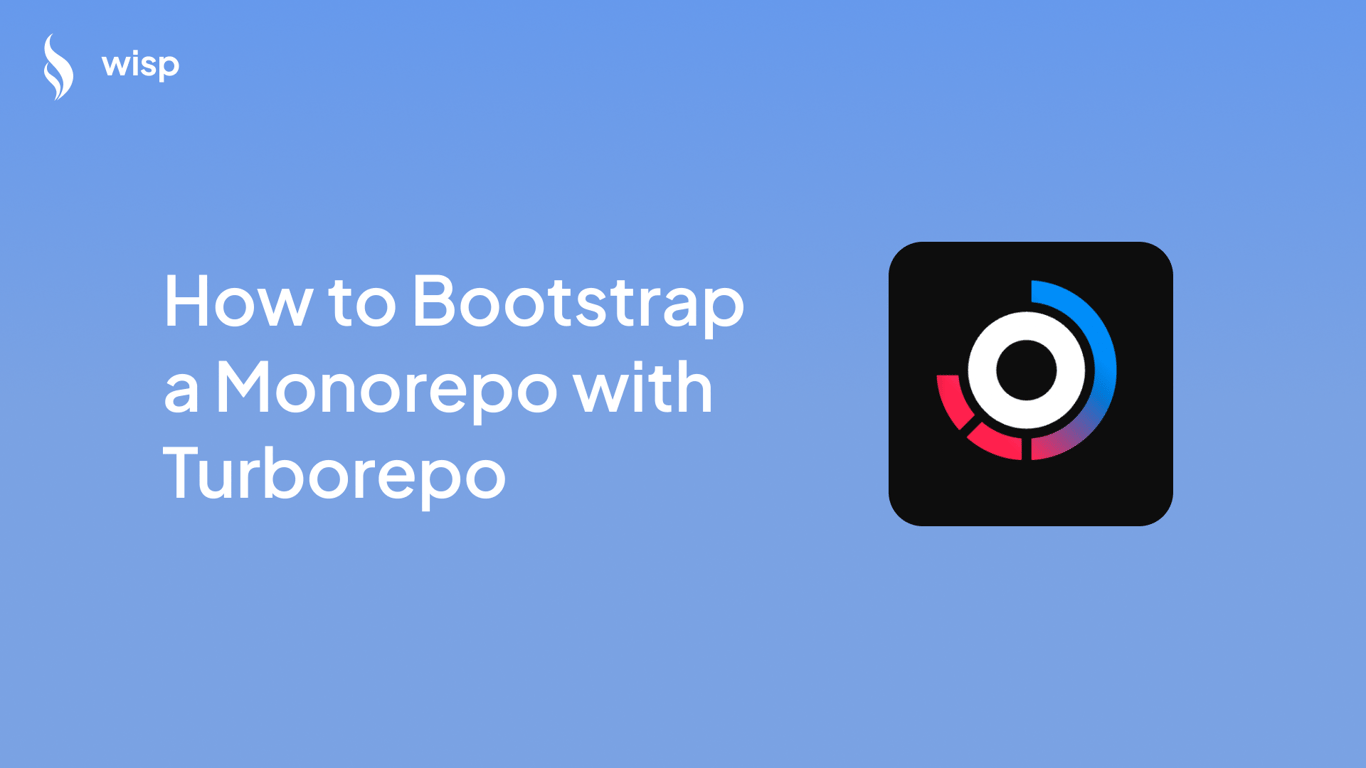
Are you tired of wrestling with multiple repositories, dealing with dependency hell, and watching your build times crawl? You're not alone. Many developers have experienced the frustration of working with poorly organized codebases that were just "thrown together and left to grow organically," as one developer described on Reddit.
The good news? Turborepo offers a powerful solution to transform this chaos into a well-structured, efficient development environment. In this guide, we'll walk through how to bootstrap a monorepo with Turborepo, addressing common pain points and implementing best practices that will save you countless hours of debugging and optimization.
Why Turborepo?
