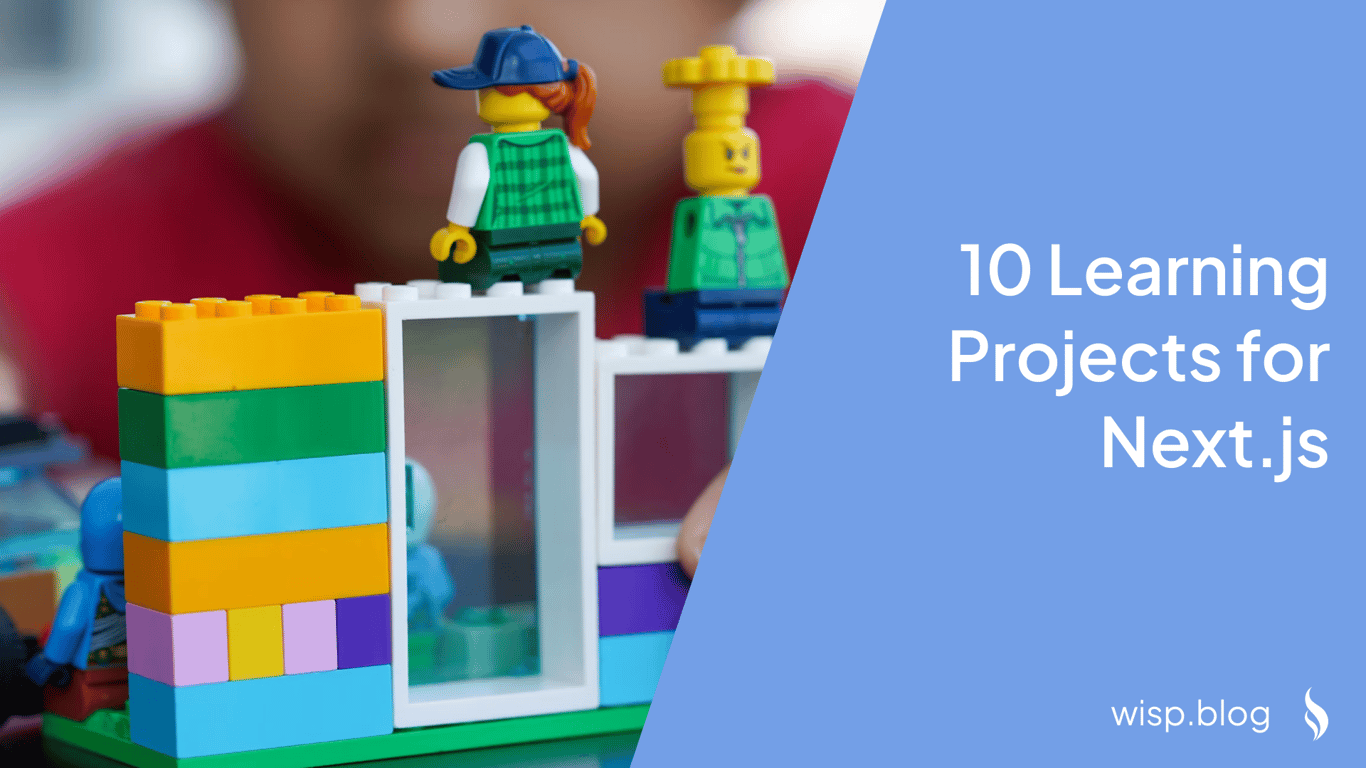
Are you tired of building the same old to-do apps and Reddit clones while learning Next.js? You're not alone. Many developers express frustration that common beginner projects don't push them beyond basic CRUD operations. While these fundamentals are important, there's a growing need for projects that challenge you to think like a real developer and problem solver.
In this comprehensive guide, we'll explore 10 carefully selected Next.js projects that will help you progress from basics to advanced concepts, each designed to teach specific skills and overcome common learning challenges.
Why These Projects Matter
Before diving into the projects, let's understand why carefully chosen learning projects are crucial:
They force you to think beyond basic data fetching and display
They help you understand real-world application architecture
They build problem-solving skills needed for complex applications
They create portfolio pieces that demonstrate your capabilities
They help you retain knowledge better than following tutorials
Project Selection Criteria
Each project in this guide has been selected based on:
Practical Value: Projects that solve real problems or create useful applications
Skill Progression: Each project builds upon previous learning
Modern Features: Utilization of Next.js 14's latest features
Complexity Balance: Challenging enough to learn from, but not overwhelming
Portfolio Potential: Projects that showcase your abilities to potential employers
1. Personal Portfolio Website
Difficulty: BeginnerTime Estimate: 1 weekCore Concepts: Routing, Static Generation, Image Optimization
Function
Create a professional portfolio website to showcase your work, skills, and achievements. This project serves as an excellent introduction to Next.js while resulting in a practical asset for your career.
