users.get.query({ id: '123' })
);
Looking Ahead
The integration of tRPC and TanStack React Query marks a significant step forward in the React ecosystem. It combines the type safety and simplicity of tRPC with the powerful data management capabilities of TanStack Query, creating a more robust and developer-friendly solution.
As the community continues to adopt and provide feedback on this integration, we can expect to see:
Further optimizations and performance improvements
Enhanced documentation and examples
Additional tooling support
More community-driven best practices
Resources and Further Reading
To dive deeper into this integration, check out these valuable resources:
Remember, while this integration offers powerful capabilities, it's essential to evaluate whether it fits your project's needs. Consider factors like team expertise, project scale, and specific requirements when deciding to adopt this solution.
The future of full-stack TypeScript development looks brighter with this integration, providing developers with the tools they need to build more robust and maintainable applications.