Before diving into the setup process, let's understand why Turborepo has become a go-to solution for modern JavaScript and TypeScript projects:
Lightning-Fast Builds: Achieve 40-85% faster builds through intelligent caching and task parallelization
Remote Caching: Share build artifacts across your team to eliminate redundant work
Simplified Dependency Management: Prevent the nightmare of "rogue imports" and mismatched versions that many developers struggle with
Flexible Configuration: Define all your tasks in a single
turbo.json
file while maintaining compatibility with npm, Yarn, or pnpm
Prerequisites
Before we begin, ensure you have:
Node.js (v14.0 or higher) installed
A package manager of your choice (npm, yarn, or pnpm)
Basic familiarity with JavaScript/TypeScript development
Git installed on your system
Initial Setup
Let's start by creating a new Turborepo project. Open your terminal and follow these steps:
First, install Turborepo globally:
npm install -g turbo
Create a new Turborepo project:
npx create-turbo@latest
This command will prompt you to:
Name your project
Choose your package manager (npm, pnpm, or yarn)
Set up your initial workspace structure
Understanding the Monorepo Structure
After initialization, you'll have a basic monorepo structure that looks something like this:
my-turborepo/
├── apps/
│ ├── web/
│ └── docs/
├── packages/
│ ├── ui/
│ └── config/
├── package.json
└── turbo.json
Let's break down each component:
The Root Directory
The root directory contains configuration files that govern your entire monorepo:
package.json
: Defines workspace settings and common dependencies
{
"private": true,
"workspaces": [
"apps/*",
"packages/*"
],
"scripts": {
"build": "turbo run build",
"dev": "turbo run dev",
"lint": "turbo run lint"
}
}
turbo.json
: Configures your build pipeline and caching behavior
{
"$schema": "https://turbo.build/schema.json",
"pipeline": {
"build": {
"dependsOn": ["^build"],
"outputs": ["dist/**"]
},
"lint": {
"outputs": []
},
"dev": {
"cache": false
}
}
}
The Apps Directory
The apps
directory contains your applications - the end products that users interact with. Each app is a separate project with its own:
Dependencies
Build configuration
Development server
Testing setup
The Packages Directory
The packages
directory houses shared code that multiple apps can use:
UI components
Configuration files
Utility functions
Business logic
This structure helps prevent the common pain point of duplicate code across projects and ensures consistency throughout your applications.
Best Practices for Monorepo Management
1. Dependency Management
One of the biggest challenges in monorepos is managing dependencies effectively. As one developer noted on Reddit, it's "too easy to add a simple 'import X from X' statement somewhere, creating unwanted extra dependencies."
To prevent this:
# Install Syncpack to manage dependencies
npm install -g syncpack
# Check for mismatched versions
syncpack list-mismatches
# Fix version mismatches
syncpack fix-mismatches
2. Optimizing Build Performance
To achieve the fastest possible builds:
Configure your pipeline in
turbo.json
:
{
"pipeline": {
"build": {
"dependsOn": ["^build"],
"outputs": ["dist/**"],
"cache": true
},
"test": {
"dependsOn": ["build"],
"outputs": ["coverage/**"],
"cache": true
}
}
}
Enable remote caching:
turbo login
turbo link
Use task dependencies wisely:
{
"pipeline": {
"dev": {
"dependsOn": ["^build"],
"cache": false
}
}
}
3. TypeScript Configuration
To avoid the common issue of mismatched TypeScript versions, create a base tsconfig.json
in your packages directory:
{
"compilerOptions": {
"target": "es5",
"module": "commonjs",
"strict": true,
"esModuleInterop": true,
"skipLibCheck": true,
"forceConsistentCasingInFileNames": true
}
}
Then extend it in each package:
{
"extends": "../../tsconfig.json",
"compilerOptions": {
"outDir": "./dist"
}
}
Advanced Features and Integration
CI/CD Integration
To address the concern that "DevOps is often brought in as an afterthought", here's how to set up efficient CI/CD pipelines with Turborepo:
Configure GitHub Actions workflow:
name: CI/CD
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- uses: actions/setup-node@v2
- name: Install dependencies
run: npm install
- name: Build
run: turbo run build
- name: Test
run: turbo run test
Enable remote caching in CI:
- name: Enable Turborepo Remote Caching
run: npx turbo login
env:
TURBO_TOKEN: ${{ secrets.TURBO_TOKEN }}
TURBO_TEAM: ${{ secrets.TURBO_TEAM }}
Monitoring and Maintenance
To keep your monorepo healthy:
Regular dependency updates:
# Update all dependencies
npm run update-dependencies
# Check for outdated packages
npm outdated
Cache management:
# Clear local cache if needed
turbo clean
# Prune old cache entries
turbo prune
Conclusion
Bootstrapping a monorepo with Turborepo addresses many common development pain points, from dependency management to build performance. While some developers express concerns about betting on new tools, Turborepo's backing by Vercel and its growing community support make it a solid choice for modern JavaScript projects.
Remember:
Start with a clear structure
Implement strict dependency management
Optimize your build pipeline
Set up proper CI/CD integration early
By following these guidelines, you'll avoid the "nightmare" of poorly organized monorepos and create a development environment that scales with your team and project needs.