Key Features to Implement
Responsive layout using modern CSS techniques
Dynamic project showcase with filtering capabilities
Contact form with email integration
Blog section with MDX support
SEO optimization using Next.js metadata
Image optimization using Next.js Image component
Learning Outcomes
Understanding of Next.js file-based routing
Implementation of static site generation (SSG)
Experience with component architecture
Mastery of image optimization techniques
Integration of third-party services
Deployment workflows with Vercel
Development Tips
Start with a simple single-page design and progressively add features. Focus on mastering the basics of Next.js routing and component structure before moving on to more advanced features like MDX integration or dynamic content loading.
2. E-commerce Store
Difficulty: IntermediateTime Estimate: 2-3 weeksCore Concepts: API Routes, Authentication, Payment Integration
Function
Build a fully functional online store that allows users to browse products, add items to cart, and complete purchases. This project introduces you to essential e-commerce functionalities and real-world application architecture.
Key Features to Implement
Product catalog with search and filtering
Shopping cart functionality with local storage
User authentication and account management
Secure payment processing with Stripe
Order management system
Admin dashboard for inventory management
Learning Outcomes
Managing complex state across components
Implementing secure authentication flows
Working with external APIs and payment gateways
Handling form validation and user input
Creating protected routes and admin areas
Understanding data persistence strategies
Development Tips
Begin with a basic product listing and gradually add features like cart functionality and user authentication. Use a headless CMS or mock API initially to focus on the frontend implementation before tackling backend integration.
3. Blog Platform with CMS
Difficulty: IntermediateTime Estimate: 1-2 weeksCore Concepts: Content Management, Markdown Processing, Dynamic Routing
Function
Develop a full-featured blog platform that supports content creation, editing, and publishing. This project helps you understand content management systems and dynamic content rendering.
Key Features to Implement
Markdown/MDX support for content writing
Category and tag management
Search functionality
Comment system
Social sharing integration
RSS feed generation
Analytics dashboard
Learning Outcomes
Working with markdown and rich text content
Implementing dynamic routing strategies
Managing user-generated content
Optimizing for SEO
Creating reusable content components
Understanding content caching strategies
Development Tips
Start by implementing basic post creation and rendering. Then progressively add features like comments, categories, and search functionality. Consider using a headless CMS like Sanity or Contentful for content management.
4. Dashboard Application
Difficulty: Intermediate to AdvancedTime Estimate: 2 weeksCore Concepts: Data Visualization, Real-time Updates, State Management
Function
Create a comprehensive analytics dashboard that visualizes data through various charts, graphs, and interactive elements. This project teaches you about handling complex data structures and creating intuitive user interfaces.
Key Features to Implement
Multiple chart types (line, bar, pie charts)
Real-time data updates
Customizable widgets
Data filtering and date range selection
Export functionality
User preferences saving
Responsive layout for different screen sizes
Learning Outcomes
Integration with charting libraries
Implementing real-time updates with WebSocket
Managing complex application state
Creating reusable dashboard components
Handling large datasets efficiently
Implementing user customization features
Development Tips
Begin with static data visualization and gradually implement real-time updates. Focus on creating a modular architecture that allows easy addition of new widget types and data sources.
5. Social Media Application
Difficulty: AdvancedTime Estimate: 3 weeksCore Concepts: Real-time Communication, File Upload, Social Features
Function
Build a social networking platform that allows users to connect, share content, and interact with each other. This project introduces you to complex user interactions and real-time features.
Key Features to Implement
User profiles and connections
Post creation with media upload
News feed with infinite scroll
Real-time notifications
Direct messaging system
Like and comment functionality
Follow/unfollow mechanics
Learning Outcomes
Implementing real-time features with WebSocket
Managing complex user relationships
Handling file uploads and media processing
Creating engaging user interfaces
Implementing infinite scroll and pagination
Understanding social graph data structures
Development Tips
Start with basic user profiles and posting functionality, then gradually add social features like following and notifications. Consider using technologies like Socket.io for real-time features and Cloudinary for media management.
6. Documentation Site
Difficulty: IntermediateTime Estimate: 1 weekCore Concepts: Static Site Generation, Search, Navigation
Function
Create a documentation website that effectively organizes and presents technical information. This project helps you understand content organization and static site generation.
Key Features to Implement
Hierarchical navigation structure
Full-text search functionality
Syntax highlighting for code blocks
Dark/light theme switching
Version control for documentation
API documentation generator
Interactive code examples
Learning Outcomes
Mastering static site generation
Implementing search functionality
Creating accessible navigation systems
Managing documentation versions
Optimizing for developer experience
Understanding content organization
Development Tips
Begin with a basic documentation structure and gradually add features like search and versioning. Consider using MDX for content management and Algolia for search functionality.
7. Progressive Web App (PWA)
Difficulty: AdvancedTime Estimate: 2 weeksCore Concepts: Service Workers, Offline Functionality, Push Notifications
Function
Develop a progressive web application that works offline and provides a native app-like experience. This project teaches you about modern web capabilities and progressive enhancement.
Key Features to Implement
Offline functionality
Push notifications
App shell architecture
Background sync
Home screen installation
Responsive design
Performance optimization
Learning Outcomes
Understanding service workers
Implementing caching strategies
Managing push notifications
Creating responsive interfaces
Optimizing performance
Handling offline states
Development Tips
Start with a basic web app and progressively enhance it with PWA features. Focus on creating a smooth offline experience before adding more advanced features like push notifications.
8. Real-time Chat Application
Difficulty: AdvancedTime Estimate: 2 weeksCore Concepts: WebSocket, Real-time Updates, User Presence
Function
Build a real-time chat application that enables instant messaging between users. This project introduces you to real-time communication and state management.
Key Features to Implement
Private and group chat functionality
Real-time message updates
User presence indicators
Message read receipts
File sharing capabilities
Emoji support
Message search and history
Learning Outcomes
Working with WebSocket connections
Managing real-time state updates
Implementing user presence
Handling file uploads
Creating responsive chat interfaces
Understanding real-time data flow
Development Tips
Begin with basic one-on-one chat functionality and gradually add features like group chats and file sharing. Consider using Socket.io or LiveKit for real-time communication.
9. Job Board Platform
Difficulty: Intermediate to AdvancedTime Estimate: 1-2 weeksCore Concepts: Search, Filtering, Payment Integration
Function
Create a job board platform where employers can post jobs and job seekers can search and apply for positions. This project helps you understand search functionality and user roles.
Key Features to Implement
Job posting and management
Advanced search and filtering
Application tracking system
Company profiles
Resume upload and parsing
Email notifications
Payment integration for job posts
Learning Outcomes
Implementing search and filtering
Managing different user roles
Handling file uploads and processing
Creating email notification systems
Integrating payment processing
Building complex forms
Development Tips
Start with basic job listing functionality and gradually add features like search, applications, and payments. Consider using Elasticsearch for search functionality and Stripe for payments.
10. Recipe Application
Difficulty: IntermediateTime Estimate: 1 weekCore Concepts: Content Management, Search, User Interaction
Function
Build a recipe sharing platform where users can discover, share, and save recipes. This project combines content management with social features.
Key Features to Implement
Recipe creation and management
Ingredient database
Cooking timer functionality
Recipe scaling calculator
Meal planning calendar
Shopping list generator
Nutritional information display
Learning Outcomes
Managing structured content
Implementing search and filtering
Creating interactive components
Handling complex calculations
Building user preference systems
Understanding data relationships
Development Tips
Begin with basic recipe management and gradually add features like meal planning and shopping lists. Consider using a nutrition API for detailed ingredient information.
Best Practices for Project Development
When working on these projects, keep these best practices in mind:
Start Small, Iterate Often
Begin with core functionality
Add features incrementally
Test thoroughly as you build
Use Modern Development Tools
Implement TypeScript for better type safety
Use ESLint and Prettier for code quality
Leverage Next.js's built-in optimizations
Focus on User Experience
Ensure responsive design
Implement loading states
Add error handling
Optimize performance
Document Your Work
Write clear documentation
Comment complex logic
Create a detailed README
Include setup instructions
Version Control Best Practices
Make regular commits
Use meaningful commit messages
Create feature branches
Review your own code
Learning Resources and Support
To help you succeed with these projects, here are some valuable resources:
Official Documentation
Next.js Documentation - Your primary source for Next.js features and APIs
React Documentation - For underlying React concepts
Vercel Documentation - For deployment and hosting
Community Support
Additional Learning Materials
Conclusion
The journey from basic to advanced Next.js development doesn't have to be filled with repetitive to-do apps and blog clones. By tackling these diverse projects, you'll:
Build real-world problem-solving skills
Create meaningful portfolio pieces
Understand complex application architecture
Master modern web development practices
Develop confidence in your abilities
Remember, as one developer noted, "you need to build something in order to really 'get it.'" These projects provide the perfect opportunity to do just that.
Start with projects that match your current skill level and gradually work your way up to more complex applications. Don't be afraid to modify these project ideas to solve problems you're passionate about or to meet specific learning goals.
The key is to move beyond tutorial hell and start building real applications. Each project will present its own challenges and learning opportunities, helping you grow into a more confident and capable Next.js developer.
Happy coding!